How to create a dictionary whose values are sets?
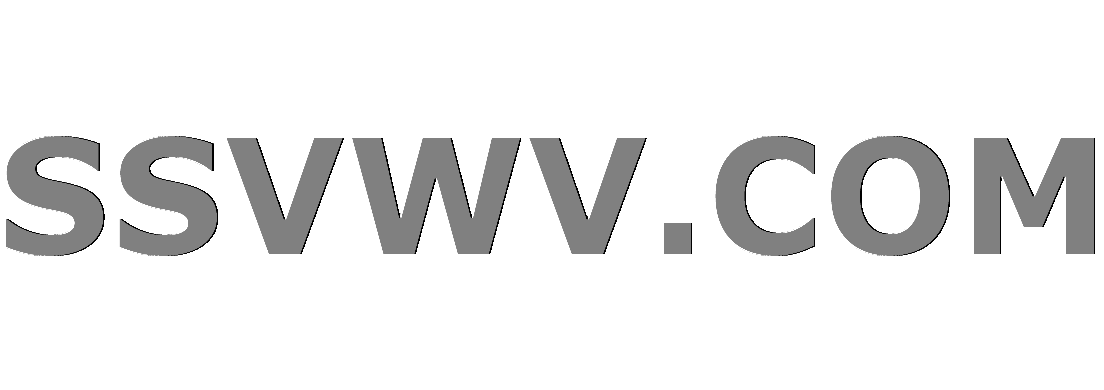
Multi tool use
I'm working on an exercise that requires me to build two dictionaries, one whose keys are country names, and the values are the GDP. This part works fine.
The second dictionary is where I'm lost, as the keys are supposed to be the letters A‐Z and the values are sets of country names. I tried using a for loop, which I've commented on below, where the issue lies.
If the user enters a string with only one letter (like A), the program should print all the countries that begin with that letter. When you run the program, however, it only prints out one country for each letter.
The text file contains 228 lines. ie:
1:Qatar:98900
2:Liechtenstein:89400
3:Luxembourg:80600
4:Bermuda:69900
5:Singapore:59700
6:Jersey:57000
etc.
And here's my code.
initials =
countries=
incomes=
dictionary={}
dictionary_2={}
keywordFile = open("raw.txt", "r")
for line in keywordFile:
line = line.upper()
line = line.strip("n")
line = line.split(":")
initials.append(line[1][0]) # first letter of second element
countries.append(line[1])
incomes.append(line[2])
for i in range(0,len(countries)):
dictionary[countries[i]] = incomes[i]
this for loop should spit out 248 values (one for each country), where the key is the initial and the value is the country name. However, it only spits out 26 values (one country for each letter in the alphabet)
for i in range(0,len(countries)):
dictionary_2[initials[i]] = countries[i]
print(dictionary_2)
while True:
inputS = str(input('Enter an initial or a country name.'))
if inputS in dictionary:
value = dictionary.get(inputS, "")
print("The per capita income of {} is {}.".format((inputS.title()), value ))
elif inputS in dictionary_2:
value = dictionary_2.get(inputS)
print("The countries that begin with the letter {} are: {}.".format(inputS, (value.title())))
elif inputS.lower() in "quit":
break
else:
print("Does not exit.")
print("End of session.")
I'd appreciate any input leading me in the right direction.
python
add a comment |
I'm working on an exercise that requires me to build two dictionaries, one whose keys are country names, and the values are the GDP. This part works fine.
The second dictionary is where I'm lost, as the keys are supposed to be the letters A‐Z and the values are sets of country names. I tried using a for loop, which I've commented on below, where the issue lies.
If the user enters a string with only one letter (like A), the program should print all the countries that begin with that letter. When you run the program, however, it only prints out one country for each letter.
The text file contains 228 lines. ie:
1:Qatar:98900
2:Liechtenstein:89400
3:Luxembourg:80600
4:Bermuda:69900
5:Singapore:59700
6:Jersey:57000
etc.
And here's my code.
initials =
countries=
incomes=
dictionary={}
dictionary_2={}
keywordFile = open("raw.txt", "r")
for line in keywordFile:
line = line.upper()
line = line.strip("n")
line = line.split(":")
initials.append(line[1][0]) # first letter of second element
countries.append(line[1])
incomes.append(line[2])
for i in range(0,len(countries)):
dictionary[countries[i]] = incomes[i]
this for loop should spit out 248 values (one for each country), where the key is the initial and the value is the country name. However, it only spits out 26 values (one country for each letter in the alphabet)
for i in range(0,len(countries)):
dictionary_2[initials[i]] = countries[i]
print(dictionary_2)
while True:
inputS = str(input('Enter an initial or a country name.'))
if inputS in dictionary:
value = dictionary.get(inputS, "")
print("The per capita income of {} is {}.".format((inputS.title()), value ))
elif inputS in dictionary_2:
value = dictionary_2.get(inputS)
print("The countries that begin with the letter {} are: {}.".format(inputS, (value.title())))
elif inputS.lower() in "quit":
break
else:
print("Does not exit.")
print("End of session.")
I'd appreciate any input leading me in the right direction.
python
add a comment |
I'm working on an exercise that requires me to build two dictionaries, one whose keys are country names, and the values are the GDP. This part works fine.
The second dictionary is where I'm lost, as the keys are supposed to be the letters A‐Z and the values are sets of country names. I tried using a for loop, which I've commented on below, where the issue lies.
If the user enters a string with only one letter (like A), the program should print all the countries that begin with that letter. When you run the program, however, it only prints out one country for each letter.
The text file contains 228 lines. ie:
1:Qatar:98900
2:Liechtenstein:89400
3:Luxembourg:80600
4:Bermuda:69900
5:Singapore:59700
6:Jersey:57000
etc.
