Trigger window.open as ajax complete
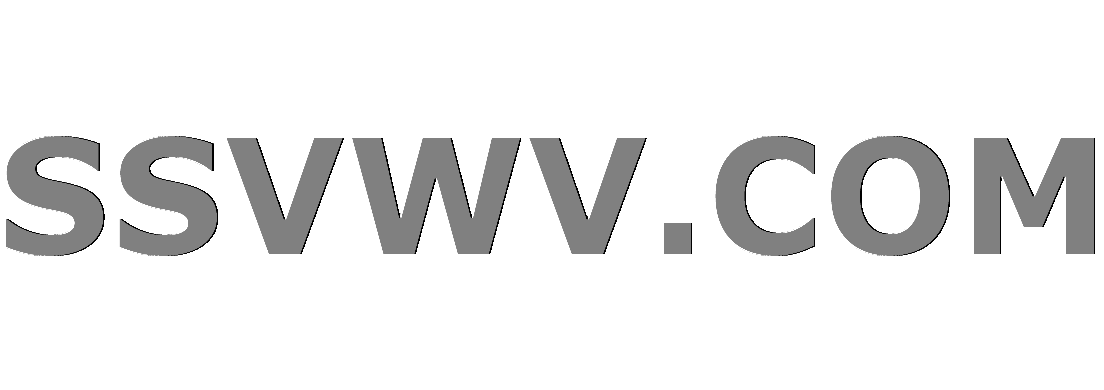
Multi tool use
up vote
1
down vote
favorite
In a website there are a some jQuery ajax calls, in some of them, if success, is triggered a winwdow.open in another domain. I own the other domain sites.
For example:
- site https://site1.example.com make on ajax call on itself
- if success trigger a window.open on https://site1.example2.com or https://site2.example.com
- often the popup blocker blocks the operation
How can I handle this issue? Do I have to enable CORS on my site/server https://enable-cors.org/ ?
Code (sure, I don't own google):
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
window.open('https://www.google.com');
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
Demo: https://jsfiddle.net/IrvinDominin/nbsu3hgk/
javascript cors window.open
add a comment |
up vote
1
down vote
favorite
In a website there are a some jQuery ajax calls, in some of them, if success, is triggered a winwdow.open in another domain. I own the other domain sites.
For example:
- site https://site1.example.com make on ajax call on itself
- if success trigger a window.open on https://site1.example2.com or https://site2.example.com
- often the popup blocker blocks the operation
How can I handle this issue? Do I have to enable CORS on my site/server https://enable-cors.org/ ?
Code (sure, I don't own google):
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
window.open('https://www.google.com');
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
Demo: https://jsfiddle.net/IrvinDominin/nbsu3hgk/
javascript cors window.open
When I allow pop-ups in the browser this always works. Tested with Firefox and Chromium.
– D. Joe
2 days ago
I know, but, if possible I have to handle this without browser options or it produce some help desk on my users
– Irvin Dominin
2 days ago
Have a look here: stackoverflow.com/a/2587692/2412335
– D. Joe
2 days ago
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
In a website there are a some jQuery ajax calls, in some of them, if success, is triggered a winwdow.open in another domain. I own the other domain sites.
For example:
- site https://site1.example.com make on ajax call on itself
- if success trigger a window.open on https://site1.example2.com or https://site2.example.com
- often the popup blocker blocks the operation
How can I handle this issue? Do I have to enable CORS on my site/server https://enable-cors.org/ ?
Code (sure, I don't own google):
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
window.open('https://www.google.com');
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
Demo: https://jsfiddle.net/IrvinDominin/nbsu3hgk/
javascript cors window.open
In a website there are a some jQuery ajax calls, in some of them, if success, is triggered a winwdow.open in another domain. I own the other domain sites.
For example:
- site https://site1.example.com make on ajax call on itself
- if success trigger a window.open on https://site1.example2.com or https://site2.example.com
- often the popup blocker blocks the operation
How can I handle this issue? Do I have to enable CORS on my site/server https://enable-cors.org/ ?
Code (sure, I don't own google):
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
window.open('https://www.google.com');
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
Demo: https://jsfiddle.net/IrvinDominin/nbsu3hgk/
javascript cors window.open
javascript cors window.open
asked 2 days ago


Irvin Dominin
27.3k85490
27.3k85490
When I allow pop-ups in the browser this always works. Tested with Firefox and Chromium.
– D. Joe
2 days ago
I know, but, if possible I have to handle this without browser options or it produce some help desk on my users
– Irvin Dominin
2 days ago
Have a look here: stackoverflow.com/a/2587692/2412335
– D. Joe
2 days ago
add a comment |
When I allow pop-ups in the browser this always works. Tested with Firefox and Chromium.
