OpenCV: Assertion failed “ dst.data == widget->original_image->data.ptr in function...
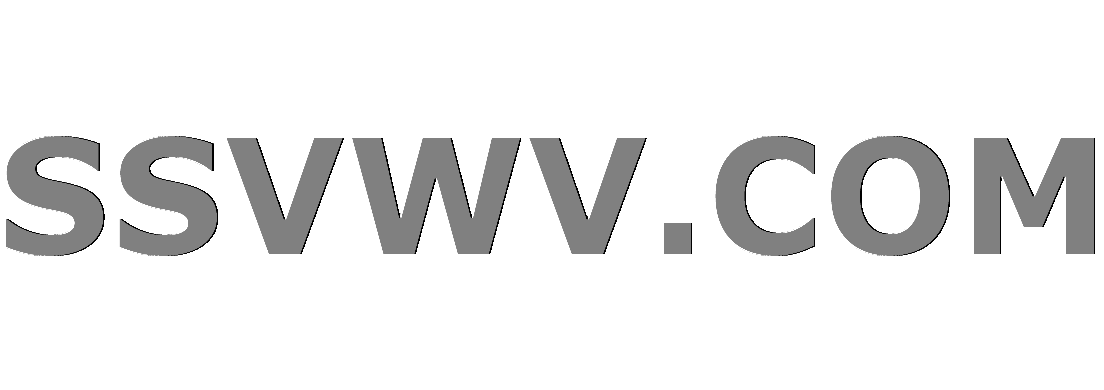
Multi tool use
IMPORTANT: I fixed the problem. Solution at the end.
What do I try to achieve?
Display an image with OpenCV cv::imshow method. (imshow Documentation)
The image which is a 3x3 matrix is created like such:
Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, -4, 1, 0, 1, 0});
To display the image I call imshow("mask", mask);
What is my problem?
Like I mentioned in the title there is an exception thrown while trying to display the image. Complete Error Message:
terminate called after throwing an instance of 'cv::Exception' what():
OpenCV(4.0.0-pre) /home/mrlab/Libraries/opencv_source/modules/highgui
/src/window_gtk.cpp:146: error: (-215:Assertion failed)
dst.data == widget->original_image->data.ptr in function 'cvImageWidgetSetImage'
Link to window_gtk.cpp
What did I already try?
- Looking for the error on the internet. Maybe someone else already encountered the same problem. nope. nothing
- Changed the matrix to only contain positive floating point values (0 to 1) in case it has problems with negative input. Initialization:
Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, 0, 1, 0, 1, 0});
same error
- Calling the two methods in different locations in case there are changes made during my other code. same error
- Writing small OpenCV program to just run these two lines. same error
- Various combinations of the above mentioned ideas. same error
- Displaying other images I read from memory instead of creating them myself. worked perfectly fine
- Saving the image via
imwrite("mask.png", mask)
Looks like
this. Pretty small I know. I scaled the values to be in range of 0 to 255 since that what png needs. works perfectly fine
Complete code around my corrupted lines:
void high_pass(){
Mat src_f;
// Fourier transform src_bw
src_f = fourier(src_bw);
// Create Laplace High Pass Kernel
Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, -4, 1, 0, 1, 0});
// In case of using fp values (0 to 1) initialize like this:
// Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, 0, 1, 0, 1, 0});
imshow("mask", mask);
// Fourier transform kernel
Mat mask_f = fourier_kernel(mask, src_f.size());
Mat hp_filtered;
// Apply filter
mulSpectrums(src_f, mask_f, hp_filtered, DFT_ROWS);
// Transform it back
dst = fourier_inv(hp_filtered);
// Swap quadrants after applying filter
dst = swap_quadrants(dst);
// Show result
//imshow(WINDOW_NAME + "high pass", dst);
}
FYI: The last line threw the same exception which is why it is commented out. I ask the question with "mask" because it is easier.
After writing the question I had another idea.
Solution: I converted the CV_32F
type matrix to a CV_8U
matrix and scaled all values to be in range of 0 to 255. This solved the problem.
