React / Redux: mapStateToProps() is called many times
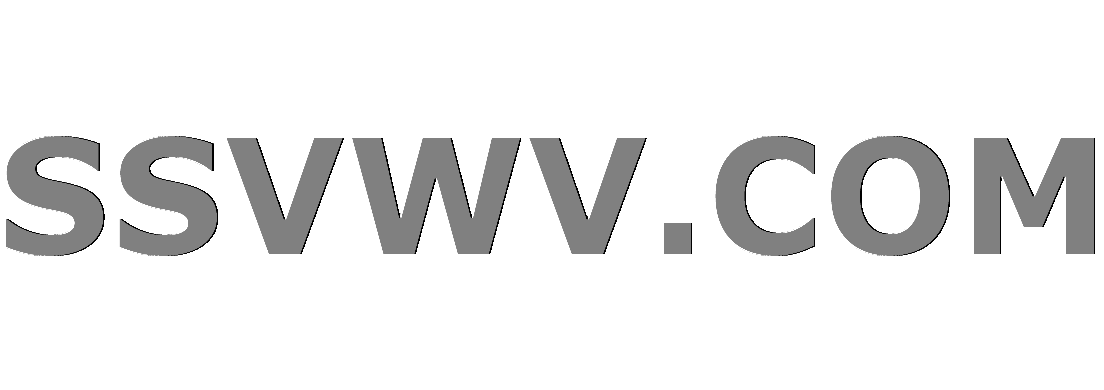
Multi tool use
I'm writing simple app using React / Redux. I notice that function mapStateToProps() is called many times. Here`s code:
class Employees extends React.Component {
componentDidMount() {
this.props.fetchEmployees();
}
}
let mapStateToProps = function mapStateToProps(state) {
console.log("foo");
return {...};
};
let mapDispatch = (dispatch) => {
return {
fetchEmployees: bindActionCreators(fetchEmployees, dispatch)
};
};
export default connect(mapStateToProps, mapDispatch)(Employees);
And what I get in console:
Is it normal or I'm doing something wrong?
reactjs redux react-redux
add a comment |
I'm writing simple app using React / Redux. I notice that function mapStateToProps() is called many times. Here`s code:
class Employees extends React.Component {
componentDidMount() {
this.props.fetchEmployees();
}
}
let mapStateToProps = function mapStateToProps(state) {
console.log("foo");
return {...};
};
let mapDispatch = (dispatch) => {
return {
fetchEmployees: bindActionCreators(fetchEmployees, dispatch)
};
};
export default connect(mapStateToProps, mapDispatch)(Employees);
And what I get in console:
Is it normal or I'm doing something wrong?
reactjs redux react-redux
1
This is normal. That's why for heavy computations in state to props people use memoised selectors, likereselect
.
– dfsq
Nov 13 '18 at 9:09
add a comment |
I'm writing simple app using React / Redux. I notice that function mapStateToProps() is called many times. Here`s code:
class Employees extends React.Component {
componentDidMount() {
this.props.fetchEmployees();
}
}
let mapStateToProps = function mapStateToProps(state) {
console.log("foo");
return {...};
};
let mapDispatch = (dispatch) => {
return {
fetchEmployees: bindActionCreators(fetchEmployees, dispatch)
};
};
export default connect(mapStateToProps, mapDispatch)(Employees);
And what I get in console:
Is it normal or I'm doing something wrong?
reactjs redux react-redux
I'm writing simple app using React / Redux. I notice that function mapStateToProps() is called many times. Here`s code:
class Employees extends React.Component {
componentDidMount() {
this.props.fetchEmployees();
}
}
let mapStateToProps = function mapStateToProps(state) {
console.log("foo");
return {...};
};
let mapDispatch = (dispatch) => {
return {
fetchEmployees: bindActionCreators(fetchEmployees, dispatch)
};
};
export default connect(mapStateToProps, mapDispatch)(Employees);
And what I get in console:
Is it normal or I'm doing something wrong?
reactjs redux react-redux
reactjs redux react-redux
asked Nov 13 '18 at 9:07
MRaimonMRaimon
466
466
1
This is normal. That's why for heavy computations in state to props people use memoised selectors, likereselect
.
– dfsq
Nov 13 '18 at 9:09
add a comment |
1
This is normal. That's why for heavy computations in state to props people use memoised selectors, likereselect
.
– dfsq
Nov 13 '18 at 9:09
1
1
This is normal. That's why for heavy computations in state to props people use memoised selectors, like
reselect
.– dfsq
Nov 13 '18 at 9:09
This is normal. That's why for heavy computations in state to props people use memoised selectors, like
reselect
.– dfsq
Nov 13 '18 at 9:09
add a comment |
1 Answer
1
active
oldest
votes
Anytime there is a change in state of redux or the parent component of your container re-renders the mapStateToProps
is being called. This however won't cause your component's render to call again provided the data returned by mapStateToProps and the props that it receives hasn't changed.
If you have some computation that you do in mapStateToProps
that returns a value, make sure you use a memoized function to do that computation or reselect
to write memoized selectors
so that the same value is returned for the same computation even if mapStateToProps
is called multiple times
thanks a lot! Yes, actually I call filter function inmapStateToProps
. Does this mean I need to usereselect
to prevent the filter function from being called too many times?
