'list' object has no attribute 'lower' in Python
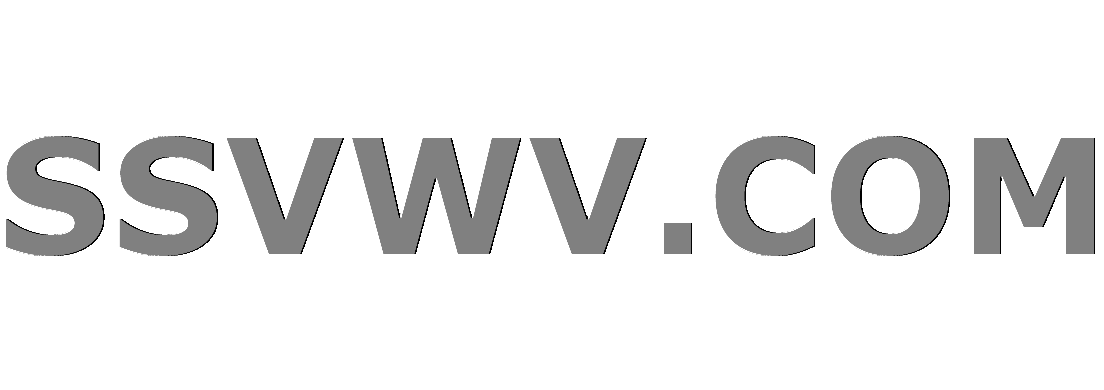
Multi tool use
function anagrams(s1, s2) is a Boolean valued function, which returns true just in case the string s1 contains the same letters as string s2 but in a different order. The function should be case insensitive --- in other words it should return the same value if any letters in either s1 or s2 are changed from upper to lower case or from lower to upper case. You may assume that the input strings contain only letters.
The function find_all_anagrams(string) takes a string as input and returns a list of all words in the file english_words.txt that are anagrams of the input string.
the function should return a list [word1, ..., wordN] such that each word in this list is a word in the dictionary file such that the value function anagrams(string, word) are True
def anagrams( string1, string2 ):
str_1 = string1.lower()
str_2 = string2.lower()
if str_1 == str_2:
return False
else:
list_1 = list( str_1 )
list_1.sort()
list_2 = list( str_2 )
list_2.sort()
return list_1 == list_2
def find_all_anagrams( string ):
with open("english_words.txt") as f:
word_list =
for line in f.readlines():
word_list.append(line.strip())
list1 = [i.split() for i in word_list]
for j in list1:
if anagrams( string, j ) == True:
return list1
else:
return
ERROR kept saying this: AttributeError: 'list' object has no attribute 'lower'
for example,word_list contains:
['pyruvates', 'python', 'pythoness', 'pythonesses', 'pythonic', 'pythons', 'pyuria', 'pyurias', 'pyx', 'pyxes']
Expected output below
Part of the txt file shown on the right:
Update:
I think I just solved it,here are my codes:
def find_all_anagrams( string ):
list1 =
with open("english_words.txt") as f:
word_list =
for line in f.readlines():
word_list.append(line.strip())
for i in word_list:
if anagrams( string, i ):
list1.append(i)
return list1
python data-science data-analysis
|
show 1 more comment
function anagrams(s1, s2) is a Boolean valued function, which returns true just in case the string s1 contains the same letters as string s2 but in a different order. The function should be case insensitive --- in other words it should return the same value if any letters in either s1 or s2 are changed from upper to lower case or from lower to upper case. You may assume that the input strings contain only letters.
The function find_all_anagrams(string) takes a string as input and returns a list of all words in the file english_words.txt that are anagrams of the input string.
