JavaScript: Find all parents for element in tree recursive
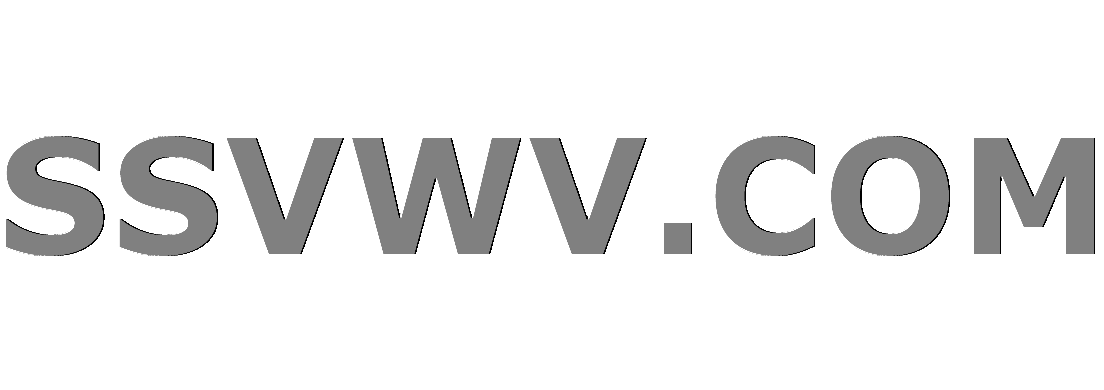
Multi tool use
I have a tree somthing like this
var datas = {
'tree': [
{
'name': 'name1',
'tree': [
{'name': 'name2'},
{'name': 'name3'},
{
'name': 'name4',
'tree': [
{'name': 'name5'},
{'name': 'name6'}
]
},
{'name': 'name7'}
]
},
{
'name': 'name8',
'tree': [
{'name': 'name9'}
]
}
]
}
I want to find all the parents of the specifig id
for example in the tree demo, if I look for 'name5' I want to find "name1,name4,name5"
I wrote this code but the results wrong and I got the ids of other elements and not the parents only
This is my code
keys: string;
pathFound: boolean = false;
getLevel(event: iEventBase, id: string, path: string): void {
if (this.pathFound) return;
event.content.forEach((key) => {
if (key.id == id) {
if(!path){
path = ;;
}
path.push(key.id);
this.keys = path;
this.pathFound = true;
return;
}
if (key.type === "page") {
if(!path){
path = ;
}
path.push(key.id);
this.getLevel(key, id, path);
}
})
}
}
this.getLevel(state.mainEvent.content.page, event.id, null);
javascript recursion tree
add a comment |
I have a tree somthing like this
var datas = {
'tree': [
{
'name': 'name1',
'tree': [
{'name': 'name2'},
{'name': 'name3'},
{
'name': 'name4',
'tree': [
{'name': 'name5'},
{'name': 'name6'}
]
},
{'name': 'name7'}
]
},
{
'name': 'name8',
'tree': [
{'name': 'name9'}
]
}
]
}
I want to find all the parents of the specifig id
for example in the tree demo, if I look for 'name5' I want to find "name1,name4,name5"
I wrote this code but the results wrong and I got the ids of other elements and not the parents only
This is my code
keys: string;
pathFound: boolean = false;
getLevel(event: iEventBase, id: string, path: string): void {
if (this.pathFound) return;
event.content.forEach((key) => {
if (key.id == id) {
if(!path){
path = ;;
}
path.push(key.id);
this.keys = path;
this.pathFound = true;
return;
}
if (key.type === "page") {
if(!path){
path = ;
}
path.push(key.id);
this.getLevel(key, id, path);
}
})
}
}
this.getLevel(state.mainEvent.content.page, event.id, null);
javascript recursion tree
add a comment |
I have a tree somthing like this
var datas = {
'tree': [
{
'name': 'name1',
'tree': [
{'name': 'name2'},
{'name': 'name3'},
{
'name': 'name4',
'tree': [
{'name': 'name5'},
{'name': 'name6'}
]
},
{'name': 'name7'}
]
},
{
'name': 'name8',
'tree': [
{'name': 'name9'}
]
}
]
}
I want to find all the parents of the specifig id
for example in the tree demo, if I look for 'name5' I want to find "name1,name4,name5"
I wrote this code but the results wrong and I got the ids of other elements and not the parents only
This is my code
keys: string;
pathFound: boolean = false;
getLevel(event: iEventBase, id: string, path: string): void {
if (this.pathFound) return;
event.content.forEach((key) => {
if (key.id == id) {
if(!path){
path = ;;
}
path.push(key.id);
this.keys = path;
this.pathFound = true;
return;
}
if (key.type === "page") {
if(!path){
path = ;
}
path.push(key.id);
this.getLevel(key, id, path);
}
})
}
}
this.getLevel(state.mainEvent.content.page, event.id, null);
javascript recursion tree
I have a tree somthing like this
var datas = {
'tree': [
{
'name': 'name1',
'tree': [
{'name': 'name2'},
{'name': 'name3'},
{
'name': 'name4',
'tree': [
{'name': 'name5'},
{'name': 'name6'}
]
},
{'name': 'name7'}
]
},
{