– D. Joe
2 days ago
I know, but, if possible I have to handle this without browser options or it produce some help desk on my users
– Irvin Dominin
2 days ago
Have a look here: stackoverflow.com/a/2587692/2412335
– D. Joe
2 days ago
When I allow pop-ups in the browser this always works. Tested with Firefox and Chromium.
– D. Joe
2 days ago
When I allow pop-ups in the browser this always works. Tested with Firefox and Chromium.
– D. Joe
2 days ago
I know, but, if possible I have to handle this without browser options or it produce some help desk on my users
– Irvin Dominin
2 days ago
I know, but, if possible I have to handle this without browser options or it produce some help desk on my users
– Irvin Dominin
2 days ago
Have a look here: stackoverflow.com/a/2587692/2412335
– D. Joe
2 days ago
Have a look here: stackoverflow.com/a/2587692/2412335
– D. Joe
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
Because window.open
requires direct user action (e. g. a click) to avoid popup-blockers, you could change your code like this:
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
//window.open('https://www.google.com');
$( "body" ).append('<button id="openPopup">Open Site</button>');
$( "#openPopup" ).click( function() {
window.open('https://www.google.com');
});
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
If the ajax request is successful, users are shown a button which they have to click to get to the other domain.
Edit: Referring to your comment below I think I found a solution:
var myPopup;
$( document ).on("click", "#myButton", function() {
myPopup = window.open("/");
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
//window.open('https://www.google.com');
myPopup.location.href = 'https://www.google.com';
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
});
You have to declare a global variable for your new window first. When your button that triggers the ajax request is clicked you open a new window that is referencing to your current domain (https://site1.example.com/ in your case). This is accepted as direct user action, so no popup blocker comes into effect.
In the complete
function you redirect that window to one of the other domains (https://site1.example2.com or https://site2.example.com), et voilà. I've tested it with activated popup blocker and it worked.
Is triggered by a click on a button, that fires the Ajax call
– Irvin Dominin
2 days ago
I guess that the click on that button is not considered a direct user action by browsers for thewindow.open
in the 'complete` function of the ajax request. The code above at least works.
– D. Joe
2 days ago
I've edited my code above to suit your needs.
– D. Joe
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Because window.open
requires direct user action (e. g. a click) to avoid popup-blockers, you could change your code like this:
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
//window.open('https://www.google.com');
$( "body" ).append('<button id="openPopup">Open Site</button>');
$( "#openPopup" ).click( function() {
window.open('https://www.google.com');
});
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
If the ajax request is successful, users are shown a button which they have to click to get to the other domain.
Edit: Referring to your comment below I think I found a solution:
var myPopup;
$( document ).on("click", "#myButton", function() {
myPopup = window.open("/");
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
//window.open('https://www.google.com');
myPopup.location.href = 'https://www.google.com';
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
});
You have to declare a global variable for your new window first. When your button that triggers the ajax request is clicked you open a new window that is referencing to your current domain (https://site1.example.com/ in your case). This is accepted as direct user action, so no popup blocker comes into effect.
In the complete
function you redirect that window to one of the other domains (https://site1.example2.com or https://site2.example.com), et voilà. I've tested it with activated popup blocker and it worked.
Is triggered by a click on a button, that fires the Ajax call
– Irvin Dominin
2 days ago
I guess that the click on that button is not considered a direct user action by browsers for thewindow.open
in the 'complete` function of the ajax request. The code above at least works.
– D. Joe
2 days ago
I've edited my code above to suit your needs.
– D. Joe
2 days ago
add a comment |
up vote
0
down vote
Because window.open
requires direct user action (e. g. a click) to avoid popup-blockers, you could change your code like this:
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
//window.open('https://www.google.com');
$( "body" ).append('<button id="openPopup">Open Site</button>');
$( "#openPopup" ).click( function() {
window.open('https://www.google.com');
});
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
If the ajax request is successful, users are shown a button which they have to click to get to the other domain.
Edit: Referring to your comment below I think I found a solution:
var myPopup;
$( document ).on("click", "#myButton", function() {
myPopup = window.open("/");
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
//window.open('https://www.google.com');
myPopup.location.href = 'https://www.google.com';
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
});
You have to declare a global variable for your new window first. When your button that triggers the ajax request is clicked you open a new window that is referencing to your current domain (https://site1.example.com/ in your case). This is accepted as direct user action, so no popup blocker comes into effect.