the function should return a list [word1, ..., wordN] such that each word in this list is a word in the dictionary file such that the value function anagrams(string, word) are True
def anagrams( string1, string2 ):
str_1 = string1.lower()
str_2 = string2.lower()
if str_1 == str_2:
return False
else:
list_1 = list( str_1 )
list_1.sort()
list_2 = list( str_2 )
list_2.sort()
return list_1 == list_2
def find_all_anagrams( string ):
with open("english_words.txt") as f:
word_list =
for line in f.readlines():
word_list.append(line.strip())
list1 = [i.split() for i in word_list]
for j in list1:
if anagrams( string, j ) == True:
return list1
else:
return
ERROR kept saying this: AttributeError: 'list' object has no attribute 'lower'
for example,word_list contains:
['pyruvates', 'python', 'pythoness', 'pythonesses', 'pythonic', 'pythons', 'pyuria', 'pyurias', 'pyx', 'pyxes']
Expected output below
Part of the txt file shown on the right:
Update:
I think I just solved it,here are my codes:
def find_all_anagrams( string ):
list1 =
with open("english_words.txt") as f:
word_list =
for line in f.readlines():
word_list.append(line.strip())
for i in word_list:
if anagrams( string, i ):
list1.append(i)
return list1
python data-science data-analysis
2
i.split() creates a list, hence the elements of list1 are list, what exactly are you trying to achieve with i.split()?
– Daniel Mesejo
Nov 12 '18 at 15:02
In the code you've displayed you only call the.lower()
function onstr_1
andstr_2
. What arestring1
andstring2
?
– Adam Mitchell
Nov 12 '18 at 15:02
how are you calling the function?
– Tilman B. aka Nerdyyy
Nov 12 '18 at 15:05
Forword_list
to look like this, there must be a single word on each line. Are you sure that is case? if that is the case, why did you callsplit
on every element ofword_list
?
– Ayxan
Nov 12 '18 at 15:43
I've no idea..... maybe my logic is wrong, the output is so wired...
– Cecilia
Nov 12 '18 at 16:55
|
show 1 more comment
function anagrams(s1, s2) is a Boolean valued function, which returns true just in case the string s1 contains the same letters as string s2 but in a different order. The function should be case insensitive --- in other words it should return the same value if any letters in either s1 or s2 are changed from upper to lower case or from lower to upper case. You may assume that the input strings contain only letters.
The function find_all_anagrams(string) takes a string as input and returns a list of all words in the file english_words.txt that are anagrams of the input string.
the function should return a list [word1, ..., wordN] such that each word in this list is a word in the dictionary file such that the value function anagrams(string, word) are True
def anagrams( string1, string2 ):
str_1 = string1.lower()
str_2 = string2.lower()
if str_1 == str_2:
return False
else:
list_1 = list( str_1 )
list_1.sort()
list_2 = list( str_2 )
list_2.sort()
return list_1 == list_2
def find_all_anagrams( string ):
with open("english_words.txt") as f:
word_list =
for line in f.readlines():
word_list.append(line.strip())
list1 = [i.split() for i in word_list]
for j in list1:
if anagrams( string, j ) == True:
return list1
else:
return
ERROR kept saying this: AttributeError: 'list' object has no attribute 'lower'
for example,word_list contains:
['pyruvates', 'python', 'pythoness', 'pythonesses', 'pythonic', 'pythons', 'pyuria', 'pyurias', 'pyx', 'pyxes']
Expected output below
Part of the txt file shown on the right:
Update:
I think I just solved it,here are my codes:
def find_all_anagrams( string ):
list1 =
with open("english_words.txt") as f:
word_list =
for line in f.readlines():
word_list.append(line.strip())
for i in word_list:
if anagrams( string, i ):
list1.append(i)
return list1
python data-science data-analysis
function anagrams(s1, s2) is a Boolean valued function, which returns true just in case the string s1 contains the same letters as string s2 but in a different order. The function should be case insensitive --- in other words it should return the same value if any letters in either s1 or s2 are changed from upper to lower case or from lower to upper case. You may assume that the input strings contain only letters.
The function find_all_anagrams(string) takes a string as input and returns a list of all words in the file english_words.txt that are anagrams of the input string.