– MRaimon
Nov 13 '18 at 9:19
1
you can choose to use reselect if you have a lot of such computation that you perform for your app, or if there are just a few, you can choose to implement a custom memoized function yourself. You can find a lot of resources to implement memoization online
– Shubham Khatri
Nov 13 '18 at 9:22
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277367%2freact-redux-mapstatetoprops-is-called-many-times%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Anytime there is a change in state of redux or the parent component of your container re-renders the mapStateToProps
is being called. This however won't cause your component's render to call again provided the data returned by mapStateToProps and the props that it receives hasn't changed.
If you have some computation that you do in mapStateToProps
that returns a value, make sure you use a memoized function to do that computation or reselect
to write memoized selectors
so that the same value is returned for the same computation even if mapStateToProps
is called multiple times
thanks a lot! Yes, actually I call filter function inmapStateToProps
. Does this mean I need to usereselect
to prevent the filter function from being called too many times?
– MRaimon
Nov 13 '18 at 9:19
1
you can choose to use reselect if you have a lot of such computation that you perform for your app, or if there are just a few, you can choose to implement a custom memoized function yourself. You can find a lot of resources to implement memoization online
– Shubham Khatri
Nov 13 '18 at 9:22
add a comment |
Anytime there is a change in state of redux or the parent component of your container re-renders the mapStateToProps
is being called. This however won't cause your component's render to call again provided the data returned by mapStateToProps and the props that it receives hasn't changed.
If you have some computation that you do in mapStateToProps
that returns a value, make sure you use a memoized function to do that computation or reselect
to write memoized selectors
so that the same value is returned for the same computation even if mapStateToProps
is called multiple times
thanks a lot! Yes, actually I call filter function inmapStateToProps
. Does this mean I need to usereselect
to prevent the filter function from being called too many times?
– MRaimon
Nov 13 '18 at 9:19
1
you can choose to use reselect if you have a lot of such computation that you perform for your app, or if there are just a few, you can choose to implement a custom memoized function yourself. You can find a lot of resources to implement memoization online
– Shubham Khatri
Nov 13 '18 at 9:22
add a comment |
Anytime there is a change in state of redux or the parent component of your container re-renders the mapStateToProps
is being called. This however won't cause your component's render to call again provided the data returned by mapStateToProps and the props that it receives hasn't changed.
If you have some computation that you do in mapStateToProps
that returns a value, make sure you use a memoized function to do that computation or reselect
to write memoized selectors
so that the same value is returned for the same computation even if mapStateToProps
is called multiple times
Anytime there is a change in state of redux or the parent component of your container re-renders the mapStateToProps
is being called. This however won't cause your component's render to call again provided the data returned by mapStateToProps and the props that it receives hasn't changed.
If you have some computation that you do in mapStateToProps
that returns a value, make sure you use a memoized function to do that computation or reselect
to write memoized selectors
so that the same value is returned for the same computation even if mapStateToProps
is called multiple times
answered Nov 13 '18 at 9:10


Shubham KhatriShubham Khatri
81.2k1599137
81.2k1599137
thanks a lot! Yes, actually I call filter function inmapStateToProps
. Does this mean I need to usereselect
to prevent the filter function from being called too many times?
– MRaimon
Nov 13 '18 at 9:19
1
you can choose to use reselect if you have a lot of such computation that you perform for your app, or if there are just a few, you can choose to implement a custom memoized function yourself. You can find a lot of resources to implement memoization online
– Shubham Khatri
Nov 13 '18 at 9:22
add a comment |
thanks a lot! Yes, actually I call filter function inmapStateToProps
. Does this mean I need to usereselect
to prevent the filter function from being called too many times?
– MRaimon
Nov 13 '18 at 9:19
1
you can choose to use reselect if you have a lot of such computation that you perform for your app, or if there are just a few, you can choose to implement a custom memoized function yourself. You can find a lot of resources to implement memoization online
– Shubham Khatri
Nov 13 '18 at 9:22
thanks a lot! Yes, actually I call filter function in
mapStateToProps
. Does this mean I need to use reselect
to prevent the filter function from being called too many times?– MRaimon
Nov 13 '18 at 9:19
thanks a lot! Yes, actually I call filter function in
mapStateToProps
. Does this mean I need to use reselect
to prevent the filter function from being called too many times?– MRaimon
Nov 13 '18 at 9:19
1
1
you can choose to use reselect if you have a lot of such computation that you perform for your app, or if there are just a few, you can choose to implement a custom memoized function yourself. You can find a lot of resources to implement memoization online
– Shubham Khatri
Nov 13 '18 at 9:22
you can choose to use reselect if you have a lot of such computation that you perform for your app, or if there are just a few, you can choose to implement a custom memoized function yourself. You can find a lot of resources to implement memoization online
– Shubham Khatri
Nov 13 '18 at 9:22
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277367%2freact-redux-mapstatetoprops-is-called-many-times%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ws5pHQ LJPu6 c3oV7XMko8y75 NGNyK6dPNN8U
1
This is normal. That's why for heavy computations in state to props people use memoised selectors, like
reselect
.– dfsq
Nov 13 '18 at 9:09