At Runtime Add X number of ComboBoxes with SelectedItem to DataGrid (WPF)
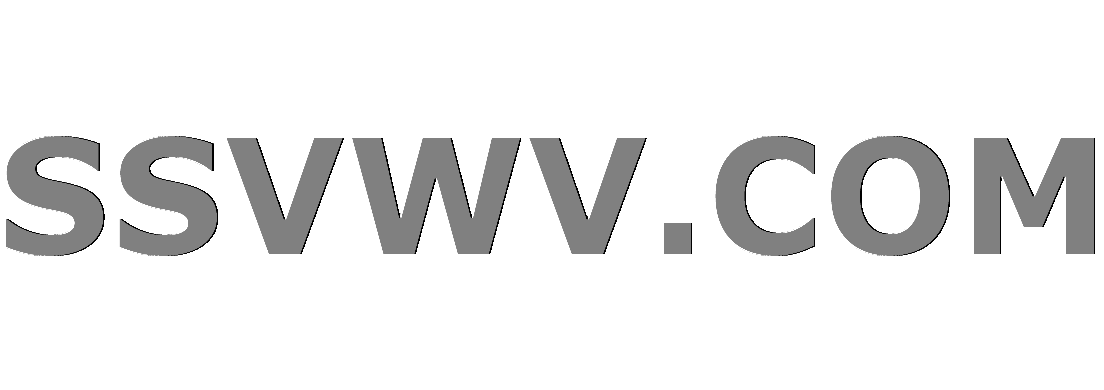
Multi tool use
I would like to create an entire row of ComboBoxes
in a DataGrid
. I have made some progress with the following:
// Declare it
private DataGridComboBoxColumn CreateCustomComboBoxDataSouce(string ColumnName)
{
string data = { "Date", "LEInt", "String" };
DataGridComboBoxColumn dgCmbCol = new DataGridComboBoxColumn();
dgCmbCol.Header = ColumnName;
dgCmbCol.ItemsSource = data;
return dgCmbCol;
}
// Later somewhere you can add this to create 20 columns:
for (int i = 0; i < 20; i++)
{
DataGridComboBoxColumn newColumn = CreateCustomComboBoxDataSouce("Column-" +i);
}
// Sadly nothing is shown unless you manually specify a new row and an
// extra column as done here below, but then you get an extra column.
DataTable table = new DataTable();
table.Columns.Add("|", typeof(string));
table.Rows.Add("");
DataGridCombo.DataContext = table;
The XAML is kept to a minimum:
<DataGrid x:Name="DataGridCombo" ItemsSource="{Binding}" Margin="0,0,0,0" />
Is there a way to set the default SelectedValue
of each ComboBox
? Within my for
loop I do have access to the desired setting. Also, is there a way to git it to show without adding the extra column? This DataGrid is being lined up with another DataGrid
that won't have that extra column.
c# wpf datagrid
add a comment |
I would like to create an entire row of ComboBoxes
in a DataGrid
. I have made some progress with the following:
// Declare it
private DataGridComboBoxColumn CreateCustomComboBoxDataSouce(string ColumnName)
{
string data = { "Date", "LEInt", "String" };
DataGridComboBoxColumn dgCmbCol = new DataGridComboBoxColumn();
dgCmbCol.Header = ColumnName;
dgCmbCol.ItemsSource = data;
return dgCmbCol;
}
// Later somewhere you can add this to create 20 columns:
for (int i = 0; i < 20; i++)
{
DataGridComboBoxColumn newColumn = CreateCustomComboBoxDataSouce("Column-" +i);
}
// Sadly nothing is shown unless you manually specify a new row and an
// extra column as done here below, but then you get an extra column.