the function should return a list [word1, ..., wordN] such that each word in this list is a word in the dictionary file such that the value function anagrams(string, word) are True
def anagrams( string1, string2 ):
str_1 = string1.lower()
str_2 = string2.lower()
if str_1 == str_2:
return False
else:
list_1 = list( str_1 )
list_1.sort()
list_2 = list( str_2 )
list_2.sort()
return list_1 == list_2
def find_all_anagrams( string ):
with open("english_words.txt") as f:
word_list =
for line in f.readlines():
word_list.append(line.strip())
list1 = [i.split() for i in word_list]
for j in list1:
if anagrams( string, j ) == True:
return list1
else:
return
ERROR kept saying this: AttributeError: 'list' object has no attribute 'lower'
for example,word_list contains:
['pyruvates', 'python', 'pythoness', 'pythonesses', 'pythonic', 'pythons', 'pyuria', 'pyurias', 'pyx', 'pyxes']
Expected output below
Part of the txt file shown on the right:
Update:
I think I just solved it,here are my codes:
def find_all_anagrams( string ):
list1 =
with open("english_words.txt") as f:
word_list =
for line in f.readlines():
word_list.append(line.strip())
for i in word_list:
if anagrams( string, i ):
list1.append(i)
return list1
python data-science data-analysis
python data-science data-analysis
edited Nov 12 '18 at 21:42
Cecilia
asked Nov 12 '18 at 14:59
CeciliaCecilia
587
587
2
i.split() creates a list, hence the elements of list1 are list, what exactly are you trying to achieve with i.split()?
– Daniel Mesejo
Nov 12 '18 at 15:02
In the code you've displayed you only call the.lower()
function onstr_1
andstr_2
. What arestring1
andstring2
?
– Adam Mitchell
Nov 12 '18 at 15:02
how are you calling the function?
– Tilman B. aka Nerdyyy
Nov 12 '18 at 15:05
Forword_list
to look like this, there must be a single word on each line. Are you sure that is case? if that is the case, why did you callsplit
on every element ofword_list
?
– Ayxan
Nov 12 '18 at 15:43
I've no idea..... maybe my logic is wrong, the output is so wired...
– Cecilia
Nov 12 '18 at 16:55
|
show 1 more comment
2
i.split() creates a list, hence the elements of list1 are list, what exactly are you trying to achieve with i.split()?
– Daniel Mesejo
Nov 12 '18 at 15:02
In the code you've displayed you only call the.lower()
function onstr_1
andstr_2
. What arestring1
andstring2
?
– Adam Mitchell
Nov 12 '18 at 15:02
how are you calling the function?
– Tilman B. aka Nerdyyy
Nov 12 '18 at 15:05
Forword_list
to look like this, there must be a single word on each line. Are you sure that is case? if that is the case, why did you callsplit
on every element ofword_list
?
– Ayxan
Nov 12 '18 at 15:43
I've no idea..... maybe my logic is wrong, the output is so wired...
– Cecilia
Nov 12 '18 at 16:55
2
2
i.split() creates a list, hence the elements of list1 are list, what exactly are you trying to achieve with i.split()?
– Daniel Mesejo
Nov 12 '18 at 15:02
i.split() creates a list, hence the elements of list1 are list, what exactly are you trying to achieve with i.split()?
– Daniel Mesejo
Nov 12 '18 at 15:02
In the code you've displayed you only call the
.lower()
function on str_1
and str_2
. What are string1
and string2
?– Adam Mitchell
Nov 12 '18 at 15:02
In the code you've displayed you only call the
.lower()
function on str_1
and str_2
. What are string1
and string2
?– Adam Mitchell
Nov 12 '18 at 15:02
how are you calling the function?
– Tilman B. aka Nerdyyy
Nov 12 '18 at 15:05
how are you calling the function?
– Tilman B. aka Nerdyyy
Nov 12 '18 at 15:05
For
word_list
to look like this, there must be a single word on each line. Are you sure that is case? if that is the case, why did you call split
on every element of word_list
?– Ayxan
Nov 12 '18 at 15:43
For
word_list
to look like this, there must be a single word on each line. Are you sure that is case? if that is the case, why did you call split
on every element of word_list
?– Ayxan
Nov 12 '18 at 15:43
I've no idea..... maybe my logic is wrong, the output is so wired...
– Cecilia
Nov 12 '18 at 16:55
I've no idea..... maybe my logic is wrong, the output is so wired...
– Cecilia
Nov 12 '18 at 16:55
|
show 1 more comment
2 Answers
2
active
oldest
votes
You are using split() function at this part:
list1 = [i.split() for i in word_list]
Let's see what the documentation tells us about that function:
str.split(sep=None, maxsplit=-1)
Return a list of the words in the
string, using sep as the delimiter string. If maxsplit is given, at
most maxsplit splits are done (thus, the list will have at most
maxsplit+1 elements). If maxsplit is not specified or -1, then there
is no limit on the number of splits (all possible splits are made).