And here's my code.
initials =
countries=
incomes=
dictionary={}
dictionary_2={}
keywordFile = open("raw.txt", "r")
for line in keywordFile:
line = line.upper()
line = line.strip("n")
line = line.split(":")
initials.append(line[1][0]) # first letter of second element
countries.append(line[1])
incomes.append(line[2])
for i in range(0,len(countries)):
dictionary[countries[i]] = incomes[i]
this for loop should spit out 248 values (one for each country), where the key is the initial and the value is the country name. However, it only spits out 26 values (one country for each letter in the alphabet)
for i in range(0,len(countries)):
dictionary_2[initials[i]] = countries[i]
print(dictionary_2)
while True:
inputS = str(input('Enter an initial or a country name.'))
if inputS in dictionary:
value = dictionary.get(inputS, "")
print("The per capita income of {} is {}.".format((inputS.title()), value ))
elif inputS in dictionary_2:
value = dictionary_2.get(inputS)
print("The countries that begin with the letter {} are: {}.".format(inputS, (value.title())))
elif inputS.lower() in "quit":
break
else:
print("Does not exit.")
print("End of session.")
I'd appreciate any input leading me in the right direction.
python
I'm working on an exercise that requires me to build two dictionaries, one whose keys are country names, and the values are the GDP. This part works fine.
The second dictionary is where I'm lost, as the keys are supposed to be the letters A‐Z and the values are sets of country names. I tried using a for loop, which I've commented on below, where the issue lies.
If the user enters a string with only one letter (like A), the program should print all the countries that begin with that letter. When you run the program, however, it only prints out one country for each letter.
The text file contains 228 lines. ie:
1:Qatar:98900
2:Liechtenstein:89400
3:Luxembourg:80600
4:Bermuda:69900
5:Singapore:59700
6:Jersey:57000
etc.
And here's my code.
initials =
countries=
incomes=
dictionary={}
dictionary_2={}
keywordFile = open("raw.txt", "r")
for line in keywordFile:
line = line.upper()
line = line.strip("n")
line = line.split(":")
initials.append(line[1][0]) # first letter of second element
countries.append(line[1])
incomes.append(line[2])
for i in range(0,len(countries)):
dictionary[countries[i]] = incomes[i]
this for loop should spit out 248 values (one for each country), where the key is the initial and the value is the country name. However, it only spits out 26 values (one country for each letter in the alphabet)
for i in range(0,len(countries)):
dictionary_2[initials[i]] = countries[i]
print(dictionary_2)
while True:
inputS = str(input('Enter an initial or a country name.'))
if inputS in dictionary:
value = dictionary.get(inputS, "")
print("The per capita income of {} is {}.".format((inputS.title()), value ))
elif inputS in dictionary_2:
value = dictionary_2.get(inputS)
print("The countries that begin with the letter {} are: {}.".format(inputS, (value.title())))
elif inputS.lower() in "quit":
break
else:
print("Does not exit.")
print("End of session.")
I'd appreciate any input leading me in the right direction.
python
python
edited Nov 12 '18 at 7:06
tripleee
88.8k12124180
88.8k12124180
asked Nov 12 '18 at 6:46


jackson3434
143
143
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
Use defaultdict
to make sure each value of your initials dict is a set, and then use the add
method. If you just use =
you'll be overwriting the initial keys value each time, defaultdict
is an easier way of using an expression like:
if initial in dict:
dict[initial].add(country)
else:
dict[initial] = {country}
See the full working example below, and also note that i'm using enumerate
instead of range(0,len(countries))
, which i'd also recommend:
#!/usr/bin/env python3
from collections import defaultdict
initials, countries, incomes = ,,
dict1 = {}
dict2 = defaultdict(set)
keywordFile = """
1:Qatar:98900
2:Liechtenstein:89400
3:Luxembourg:80600
4:Bermuda:69900
5:Singapore:59700
6:Jersey:57000
""".split("nn")
for line in keywordFile:
line = line.upper().strip("n").split(":")
initials.append(line[1][0])
countries.append(line[1])
incomes.append(line[2])
for i,country in enumerate(countries):
dict1[country] = incomes[i]
dict2[initials[i]].add(country)
print(dict2["L"])
Result:
{'LUXEMBOURG', 'LIECHTENSTEIN'}
see: https://docs.python.org/3/library/collections.html#collections.defaultdict
add a comment |
The values for dictionary2
should be such that they can contain a list of countries. One option is to use a list as the values in your dictionary. In your code, you are overwriting the values for each key whenever a new country with the same initial is to be added as the value.
Moreover, you can use the setdefault
method of the dictionary
type. This code:
dictionary2 = {}
for country in countries:
dictionary2.setdefault(country[0], ).append(country)
should be enough to create the second dictionary elegantly.
setdefault
, either returns the value for the key (in this case the key is set to the first letter of the country name) if it already exists, or inserts a new key (again, the first letter of the country) into the dictionary with a value that is an empty set .
edit
if you want your values to be set (for faster lookup/membership test), you can use the following lines:
dictionary2 = {}
for country in countries:
dictionary2.setdefault(country[0], set()).add(country)
Thesetdefault
is fine, butdefaultdict
is the preferred approach.
– VPfB
Nov 12 '18 at 8:16
add a comment |
Here's a link to a live functioning version of the OP's code online.
The keys in Python dict
objects are unique. There can only ever be one 'L'
key a single dict
. What happens in your code is that first the key/value pair 'L':'Liechtenstein'
is inserted into dictionary_2
. However, in a subsequent iteration of the for
loop, 'L':'Liechtenstein'
is overwritten by 'L':Luxembourg
. This kind of overwriting is sometimes referred to as "clobbering".
Fix
One way to get the result that you seem to be after would be to rewrite that for
loop:
for i in range(0,len(countries)):
dictionary_2[initials[i]] = dictionary_2.get(initials[i], set()) | {countries[i]}
print(dictionary_2)
Also, you have to rewrite the related elif
statement beneath that:
elif inputS in dictionary_2:
titles = ', '.join([v.title() for v in dictionary_2[inputS]])
print("The countries that begin with the letter {} are: {}.".format(inputS, titles))
Explanation
Here's a complete explanation of the dictionary_2[initials[i]] = dictionary_2.get(initials[i], set()) | {countries[i]}
line above:
dictionary_2.get(initials[i], set())
- If
initials[i]
is a key indictionary_2
, this will return the associated value. Ifinitials[i]
is not in the dictionary, it will return the empty setset()
instead.