DataTable table = new DataTable();
table.Columns.Add("|", typeof(string));
table.Rows.Add("");
DataGridCombo.DataContext = table;
The XAML is kept to a minimum:
<DataGrid x:Name="DataGridCombo" ItemsSource="{Binding}" Margin="0,0,0,0" />
Is there a way to set the default SelectedValue
of each ComboBox
? Within my for
loop I do have access to the desired setting. Also, is there a way to git it to show without adding the extra column? This DataGrid is being lined up with another DataGrid
that won't have that extra column.
c# wpf datagrid
add a comment |
I would like to create an entire row of ComboBoxes
in a DataGrid
. I have made some progress with the following:
// Declare it
private DataGridComboBoxColumn CreateCustomComboBoxDataSouce(string ColumnName)
{
string data = { "Date", "LEInt", "String" };
DataGridComboBoxColumn dgCmbCol = new DataGridComboBoxColumn();
dgCmbCol.Header = ColumnName;
dgCmbCol.ItemsSource = data;
return dgCmbCol;
}
// Later somewhere you can add this to create 20 columns:
for (int i = 0; i < 20; i++)
{
DataGridComboBoxColumn newColumn = CreateCustomComboBoxDataSouce("Column-" +i);
}
// Sadly nothing is shown unless you manually specify a new row and an
// extra column as done here below, but then you get an extra column.
DataTable table = new DataTable();
table.Columns.Add("|", typeof(string));
table.Rows.Add("");
DataGridCombo.DataContext = table;
The XAML is kept to a minimum:
<DataGrid x:Name="DataGridCombo" ItemsSource="{Binding}" Margin="0,0,0,0" />
Is there a way to set the default SelectedValue
of each ComboBox
? Within my for
loop I do have access to the desired setting. Also, is there a way to git it to show without adding the extra column? This DataGrid is being lined up with another DataGrid
that won't have that extra column.
c# wpf datagrid
I would like to create an entire row of ComboBoxes
in a DataGrid
. I have made some progress with the following:
// Declare it
private DataGridComboBoxColumn CreateCustomComboBoxDataSouce(string ColumnName)
{
string data = { "Date", "LEInt", "String" };
DataGridComboBoxColumn dgCmbCol = new DataGridComboBoxColumn();
dgCmbCol.Header = ColumnName;
dgCmbCol.ItemsSource = data;
return dgCmbCol;
}
// Later somewhere you can add this to create 20 columns:
for (int i = 0; i < 20; i++)
{
DataGridComboBoxColumn newColumn = CreateCustomComboBoxDataSouce("Column-" +i);
}
// Sadly nothing is shown unless you manually specify a new row and an
// extra column as done here below, but then you get an extra column.
DataTable table = new DataTable();
table.Columns.Add("|", typeof(string));
table.Rows.Add("");
DataGridCombo.DataContext = table;
The XAML is kept to a minimum:
<DataGrid x:Name="DataGridCombo" ItemsSource="{Binding}" Margin="0,0,0,0" />
Is there a way to set the default SelectedValue
of each ComboBox
? Within my for
loop I do have access to the desired setting. Also, is there a way to git it to show without adding the extra column? This DataGrid is being lined up with another DataGrid
that won't have that extra column.
c# wpf datagrid
c# wpf datagrid
edited Nov 14 '18 at 12:54


mm8
83.3k81931
83.3k81931
asked Nov 13 '18 at 20:01


AlanAlan
816818
816818
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Is there a way to set the default
SelectedValue
of eachComboBox
?
Add a DataColumn
to the DataTable
per DataGridComboBoxColumn
, and then set the value of the column for each row to the desired selected value:
const int n = 20;
DataTable table = new DataTable();
for (int i = 0; i<n; i++)
{
string columnName = "Column-" + i;
DataGridCombo.Columns.Add(CreateCustomComboBoxDataSouce(columnName));
table.Columns.Add(columnName, typeof(string));
}
table.Rows.Add(new object[n]);
//select some values...
table.Rows[0][1] = "LEInt";
table.Rows[0][5] = "String";
DataGridCombo.DataContext = table;
Also, is there a way to git it to show without adding the extra column?
Set the HorizontalAlignment
property to Left
to prevent the DataGrid
from stretching horizontally:
<DataGrid x:Name="DataGridCombo" ItemsSource="{Binding}" HorizontalAlignment="Left" />
Thank you so much mm8, when I tried doing that for some reason I have 20 blank columns, then 2 over (in position 22) for index[0][1]
I getLEInt
as a string with no drop down ability, and then a few more over I getString
for[0][5]
. When I set a breakpoint and examinetable
it only has the 20 items and things are set in the right place. I think the problem is that in the for loop isDataGridCombo.Columns.Add(CreateCustomComboBoxDataSouce(columnName));
which adds all those to theDataGrid
and thentable
is appended to the end. Can we to add theComboBox
toDataTable
?