This is something I should have thought of first. For some reason it took me one hour to realize. Just in case someone else is encountering the same error or mental block I still post this here.
c++ opencv highgui
add a comment |
IMPORTANT: I fixed the problem. Solution at the end.
What do I try to achieve?
Display an image with OpenCV cv::imshow method. (imshow Documentation)
The image which is a 3x3 matrix is created like such:
Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, -4, 1, 0, 1, 0});
To display the image I call imshow("mask", mask);
What is my problem?
Like I mentioned in the title there is an exception thrown while trying to display the image. Complete Error Message:
terminate called after throwing an instance of 'cv::Exception' what():
OpenCV(4.0.0-pre) /home/mrlab/Libraries/opencv_source/modules/highgui
/src/window_gtk.cpp:146: error: (-215:Assertion failed)
dst.data == widget->original_image->data.ptr in function 'cvImageWidgetSetImage'
Link to window_gtk.cpp
What did I already try?
- Looking for the error on the internet. Maybe someone else already encountered the same problem. nope. nothing
- Changed the matrix to only contain positive floating point values (0 to 1) in case it has problems with negative input. Initialization:
Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, 0, 1, 0, 1, 0});
same error
- Calling the two methods in different locations in case there are changes made during my other code. same error
- Writing small OpenCV program to just run these two lines. same error
- Various combinations of the above mentioned ideas. same error
- Displaying other images I read from memory instead of creating them myself. worked perfectly fine
- Saving the image via
imwrite("mask.png", mask)
Looks like
this. Pretty small I know. I scaled the values to be in range of 0 to 255 since that what png needs. works perfectly fine
Complete code around my corrupted lines:
void high_pass(){
Mat src_f;
// Fourier transform src_bw
src_f = fourier(src_bw);
// Create Laplace High Pass Kernel
Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, -4, 1, 0, 1, 0});
// In case of using fp values (0 to 1) initialize like this:
// Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, 0, 1, 0, 1, 0});
imshow("mask", mask);
// Fourier transform kernel
Mat mask_f = fourier_kernel(mask, src_f.size());
Mat hp_filtered;
// Apply filter
mulSpectrums(src_f, mask_f, hp_filtered, DFT_ROWS);
// Transform it back
dst = fourier_inv(hp_filtered);
// Swap quadrants after applying filter
dst = swap_quadrants(dst);
// Show result
//imshow(WINDOW_NAME + "high pass", dst);
}
FYI: The last line threw the same exception which is why it is commented out. I ask the question with "mask" because it is easier.
After writing the question I had another idea.
Solution: I converted the CV_32F
type matrix to a CV_8U
matrix and scaled all values to be in range of 0 to 255. This solved the problem.
This is something I should have thought of first. For some reason it took me one hour to realize. Just in case someone else is encountering the same error or mental block I still post this here.
c++ opencv highgui
I am having the same issue. Fixed it by reverting to the latest released version of OpenCV (3.4.3).
– Nikolaj Fogh
Nov 13 '18 at 15:02
Ok. Good to know. I should have mentioned that I use OpenCV Version 4.0.0
– Philipp G.
Nov 14 '18 at 15:28
add a comment |
IMPORTANT: I fixed the problem. Solution at the end.
What do I try to achieve?
Display an image with OpenCV cv::imshow method. (imshow Documentation)
The image which is a 3x3 matrix is created like such:
Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, -4, 1, 0, 1, 0});
To display the image I call imshow("mask", mask);
What is my problem?
Like I mentioned in the title there is an exception thrown while trying to display the image. Complete Error Message:
terminate called after throwing an instance of 'cv::Exception' what():
OpenCV(4.0.0-pre) /home/mrlab/Libraries/opencv_source/modules/highgui
/src/window_gtk.cpp:146: error: (-215:Assertion failed)
dst.data == widget->original_image->data.ptr in function 'cvImageWidgetSetImage'
Link to window_gtk.cpp
What did I already try?