'name': 'name8',
'tree': [
{'name': 'name9'}
]
}
]
}
I want to find all the parents of the specifig id
for example in the tree demo, if I look for 'name5' I want to find "name1,name4,name5"
I wrote this code but the results wrong and I got the ids of other elements and not the parents only
This is my code
keys: string;
pathFound: boolean = false;
getLevel(event: iEventBase, id: string, path: string): void {
if (this.pathFound) return;
event.content.forEach((key) => {
if (key.id == id) {
if(!path){
path = ;;
}
path.push(key.id);
this.keys = path;
this.pathFound = true;
return;
}
if (key.type === "page") {
if(!path){
path = ;
}
path.push(key.id);
this.getLevel(key, id, path);
}
})
}
}
this.getLevel(state.mainEvent.content.page, event.id, null);
javascript recursion tree
javascript recursion tree
edited Nov 13 '18 at 9:20
wanttobeprofessional
1,03731323
1,03731323
asked Nov 13 '18 at 8:59
24sharon24sharon
4942935
4942935
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You could use a recursive approach by checking the name and return the name in an array or return the path to the object by calling the function again.
function find(array, name) {
var result;
array.some(o => {
var temp;
if (o.name === name) {
return result = [name];
}
temp = find(o.tree || , name);
if (temp) {
return result = [o.name, ...temp];
}
});
return result;
}
var datas = { tree: [{ name: 'name1', tree: [{ name: 'name2' }, { name: 'name3' }, { name: 'name4', tree: [{ name: 'name5' }, { name: 'name6' }] }, { name: 'name7' }] }, { name: 'name8', tree: [{ name: 'name9' }] }] };
console.log(find(datas.tree, 'name5'));
.as-console-wrapper { max-height: 100% !important; top: 0; }
add a comment |
Perhaps you could take a recursive approach to this problem. The basic idea here is to look down through the tree for a node whose name matches the node name that your looking to find parents for (ie name5
).
Once such a node has been found, then return an array, appending the corresponding parent name of the node to the array until the recursion completely unwinds.
So, something along the lines of this should work:
var datas = {
'tree': [
{
'name': 'name1',
'tree': [
{'name': 'name2'},
{'name': 'name3'},
{
'name': 'name4',
'tree': [
{'name': 'name5'},
{'name': 'name6'}
]
},
{'name': 'name7'}
]
},
{
'name': 'name8',
'tree': [
{'name': 'name9'}
]
}
]
}
function findParents(node, searchForName) {
// If current node name matches the search name, return
// empty array which is the beginning of our parent result
if(node.name === searchForName) {
return
}
// Otherwise, if this node has a tree field/value, recursively
// process the nodes in this tree array
if(Array.isArray(node.tree)) {
for(var treeNode of node.tree) {
// Recursively process treeNode. If an array result is
// returned, then add the treeNode.name to that result
// and return recursively
const childResult = findParents(treeNode, searchForName)
if(Array.isArray(childResult)) {
return [ treeNode.name ].concat( childResult );
}
}
}
}
console.log( findParents(datas, 'name5') )
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277219%2fjavascript-find-all-parents-for-element-in-tree-recursive%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You could use a recursive approach by checking the name and return the name in an array or return the path to the object by calling the function again.