– Alan
Nov 14 '18 at 17:13
I just noticed something. I normally had headers turned off, but that DataGrid has Columns namedColumn-0 -Column-19
and then appended to the end isColumn-0 -Column-19
again, and in the second set is where theLEInt
andString
are set. Normally I thought Column names had to be unique, but I guess because the DataType is different, it is allowed to repeat?
– Alan
Nov 14 '18 at 18:50
It works just fine for me so you are apparently doing something wrong.
– mm8
Nov 15 '18 at 16:04
Thank you so much mm8 for your time and effort. I created a sample empty project and pasted in the code and got the same issues. Here is a video showing what happens to me: streamable.com/0kghj
– Alan
Nov 15 '18 at 17:31
add a comment |
After a lot of working around the issue, I eventually came to the conclusion that what I was trying to do was either not possible, or unreasonable. I changed to using a StackPanel
with horizontal orientation. Here are the relevant snippets:
//XAML Layout
<StackPanel Orientation="Horizontal" VerticalAlignment="Top" Name="ComboBoxPanel" Grid.Row="1" Margin="0,0,0,0" HorizontalAlignment="Left" />
//Function to create new box and set options
private ComboBox CreateComboBox(string ColumnName, string selectedItem, int width)
{
string data = { "Date", "LEInt", "String" };
ComboBox dgCmbCol = new ComboBox();
dgCmbCol.ItemsSource = data;
dgCmbCol.Name = ColumnName;
dgCmbCol.Width = width;
dgCmbCol.SelectedItem = selectedItem;
dgCmbCol.SelectionChanged += DataTypeSelectionChanged;
return dgCmbCol;
}
// This reads a JSON file and uses it's options to set the width of all the columns
// for the DataGrid and matches the ComboBoxes to it so they all line up
dynamic TableData = JsonConvert.DeserializeObject(Config.DataLayoutDefinition);
ComboBoxPanel.Children.Clear(); //Since this is run on user interaction, clear old contents.
for (int i = 0; i < DataGridView.Columns.Count; i++)
{
int width = TableData.data[i].ColumnWidth;
if (TableData.data[i].DataType == "String")
{
width = 125;
}
else if (TableData.data[i].DataType == "Date")
{
width = 150;
}
else if (TableData.data[i].DataType == "LEInt")
{
width = 80;
}
else
{
width = 100;
}
ComboBoxPanel.Children.Add(CreateComboBox(TableData.data[i].Column.ToString(), TableData.data[i].DataType.ToString(), width));
DataGridView.Columns[i].Width = width;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53288632%2fat-runtime-add-x-number-of-comboboxes-with-selecteditem-to-datagrid-wpf%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Is there a way to set the default
SelectedValue
of eachComboBox
?
Add a DataColumn
to the DataTable
per DataGridComboBoxColumn
, and then set the value of the column for each row to the desired selected value:
const int n = 20;
DataTable table = new DataTable();
for (int i = 0; i<n; i++)
{
string columnName = "Column-" + i;
DataGridCombo.Columns.Add(CreateCustomComboBoxDataSouce(columnName));
table.Columns.Add(columnName, typeof(string));
}
table.Rows.Add(new object[n]);
//select some values...
table.Rows[0][1] = "LEInt";
table.Rows[0][5] = "String";
DataGridCombo.DataContext = table;
Also, is there a way to git it to show without adding the extra column?
Set the HorizontalAlignment
property to Left
to prevent the DataGrid
from stretching horizontally:
<DataGrid x:Name="DataGridCombo" ItemsSource="{Binding}" HorizontalAlignment="Left" />
Thank you so much mm8, when I tried doing that for some reason I have 20 blank columns, then 2 over (in position 22) for index[0][1]
I getLEInt
as a string with no drop down ability, and then a few more over I getString
for[0][5]
. When I set a breakpoint and examinetable
it only has the 20 items and things are set in the right place. I think the problem is that in the for loop isDataGridCombo.Columns.Add(CreateCustomComboBoxDataSouce(columnName));
which adds all those to theDataGrid
and thentable
is appended to the end. Can we to add theComboBox
toDataTable
?
– Alan
Nov 14 '18 at 17:13
I just noticed something. I normally had headers turned off, but that DataGrid has Columns namedColumn-0 -Column-19
and then appended to the end isColumn-0 -Column-19
again, and in the second set is where theLEInt
andString
are set. Normally I thought Column names had to be unique, but I guess because the DataType is different, it is allowed to repeat?