- If
{countries[i]}
- This creates a new set with a single member in it,
countries[i]
.
- This creates a new set with a single member in it,
dictionary_2.get(initials[i], set()) | {countries[i]}
- The
|
operator adds all of the members of two sets together and returns the result.
- The
dictionary_2[initials[i]] = ...
- The right hand side of the line either creates a new set, or adds to an existing one. This bit of code assigns that newly created/expanded set back to
dictionary_2
.
- The right hand side of the line either creates a new set, or adds to an existing one. This bit of code assigns that newly created/expanded set back to
Notes
The above code sets the values of dictionary_2
as sets. If you want to use list values, use this version of the for
loop instead:
for i in range(0,len(countries)):
dictionary_2[initials[i]] = dictionary_2.get(initials[i], ) + [countries[i]]
print(dictionary_2)
add a comment |
You're very close to what you're looking for, You could populate your dictionaries respectively while looping over the contents of the file raw.txt
that you're reading. You can also read the contents of the file first and then perform the necessary operations to populate the dictionaries. You could achieve your requirement with nice oneliners in python using dict comprehensions and groupby
. Here's an example:
country_per_capita_dict = {}
letter_countries_dict = {}
keywordFile = [line.strip() for line in open('raw.txt' ,'r').readlines()]
You now have a list of all lines in the keywordFile
as follows:
['1:Qatar:98900', '2:Liechtenstein:89400', '3:Luxembourg:80600', '4:Bermuda:69900', '5:Singapore:59700', '6:Jersey:57000', '7:Libya:1000', '8:Sri Lanka:5000']
As you loop over the items, you can split(':')
and use the [1]
and [2]
index values as required.
You could use dictionary comprehension as follows:
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
Which results in:
{'Qatar': '98900', 'Libya': '1000', 'Singapore': '59700', 'Luxembourg': '80600', 'Liechtenstein': '89400', 'Bermuda': '69900', 'Jersey': '57000'}
Similarly using groupby
from itertools
you can obtain:
from itertools import groupby
country_list = country_per_capita_dict.keys()
country_list.sort()
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
Which results in the required dictionary of initial : [list of countries]
{'Q': ['Qatar'], 'S': ['Singapore'], 'B': ['Bermuda'], 'L': ['Luxembourg', 'Liechtenstein'], 'J': ['Jersey']}
A complete example is as follows:
from itertools import groupby
country_per_capita_dict = {}
letter_countries_dict = {}
keywordFile = [line.strip() for line in open('raw.txt' ,'r').readlines()]
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
country_list = country_per_capita_dict.keys()
country_list.sort()
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
print (country_per_capita_dict)
print (letter_countries_dict)
Explanation:
The line:
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
loops over the following list
['1:Qatar:98900', '2:Liechtenstein:89400', '3:Luxembourg:80600', '4:Bermuda:69900', '5:Singapore:59700', '6:Jersey:57000', '7:Libya:1000', '8:Sri Lanka:5000']
and splits each entry in the list by :
It then takes the value at index [1]
and [2]
which are the country names and the per capita value and makes them into a dictionary.
country_list = country_per_capita_dict.keys()
country_list.sort()
This line, extracts the name of all the countries from the dictionary created before into a list and sorts them alphabetically for groupby
to work correctly.
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
This lambda expression takes the input as the list of countries and groups together the names of countries where each x
starts with x[0]
into list(g)
.
groupby
only works like you describe if the the data is sorted (in this case, alphabetically). Otherwise,groupby
will split the data into a separate group every time the return value ofkey
changes, regardless of whether two groups share that samekey
value. You should definitely add a sort operation somewhere in your code. Preferably after you docountry_list = country_per_capita_dict.keys()
, since dicts aren't ordered in versions of Python earlier than 3.6
– tel
Nov 12 '18 at 13:05
Thanks @tel, Good catch! I made the necessary edits.