function find(array, name) {
var result;
array.some(o => {
var temp;
if (o.name === name) {
return result = [name];
}
temp = find(o.tree || , name);
if (temp) {
return result = [o.name, ...temp];
}
});
return result;
}
var datas = { tree: [{ name: 'name1', tree: [{ name: 'name2' }, { name: 'name3' }, { name: 'name4', tree: [{ name: 'name5' }, { name: 'name6' }] }, { name: 'name7' }] }, { name: 'name8', tree: [{ name: 'name9' }] }] };
console.log(find(datas.tree, 'name5'));
.as-console-wrapper { max-height: 100% !important; top: 0; }
add a comment |
You could use a recursive approach by checking the name and return the name in an array or return the path to the object by calling the function again.
function find(array, name) {
var result;
array.some(o => {
var temp;
if (o.name === name) {
return result = [name];
}
temp = find(o.tree || , name);
if (temp) {
return result = [o.name, ...temp];
}
});
return result;
}
var datas = { tree: [{ name: 'name1', tree: [{ name: 'name2' }, { name: 'name3' }, { name: 'name4', tree: [{ name: 'name5' }, { name: 'name6' }] }, { name: 'name7' }] }, { name: 'name8', tree: [{ name: 'name9' }] }] };
console.log(find(datas.tree, 'name5'));
.as-console-wrapper { max-height: 100% !important; top: 0; }
add a comment |
You could use a recursive approach by checking the name and return the name in an array or return the path to the object by calling the function again.
function find(array, name) {
var result;
array.some(o => {
var temp;
if (o.name === name) {
return result = [name];
}
temp = find(o.tree || , name);
if (temp) {
return result = [o.name, ...temp];
}
});
return result;
}
var datas = { tree: [{ name: 'name1', tree: [{ name: 'name2' }, { name: 'name3' }, { name: 'name4', tree: [{ name: 'name5' }, { name: 'name6' }] }, { name: 'name7' }] }, { name: 'name8', tree: [{ name: 'name9' }] }] };
console.log(find(datas.tree, 'name5'));
.as-console-wrapper { max-height: 100% !important; top: 0; }
You could use a recursive approach by checking the name and return the name in an array or return the path to the object by calling the function again.
function find(array, name) {
var result;
array.some(o => {
var temp;
if (o.name === name) {
return result = [name];
}
temp = find(o.tree || , name);
if (temp) {
return result = [o.name, ...temp];
}
});
return result;
}
var datas = { tree: [{ name: 'name1', tree: [{ name: 'name2' }, { name: 'name3' }, { name: 'name4', tree: [{ name: 'name5' }, { name: 'name6' }] }, { name: 'name7' }] }, { name: 'name8', tree: [{ name: 'name9' }] }] };
console.log(find(datas.tree, 'name5'));
.as-console-wrapper { max-height: 100% !important; top: 0; }
function find(array, name) {
var result;
array.some(o => {
var temp;
if (o.name === name) {
return result = [name];
}
temp = find(o.tree || , name);
if (temp) {
return result = [o.name, ...temp];
}
});
return result;
}
var datas = { tree: [{ name: 'name1', tree: [{ name: 'name2' }, { name: 'name3' }, { name: 'name4', tree: [{ name: 'name5' }, { name: 'name6' }] }, { name: 'name7' }] }, { name: 'name8', tree: [{ name: 'name9' }] }] };
console.log(find(datas.tree, 'name5'));
.as-console-wrapper { max-height: 100% !important; top: 0; }
function find(array, name) {
var result;
array.some(o => {
var temp;
if (o.name === name) {
return result = [name];
}
temp = find(o.tree || , name);
if (temp) {
return result = [o.name, ...temp];
}
});
return result;
}
var datas = { tree: [{ name: 'name1', tree: [{ name: 'name2' }, { name: 'name3' }, { name: 'name4', tree: [{ name: 'name5' }, { name: 'name6' }] }, { name: 'name7' }] }, { name: 'name8', tree: [{ name: 'name9' }] }] };
console.log(find(datas.tree, 'name5'));
.as-console-wrapper { max-height: 100% !important; top: 0; }
answered Nov 13 '18 at 9:17


Nina ScholzNina Scholz
181k1494163
181k1494163
add a comment |
add a comment |
Perhaps you could take a recursive approach to this problem. The basic idea here is to look down through the tree for a node whose name matches the node name that your looking to find parents for (ie name5
).