– Alan
Nov 14 '18 at 18:50
It works just fine for me so you are apparently doing something wrong.
– mm8
Nov 15 '18 at 16:04
Thank you so much mm8 for your time and effort. I created a sample empty project and pasted in the code and got the same issues. Here is a video showing what happens to me: streamable.com/0kghj
– Alan
Nov 15 '18 at 17:31
add a comment |
Is there a way to set the default
SelectedValue
of eachComboBox
?
Add a DataColumn
to the DataTable
per DataGridComboBoxColumn
, and then set the value of the column for each row to the desired selected value:
const int n = 20;
DataTable table = new DataTable();
for (int i = 0; i<n; i++)
{
string columnName = "Column-" + i;
DataGridCombo.Columns.Add(CreateCustomComboBoxDataSouce(columnName));
table.Columns.Add(columnName, typeof(string));
}
table.Rows.Add(new object[n]);
//select some values...
table.Rows[0][1] = "LEInt";
table.Rows[0][5] = "String";
DataGridCombo.DataContext = table;
Also, is there a way to git it to show without adding the extra column?
Set the HorizontalAlignment
property to Left
to prevent the DataGrid
from stretching horizontally:
<DataGrid x:Name="DataGridCombo" ItemsSource="{Binding}" HorizontalAlignment="Left" />
Thank you so much mm8, when I tried doing that for some reason I have 20 blank columns, then 2 over (in position 22) for index[0][1]
I getLEInt
as a string with no drop down ability, and then a few more over I getString
for[0][5]
. When I set a breakpoint and examinetable
it only has the 20 items and things are set in the right place. I think the problem is that in the for loop isDataGridCombo.Columns.Add(CreateCustomComboBoxDataSouce(columnName));
which adds all those to theDataGrid
and thentable
is appended to the end. Can we to add theComboBox
toDataTable
?
– Alan
Nov 14 '18 at 17:13
I just noticed something. I normally had headers turned off, but that DataGrid has Columns namedColumn-0 -Column-19
and then appended to the end isColumn-0 -Column-19
again, and in the second set is where theLEInt
andString
are set. Normally I thought Column names had to be unique, but I guess because the DataType is different, it is allowed to repeat?
– Alan
Nov 14 '18 at 18:50
It works just fine for me so you are apparently doing something wrong.
– mm8
Nov 15 '18 at 16:04
Thank you so much mm8 for your time and effort. I created a sample empty project and pasted in the code and got the same issues. Here is a video showing what happens to me: streamable.com/0kghj
– Alan
Nov 15 '18 at 17:31
add a comment |
Is there a way to set the default
SelectedValue
of eachComboBox
?
Add a DataColumn
to the DataTable
per DataGridComboBoxColumn
, and then set the value of the column for each row to the desired selected value:
const int n = 20;
DataTable table = new DataTable();
for (int i = 0; i<n; i++)
{
string columnName = "Column-" + i;
DataGridCombo.Columns.Add(CreateCustomComboBoxDataSouce(columnName));
table.Columns.Add(columnName, typeof(string));
}
table.Rows.Add(new object[n]);
//select some values...
table.Rows[0][1] = "LEInt";
table.Rows[0][5] = "String";
DataGridCombo.DataContext = table;
Also, is there a way to git it to show without adding the extra column?
Set the HorizontalAlignment
property to Left
to prevent the DataGrid
from stretching horizontally:
<DataGrid x:Name="DataGridCombo" ItemsSource="{Binding}" HorizontalAlignment="Left" />
Is there a way to set the default
SelectedValue
of eachComboBox
?
Add a DataColumn
to the DataTable
per DataGridComboBoxColumn
, and then set the value of the column for each row to the desired selected value:
const int n = 20;
DataTable table = new DataTable();
for (int i = 0; i<n; i++)
{
string columnName = "Column-" + i;
DataGridCombo.Columns.Add(CreateCustomComboBoxDataSouce(columnName));
table.Columns.Add(columnName, typeof(string));
}
table.Rows.Add(new object[n]);
//select some values...
table.Rows[0][1] = "LEInt";
table.Rows[0][5] = "String";
DataGridCombo.DataContext = table;
Also, is there a way to git it to show without adding the extra column?