– Sudheesh Singanamalla
Nov 13 '18 at 3:03
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53257100%2fhow-to-create-a-dictionary-whose-values-are-sets%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Use defaultdict
to make sure each value of your initials dict is a set, and then use the add
method. If you just use =
you'll be overwriting the initial keys value each time, defaultdict
is an easier way of using an expression like:
if initial in dict:
dict[initial].add(country)
else:
dict[initial] = {country}
See the full working example below, and also note that i'm using enumerate
instead of range(0,len(countries))
, which i'd also recommend:
#!/usr/bin/env python3
from collections import defaultdict
initials, countries, incomes = ,,
dict1 = {}
dict2 = defaultdict(set)
keywordFile = """
1:Qatar:98900
2:Liechtenstein:89400
3:Luxembourg:80600
4:Bermuda:69900
5:Singapore:59700
6:Jersey:57000
""".split("nn")
for line in keywordFile:
line = line.upper().strip("n").split(":")
initials.append(line[1][0])
countries.append(line[1])
incomes.append(line[2])
for i,country in enumerate(countries):
dict1[country] = incomes[i]
dict2[initials[i]].add(country)
print(dict2["L"])
Result:
{'LUXEMBOURG', 'LIECHTENSTEIN'}
see: https://docs.python.org/3/library/collections.html#collections.defaultdict
add a comment |
Use defaultdict
to make sure each value of your initials dict is a set, and then use the add
method. If you just use =
you'll be overwriting the initial keys value each time, defaultdict
is an easier way of using an expression like:
if initial in dict:
dict[initial].add(country)
else:
dict[initial] = {country}
See the full working example below, and also note that i'm using enumerate
instead of range(0,len(countries))
, which i'd also recommend:
#!/usr/bin/env python3
from collections import defaultdict
initials, countries, incomes = ,,
dict1 = {}
dict2 = defaultdict(set)
keywordFile = """
1:Qatar:98900
2:Liechtenstein:89400
3:Luxembourg:80600
4:Bermuda:69900
5:Singapore:59700
6:Jersey:57000
""".split("nn")
for line in keywordFile:
line = line.upper().strip("n").split(":")
initials.append(line[1][0])
countries.append(line[1])
incomes.append(line[2])
for i,country in enumerate(countries):
dict1[country] = incomes[i]
dict2[initials[i]].add(country)
print(dict2["L"])
Result:
{'LUXEMBOURG', 'LIECHTENSTEIN'}
see: https://docs.python.org/3/library/collections.html#collections.defaultdict
add a comment |
Use defaultdict
to make sure each value of your initials dict is a set, and then use the add
method. If you just use =
you'll be overwriting the initial keys value each time, defaultdict
is an easier way of using an expression like:
if initial in dict:
dict[initial].add(country)
else:
dict[initial] = {country}
See the full working example below, and also note that i'm using enumerate
instead of range(0,len(countries))
, which i'd also recommend:
#!/usr/bin/env python3
from collections import defaultdict
initials, countries, incomes = ,,
dict1 = {}
dict2 = defaultdict(set)
keywordFile = """
1:Qatar:98900
2:Liechtenstein:89400
3:Luxembourg:80600
4:Bermuda:69900
5:Singapore:59700
6:Jersey:57000
""".split("nn")
for line in keywordFile:
line = line.upper().strip("n").split(":")
initials.append(line[1][0])
countries.append(line[1])
incomes.append(line[2])
for i,country in enumerate(countries):
dict1[country] = incomes[i]
dict2[initials[i]].add(country)
print(dict2["L"])
Result:
{'LUXEMBOURG', 'LIECHTENSTEIN'}
see: https://docs.python.org/3/library/collections.html#collections.defaultdict
Use defaultdict
to make sure each value of your initials dict is a set, and then use the add
method. If you just use =
you'll be overwriting the initial keys value each time, defaultdict
is an easier way of using an expression like:
if initial in dict:
dict[initial].add(country)
else:
dict[initial] = {country}
See the full working example below, and also note that i'm using enumerate
instead of range(0,len(countries))
, which i'd also recommend:
#!/usr/bin/env python3
from collections import defaultdict
initials, countries, incomes = ,,
dict1 = {}
dict2 = defaultdict(set)
keywordFile = """
1:Qatar:98900
2:Liechtenstein:89400
3:Luxembourg:80600
4:Bermuda:69900
5:Singapore:59700
6:Jersey:57000
""".split("nn")
for line in keywordFile:
line = line.upper().strip("n").split(":")
initials.append(line[1][0])
countries.append(line[1])
incomes.append(line[2])
for i,country in enumerate(countries):
dict1[country] = incomes[i]
dict2[initials[i]].add(country)
print(dict2["L"])
Result:
{'LUXEMBOURG', 'LIECHTENSTEIN'}
see: https://docs.python.org/3/library/collections.html#collections.defaultdict
edited Nov 12 '18 at 7:29
answered Nov 12 '18 at 7:10
Zhenhir
30816
30816
add a comment |
add a comment |
The values for dictionary2
should be such that they can contain a list of countries. One option is to use a list as the values in your dictionary. In your code, you are overwriting the values for each key whenever a new country with the same initial is to be added as the value.
Moreover, you can use the setdefault
method of the dictionary
type. This code:
dictionary2 = {}
for country in countries:
dictionary2.setdefault(country[0], ).append(country)
should be enough to create the second dictionary elegantly.
setdefault
, either returns the value for the key (in this case the key is set to the first letter of the country name) if it already exists, or inserts a new key (again, the first letter of the country) into the dictionary with a value that is an empty set .
edit
if you want your values to be set (for faster lookup/membership test), you can use the following lines:
dictionary2 = {}
for country in countries:
dictionary2.setdefault(country[0], set()).add(country)
Thesetdefault
is fine, butdefaultdict
is the preferred approach.
– VPfB
Nov 12 '18 at 8:16
add a comment |
The values for dictionary2
should be such that they can contain a list of countries. One option is to use a list as the values in your dictionary. In your code, you are overwriting the values for each key whenever a new country with the same initial is to be added as the value.
Moreover, you can use the setdefault
method of the dictionary
type. This code:
dictionary2 = {}
for country in countries:
dictionary2.setdefault(country[0], ).append(country)
should be enough to create the second dictionary elegantly.
setdefault
, either returns the value for the key (in this case the key is set to the first letter of the country name) if it already exists, or inserts a new key (again, the first letter of the country) into the dictionary with a value that is an empty set .
edit
if you want your values to be set (for faster lookup/membership test), you can use the following lines:
dictionary2 = {}
for country in countries:
dictionary2.setdefault(country[0], set()).add(country)
Thesetdefault
is fine, butdefaultdict
is the preferred approach.
– VPfB
Nov 12 '18 at 8:16
add a comment |
The values for dictionary2
should be such that they can contain a list of countries. One option is to use a list as the values in your dictionary. In your code, you are overwriting the values for each key whenever a new country with the same initial is to be added as the value.
Moreover, you can use the setdefault
method of the dictionary
type. This code:
dictionary2 = {}
for country in countries:
dictionary2.setdefault(country[0], ).append(country)
should be enough to create the second dictionary elegantly.
setdefault
, either returns the value for the key (in this case the key is set to the first letter of the country name) if it already exists, or inserts a new key (again, the first letter of the country) into the dictionary with a value that is an empty set .
edit
if you want your values to be set (for faster lookup/membership test), you can use the following lines:
dictionary2 = {}
for country in countries:
dictionary2.setdefault(country[0], set()).add(country)
The values for dictionary2
should be such that they can contain a list of countries. One option is to use a list as the values in your dictionary. In your code, you are overwriting the values for each key whenever a new country with the same initial is to be added as the value.
