Python finding words in file based on passed value
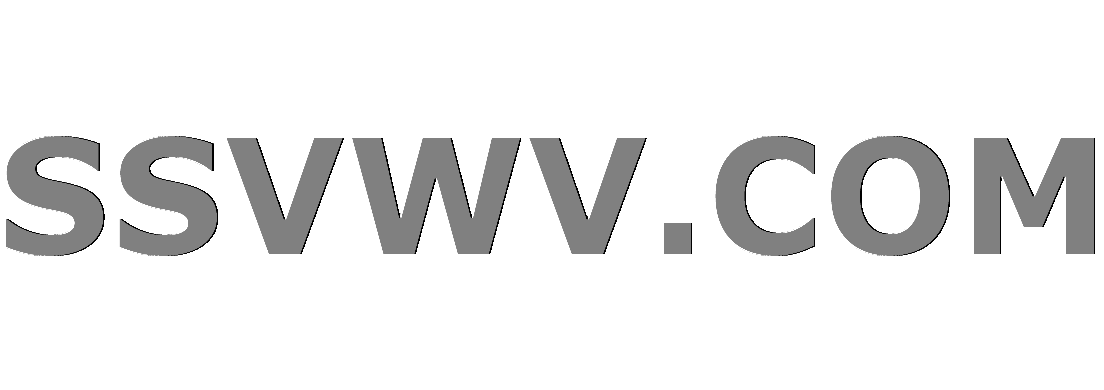
Multi tool use
up vote
0
down vote
favorite
I want to create simply method, where programm will find word or words in file, based on passed value from command line.
class Option():
SCRABBLES_SCORES = [(1, "E A O I N R T L S U"), (2, "D G"), (3, "B C M P"),
(4, "F H V W Y"), (5, "K"), (8, "J X"), (10, "Q Z")]
global LETTER_SCORES
LETTER_SCORES = {letter: score for score, letters in SCRABBLES_SCORES
for letter in letters.split()}
def word_from_score(self,score):
file = [line.rstrip('n') for line in open('dictionary.txt', "r")]
For example, if I run python main.py -s 7 it will return KOT. I know how to create argparse, but have problem with finding words and adding them to list
[EDIT]
I wrote something like this:
def word_from_score(self,score):
file = [line.rstrip('n') for line in open('dictionary.txt', "r")]
return [word for word in file if sum([LETTER_SCORES[letter] for letter in word ]) == score]
python
add a comment |
up vote
0
down vote
favorite
I want to create simply method, where programm will find word or words in file, based on passed value from command line.
class Option():
SCRABBLES_SCORES = [(1, "E A O I N R T L S U"), (2, "D G"), (3, "B C M P"),
(4, "F H V W Y"), (5, "K"), (8, "J X"), (10, "Q Z")]
global LETTER_SCORES
LETTER_SCORES = {letter: score for score, letters in SCRABBLES_SCORES
for letter in letters.split()}
def word_from_score(self,score):
file = [line.rstrip('n') for line in open('dictionary.txt', "r")]
For example, if I run python main.py -s 7 it will return KOT. I know how to create argparse, but have problem with finding words and adding them to list
[EDIT]
I wrote something like this:
def word_from_score(self,score):
file = [line.rstrip('n') for line in open('dictionary.txt', "r")]
return [word for word in file if sum([LETTER_SCORES[letter] for letter in word ]) == score]
python
To make sure I understand it right, your problem is that you have a list for valid words read (correctly without trouble) from your file, and you are now looking for a way to check which of those words can be created with a set of letters passed via command-line?
– Henning Koehler
2 days ago
@Henning Koehler not really. I got file with words. Now from command line, I want to write python main.py -s 7. 7 is a score I want to find in file. For example KOT return value 7. And I want to do opposite. I want to write 7 as argument and get KOT as result or other words I have in file and sum of characters based on LETTER_SCORES is equal to passed argument
– Frendom
2 days ago
So you are looking for a way to compute the sum of char values of the words parsed so that you can compare it to the target score?
– Henning Koehler
2 days ago
Exactly. And in file can be a lot of words that sum of letters is equal to my target from command line, so I want all of them store in list
– Frendom
2 days ago
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I want to create simply method, where programm will find word or words in file, based on passed value from command line.
class Option():
SCRABBLES_SCORES = [(1, "E A O I N R T L S U"), (2, "D G"), (3, "B C M P"),
(4, "F H V W Y"), (5, "K"), (8, "J X"), (10, "Q Z")]
global LETTER_SCORES
LETTER_SCORES = {letter: score for score, letters in SCRABBLES_SCORES
for letter in letters.split()}
def word_from_score(self,score):
file = [line.rstrip('n') for line in open('dictionary.txt', "r")]
For example, if I run python main.py -s 7 it will return KOT. I know how to create argparse, but have problem with finding words and adding them to list
[EDIT]
I wrote something like this:
def word_from_score(self,score):
file = [line.rstrip('n') for line in open('dictionary.txt', "r")]
return [word for word in file if sum([LETTER_SCORES[letter] for letter in word ]) == score]
python
I want to create simply method, where programm will find word or words in file, based on passed value from command line.