Set the HorizontalAlignment
property to Left
to prevent the DataGrid
from stretching horizontally:
<DataGrid x:Name="DataGridCombo" ItemsSource="{Binding}" HorizontalAlignment="Left" />
answered Nov 14 '18 at 12:53


mm8mm8
83.3k81931
83.3k81931
Thank you so much mm8, when I tried doing that for some reason I have 20 blank columns, then 2 over (in position 22) for index[0][1]
I getLEInt
as a string with no drop down ability, and then a few more over I getString
for[0][5]
. When I set a breakpoint and examinetable
it only has the 20 items and things are set in the right place. I think the problem is that in the for loop isDataGridCombo.Columns.Add(CreateCustomComboBoxDataSouce(columnName));
which adds all those to theDataGrid
and thentable
is appended to the end. Can we to add theComboBox
toDataTable
?
– Alan
Nov 14 '18 at 17:13
I just noticed something. I normally had headers turned off, but that DataGrid has Columns namedColumn-0 -Column-19
and then appended to the end isColumn-0 -Column-19
again, and in the second set is where theLEInt
andString
are set. Normally I thought Column names had to be unique, but I guess because the DataType is different, it is allowed to repeat?
– Alan
Nov 14 '18 at 18:50
It works just fine for me so you are apparently doing something wrong.
– mm8
Nov 15 '18 at 16:04
Thank you so much mm8 for your time and effort. I created a sample empty project and pasted in the code and got the same issues. Here is a video showing what happens to me: streamable.com/0kghj
– Alan
Nov 15 '18 at 17:31
add a comment |
Thank you so much mm8, when I tried doing that for some reason I have 20 blank columns, then 2 over (in position 22) for index[0][1]
I getLEInt
as a string with no drop down ability, and then a few more over I getString
for[0][5]
. When I set a breakpoint and examinetable
it only has the 20 items and things are set in the right place. I think the problem is that in the for loop isDataGridCombo.Columns.Add(CreateCustomComboBoxDataSouce(columnName));
which adds all those to theDataGrid
and thentable
is appended to the end. Can we to add theComboBox
toDataTable
?
– Alan
Nov 14 '18 at 17:13
I just noticed something. I normally had headers turned off, but that DataGrid has Columns namedColumn-0 -Column-19
and then appended to the end isColumn-0 -Column-19
again, and in the second set is where theLEInt
andString
are set. Normally I thought Column names had to be unique, but I guess because the DataType is different, it is allowed to repeat?
– Alan
Nov 14 '18 at 18:50
It works just fine for me so you are apparently doing something wrong.
– mm8
Nov 15 '18 at 16:04
Thank you so much mm8 for your time and effort. I created a sample empty project and pasted in the code and got the same issues. Here is a video showing what happens to me: streamable.com/0kghj
– Alan
Nov 15 '18 at 17:31
Thank you so much mm8, when I tried doing that for some reason I have 20 blank columns, then 2 over (in position 22) for index
[0][1]
I get LEInt
as a string with no drop down ability, and then a few more over I get String
for [0][5]
. When I set a breakpoint and examine table
it only has the 20 items and things are set in the right place. I think the problem is that in the for loop is DataGridCombo.Columns.Add(CreateCustomComboBoxDataSouce(columnName));
which adds all those to the DataGrid
and then table
is appended to the end. Can we to add the ComboBox
to DataTable
?– Alan
Nov 14 '18 at 17:13
Thank you so much mm8, when I tried doing that for some reason I have 20 blank columns, then 2 over (in position 22) for index
[0][1]
I get LEInt
as a string with no drop down ability, and then a few more over I get String
for [0][5]
. When I set a breakpoint and examine table
it only has the 20 items and things are set in the right place. I think the problem is that in the for loop is DataGridCombo.Columns.Add(CreateCustomComboBoxDataSouce(columnName));
which adds all those to the DataGrid
and then table
is appended to the end. Can we to add the ComboBox
to DataTable
?– Alan
Nov 14 '18 at 17:13
I just noticed something. I normally had headers turned off, but that DataGrid has Columns named
Column-0 -Column-19
and then appended to the end is Column-0 -Column-19
again, and in the second set is where the LEInt
and String
are set. Normally I thought Column names had to be unique, but I guess because the DataType is different, it is allowed to repeat?– Alan
Nov 14 '18 at 18:50
I just noticed something. I normally had headers turned off, but that DataGrid has Columns named
Column-0 -Column-19
and then appended to the end is Column-0 -Column-19
again, and in the second set is where the LEInt
and String
are set. Normally I thought Column names had to be unique, but I guess because the DataType is different, it is allowed to repeat?– Alan
Nov 14 '18 at 18:50
It works just fine for me so you are apparently doing something wrong.