Moreover, you can use the setdefault
method of the dictionary
type. This code:
dictionary2 = {}
for country in countries:
dictionary2.setdefault(country[0], ).append(country)
should be enough to create the second dictionary elegantly.
setdefault
, either returns the value for the key (in this case the key is set to the first letter of the country name) if it already exists, or inserts a new key (again, the first letter of the country) into the dictionary with a value that is an empty set .
edit
if you want your values to be set (for faster lookup/membership test), you can use the following lines:
dictionary2 = {}
for country in countries:
dictionary2.setdefault(country[0], set()).add(country)
edited Nov 12 '18 at 7:37
answered Nov 12 '18 at 7:12
SMir
3471515
3471515
Thesetdefault
is fine, butdefaultdict
is the preferred approach.
– VPfB
Nov 12 '18 at 8:16
add a comment |
Thesetdefault
is fine, butdefaultdict
is the preferred approach.
– VPfB
Nov 12 '18 at 8:16
The
setdefault
is fine, but defaultdict
is the preferred approach.– VPfB
Nov 12 '18 at 8:16
The
setdefault
is fine, but defaultdict
is the preferred approach.– VPfB
Nov 12 '18 at 8:16
add a comment |
Here's a link to a live functioning version of the OP's code online.
The keys in Python dict
objects are unique. There can only ever be one 'L'
key a single dict
. What happens in your code is that first the key/value pair 'L':'Liechtenstein'
is inserted into dictionary_2
. However, in a subsequent iteration of the for
loop, 'L':'Liechtenstein'
is overwritten by 'L':Luxembourg
. This kind of overwriting is sometimes referred to as "clobbering".
Fix
One way to get the result that you seem to be after would be to rewrite that for
loop:
for i in range(0,len(countries)):
dictionary_2[initials[i]] = dictionary_2.get(initials[i], set()) | {countries[i]}
print(dictionary_2)
Also, you have to rewrite the related elif
statement beneath that:
elif inputS in dictionary_2:
titles = ', '.join([v.title() for v in dictionary_2[inputS]])
print("The countries that begin with the letter {} are: {}.".format(inputS, titles))
Explanation
Here's a complete explanation of the dictionary_2[initials[i]] = dictionary_2.get(initials[i], set()) | {countries[i]}
line above:
dictionary_2.get(initials[i], set())
- If
initials[i]
is a key indictionary_2
, this will return the associated value. Ifinitials[i]
is not in the dictionary, it will return the empty setset()
instead.
- If
{countries[i]}
- This creates a new set with a single member in it,
countries[i]
.
- This creates a new set with a single member in it,
dictionary_2.get(initials[i], set()) | {countries[i]}
- The
|
operator adds all of the members of two sets together and returns the result.
- The
dictionary_2[initials[i]] = ...
- The right hand side of the line either creates a new set, or adds to an existing one. This bit of code assigns that newly created/expanded set back to
dictionary_2
.
- The right hand side of the line either creates a new set, or adds to an existing one. This bit of code assigns that newly created/expanded set back to
Notes
The above code sets the values of dictionary_2
as sets. If you want to use list values, use this version of the for
loop instead:
for i in range(0,len(countries)):
dictionary_2[initials[i]] = dictionary_2.get(initials[i], ) + [countries[i]]
print(dictionary_2)
add a comment |
Here's a link to a live functioning version of the OP's code online.
The keys in Python dict
objects are unique. There can only ever be one 'L'
key a single dict
. What happens in your code is that first the key/value pair 'L':'Liechtenstein'
is inserted into dictionary_2
. However, in a subsequent iteration of the for
loop, 'L':'Liechtenstein'
is overwritten by 'L':Luxembourg
. This kind of overwriting is sometimes referred to as "clobbering".
Fix
One way to get the result that you seem to be after would be to rewrite that for
loop:
for i in range(0,len(countries)):
dictionary_2[initials[i]] = dictionary_2.get(initials[i], set()) | {countries[i]}
print(dictionary_2)
Also, you have to rewrite the related elif
statement beneath that:
elif inputS in dictionary_2:
titles = ', '.join([v.title() for v in dictionary_2[inputS]])
print("The countries that begin with the letter {} are: {}.".format(inputS, titles))
Explanation
Here's a complete explanation of the dictionary_2[initials[i]] = dictionary_2.get(initials[i], set()) | {countries[i]}
line above:
dictionary_2.get(initials[i], set())
- If
initials[i]
is a key indictionary_2
, this will return the associated value. Ifinitials[i]
is not in the dictionary, it will return the empty setset()
instead.
- If
{countries[i]}
- This creates a new set with a single member in it,
countries[i]
.
- This creates a new set with a single member in it,
dictionary_2.get(initials[i], set()) | {countries[i]}
- The
|
operator adds all of the members of two sets together and returns the result.
- The
dictionary_2[initials[i]] = ...
- The right hand side of the line either creates a new set, or adds to an existing one. This bit of code assigns that newly created/expanded set back to
dictionary_2
.
- The right hand side of the line either creates a new set, or adds to an existing one. This bit of code assigns that newly created/expanded set back to
Notes
The above code sets the values of dictionary_2
as sets. If you want to use list values, use this version of the for
loop instead:
for i in range(0,len(countries)):
dictionary_2[initials[i]] = dictionary_2.get(initials[i], ) + [countries[i]]
print(dictionary_2)
add a comment |
Here's a link to a live functioning version of the OP's code online.
The keys in Python dict
objects are unique. There can only ever be one 'L'
key a single dict
. What happens in your code is that first the key/value pair 'L':'Liechtenstein'
is inserted into dictionary_2
. However, in a subsequent iteration of the for
loop, 'L':'Liechtenstein'
is overwritten by 'L':Luxembourg
. This kind of overwriting is sometimes referred to as "clobbering".