class Option():
SCRABBLES_SCORES = [(1, "E A O I N R T L S U"), (2, "D G"), (3, "B C M P"),
(4, "F H V W Y"), (5, "K"), (8, "J X"), (10, "Q Z")]
global LETTER_SCORES
LETTER_SCORES = {letter: score for score, letters in SCRABBLES_SCORES
for letter in letters.split()}
def word_from_score(self,score):
file = [line.rstrip('n') for line in open('dictionary.txt', "r")]
For example, if I run python main.py -s 7 it will return KOT. I know how to create argparse, but have problem with finding words and adding them to list
[EDIT]
I wrote something like this:
def word_from_score(self,score):
file = [line.rstrip('n') for line in open('dictionary.txt', "r")]
return [word for word in file if sum([LETTER_SCORES[letter] for letter in word ]) == score]
python
python
edited 2 days ago
asked 2 days ago
Frendom
195
195
To make sure I understand it right, your problem is that you have a list for valid words read (correctly without trouble) from your file, and you are now looking for a way to check which of those words can be created with a set of letters passed via command-line?
– Henning Koehler
2 days ago
@Henning Koehler not really. I got file with words. Now from command line, I want to write python main.py -s 7. 7 is a score I want to find in file. For example KOT return value 7. And I want to do opposite. I want to write 7 as argument and get KOT as result or other words I have in file and sum of characters based on LETTER_SCORES is equal to passed argument
– Frendom
2 days ago
So you are looking for a way to compute the sum of char values of the words parsed so that you can compare it to the target score?
– Henning Koehler
2 days ago
Exactly. And in file can be a lot of words that sum of letters is equal to my target from command line, so I want all of them store in list
– Frendom
2 days ago
add a comment |
To make sure I understand it right, your problem is that you have a list for valid words read (correctly without trouble) from your file, and you are now looking for a way to check which of those words can be created with a set of letters passed via command-line?
– Henning Koehler
2 days ago
@Henning Koehler not really. I got file with words. Now from command line, I want to write python main.py -s 7. 7 is a score I want to find in file. For example KOT return value 7. And I want to do opposite. I want to write 7 as argument and get KOT as result or other words I have in file and sum of characters based on LETTER_SCORES is equal to passed argument
– Frendom
2 days ago
So you are looking for a way to compute the sum of char values of the words parsed so that you can compare it to the target score?
– Henning Koehler
2 days ago
Exactly. And in file can be a lot of words that sum of letters is equal to my target from command line, so I want all of them store in list
– Frendom
2 days ago
To make sure I understand it right, your problem is that you have a list for valid words read (correctly without trouble) from your file, and you are now looking for a way to check which of those words can be created with a set of letters passed via command-line?
– Henning Koehler
2 days ago
To make sure I understand it right, your problem is that you have a list for valid words read (correctly without trouble) from your file, and you are now looking for a way to check which of those words can be created with a set of letters passed via command-line?
– Henning Koehler
2 days ago
@Henning Koehler not really. I got file with words. Now from command line, I want to write python main.py -s 7. 7 is a score I want to find in file. For example KOT return value 7. And I want to do opposite. I want to write 7 as argument and get KOT as result or other words I have in file and sum of characters based on LETTER_SCORES is equal to passed argument
– Frendom
2 days ago
@Henning Koehler not really. I got file with words. Now from command line, I want to write python main.py -s 7. 7 is a score I want to find in file. For example KOT return value 7. And I want to do opposite. I want to write 7 as argument and get KOT as result or other words I have in file and sum of characters based on LETTER_SCORES is equal to passed argument
– Frendom
2 days ago
So you are looking for a way to compute the sum of char values of the words parsed so that you can compare it to the target score?
– Henning Koehler
2 days ago
So you are looking for a way to compute the sum of char values of the words parsed so that you can compare it to the target score?
– Henning Koehler
2 days ago
Exactly. And in file can be a lot of words that sum of letters is equal to my target from command line, so I want all of them store in list
– Frendom
2 days ago
Exactly. And in file can be a lot of words that sum of letters is equal to my target from command line, so I want all of them store in list
– Frendom
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
List comprehension is your friend here. To compute the score of a word:
def scoreOf(word):
return sum( [ LETTER_SCORES[letter] for letter in word ] )
and to filter out all the matching words:
def wordsWithScore(allWords, targetScore):
return [ word for word in allWords if scoreOf(word) == targetScore ]
Or if you really want to, you can even combine them into a one-liner:
return [ word for word in allWords
if sum( [ LETTER_SCORES[letter] for letter in word ] ) == targetScore ]
I changed my first post.
– Frendom
2 days ago
So if that is working now, then please accept the answer. Otherwise you'll need to clarify what it is you are looking for.