It returns a list, and you added that list to your own list. I can see that word_list
is meant to hold lines of words. Let's assume that word_list
looks like this:
word_list = ["hello darkness my", "old friend I've", "come to see you", "again"]
What happens after list1 = [i.split() for i in word_list]
?
list1 = [i.split() for i in word_list]
print(list1)
Output:
[['hello', 'darkness', 'my'], ['old', 'friend', "I've"], ['come', 'to', 'see', 'you'], ['again']]
As you can see, elements are individual lists. At this part of your code:
for j in list1:
if anagrams( string, j ) == True:
return list1
else:
return
j
is a list, therefore here:
def anagrams( string1, string2 ):
str_1 = string1.lower()
str_2 = string2.lower()
str_2 = string2.lower()
is trying to call lower method on a list, which isn't a valid method for a list
object, and that's why Python is complaining.
List Comprehension might look "cool" but often using simple loops benefits your code's readability, and in some cases might even avoid mistakes like this one. Here is my alternative:
list1 =
for i in word_list:
for word in i.split():
list1.append(word)
see the output:
print(list1)
['hello', 'darkness', 'my', 'old', 'friend', "I've", 'come', 'to', 'see', 'you', 'again']
Single words as you intended.
@Cecilia Int that case the resulting list is[['pyruvates'], ['python'], ['pythoness'], ['pythonesses'], ['pythonic'], ['pythons'], ['pyuria'], ['pyurias'], ['pyx'], ['pyxes']]
. Elements are still individual lists and each has a single element of its own
– Ayxan
Nov 12 '18 at 16:59
Thanks, but the output is not what I expected.
– Cecilia
Nov 12 '18 at 17:04
@Cecilia Output of my code? How did you use it? What was your expected output?
– Ayxan
Nov 12 '18 at 17:05
I added a picture above(expected output), not your codes, it's mine output, three empty [ ]
– Cecilia
Nov 12 '18 at 17:07
@Cecilia can you add the whole program? At least enough of the code that I can run it on my own and see what's going on. You are not even calling those functions in the question. And also please addenglish_words.txt
– Ayxan
Nov 12 '18 at 17:14
|
show 1 more comment
As indicated by the error message, list does not have an attribute .lower
I guess what you meant do to is access a string within the list with a .lower
attribute.
For example:
mylist[index].lower()
where index corresponds to the string position within the list.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53264809%2flist-object-has-no-attribute-lower-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You are using split() function at this part:
list1 = [i.split() for i in word_list]
Let's see what the documentation tells us about that function:
str.split(sep=None, maxsplit=-1)
Return a list of the words in the
string, using sep as the delimiter string. If maxsplit is given, at
most maxsplit splits are done (thus, the list will have at most
maxsplit+1 elements). If maxsplit is not specified or -1, then there
is no limit on the number of splits (all possible splits are made).
It returns a list, and you added that list to your own list. I can see that word_list
is meant to hold lines of words. Let's assume that word_list
looks like this:
word_list = ["hello darkness my", "old friend I've", "come to see you", "again"]
What happens after list1 = [i.split() for i in word_list]
?
list1 = [i.split() for i in word_list]
print(list1)
Output:
[['hello', 'darkness', 'my'], ['old', 'friend', "I've"], ['come', 'to', 'see', 'you'], ['again']]
As you can see, elements are individual lists. At this part of your code:
for j in list1:
if anagrams( string, j ) == True:
return list1
else:
return
j
is a list, therefore here:
def anagrams( string1, string2 ):
str_1 = string1.lower()
str_2 = string2.lower()
str_2 = string2.lower()
is trying to call lower method on a list, which isn't a valid method for a list
object, and that's why Python is complaining.
List Comprehension might look "cool" but often using simple loops benefits your code's readability, and in some cases might even avoid mistakes like this one. Here is my alternative:
list1 =
for i in word_list:
for word in i.split():
list1.append(word)
see the output:
print(list1)
['hello', 'darkness', 'my', 'old', 'friend', "I've", 'come', 'to', 'see', 'you', 'again']
Single words as you intended.
