return the key whose values are repeated
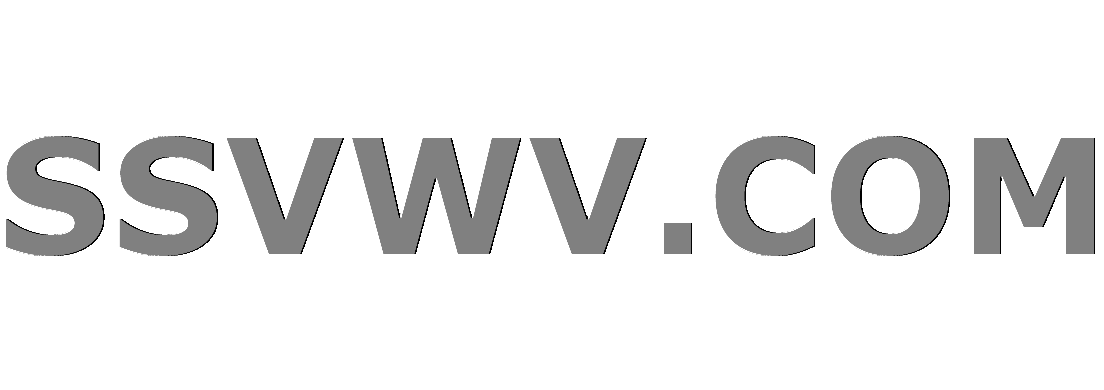
Multi tool use
I have dictionary whose values of the keys are lists. each list may contain some values repeated more than once like this
{'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'], 'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'], 'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
I want to do loop that takes the keys whose values have frequencies more than 1
for example:
VDD: ['A3':8]
X : ['A1':8, 'B':2]
how this can be done?
python dictionary for-loop counter
add a comment |
I have dictionary whose values of the keys are lists. each list may contain some values repeated more than once like this
{'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'], 'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'], 'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
I want to do loop that takes the keys whose values have frequencies more than 1
for example:
VDD: ['A3':8]
X : ['A1':8, 'B':2]
how this can be done?
python dictionary for-loop counter
would you accept pandas?
– W-B
Nov 12 '18 at 0:38
@W-B what is pandas?
– M.salameh
Nov 12 '18 at 0:40
add a comment |
I have dictionary whose values of the keys are lists. each list may contain some values repeated more than once like this
{'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'], 'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'], 'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
I want to do loop that takes the keys whose values have frequencies more than 1
for example:
VDD: ['A3':8]
X : ['A1':8, 'B':2]
how this can be done?
python dictionary for-loop counter
I have dictionary whose values of the keys are lists. each list may contain some values repeated more than once like this
{'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'], 'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'], 'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
I want to do loop that takes the keys whose values have frequencies more than 1
for example:
VDD: ['A3':8]
X : ['A1':8, 'B':2]
how this can be done?
python dictionary for-loop counter
python dictionary for-loop counter
edited Nov 12 '18 at 0:45


jpp
91.7k2052102
91.7k2052102
asked Nov 12 '18 at 0:36
M.salameh
597
597
would you accept pandas?
– W-B
Nov 12 '18 at 0:38
@W-B what is pandas?
– M.salameh
Nov 12 '18 at 0:40
add a comment |
would you accept pandas?
– W-B
Nov 12 '18 at 0:38
@W-B what is pandas?
– M.salameh
Nov 12 '18 at 0:40
would you accept pandas?
– W-B
Nov 12 '18 at 0:38
would you accept pandas?
– W-B
Nov 12 '18 at 0:38
@W-B what is pandas?
– M.salameh
Nov 12 '18 at 0:40
@W-B what is pandas?
– M.salameh
Nov 12 '18 at 0:40
add a comment |
3 Answers
3
active
oldest
votes
Using collections.Counter
:
from collections import Counter
# count values in lists, only including counts greater than 1
c = {k: {val: count for val, count in Counter(v).items() if count > 1}
for k, v in d.items()}
# isolate only keys where Counter value is non-empty
res = {k: v for k, v in c.items() if v}
{'VDD': {'A3': 8},
'X': {'B': 2, 'A1': 8}}
Line continuation isn't needed inside a parenthesised expression ;-)
– coldspeed
Nov 12 '18 at 0:56
@coldspeed, Yep I know :). But I find it more readable.. I can see myself seeing}
at the end of one line and thinking the comprehension ends there!