Fix
One way to get the result that you seem to be after would be to rewrite that for
loop:
for i in range(0,len(countries)):
dictionary_2[initials[i]] = dictionary_2.get(initials[i], set()) | {countries[i]}
print(dictionary_2)
Also, you have to rewrite the related elif
statement beneath that:
elif inputS in dictionary_2:
titles = ', '.join([v.title() for v in dictionary_2[inputS]])
print("The countries that begin with the letter {} are: {}.".format(inputS, titles))
Explanation
Here's a complete explanation of the dictionary_2[initials[i]] = dictionary_2.get(initials[i], set()) | {countries[i]}
line above:
dictionary_2.get(initials[i], set())
- If
initials[i]
is a key indictionary_2
, this will return the associated value. Ifinitials[i]
is not in the dictionary, it will return the empty setset()
instead.
- If
{countries[i]}
- This creates a new set with a single member in it,
countries[i]
.
- This creates a new set with a single member in it,
dictionary_2.get(initials[i], set()) | {countries[i]}
- The
|
operator adds all of the members of two sets together and returns the result.
- The
dictionary_2[initials[i]] = ...
- The right hand side of the line either creates a new set, or adds to an existing one. This bit of code assigns that newly created/expanded set back to
dictionary_2
.
- The right hand side of the line either creates a new set, or adds to an existing one. This bit of code assigns that newly created/expanded set back to
Notes
The above code sets the values of dictionary_2
as sets. If you want to use list values, use this version of the for
loop instead:
for i in range(0,len(countries)):
dictionary_2[initials[i]] = dictionary_2.get(initials[i], ) + [countries[i]]
print(dictionary_2)
Here's a link to a live functioning version of the OP's code online.
The keys in Python dict
objects are unique. There can only ever be one 'L'
key a single dict
. What happens in your code is that first the key/value pair 'L':'Liechtenstein'
is inserted into dictionary_2
. However, in a subsequent iteration of the for
loop, 'L':'Liechtenstein'
is overwritten by 'L':Luxembourg
. This kind of overwriting is sometimes referred to as "clobbering".
Fix
One way to get the result that you seem to be after would be to rewrite that for
loop:
for i in range(0,len(countries)):
dictionary_2[initials[i]] = dictionary_2.get(initials[i], set()) | {countries[i]}
print(dictionary_2)
Also, you have to rewrite the related elif
statement beneath that:
elif inputS in dictionary_2:
titles = ', '.join([v.title() for v in dictionary_2[inputS]])
print("The countries that begin with the letter {} are: {}.".format(inputS, titles))
Explanation
Here's a complete explanation of the dictionary_2[initials[i]] = dictionary_2.get(initials[i], set()) | {countries[i]}
line above:
dictionary_2.get(initials[i], set())
- If
initials[i]
is a key indictionary_2
, this will return the associated value. Ifinitials[i]
is not in the dictionary, it will return the empty setset()
instead.
- If
{countries[i]}
- This creates a new set with a single member in it,
countries[i]
.
- This creates a new set with a single member in it,
dictionary_2.get(initials[i], set()) | {countries[i]}
- The
|
operator adds all of the members of two sets together and returns the result.
- The
dictionary_2[initials[i]] = ...
- The right hand side of the line either creates a new set, or adds to an existing one. This bit of code assigns that newly created/expanded set back to
dictionary_2
.
- The right hand side of the line either creates a new set, or adds to an existing one. This bit of code assigns that newly created/expanded set back to
Notes
The above code sets the values of dictionary_2
as sets. If you want to use list values, use this version of the for
loop instead:
for i in range(0,len(countries)):
dictionary_2[initials[i]] = dictionary_2.get(initials[i], ) + [countries[i]]
print(dictionary_2)
edited Nov 12 '18 at 7:51
answered Nov 12 '18 at 7:02


tel
6,15821430
6,15821430
add a comment |
add a comment |
You're very close to what you're looking for, You could populate your dictionaries respectively while looping over the contents of the file raw.txt
that you're reading. You can also read the contents of the file first and then perform the necessary operations to populate the dictionaries. You could achieve your requirement with nice oneliners in python using dict comprehensions and groupby
. Here's an example:
country_per_capita_dict = {}
letter_countries_dict = {}
keywordFile = [line.strip() for line in open('raw.txt' ,'r').readlines()]
You now have a list of all lines in the keywordFile
as follows:
['1:Qatar:98900', '2:Liechtenstein:89400', '3:Luxembourg:80600', '4:Bermuda:69900', '5:Singapore:59700', '6:Jersey:57000', '7:Libya:1000', '8:Sri Lanka:5000']
As you loop over the items, you can split(':')
and use the [1]
and [2]
index values as required.
You could use dictionary comprehension as follows:
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
Which results in:
{'Qatar': '98900', 'Libya': '1000', 'Singapore': '59700', 'Luxembourg': '80600', 'Liechtenstein': '89400', 'Bermuda': '69900', 'Jersey': '57000'}
Similarly using groupby
from itertools
you can obtain:
from itertools import groupby
country_list = country_per_capita_dict.keys()
country_list.sort()
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
Which results in the required dictionary of initial : [list of countries]
{'Q': ['Qatar'], 'S': ['Singapore'], 'B': ['Bermuda'], 'L': ['Luxembourg', 'Liechtenstein'], 'J': ['Jersey']}
A complete example is as follows:
from itertools import groupby
country_per_capita_dict = {}
letter_countries_dict = {}
keywordFile = [line.strip() for line in open('raw.txt' ,'r').readlines()]
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
country_list = country_per_capita_dict.keys()
country_list.sort()
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
print (country_per_capita_dict)
print (letter_countries_dict)
Explanation:
The line:
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
loops over the following list
['1:Qatar:98900', '2:Liechtenstein:89400', '3:Luxembourg:80600', '4:Bermuda:69900', '5:Singapore:59700', '6:Jersey:57000', '7:Libya:1000', '8:Sri Lanka:5000']
and splits each entry in the list by :
It then takes the value at index [1]
and [2]
which are the country names and the per capita value and makes them into a dictionary.
country_list = country_per_capita_dict.keys()
country_list.sort()
This line, extracts the name of all the countries from the dictionary created before into a list and sorts them alphabetically for groupby
to work correctly.
