Sort list of objects containing other objects by string C# Linq
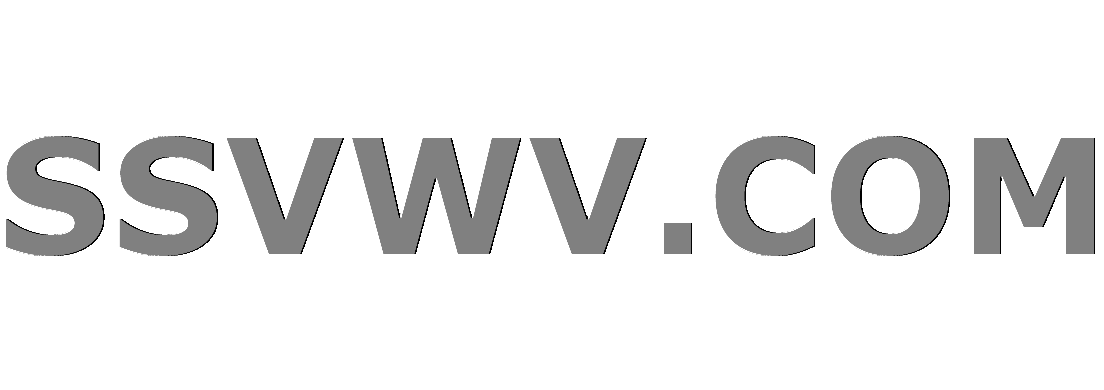
Multi tool use
I want to add functionality to sort data, by getting the string of the sort column from the Angular frontend. Here follows a short scenario.
The database model of ObjectA : Entity, IAggregateRoot. ObjectA has simple elements like Id and Name, but also has an extra object ObjectB. ObjectB has simple elements like Id and Name, but also has an extra object ObjectC, which only has simple objects Id and Name.
ObjectA: {
Id: Guid,
Name: String,
ObjectB: {
Id: Guid,
Name: String,
ObjectC: {
Id: Guid,
Name: String,
}
}
}
The model that is given to the frontend is flattened with only important elements:
ObjectA: {
Id: Guid,
Name: String,
ObjectBName: String,
ObjectCName: String
}
Now, a list of ObjectA is displayed, and I want to add sorting on it. I receive the element name (string) from the frontend to sort on, e.g. id. This sort is easily done in the backend by a Linq query like:
return objectAList.OrderBy(x => GetPropertyToSort(x, orderByString));
With the function GetPropertyToSort():
private string GetPropertyToSort(object obj, string orderBy)
{
var prop = obj.GetType().GetProperty(char.ToUpper(orderBy[0]) + orderBy.Substring(1));
var propValue = prop.GetValue(obj)?.ToString();
return propValue;
}
But how can sorting by another object element, e.g. objectCName, be achieved by receiving only the flattened string value (which is actually the element ObjectA.ObjectB.ObjectC.Name)?
entity-framework linq asp.net-core-2.0 .net-standard-2.0
add a comment |
I want to add functionality to sort data, by getting the string of the sort column from the Angular frontend. Here follows a short scenario.
The database model of ObjectA : Entity, IAggregateRoot. ObjectA has simple elements like Id and Name, but also has an extra object ObjectB. ObjectB has simple elements like Id and Name, but also has an extra object ObjectC, which only has simple objects Id and Name.
ObjectA: {
Id: Guid,
Name: String,
ObjectB: {
Id: Guid,
Name: String,
ObjectC: {
Id: Guid,
Name: String,
}
}
}
The model that is given to the frontend is flattened with only important elements:
ObjectA: {
Id: Guid,
Name: String,
ObjectBName: String,
ObjectCName: String
}
Now, a list of ObjectA is displayed, and I want to add sorting on it. I receive the element name (string) from the frontend to sort on, e.g. id. This sort is easily done in the backend by a Linq query like:
return objectAList.OrderBy(x => GetPropertyToSort(x, orderByString));
With the function GetPropertyToSort():
private string GetPropertyToSort(object obj, string orderBy)
{
var prop = obj.GetType().GetProperty(char.ToUpper(orderBy[0]) + orderBy.Substring(1));
var propValue = prop.GetValue(obj)?.ToString();
return propValue;
}
But how can sorting by another object element, e.g. objectCName, be achieved by receiving only the flattened string value (which is actually the element ObjectA.ObjectB.ObjectC.Name)?
entity-framework linq asp.net-core-2.0 .net-standard-2.0
IsorderByString == "ObjectCName"
ororderByString == "ObjectA.ObjectB.ObjectC.Name"
? (If it's the former, and the ordered list is the flattened one, you can still useGetPropertyToSort
)
– HeyJude
Nov 21 '18 at 14:55
add a comment |
I want to add functionality to sort data, by getting the string of the sort column from the Angular frontend. Here follows a short scenario.