– jpp
Nov 12 '18 at 0:57
add a comment |
You could use Counter:
from collections import Counter
data = {'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'], 'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'], 'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
result = {key : { e: count for e, count in Counter(values).items() if count > 1} for key, values in data.items() if any(value > 1 for value in Counter(values).values())}
print(result)
Output
{'VDD': {'A3': 8}, 'X': {'B': 2, 'A1': 8}}
Or if you prefer the values as a list of tuples:
from collections import Counter
data = {'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'],
'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'],
'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
result = {key: [(element, count) for element, count in counts.items() if count > 1] for key, counts in map(lambda x: (x[0], Counter(x[1])), data.items()) if
any(count > 1 for count in counts.values())}
print(result)
Output
{'VDD': [('A3', 8)], 'X': [('A1', 8), ('B', 2)]}
add a comment |
The first step is to reverse the mapping.
valuekeydict = {}
for k, vs in orig_dict.items():
for v in vs:
valuekeydict.setdefault(v, ).append(v)
Then grab a set of all values where len > 1
result = {v for vs in valuekeydict.values() for v in vs if len(vs) > 1}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53254661%2freturn-the-key-whose-values-are-repeated%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Using collections.Counter
:
from collections import Counter
# count values in lists, only including counts greater than 1
c = {k: {val: count for val, count in Counter(v).items() if count > 1}
for k, v in d.items()}
# isolate only keys where Counter value is non-empty
res = {k: v for k, v in c.items() if v}
{'VDD': {'A3': 8},
'X': {'B': 2, 'A1': 8}}
Line continuation isn't needed inside a parenthesised expression ;-)
– coldspeed
Nov 12 '18 at 0:56
@coldspeed, Yep I know :). But I find it more readable.. I can see myself seeing}
at the end of one line and thinking the comprehension ends there!
– jpp
Nov 12 '18 at 0:57
add a comment |
Using collections.Counter
:
from collections import Counter
# count values in lists, only including counts greater than 1
c = {k: {val: count for val, count in Counter(v).items() if count > 1}
for k, v in d.items()}
# isolate only keys where Counter value is non-empty
res = {k: v for k, v in c.items() if v}
{'VDD': {'A3': 8},
'X': {'B': 2, 'A1': 8}}
Line continuation isn't needed inside a parenthesised expression ;-)
– coldspeed
Nov 12 '18 at 0:56
@coldspeed, Yep I know :). But I find it more readable.. I can see myself seeing}
at the end of one line and thinking the comprehension ends there!
– jpp
Nov 12 '18 at 0:57
add a comment |
Using collections.Counter
:
from collections import Counter
# count values in lists, only including counts greater than 1
c = {k: {val: count for val, count in Counter(v).items() if count > 1}
for k, v in d.items()}
# isolate only keys where Counter value is non-empty
res = {k: v for k, v in c.items() if v}
{'VDD': {'A3': 8},
'X': {'B': 2, 'A1': 8}}
Using collections.Counter
:
from collections import Counter
# count values in lists, only including counts greater than 1
c = {k: {val: count for val, count in Counter(v).items() if count > 1}
for k, v in d.items()}
# isolate only keys where Counter value is non-empty
res = {k: v for k, v in c.items() if v}
{'VDD': {'A3': 8},
'X': {'B': 2, 'A1': 8}}
answered Nov 12 '18 at 0:43


jpp
91.7k2052102
91.7k2052102
Line continuation isn't needed inside a parenthesised expression ;-)
– coldspeed
Nov 12 '18 at 0:56
@coldspeed, Yep I know :). But I find it more readable.. I can see myself seeing}
at the end of one line and thinking the comprehension ends there!
– jpp
Nov 12 '18 at 0:57
add a comment |
Line continuation isn't needed inside a parenthesised expression ;-)
– coldspeed
Nov 12 '18 at 0:56
@coldspeed, Yep I know :). But I find it more readable.. I can see myself seeing}
at the end of one line and thinking the comprehension ends there!