– mm8
Nov 15 '18 at 16:04
It works just fine for me so you are apparently doing something wrong.
– mm8
Nov 15 '18 at 16:04
Thank you so much mm8 for your time and effort. I created a sample empty project and pasted in the code and got the same issues. Here is a video showing what happens to me: streamable.com/0kghj
– Alan
Nov 15 '18 at 17:31
Thank you so much mm8 for your time and effort. I created a sample empty project and pasted in the code and got the same issues. Here is a video showing what happens to me: streamable.com/0kghj
– Alan
Nov 15 '18 at 17:31
add a comment |
After a lot of working around the issue, I eventually came to the conclusion that what I was trying to do was either not possible, or unreasonable. I changed to using a StackPanel
with horizontal orientation. Here are the relevant snippets:
//XAML Layout
<StackPanel Orientation="Horizontal" VerticalAlignment="Top" Name="ComboBoxPanel" Grid.Row="1" Margin="0,0,0,0" HorizontalAlignment="Left" />
//Function to create new box and set options
private ComboBox CreateComboBox(string ColumnName, string selectedItem, int width)
{
string data = { "Date", "LEInt", "String" };
ComboBox dgCmbCol = new ComboBox();
dgCmbCol.ItemsSource = data;
dgCmbCol.Name = ColumnName;
dgCmbCol.Width = width;
dgCmbCol.SelectedItem = selectedItem;
dgCmbCol.SelectionChanged += DataTypeSelectionChanged;
return dgCmbCol;
}
// This reads a JSON file and uses it's options to set the width of all the columns
// for the DataGrid and matches the ComboBoxes to it so they all line up
dynamic TableData = JsonConvert.DeserializeObject(Config.DataLayoutDefinition);
ComboBoxPanel.Children.Clear(); //Since this is run on user interaction, clear old contents.
for (int i = 0; i < DataGridView.Columns.Count; i++)
{
int width = TableData.data[i].ColumnWidth;
if (TableData.data[i].DataType == "String")
{
width = 125;
}
else if (TableData.data[i].DataType == "Date")
{
width = 150;
}
else if (TableData.data[i].DataType == "LEInt")
{
width = 80;
}
else
{
width = 100;
}
ComboBoxPanel.Children.Add(CreateComboBox(TableData.data[i].Column.ToString(), TableData.data[i].DataType.ToString(), width));
DataGridView.Columns[i].Width = width;
}
add a comment |
After a lot of working around the issue, I eventually came to the conclusion that what I was trying to do was either not possible, or unreasonable. I changed to using a StackPanel
with horizontal orientation. Here are the relevant snippets:
//XAML Layout
<StackPanel Orientation="Horizontal" VerticalAlignment="Top" Name="ComboBoxPanel" Grid.Row="1" Margin="0,0,0,0" HorizontalAlignment="Left" />
//Function to create new box and set options
private ComboBox CreateComboBox(string ColumnName, string selectedItem, int width)
{
string data = { "Date", "LEInt", "String" };
ComboBox dgCmbCol = new ComboBox();
dgCmbCol.ItemsSource = data;
dgCmbCol.Name = ColumnName;
dgCmbCol.Width = width;
dgCmbCol.SelectedItem = selectedItem;
dgCmbCol.SelectionChanged += DataTypeSelectionChanged;
return dgCmbCol;
}
// This reads a JSON file and uses it's options to set the width of all the columns
// for the DataGrid and matches the ComboBoxes to it so they all line up
dynamic TableData = JsonConvert.DeserializeObject(Config.DataLayoutDefinition);
ComboBoxPanel.Children.Clear(); //Since this is run on user interaction, clear old contents.