Once such a node has been found, then return an array, appending the corresponding parent name of the node to the array until the recursion completely unwinds.
So, something along the lines of this should work:
var datas = {
'tree': [
{
'name': 'name1',
'tree': [
{'name': 'name2'},
{'name': 'name3'},
{
'name': 'name4',
'tree': [
{'name': 'name5'},
{'name': 'name6'}
]
},
{'name': 'name7'}
]
},
{
'name': 'name8',
'tree': [
{'name': 'name9'}
]
}
]
}
function findParents(node, searchForName) {
// If current node name matches the search name, return
// empty array which is the beginning of our parent result
if(node.name === searchForName) {
return
}
// Otherwise, if this node has a tree field/value, recursively
// process the nodes in this tree array
if(Array.isArray(node.tree)) {
for(var treeNode of node.tree) {
// Recursively process treeNode. If an array result is
// returned, then add the treeNode.name to that result
// and return recursively
const childResult = findParents(treeNode, searchForName)
if(Array.isArray(childResult)) {
return [ treeNode.name ].concat( childResult );
}
}
}
}
console.log( findParents(datas, 'name5') )
add a comment |
Perhaps you could take a recursive approach to this problem. The basic idea here is to look down through the tree for a node whose name matches the node name that your looking to find parents for (ie name5
).
Once such a node has been found, then return an array, appending the corresponding parent name of the node to the array until the recursion completely unwinds.
So, something along the lines of this should work:
var datas = {
'tree': [
{
'name': 'name1',
'tree': [
{'name': 'name2'},
{'name': 'name3'},
{
'name': 'name4',
'tree': [
{'name': 'name5'},
{'name': 'name6'}
]
},
{'name': 'name7'}
]
},
{
'name': 'name8',
'tree': [
{'name': 'name9'}
]
}
]
}
function findParents(node, searchForName) {
// If current node name matches the search name, return
// empty array which is the beginning of our parent result
if(node.name === searchForName) {
return
}
// Otherwise, if this node has a tree field/value, recursively
// process the nodes in this tree array
if(Array.isArray(node.tree)) {
for(var treeNode of node.tree) {
// Recursively process treeNode. If an array result is
// returned, then add the treeNode.name to that result
// and return recursively
const childResult = findParents(treeNode, searchForName)
if(Array.isArray(childResult)) {
return [ treeNode.name ].concat( childResult );
}
}
}
}
console.log( findParents(datas, 'name5') )
add a comment |
Perhaps you could take a recursive approach to this problem. The basic idea here is to look down through the tree for a node whose name matches the node name that your looking to find parents for (ie name5
).
Once such a node has been found, then return an array, appending the corresponding parent name of the node to the array until the recursion completely unwinds.