@Cecilia Int that case the resulting list is[['pyruvates'], ['python'], ['pythoness'], ['pythonesses'], ['pythonic'], ['pythons'], ['pyuria'], ['pyurias'], ['pyx'], ['pyxes']]
. Elements are still individual lists and each has a single element of its own
– Ayxan
Nov 12 '18 at 16:59
Thanks, but the output is not what I expected.
– Cecilia
Nov 12 '18 at 17:04
@Cecilia Output of my code? How did you use it? What was your expected output?
– Ayxan
Nov 12 '18 at 17:05
I added a picture above(expected output), not your codes, it's mine output, three empty [ ]
– Cecilia
Nov 12 '18 at 17:07
@Cecilia can you add the whole program? At least enough of the code that I can run it on my own and see what's going on. You are not even calling those functions in the question. And also please addenglish_words.txt
– Ayxan
Nov 12 '18 at 17:14
|
show 1 more comment
You are using split() function at this part:
list1 = [i.split() for i in word_list]
Let's see what the documentation tells us about that function:
str.split(sep=None, maxsplit=-1)
Return a list of the words in the
string, using sep as the delimiter string. If maxsplit is given, at
most maxsplit splits are done (thus, the list will have at most
maxsplit+1 elements). If maxsplit is not specified or -1, then there
is no limit on the number of splits (all possible splits are made).
It returns a list, and you added that list to your own list. I can see that word_list
is meant to hold lines of words. Let's assume that word_list
looks like this:
word_list = ["hello darkness my", "old friend I've", "come to see you", "again"]
What happens after list1 = [i.split() for i in word_list]
?
list1 = [i.split() for i in word_list]
print(list1)
Output:
[['hello', 'darkness', 'my'], ['old', 'friend', "I've"], ['come', 'to', 'see', 'you'], ['again']]
As you can see, elements are individual lists. At this part of your code:
for j in list1:
if anagrams( string, j ) == True:
return list1
else:
return
j
is a list, therefore here:
def anagrams( string1, string2 ):
str_1 = string1.lower()
str_2 = string2.lower()
str_2 = string2.lower()
is trying to call lower method on a list, which isn't a valid method for a list
object, and that's why Python is complaining.
List Comprehension might look "cool" but often using simple loops benefits your code's readability, and in some cases might even avoid mistakes like this one. Here is my alternative:
list1 =
for i in word_list:
for word in i.split():
list1.append(word)
see the output:
print(list1)
['hello', 'darkness', 'my', 'old', 'friend', "I've", 'come', 'to', 'see', 'you', 'again']
Single words as you intended.
@Cecilia Int that case the resulting list is[['pyruvates'], ['python'], ['pythoness'], ['pythonesses'], ['pythonic'], ['pythons'], ['pyuria'], ['pyurias'], ['pyx'], ['pyxes']]
. Elements are still individual lists and each has a single element of its own
– Ayxan
Nov 12 '18 at 16:59
Thanks, but the output is not what I expected.
– Cecilia
Nov 12 '18 at 17:04
@Cecilia Output of my code? How did you use it? What was your expected output?
– Ayxan
Nov 12 '18 at 17:05
I added a picture above(expected output), not your codes, it's mine output, three empty [ ]
– Cecilia
Nov 12 '18 at 17:07
@Cecilia can you add the whole program? At least enough of the code that I can run it on my own and see what's going on. You are not even calling those functions in the question. And also please addenglish_words.txt
– Ayxan
Nov 12 '18 at 17:14
|
show 1 more comment
You are using split() function at this part:
list1 = [i.split() for i in word_list]
Let's see what the documentation tells us about that function:
str.split(sep=None, maxsplit=-1)
Return a list of the words in the
string, using sep as the delimiter string. If maxsplit is given, at
most maxsplit splits are done (thus, the list will have at most
maxsplit+1 elements). If maxsplit is not specified or -1, then there
is no limit on the number of splits (all possible splits are made).