for (int i = 0; i < DataGridView.Columns.Count; i++)
{
int width = TableData.data[i].ColumnWidth;
if (TableData.data[i].DataType == "String")
{
width = 125;
}
else if (TableData.data[i].DataType == "Date")
{
width = 150;
}
else if (TableData.data[i].DataType == "LEInt")
{
width = 80;
}
else
{
width = 100;
}
ComboBoxPanel.Children.Add(CreateComboBox(TableData.data[i].Column.ToString(), TableData.data[i].DataType.ToString(), width));
DataGridView.Columns[i].Width = width;
}
add a comment |
After a lot of working around the issue, I eventually came to the conclusion that what I was trying to do was either not possible, or unreasonable. I changed to using a StackPanel
with horizontal orientation. Here are the relevant snippets:
//XAML Layout
<StackPanel Orientation="Horizontal" VerticalAlignment="Top" Name="ComboBoxPanel" Grid.Row="1" Margin="0,0,0,0" HorizontalAlignment="Left" />
//Function to create new box and set options
private ComboBox CreateComboBox(string ColumnName, string selectedItem, int width)
{
string data = { "Date", "LEInt", "String" };
ComboBox dgCmbCol = new ComboBox();
dgCmbCol.ItemsSource = data;
dgCmbCol.Name = ColumnName;
dgCmbCol.Width = width;
dgCmbCol.SelectedItem = selectedItem;
dgCmbCol.SelectionChanged += DataTypeSelectionChanged;
return dgCmbCol;
}
// This reads a JSON file and uses it's options to set the width of all the columns
// for the DataGrid and matches the ComboBoxes to it so they all line up
dynamic TableData = JsonConvert.DeserializeObject(Config.DataLayoutDefinition);
ComboBoxPanel.Children.Clear(); //Since this is run on user interaction, clear old contents.
for (int i = 0; i < DataGridView.Columns.Count; i++)
{
int width = TableData.data[i].ColumnWidth;
if (TableData.data[i].DataType == "String")
{
width = 125;
}
else if (TableData.data[i].DataType == "Date")
{
width = 150;
}
else if (TableData.data[i].DataType == "LEInt")
{
width = 80;
}
else
{
width = 100;
}
ComboBoxPanel.Children.Add(CreateComboBox(TableData.data[i].Column.ToString(), TableData.data[i].DataType.ToString(), width));
DataGridView.Columns[i].Width = width;
}
After a lot of working around the issue, I eventually came to the conclusion that what I was trying to do was either not possible, or unreasonable. I changed to using a StackPanel
with horizontal orientation. Here are the relevant snippets:
//XAML Layout
<StackPanel Orientation="Horizontal" VerticalAlignment="Top" Name="ComboBoxPanel" Grid.Row="1" Margin="0,0,0,0" HorizontalAlignment="Left" />
//Function to create new box and set options
private ComboBox CreateComboBox(string ColumnName, string selectedItem, int width)
{
string data = { "Date", "LEInt", "String" };
ComboBox dgCmbCol = new ComboBox();
dgCmbCol.ItemsSource = data;
dgCmbCol.Name = ColumnName;
dgCmbCol.Width = width;
dgCmbCol.SelectedItem = selectedItem;
dgCmbCol.SelectionChanged += DataTypeSelectionChanged;
return dgCmbCol;
}
// This reads a JSON file and uses it's options to set the width of all the columns
// for the DataGrid and matches the ComboBoxes to it so they all line up
dynamic TableData = JsonConvert.DeserializeObject(Config.DataLayoutDefinition);
ComboBoxPanel.Children.Clear(); //Since this is run on user interaction, clear old contents.
for (int i = 0; i < DataGridView.Columns.Count; i++)
{
int width = TableData.data[i].ColumnWidth;
if (TableData.data[i].DataType == "String")
{
width = 125;
}
else if (TableData.data[i].DataType == "Date")
{
width = 150;
}
else if (TableData.data[i].DataType == "LEInt")
{
width = 80;
}
else
{
width = 100;
}
ComboBoxPanel.Children.Add(CreateComboBox(TableData.data[i].Column.ToString(), TableData.data[i].DataType.ToString(), width));
DataGridView.Columns[i].Width = width;
}
answered Nov 14 '18 at 21:31


AlanAlan
816818
816818
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53288632%2fat-runtime-add-x-number-of-comboboxes-with-selecteditem-to-datagrid-wpf%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JBm,XkfNWoRWI SYa4xT 5Py qCPiG56EESY,pAI7