– Henning Koehler
2 days ago
Yeah, I will do that if everything work fine. But I want these selected words add do list and return only 1, based on random
– Frendom
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
List comprehension is your friend here. To compute the score of a word:
def scoreOf(word):
return sum( [ LETTER_SCORES[letter] for letter in word ] )
and to filter out all the matching words:
def wordsWithScore(allWords, targetScore):
return [ word for word in allWords if scoreOf(word) == targetScore ]
Or if you really want to, you can even combine them into a one-liner:
return [ word for word in allWords
if sum( [ LETTER_SCORES[letter] for letter in word ] ) == targetScore ]
I changed my first post.
– Frendom
2 days ago
So if that is working now, then please accept the answer. Otherwise you'll need to clarify what it is you are looking for.
– Henning Koehler
2 days ago
Yeah, I will do that if everything work fine. But I want these selected words add do list and return only 1, based on random
– Frendom
2 days ago
add a comment |
up vote
0
down vote
accepted
List comprehension is your friend here. To compute the score of a word:
def scoreOf(word):
return sum( [ LETTER_SCORES[letter] for letter in word ] )
and to filter out all the matching words:
def wordsWithScore(allWords, targetScore):
return [ word for word in allWords if scoreOf(word) == targetScore ]
Or if you really want to, you can even combine them into a one-liner:
return [ word for word in allWords
if sum( [ LETTER_SCORES[letter] for letter in word ] ) == targetScore ]
I changed my first post.
– Frendom
2 days ago
So if that is working now, then please accept the answer. Otherwise you'll need to clarify what it is you are looking for.
– Henning Koehler
2 days ago
Yeah, I will do that if everything work fine. But I want these selected words add do list and return only 1, based on random
– Frendom
2 days ago
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
List comprehension is your friend here. To compute the score of a word:
def scoreOf(word):
return sum( [ LETTER_SCORES[letter] for letter in word ] )
and to filter out all the matching words:
def wordsWithScore(allWords, targetScore):
return [ word for word in allWords if scoreOf(word) == targetScore ]
Or if you really want to, you can even combine them into a one-liner:
return [ word for word in allWords
if sum( [ LETTER_SCORES[letter] for letter in word ] ) == targetScore ]
List comprehension is your friend here. To compute the score of a word:
def scoreOf(word):
return sum( [ LETTER_SCORES[letter] for letter in word ] )
and to filter out all the matching words:
def wordsWithScore(allWords, targetScore):
return [ word for word in allWords if scoreOf(word) == targetScore ]
Or if you really want to, you can even combine them into a one-liner:
return [ word for word in allWords
if sum( [ LETTER_SCORES[letter] for letter in word ] ) == targetScore ]
answered 2 days ago
Henning Koehler
1,080510
1,080510
I changed my first post.
– Frendom
2 days ago
So if that is working now, then please accept the answer. Otherwise you'll need to clarify what it is you are looking for.
– Henning Koehler
2 days ago
Yeah, I will do that if everything work fine. But I want these selected words add do list and return only 1, based on random
– Frendom
2 days ago
add a comment |
I changed my first post.
– Frendom
2 days ago
So if that is working now, then please accept the answer. Otherwise you'll need to clarify what it is you are looking for.
– Henning Koehler
2 days ago
Yeah, I will do that if everything work fine. But I want these selected words add do list and return only 1, based on random
– Frendom
2 days ago
I changed my first post.
– Frendom
2 days ago
I changed my first post.
– Frendom
2 days ago
So if that is working now, then please accept the answer. Otherwise you'll need to clarify what it is you are looking for.
– Henning Koehler
2 days ago
So if that is working now, then please accept the answer. Otherwise you'll need to clarify what it is you are looking for.
– Henning Koehler
2 days ago
Yeah, I will do that if everything work fine. But I want these selected words add do list and return only 1, based on random
– Frendom
2 days ago
Yeah, I will do that if everything work fine. But I want these selected words add do list and return only 1, based on random
– Frendom
2 days ago
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53238471%2fpython-finding-words-in-file-based-on-passed-value%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
sIp4,Jg,nYcwG,lGwi cQPT7VW0Cc81BC MH,3
To make sure I understand it right, your problem is that you have a list for valid words read (correctly without trouble) from your file, and you are now looking for a way to check which of those words can be created with a set of letters passed via command-line?
– Henning Koehler
2 days ago
@Henning Koehler not really. I got file with words. Now from command line, I want to write python main.py -s 7. 7 is a score I want to find in file. For example KOT return value 7. And I want to do opposite. I want to write 7 as argument and get KOT as result or other words I have in file and sum of characters based on LETTER_SCORES is equal to passed argument
– Frendom
2 days ago
So you are looking for a way to compute the sum of char values of the words parsed so that you can compare it to the target score?
– Henning Koehler
2 days ago
Exactly. And in file can be a lot of words that sum of letters is equal to my target from command line, so I want all of them store in list
– Frendom
2 days ago