It returns a list, and you added that list to your own list. I can see that word_list
is meant to hold lines of words. Let's assume that word_list
looks like this:
word_list = ["hello darkness my", "old friend I've", "come to see you", "again"]
What happens after list1 = [i.split() for i in word_list]
?
list1 = [i.split() for i in word_list]
print(list1)
Output:
[['hello', 'darkness', 'my'], ['old', 'friend', "I've"], ['come', 'to', 'see', 'you'], ['again']]
As you can see, elements are individual lists. At this part of your code:
for j in list1:
if anagrams( string, j ) == True:
return list1
else:
return
j
is a list, therefore here:
def anagrams( string1, string2 ):
str_1 = string1.lower()
str_2 = string2.lower()
str_2 = string2.lower()
is trying to call lower method on a list, which isn't a valid method for a list
object, and that's why Python is complaining.
List Comprehension might look "cool" but often using simple loops benefits your code's readability, and in some cases might even avoid mistakes like this one. Here is my alternative:
list1 =
for i in word_list:
for word in i.split():
list1.append(word)
see the output:
print(list1)
['hello', 'darkness', 'my', 'old', 'friend', "I've", 'come', 'to', 'see', 'you', 'again']
Single words as you intended.
You are using split() function at this part:
list1 = [i.split() for i in word_list]
Let's see what the documentation tells us about that function:
str.split(sep=None, maxsplit=-1)
Return a list of the words in the
string, using sep as the delimiter string. If maxsplit is given, at
most maxsplit splits are done (thus, the list will have at most
maxsplit+1 elements). If maxsplit is not specified or -1, then there
is no limit on the number of splits (all possible splits are made).
It returns a list, and you added that list to your own list. I can see that word_list
is meant to hold lines of words. Let's assume that word_list
looks like this:
word_list = ["hello darkness my", "old friend I've", "come to see you", "again"]
What happens after list1 = [i.split() for i in word_list]
?
list1 = [i.split() for i in word_list]
print(list1)
Output:
[['hello', 'darkness', 'my'], ['old', 'friend', "I've"], ['come', 'to', 'see', 'you'], ['again']]
As you can see, elements are individual lists. At this part of your code:
for j in list1:
if anagrams( string, j ) == True:
return list1
else:
return
j
is a list, therefore here:
def anagrams( string1, string2 ):
str_1 = string1.lower()
str_2 = string2.lower()
str_2 = string2.lower()
is trying to call lower method on a list, which isn't a valid method for a list
object, and that's why Python is complaining.
List Comprehension might look "cool" but often using simple loops benefits your code's readability, and in some cases might even avoid mistakes like this one. Here is my alternative:
list1 =
for i in word_list:
for word in i.split():
list1.append(word)
see the output:
print(list1)
['hello', 'darkness', 'my', 'old', 'friend', "I've", 'come', 'to', 'see', 'you', 'again']
Single words as you intended.
edited Nov 12 '18 at 15:37
answered Nov 12 '18 at 15:30


AyxanAyxan
1,573115
1,573115
@Cecilia Int that case the resulting list is[['pyruvates'], ['python'], ['pythoness'], ['pythonesses'], ['pythonic'], ['pythons'], ['pyuria'], ['pyurias'], ['pyx'], ['pyxes']]
. Elements are still individual lists and each has a single element of its own
– Ayxan
Nov 12 '18 at 16:59
Thanks, but the output is not what I expected.
– Cecilia
Nov 12 '18 at 17:04
@Cecilia Output of my code? How did you use it? What was your expected output?
– Ayxan
Nov 12 '18 at 17:05
I added a picture above(expected output), not your codes, it's mine output, three empty [ ]
– Cecilia
Nov 12 '18 at 17:07
@Cecilia can you add the whole program? At least enough of the code that I can run it on my own and see what's going on. You are not even calling those functions in the question. And also please addenglish_words.txt
– Ayxan
Nov 12 '18 at 17:14
|
show 1 more comment
@Cecilia Int that case the resulting list is[['pyruvates'], ['python'], ['pythoness'], ['pythonesses'], ['pythonic'], ['pythons'], ['pyuria'], ['pyurias'], ['pyx'], ['pyxes']]
. Elements are still individual lists and each has a single element of its own
– Ayxan
Nov 12 '18 at 16:59
Thanks, but the output is not what I expected.