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
This lambda expression takes the input as the list of countries and groups together the names of countries where each x
starts with x[0]
into list(g)
.
groupby
only works like you describe if the the data is sorted (in this case, alphabetically). Otherwise,groupby
will split the data into a separate group every time the return value ofkey
changes, regardless of whether two groups share that samekey
value. You should definitely add a sort operation somewhere in your code. Preferably after you docountry_list = country_per_capita_dict.keys()
, since dicts aren't ordered in versions of Python earlier than 3.6
– tel
Nov 12 '18 at 13:05
Thanks @tel, Good catch! I made the necessary edits.
– Sudheesh Singanamalla
Nov 13 '18 at 3:03
add a comment |
You're very close to what you're looking for, You could populate your dictionaries respectively while looping over the contents of the file raw.txt
that you're reading. You can also read the contents of the file first and then perform the necessary operations to populate the dictionaries. You could achieve your requirement with nice oneliners in python using dict comprehensions and groupby
. Here's an example:
country_per_capita_dict = {}
letter_countries_dict = {}
keywordFile = [line.strip() for line in open('raw.txt' ,'r').readlines()]
You now have a list of all lines in the keywordFile
as follows:
['1:Qatar:98900', '2:Liechtenstein:89400', '3:Luxembourg:80600', '4:Bermuda:69900', '5:Singapore:59700', '6:Jersey:57000', '7:Libya:1000', '8:Sri Lanka:5000']
As you loop over the items, you can split(':')
and use the [1]
and [2]
index values as required.
You could use dictionary comprehension as follows:
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
Which results in:
{'Qatar': '98900', 'Libya': '1000', 'Singapore': '59700', 'Luxembourg': '80600', 'Liechtenstein': '89400', 'Bermuda': '69900', 'Jersey': '57000'}
Similarly using groupby
from itertools
you can obtain:
from itertools import groupby
country_list = country_per_capita_dict.keys()
country_list.sort()
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
Which results in the required dictionary of initial : [list of countries]
{'Q': ['Qatar'], 'S': ['Singapore'], 'B': ['Bermuda'], 'L': ['Luxembourg', 'Liechtenstein'], 'J': ['Jersey']}
A complete example is as follows:
from itertools import groupby
country_per_capita_dict = {}
letter_countries_dict = {}
keywordFile = [line.strip() for line in open('raw.txt' ,'r').readlines()]
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
country_list = country_per_capita_dict.keys()
country_list.sort()
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
print (country_per_capita_dict)
print (letter_countries_dict)
Explanation:
The line:
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
loops over the following list
['1:Qatar:98900', '2:Liechtenstein:89400', '3:Luxembourg:80600', '4:Bermuda:69900', '5:Singapore:59700', '6:Jersey:57000', '7:Libya:1000', '8:Sri Lanka:5000']
and splits each entry in the list by :
It then takes the value at index [1]
and [2]
which are the country names and the per capita value and makes them into a dictionary.
country_list = country_per_capita_dict.keys()
country_list.sort()
This line, extracts the name of all the countries from the dictionary created before into a list and sorts them alphabetically for groupby
to work correctly.
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
This lambda expression takes the input as the list of countries and groups together the names of countries where each x
starts with x[0]
into list(g)
.
groupby
only works like you describe if the the data is sorted (in this case, alphabetically). Otherwise,groupby
will split the data into a separate group every time the return value ofkey
changes, regardless of whether two groups share that samekey
value. You should definitely add a sort operation somewhere in your code. Preferably after you docountry_list = country_per_capita_dict.keys()
, since dicts aren't ordered in versions of Python earlier than 3.6
– tel
Nov 12 '18 at 13:05
Thanks @tel, Good catch! I made the necessary edits.
– Sudheesh Singanamalla
Nov 13 '18 at 3:03
add a comment |
You're very close to what you're looking for, You could populate your dictionaries respectively while looping over the contents of the file raw.txt
that you're reading. You can also read the contents of the file first and then perform the necessary operations to populate the dictionaries. You could achieve your requirement with nice oneliners in python using dict comprehensions and groupby
. Here's an example:
country_per_capita_dict = {}
letter_countries_dict = {}
keywordFile = [line.strip() for line in open('raw.txt' ,'r').readlines()]
You now have a list of all lines in the keywordFile
as follows:
['1:Qatar:98900', '2:Liechtenstein:89400', '3:Luxembourg:80600', '4:Bermuda:69900', '5:Singapore:59700', '6:Jersey:57000', '7:Libya:1000', '8:Sri Lanka:5000']
As you loop over the items, you can split(':')
and use the [1]
and [2]
index values as required.
You could use dictionary comprehension as follows:
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
Which results in:
{'Qatar': '98900', 'Libya': '1000', 'Singapore': '59700', 'Luxembourg': '80600', 'Liechtenstein': '89400', 'Bermuda': '69900', 'Jersey': '57000'}
Similarly using groupby
from itertools
you can obtain:
from itertools import groupby
country_list = country_per_capita_dict.keys()
country_list.sort()
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
Which results in the required dictionary of initial : [list of countries]
{'Q': ['Qatar'], 'S': ['Singapore'], 'B': ['Bermuda'], 'L': ['Luxembourg', 'Liechtenstein'], 'J': ['Jersey']}
A complete example is as follows:
from itertools import groupby
country_per_capita_dict = {}
letter_countries_dict = {}
keywordFile = [line.strip() for line in open('raw.txt' ,'r').readlines()]
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
country_list = country_per_capita_dict.keys()
country_list.sort()
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
print (country_per_capita_dict)
print (letter_countries_dict)
Explanation:
The line:
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
loops over the following list
['1:Qatar:98900', '2:Liechtenstein:89400', '3:Luxembourg:80600', '4:Bermuda:69900', '5:Singapore:59700', '6:Jersey:57000', '7:Libya:1000', '8:Sri Lanka:5000']
and splits each entry in the list by :
It then takes the value at index [1]
and [2]
which are the country names and the per capita value and makes them into a dictionary.
country_list = country_per_capita_dict.keys()
country_list.sort()
This line, extracts the name of all the countries from the dictionary created before into a list and sorts them alphabetically for groupby
to work correctly.