So, something along the lines of this should work:
var datas = {
'tree': [
{
'name': 'name1',
'tree': [
{'name': 'name2'},
{'name': 'name3'},
{
'name': 'name4',
'tree': [
{'name': 'name5'},
{'name': 'name6'}
]
},
{'name': 'name7'}
]
},
{
'name': 'name8',
'tree': [
{'name': 'name9'}
]
}
]
}
function findParents(node, searchForName) {
// If current node name matches the search name, return
// empty array which is the beginning of our parent result
if(node.name === searchForName) {
return
}
// Otherwise, if this node has a tree field/value, recursively
// process the nodes in this tree array
if(Array.isArray(node.tree)) {
for(var treeNode of node.tree) {
// Recursively process treeNode. If an array result is
// returned, then add the treeNode.name to that result
// and return recursively
const childResult = findParents(treeNode, searchForName)
if(Array.isArray(childResult)) {
return [ treeNode.name ].concat( childResult );
}
}
}
}
console.log( findParents(datas, 'name5') )
Perhaps you could take a recursive approach to this problem. The basic idea here is to look down through the tree for a node whose name matches the node name that your looking to find parents for (ie name5
).
Once such a node has been found, then return an array, appending the corresponding parent name of the node to the array until the recursion completely unwinds.
So, something along the lines of this should work:
var datas = {
'tree': [
{
'name': 'name1',
'tree': [
{'name': 'name2'},
{'name': 'name3'},
{
'name': 'name4',
'tree': [
{'name': 'name5'},
{'name': 'name6'}
]
},
{'name': 'name7'}
]
},
{
'name': 'name8',
'tree': [
{'name': 'name9'}
]
}
]
}
function findParents(node, searchForName) {
// If current node name matches the search name, return
// empty array which is the beginning of our parent result
if(node.name === searchForName) {
return
}
// Otherwise, if this node has a tree field/value, recursively
// process the nodes in this tree array
if(Array.isArray(node.tree)) {
for(var treeNode of node.tree) {
// Recursively process treeNode. If an array result is
// returned, then add the treeNode.name to that result
// and return recursively
const childResult = findParents(treeNode, searchForName)
if(Array.isArray(childResult)) {
return [ treeNode.name ].concat( childResult );
}
}
}
}
console.log( findParents(datas, 'name5') )
var datas = {
'tree': [
{
'name': 'name1',
'tree': [
{'name': 'name2'},
{'name': 'name3'},
{
'name': 'name4',
'tree': [
{'name': 'name5'},
{'name': 'name6'}
]
},
{'name': 'name7'}
]
},
{
'name': 'name8',
'tree': [
{'name': 'name9'}
]
}
]
}
function findParents(node, searchForName) {
// If current node name matches the search name, return
// empty array which is the beginning of our parent result
if(node.name === searchForName) {
return
}
// Otherwise, if this node has a tree field/value, recursively
// process the nodes in this tree array
if(Array.isArray(node.tree)) {
for(var treeNode of node.tree) {
// Recursively process treeNode. If an array result is
// returned, then add the treeNode.name to that result
// and return recursively
const childResult = findParents(treeNode, searchForName)
if(Array.isArray(childResult)) {
return [ treeNode.name ].concat( childResult );
}
}
}
}
console.log( findParents(datas, 'name5') )
var datas = {
'tree': [
{
'name': 'name1',
'tree': [
{'name': 'name2'},
{'name': 'name3'},
{
'name': 'name4',
'tree': [
{'name': 'name5'},
{'name': 'name6'}
]
},
{'name': 'name7'}
]
},
{
'name': 'name8',
'tree': [
{'name': 'name9'}
]
}
]
}
function findParents(node, searchForName) {
// If current node name matches the search name, return
// empty array which is the beginning of our parent result
if(node.name === searchForName) {
return
}
// Otherwise, if this node has a tree field/value, recursively
// process the nodes in this tree array
if(Array.isArray(node.tree)) {
for(var treeNode of node.tree) {
// Recursively process treeNode. If an array result is
// returned, then add the treeNode.name to that result
// and return recursively
const childResult = findParents(treeNode, searchForName)
if(Array.isArray(childResult)) {
return [ treeNode.name ].concat( childResult );
}
}
}
}
console.log( findParents(datas, 'name5') )
edited Nov 13 '18 at 9:12
answered Nov 13 '18 at 9:05


Dacre DennyDacre Denny
11.4k41030
11.4k41030
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277219%2fjavascript-find-all-parents-for-element-in-tree-recursive%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0EHorD vWmSdg9GSpRoBB,Wi3QE2sAUSTN4CHHHm