- Looking for the error on the internet. Maybe someone else already encountered the same problem. nope. nothing
- Changed the matrix to only contain positive floating point values (0 to 1) in case it has problems with negative input. Initialization:
Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, 0, 1, 0, 1, 0});
same error
- Calling the two methods in different locations in case there are changes made during my other code. same error
- Writing small OpenCV program to just run these two lines. same error
- Various combinations of the above mentioned ideas. same error
- Displaying other images I read from memory instead of creating them myself. worked perfectly fine
- Saving the image via
imwrite("mask.png", mask)
Looks like
this. Pretty small I know. I scaled the values to be in range of 0 to 255 since that what png needs. works perfectly fine
Complete code around my corrupted lines:
void high_pass(){
Mat src_f;
// Fourier transform src_bw
src_f = fourier(src_bw);
// Create Laplace High Pass Kernel
Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, -4, 1, 0, 1, 0});
// In case of using fp values (0 to 1) initialize like this:
// Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, 0, 1, 0, 1, 0});
imshow("mask", mask);
// Fourier transform kernel
Mat mask_f = fourier_kernel(mask, src_f.size());
Mat hp_filtered;
// Apply filter
mulSpectrums(src_f, mask_f, hp_filtered, DFT_ROWS);
// Transform it back
dst = fourier_inv(hp_filtered);
// Swap quadrants after applying filter
dst = swap_quadrants(dst);
// Show result
//imshow(WINDOW_NAME + "high pass", dst);
}
FYI: The last line threw the same exception which is why it is commented out. I ask the question with "mask" because it is easier.
After writing the question I had another idea.
Solution: I converted the CV_32F
type matrix to a CV_8U
matrix and scaled all values to be in range of 0 to 255. This solved the problem.
This is something I should have thought of first. For some reason it took me one hour to realize. Just in case someone else is encountering the same error or mental block I still post this here.
c++ opencv highgui
IMPORTANT: I fixed the problem. Solution at the end.
What do I try to achieve?
Display an image with OpenCV cv::imshow method. (imshow Documentation)
The image which is a 3x3 matrix is created like such:
Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, -4, 1, 0, 1, 0});
To display the image I call imshow("mask", mask);
What is my problem?
Like I mentioned in the title there is an exception thrown while trying to display the image. Complete Error Message:
terminate called after throwing an instance of 'cv::Exception' what():
OpenCV(4.0.0-pre) /home/mrlab/Libraries/opencv_source/modules/highgui
/src/window_gtk.cpp:146: error: (-215:Assertion failed)
dst.data == widget->original_image->data.ptr in function 'cvImageWidgetSetImage'
Link to window_gtk.cpp
What did I already try?
- Looking for the error on the internet. Maybe someone else already encountered the same problem. nope. nothing
- Changed the matrix to only contain positive floating point values (0 to 1) in case it has problems with negative input. Initialization:
Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, 0, 1, 0, 1, 0});
same error
- Calling the two methods in different locations in case there are changes made during my other code. same error
- Writing small OpenCV program to just run these two lines. same error
- Various combinations of the above mentioned ideas. same error
- Displaying other images I read from memory instead of creating them myself. worked perfectly fine
- Saving the image via
imwrite("mask.png", mask)
Looks like
this. Pretty small I know. I scaled the values to be in range of 0 to 255 since that what png needs. works perfectly fine
Complete code around my corrupted lines:
void high_pass(){
Mat src_f;
// Fourier transform src_bw
src_f = fourier(src_bw);
// Create Laplace High Pass Kernel
Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, -4, 1, 0, 1, 0});
// In case of using fp values (0 to 1) initialize like this:
// Mat mask(3, 3, CV_32F, new float[9]{0, 1, 0, 1, 0, 1, 0, 1, 0});
imshow("mask", mask);
// Fourier transform kernel
Mat mask_f = fourier_kernel(mask, src_f.size());
Mat hp_filtered;
// Apply filter
mulSpectrums(src_f, mask_f, hp_filtered, DFT_ROWS);
// Transform it back
dst = fourier_inv(hp_filtered);
// Swap quadrants after applying filter
dst = swap_quadrants(dst);
// Show result
//imshow(WINDOW_NAME + "high pass", dst);
}
FYI: The last line threw the same exception which is why it is commented out. I ask the question with "mask" because it is easier.