– Cecilia
Nov 12 '18 at 17:04
@Cecilia Output of my code? How did you use it? What was your expected output?
– Ayxan
Nov 12 '18 at 17:05
I added a picture above(expected output), not your codes, it's mine output, three empty [ ]
– Cecilia
Nov 12 '18 at 17:07
@Cecilia can you add the whole program? At least enough of the code that I can run it on my own and see what's going on. You are not even calling those functions in the question. And also please addenglish_words.txt
– Ayxan
Nov 12 '18 at 17:14
@Cecilia Int that case the resulting list is
[['pyruvates'], ['python'], ['pythoness'], ['pythonesses'], ['pythonic'], ['pythons'], ['pyuria'], ['pyurias'], ['pyx'], ['pyxes']]
. Elements are still individual lists and each has a single element of its own– Ayxan
Nov 12 '18 at 16:59
@Cecilia Int that case the resulting list is
[['pyruvates'], ['python'], ['pythoness'], ['pythonesses'], ['pythonic'], ['pythons'], ['pyuria'], ['pyurias'], ['pyx'], ['pyxes']]
. Elements are still individual lists and each has a single element of its own– Ayxan
Nov 12 '18 at 16:59
Thanks, but the output is not what I expected.
– Cecilia
Nov 12 '18 at 17:04
Thanks, but the output is not what I expected.
– Cecilia
Nov 12 '18 at 17:04
@Cecilia Output of my code? How did you use it? What was your expected output?
– Ayxan
Nov 12 '18 at 17:05
@Cecilia Output of my code? How did you use it? What was your expected output?
– Ayxan
Nov 12 '18 at 17:05
I added a picture above(expected output), not your codes, it's mine output, three empty [ ]
– Cecilia
Nov 12 '18 at 17:07
I added a picture above(expected output), not your codes, it's mine output, three empty [ ]
– Cecilia
Nov 12 '18 at 17:07
@Cecilia can you add the whole program? At least enough of the code that I can run it on my own and see what's going on. You are not even calling those functions in the question. And also please add
english_words.txt
– Ayxan
Nov 12 '18 at 17:14
@Cecilia can you add the whole program? At least enough of the code that I can run it on my own and see what's going on. You are not even calling those functions in the question. And also please add
english_words.txt
– Ayxan
Nov 12 '18 at 17:14
|
show 1 more comment
As indicated by the error message, list does not have an attribute .lower
I guess what you meant do to is access a string within the list with a .lower
attribute.
For example:
mylist[index].lower()
where index corresponds to the string position within the list.
add a comment |
As indicated by the error message, list does not have an attribute .lower
I guess what you meant do to is access a string within the list with a .lower
attribute.
For example:
mylist[index].lower()
where index corresponds to the string position within the list.
add a comment |
As indicated by the error message, list does not have an attribute .lower
I guess what you meant do to is access a string within the list with a .lower
attribute.
For example:
mylist[index].lower()
where index corresponds to the string position within the list.
As indicated by the error message, list does not have an attribute .lower
I guess what you meant do to is access a string within the list with a .lower
attribute.
For example:
mylist[index].lower()
where index corresponds to the string position within the list.
answered Nov 12 '18 at 15:02


dheinzdheinz
1277
1277
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53264809%2flist-object-has-no-attribute-lower-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5ZWlfl,NJRUBc l0,fbwK3rWc6Dq,s1PFE4mu28jXYZDsm5,Lk4pT7,V
2
i.split() creates a list, hence the elements of list1 are list, what exactly are you trying to achieve with i.split()?
– Daniel Mesejo
Nov 12 '18 at 15:02
In the code you've displayed you only call the
.lower()
function onstr_1
andstr_2
. What arestring1
andstring2
?– Adam Mitchell
Nov 12 '18 at 15:02
how are you calling the function?
– Tilman B. aka Nerdyyy
Nov 12 '18 at 15:05
For
word_list
to look like this, there must be a single word on each line. Are you sure that is case? if that is the case, why did you callsplit
on every element ofword_list
?– Ayxan
Nov 12 '18 at 15:43
I've no idea..... maybe my logic is wrong, the output is so wired...
– Cecilia
Nov 12 '18 at 16:55