– jpp
Nov 12 '18 at 0:57
Line continuation isn't needed inside a parenthesised expression ;-)
– coldspeed
Nov 12 '18 at 0:56
Line continuation isn't needed inside a parenthesised expression ;-)
– coldspeed
Nov 12 '18 at 0:56
@coldspeed, Yep I know :). But I find it more readable.. I can see myself seeing
}
at the end of one line and thinking the comprehension ends there!– jpp
Nov 12 '18 at 0:57
@coldspeed, Yep I know :). But I find it more readable.. I can see myself seeing
}
at the end of one line and thinking the comprehension ends there!– jpp
Nov 12 '18 at 0:57
add a comment |
You could use Counter:
from collections import Counter
data = {'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'], 'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'], 'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
result = {key : { e: count for e, count in Counter(values).items() if count > 1} for key, values in data.items() if any(value > 1 for value in Counter(values).values())}
print(result)
Output
{'VDD': {'A3': 8}, 'X': {'B': 2, 'A1': 8}}
Or if you prefer the values as a list of tuples:
from collections import Counter
data = {'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'],
'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'],
'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
result = {key: [(element, count) for element, count in counts.items() if count > 1] for key, counts in map(lambda x: (x[0], Counter(x[1])), data.items()) if
any(count > 1 for count in counts.values())}
print(result)
Output
{'VDD': [('A3', 8)], 'X': [('A1', 8), ('B', 2)]}
add a comment |
You could use Counter:
from collections import Counter
data = {'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'], 'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'], 'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
result = {key : { e: count for e, count in Counter(values).items() if count > 1} for key, values in data.items() if any(value > 1 for value in Counter(values).values())}
print(result)
Output
{'VDD': {'A3': 8}, 'X': {'B': 2, 'A1': 8}}
Or if you prefer the values as a list of tuples:
from collections import Counter
data = {'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'],
'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'],
'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
result = {key: [(element, count) for element, count in counts.items() if count > 1] for key, counts in map(lambda x: (x[0], Counter(x[1])), data.items()) if
any(count > 1 for count in counts.values())}
print(result)
Output
{'VDD': [('A3', 8)], 'X': [('A1', 8), ('B', 2)]}
add a comment |
You could use Counter:
from collections import Counter
data = {'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'], 'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'], 'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
result = {key : { e: count for e, count in Counter(values).items() if count > 1} for key, values in data.items() if any(value > 1 for value in Counter(values).values())}
print(result)
Output
{'VDD': {'A3': 8}, 'X': {'B': 2, 'A1': 8}}
Or if you prefer the values as a list of tuples:
from collections import Counter
data = {'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'],
'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'],
'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
result = {key: [(element, count) for element, count in counts.items() if count > 1] for key, counts in map(lambda x: (x[0], Counter(x[1])), data.items()) if
any(count > 1 for count in counts.values())}
print(result)
Output
{'VDD': [('A3', 8)], 'X': [('A1', 8), ('B', 2)]}
You could use Counter:
from collections import Counter
data = {'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'], 'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'], 'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
result = {key : { e: count for e, count in Counter(values).items() if count > 1} for key, values in data.items() if any(value > 1 for value in Counter(values).values())}
print(result)
Output
{'VDD': {'A3': 8}, 'X': {'B': 2, 'A1': 8}}
Or if you prefer the values as a list of tuples:
from collections import Counter
data = {'VSS': ['A2', 'A3', 'A1'], 'X_P1_1': ['A2', 'A1'], 'X_P2': ['A3', 'A2'], 'X_P1_3': ['A2', 'A1'],
'VDD': ['A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'A3', 'B', 'A3'],
'X': ['B', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'A1', 'B', 'A1']}
result = {key: [(element, count) for element, count in counts.items() if count > 1] for key, counts in map(lambda x: (x[0], Counter(x[1])), data.items()) if
any(count > 1 for count in counts.values())}
print(result)
Output
{'VDD': [('A3', 8)], 'X': [('A1', 8), ('B', 2)]}
edited Nov 12 '18 at 0:47
answered Nov 12 '18 at 0:42


Daniel Mesejo
13.4k11027
13.4k11027
add a comment |
add a comment |
The first step is to reverse the mapping.
valuekeydict = {}
for k, vs in orig_dict.items():
for v in vs:
valuekeydict.setdefault(v, ).append(v)
Then grab a set of all values where len > 1
result = {v for vs in valuekeydict.values() for v in vs if len(vs) > 1}
add a comment |
The first step is to reverse the mapping.
valuekeydict = {}
for k, vs in orig_dict.items():
for v in vs:
valuekeydict.setdefault(v, ).append(v)
Then grab a set of all values where len > 1
result = {v for vs in valuekeydict.values() for v in vs if len(vs) > 1}
add a comment |
The first step is to reverse the mapping.
valuekeydict = {}
for k, vs in orig_dict.items():
for v in vs:
valuekeydict.setdefault(v, ).append(v)
Then grab a set of all values where len > 1
result = {v for vs in valuekeydict.values() for v in vs if len(vs) > 1}
The first step is to reverse the mapping.
valuekeydict = {}
for k, vs in orig_dict.items():
for v in vs:
valuekeydict.setdefault(v, ).append(v)
Then grab a set of all values where len > 1
result = {v for vs in valuekeydict.values() for v in vs if len(vs) > 1}
answered Nov 12 '18 at 0:44
Adam Smith
33.2k53174
33.2k53174
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53254661%2freturn-the-key-whose-values-are-repeated%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZKOSFg3HFwlj7X0OCmxg,yjERs1fiG u4a91hH9,TlGcG2yUCq afeP5Ido,lX8FMQQEvsJcV5,y
would you accept pandas?
– W-B
Nov 12 '18 at 0:38
@W-B what is pandas?
– M.salameh
Nov 12 '18 at 0:40