After writing the question I had another idea.
Solution: I converted the CV_32F
type matrix to a CV_8U
matrix and scaled all values to be in range of 0 to 255. This solved the problem.
This is something I should have thought of first. For some reason it took me one hour to realize. Just in case someone else is encountering the same error or mental block I still post this here.
c++ opencv highgui
c++ opencv highgui
asked Nov 12 '18 at 18:13


Philipp G.Philipp G.
13
13
I am having the same issue. Fixed it by reverting to the latest released version of OpenCV (3.4.3).
– Nikolaj Fogh
Nov 13 '18 at 15:02
Ok. Good to know. I should have mentioned that I use OpenCV Version 4.0.0
– Philipp G.
Nov 14 '18 at 15:28
add a comment |
I am having the same issue. Fixed it by reverting to the latest released version of OpenCV (3.4.3).
– Nikolaj Fogh
Nov 13 '18 at 15:02
Ok. Good to know. I should have mentioned that I use OpenCV Version 4.0.0
– Philipp G.
Nov 14 '18 at 15:28
I am having the same issue. Fixed it by reverting to the latest released version of OpenCV (3.4.3).
– Nikolaj Fogh
Nov 13 '18 at 15:02
I am having the same issue. Fixed it by reverting to the latest released version of OpenCV (3.4.3).
– Nikolaj Fogh
Nov 13 '18 at 15:02
Ok. Good to know. I should have mentioned that I use OpenCV Version 4.0.0
– Philipp G.
Nov 14 '18 at 15:28
Ok. Good to know. I should have mentioned that I use OpenCV Version 4.0.0
– Philipp G.
Nov 14 '18 at 15:28
add a comment |
1 Answer
1
active
oldest
votes
Solution: I converted the CV_32F type matrix to a CV_8U matrix and scaled all values to be in range of 0 to 255. This solved the problem.
Edit: As stated by Nikolaj Fogh it is also possible to revert to OpenCV Version 3.4.3. I did not test it myself.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267856%2fopencv-assertion-failed-dst-data-widget-original-image-data-ptr-in-funct%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Solution: I converted the CV_32F type matrix to a CV_8U matrix and scaled all values to be in range of 0 to 255. This solved the problem.
Edit: As stated by Nikolaj Fogh it is also possible to revert to OpenCV Version 3.4.3. I did not test it myself.
add a comment |
Solution: I converted the CV_32F type matrix to a CV_8U matrix and scaled all values to be in range of 0 to 255. This solved the problem.
Edit: As stated by Nikolaj Fogh it is also possible to revert to OpenCV Version 3.4.3. I did not test it myself.
add a comment |
Solution: I converted the CV_32F type matrix to a CV_8U matrix and scaled all values to be in range of 0 to 255. This solved the problem.
Edit: As stated by Nikolaj Fogh it is also possible to revert to OpenCV Version 3.4.3. I did not test it myself.
Solution: I converted the CV_32F type matrix to a CV_8U matrix and scaled all values to be in range of 0 to 255. This solved the problem.
Edit: As stated by Nikolaj Fogh it is also possible to revert to OpenCV Version 3.4.3. I did not test it myself.
edited Nov 21 '18 at 14:35
answered Nov 12 '18 at 18:14


Philipp G.Philipp G.
13
13
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267856%2fopencv-assertion-failed-dst-data-widget-original-image-data-ptr-in-funct%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Wr9GIAt0S0XR,MPfr
I am having the same issue. Fixed it by reverting to the latest released version of OpenCV (3.4.3).
– Nikolaj Fogh
Nov 13 '18 at 15:02
Ok. Good to know. I should have mentioned that I use OpenCV Version 4.0.0
– Philipp G.
Nov 14 '18 at 15:28