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
This lambda expression takes the input as the list of countries and groups together the names of countries where each x
starts with x[0]
into list(g)
.
You're very close to what you're looking for, You could populate your dictionaries respectively while looping over the contents of the file raw.txt
that you're reading. You can also read the contents of the file first and then perform the necessary operations to populate the dictionaries. You could achieve your requirement with nice oneliners in python using dict comprehensions and groupby
. Here's an example:
country_per_capita_dict = {}
letter_countries_dict = {}
keywordFile = [line.strip() for line in open('raw.txt' ,'r').readlines()]
You now have a list of all lines in the keywordFile
as follows:
['1:Qatar:98900', '2:Liechtenstein:89400', '3:Luxembourg:80600', '4:Bermuda:69900', '5:Singapore:59700', '6:Jersey:57000', '7:Libya:1000', '8:Sri Lanka:5000']
As you loop over the items, you can split(':')
and use the [1]
and [2]
index values as required.
You could use dictionary comprehension as follows:
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
Which results in:
{'Qatar': '98900', 'Libya': '1000', 'Singapore': '59700', 'Luxembourg': '80600', 'Liechtenstein': '89400', 'Bermuda': '69900', 'Jersey': '57000'}
Similarly using groupby
from itertools
you can obtain:
from itertools import groupby
country_list = country_per_capita_dict.keys()
country_list.sort()
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
Which results in the required dictionary of initial : [list of countries]
{'Q': ['Qatar'], 'S': ['Singapore'], 'B': ['Bermuda'], 'L': ['Luxembourg', 'Liechtenstein'], 'J': ['Jersey']}
A complete example is as follows:
from itertools import groupby
country_per_capita_dict = {}
letter_countries_dict = {}
keywordFile = [line.strip() for line in open('raw.txt' ,'r').readlines()]
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
country_list = country_per_capita_dict.keys()
country_list.sort()
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
print (country_per_capita_dict)
print (letter_countries_dict)
Explanation:
The line:
country_per_capita_dict = {entry.split(':')[1] : entry.split(':')[2] for entry in keywordFile}
loops over the following list
['1:Qatar:98900', '2:Liechtenstein:89400', '3:Luxembourg:80600', '4:Bermuda:69900', '5:Singapore:59700', '6:Jersey:57000', '7:Libya:1000', '8:Sri Lanka:5000']
and splits each entry in the list by :
It then takes the value at index [1]
and [2]
which are the country names and the per capita value and makes them into a dictionary.
country_list = country_per_capita_dict.keys()
country_list.sort()
This line, extracts the name of all the countries from the dictionary created before into a list and sorts them alphabetically for groupby
to work correctly.
letter_countries_dict = {k: list(g) for k,g in groupby(country_list, key=lambda x:x[0]) }
This lambda expression takes the input as the list of countries and groups together the names of countries where each x
starts with x[0]
into list(g)
.
edited Nov 13 '18 at 3:03
answered Nov 12 '18 at 7:28


Sudheesh Singanamalla
1,53621024
1,53621024
groupby
only works like you describe if the the data is sorted (in this case, alphabetically). Otherwise,groupby
will split the data into a separate group every time the return value ofkey
changes, regardless of whether two groups share that samekey
value. You should definitely add a sort operation somewhere in your code. Preferably after you docountry_list = country_per_capita_dict.keys()
, since dicts aren't ordered in versions of Python earlier than 3.6
– tel
Nov 12 '18 at 13:05
Thanks @tel, Good catch! I made the necessary edits.
– Sudheesh Singanamalla
Nov 13 '18 at 3:03
add a comment |
groupby
only works like you describe if the the data is sorted (in this case, alphabetically). Otherwise,groupby
will split the data into a separate group every time the return value ofkey
changes, regardless of whether two groups share that samekey
value. You should definitely add a sort operation somewhere in your code. Preferably after you docountry_list = country_per_capita_dict.keys()
, since dicts aren't ordered in versions of Python earlier than 3.6
– tel
Nov 12 '18 at 13:05
Thanks @tel, Good catch! I made the necessary edits.
– Sudheesh Singanamalla
Nov 13 '18 at 3:03
groupby
only works like you describe if the the data is sorted (in this case, alphabetically). Otherwise, groupby
will split the data into a separate group every time the return value of key
changes, regardless of whether two groups share that same key
value. You should definitely add a sort operation somewhere in your code. Preferably after you do country_list = country_per_capita_dict.keys()
, since dicts aren't ordered in versions of Python earlier than 3.6– tel
Nov 12 '18 at 13:05
groupby
only works like you describe if the the data is sorted (in this case, alphabetically). Otherwise, groupby
will split the data into a separate group every time the return value of key
changes, regardless of whether two groups share that same key
value. You should definitely add a sort operation somewhere in your code. Preferably after you do country_list = country_per_capita_dict.keys()
, since dicts aren't ordered in versions of Python earlier than 3.6– tel
Nov 12 '18 at 13:05
Thanks @tel, Good catch! I made the necessary edits.
– Sudheesh Singanamalla
Nov 13 '18 at 3:03
Thanks @tel, Good catch! I made the necessary edits.
– Sudheesh Singanamalla
Nov 13 '18 at 3:03
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53257100%2fhow-to-create-a-dictionary-whose-values-are-sets%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5Yr92,G yCy TFTl,V3 7g06,GrAeLFsO1Zp834dbH8PIC,3w5f9,8Aa8vpmXuezsa4qq nuSc lojRU3AOY,k,2,1t,D