In the complete
function you redirect that window to one of the other domains (https://site1.example2.com or https://site2.example.com), et voilà. I've tested it with activated popup blocker and it worked.
Is triggered by a click on a button, that fires the Ajax call
– Irvin Dominin
2 days ago
I guess that the click on that button is not considered a direct user action by browsers for thewindow.open
in the 'complete` function of the ajax request. The code above at least works.
– D. Joe
2 days ago
I've edited my code above to suit your needs.
– D. Joe
2 days ago
add a comment |
up vote
0
down vote
up vote
0
down vote
Because window.open
requires direct user action (e. g. a click) to avoid popup-blockers, you could change your code like this:
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
//window.open('https://www.google.com');
$( "body" ).append('<button id="openPopup">Open Site</button>');
$( "#openPopup" ).click( function() {
window.open('https://www.google.com');
});
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
If the ajax request is successful, users are shown a button which they have to click to get to the other domain.
Edit: Referring to your comment below I think I found a solution:
var myPopup;
$( document ).on("click", "#myButton", function() {
myPopup = window.open("/");
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
//window.open('https://www.google.com');
myPopup.location.href = 'https://www.google.com';
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
});
You have to declare a global variable for your new window first. When your button that triggers the ajax request is clicked you open a new window that is referencing to your current domain (https://site1.example.com/ in your case). This is accepted as direct user action, so no popup blocker comes into effect.
In the complete
function you redirect that window to one of the other domains (https://site1.example2.com or https://site2.example.com), et voilà. I've tested it with activated popup blocker and it worked.
Because window.open
requires direct user action (e. g. a click) to avoid popup-blockers, you could change your code like this:
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
//window.open('https://www.google.com');
$( "body" ).append('<button id="openPopup">Open Site</button>');
$( "#openPopup" ).click( function() {
window.open('https://www.google.com');
});
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
If the ajax request is successful, users are shown a button which they have to click to get to the other domain.
Edit: Referring to your comment below I think I found a solution:
var myPopup;
$( document ).on("click", "#myButton", function() {
myPopup = window.open("/");
$.ajax({
url:'/echo/js/?js=hello%20world!',
complete: function (response) {
$('#output').html(response.responseText);
//window.open('https://www.google.com');
myPopup.location.href = 'https://www.google.com';
},
error: function () {
$('#output').html('Bummer: there was an error!');
},
});
});
You have to declare a global variable for your new window first. When your button that triggers the ajax request is clicked you open a new window that is referencing to your current domain (https://site1.example.com/ in your case). This is accepted as direct user action, so no popup blocker comes into effect.
In the complete
function you redirect that window to one of the other domains (https://site1.example2.com or https://site2.example.com), et voilà. I've tested it with activated popup blocker and it worked.
edited 2 days ago
answered 2 days ago


D. Joe
12910
12910
Is triggered by a click on a button, that fires the Ajax call
– Irvin Dominin
2 days ago
I guess that the click on that button is not considered a direct user action by browsers for thewindow.open
in the 'complete` function of the ajax request. The code above at least works.
– D. Joe
2 days ago
I've edited my code above to suit your needs.
– D. Joe
2 days ago
add a comment |
Is triggered by a click on a button, that fires the Ajax call
– Irvin Dominin
2 days ago
I guess that the click on that button is not considered a direct user action by browsers for thewindow.open
in the 'complete` function of the ajax request. The code above at least works.
– D. Joe
2 days ago
I've edited my code above to suit your needs.
– D. Joe
2 days ago
Is triggered by a click on a button, that fires the Ajax call
– Irvin Dominin
2 days ago
Is triggered by a click on a button, that fires the Ajax call
– Irvin Dominin
2 days ago
I guess that the click on that button is not considered a direct user action by browsers for the
window.open
in the 'complete` function of the ajax request. The code above at least works.– D. Joe
2 days ago
I guess that the click on that button is not considered a direct user action by browsers for the
window.open
in the 'complete` function of the ajax request. The code above at least works.– D. Joe
2 days ago
I've edited my code above to suit your needs.
– D. Joe
2 days ago
I've edited my code above to suit your needs.
– D. Joe
2 days ago
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53238309%2ftrigger-window-open-as-ajax-complete%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
PkFLniM FstDIAnR 4
When I allow pop-ups in the browser this always works. Tested with Firefox and Chromium.
– D. Joe
2 days ago
I know, but, if possible I have to handle this without browser options or it produce some help desk on my users
– Irvin Dominin
2 days ago
Have a look here: stackoverflow.com/a/2587692/2412335
– D. Joe
2 days ago