The database model of ObjectA : Entity, IAggregateRoot. ObjectA has simple elements like Id and Name, but also has an extra object ObjectB. ObjectB has simple elements like Id and Name, but also has an extra object ObjectC, which only has simple objects Id and Name.
ObjectA: {
Id: Guid,
Name: String,
ObjectB: {
Id: Guid,
Name: String,
ObjectC: {
Id: Guid,
Name: String,
}
}
}
The model that is given to the frontend is flattened with only important elements:
ObjectA: {
Id: Guid,
Name: String,
ObjectBName: String,
ObjectCName: String
}
Now, a list of ObjectA is displayed, and I want to add sorting on it. I receive the element name (string) from the frontend to sort on, e.g. id. This sort is easily done in the backend by a Linq query like:
return objectAList.OrderBy(x => GetPropertyToSort(x, orderByString));
With the function GetPropertyToSort():
private string GetPropertyToSort(object obj, string orderBy)
{
var prop = obj.GetType().GetProperty(char.ToUpper(orderBy[0]) + orderBy.Substring(1));
var propValue = prop.GetValue(obj)?.ToString();
return propValue;
}
But how can sorting by another object element, e.g. objectCName, be achieved by receiving only the flattened string value (which is actually the element ObjectA.ObjectB.ObjectC.Name)?
entity-framework linq asp.net-core-2.0 .net-standard-2.0
I want to add functionality to sort data, by getting the string of the sort column from the Angular frontend. Here follows a short scenario.
The database model of ObjectA : Entity, IAggregateRoot. ObjectA has simple elements like Id and Name, but also has an extra object ObjectB. ObjectB has simple elements like Id and Name, but also has an extra object ObjectC, which only has simple objects Id and Name.
ObjectA: {
Id: Guid,
Name: String,
ObjectB: {
Id: Guid,
Name: String,
ObjectC: {
Id: Guid,
Name: String,
}
}
}
The model that is given to the frontend is flattened with only important elements:
ObjectA: {
Id: Guid,
Name: String,
ObjectBName: String,
ObjectCName: String
}
Now, a list of ObjectA is displayed, and I want to add sorting on it. I receive the element name (string) from the frontend to sort on, e.g. id. This sort is easily done in the backend by a Linq query like:
return objectAList.OrderBy(x => GetPropertyToSort(x, orderByString));
With the function GetPropertyToSort():
private string GetPropertyToSort(object obj, string orderBy)
{
var prop = obj.GetType().GetProperty(char.ToUpper(orderBy[0]) + orderBy.Substring(1));
var propValue = prop.GetValue(obj)?.ToString();
return propValue;
}
But how can sorting by another object element, e.g. objectCName, be achieved by receiving only the flattened string value (which is actually the element ObjectA.ObjectB.ObjectC.Name)?
entity-framework linq asp.net-core-2.0 .net-standard-2.0
entity-framework linq asp.net-core-2.0 .net-standard-2.0
edited Nov 13 '18 at 9:56
Robbert Raats
asked Nov 13 '18 at 9:09
Robbert RaatsRobbert Raats
12414
12414
IsorderByString == "ObjectCName"
ororderByString == "ObjectA.ObjectB.ObjectC.Name"
? (If it's the former, and the ordered list is the flattened one, you can still useGetPropertyToSort
)
– HeyJude
Nov 21 '18 at 14:55
add a comment |
IsorderByString == "ObjectCName"
ororderByString == "ObjectA.ObjectB.ObjectC.Name"
? (If it's the former, and the ordered list is the flattened one, you can still useGetPropertyToSort
)
– HeyJude
Nov 21 '18 at 14:55
Is
orderByString == "ObjectCName"
or orderByString == "ObjectA.ObjectB.ObjectC.Name"
? (If it's the former, and the ordered list is the flattened one, you can still use GetPropertyToSort
)– HeyJude
Nov 21 '18 at 14:55
Is
orderByString == "ObjectCName"
or orderByString == "ObjectA.ObjectB.ObjectC.Name"
? (If it's the former, and the ordered list is the flattened one, you can still use GetPropertyToSort
)– HeyJude
Nov 21 '18 at 14:55
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277404%2fsort-list-of-objects-containing-other-objects-by-string-c-sharp-linq%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277404%2fsort-list-of-objects-containing-other-objects-by-string-c-sharp-linq%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7o,HE owb EBKIF7ywzpYv 8snLFg,U v,Tf3a,Q,SKw1ihHbAEXgMIOWr pPDl 0 Y5hxZRR wRh4LTQe,25GAfjJi
Is
orderByString == "ObjectCName"
ororderByString == "ObjectA.ObjectB.ObjectC.Name"
? (If it's the former, and the ordered list is the flattened one, you can still useGetPropertyToSort
)– HeyJude
Nov 21 '18 at 14:55