Close socket connection in request.js
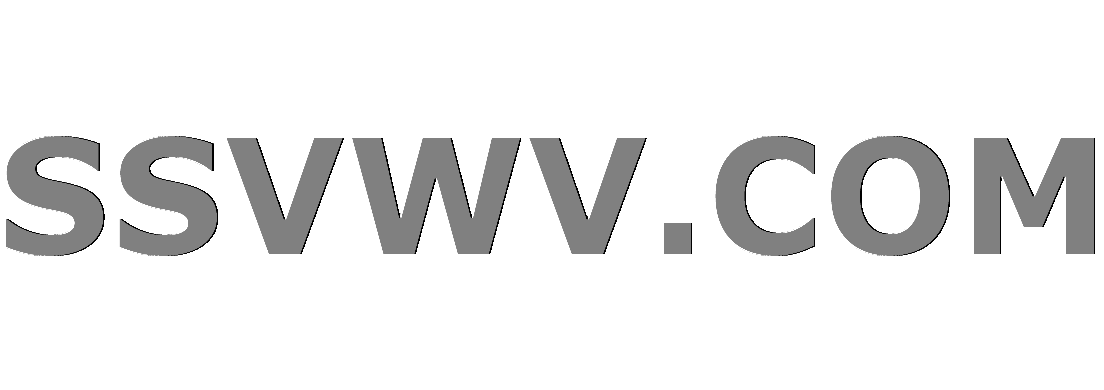
Multi tool use
I have a program that uses 'request' module to fetch html.
Is there a way to manually close socket connection after receiving a response other than abort as well as remove all listeners from that particular request instance.
The main problem is that, as the program continues making requests, the number of file descriptors and listeners, keeps growing, regardless of using abort() after the fetch is done.
Finally, the program ends after it reaches a maxListeners size, which is already set for 20000.
const request=require('request')
fetchUrl (params, tag, callBack) {
this.url = params.url
this.tag = tag
let headers = { 'User-Agent': params.useragent }
let options = { followRedirects: false, keepAlive: false, url: params.url, headers: headers, gzip: true, encoding: null }
let proxy = 'None'
let userAgent = params.useragent
if (parseInt(ConfigHandler.getConfig().spfUseProxy) === 1) {
if (parseInt(ConfigHandler.getConfig().spfUseHermesUserAgent) === 1) {
userAgent = params.useragent + ' Hughes-PFB/' +
params.agentid + '/' + params.tracetags
headers['User-Agent'] = userAgent
headers['Connection'] = 'Close'
}
proxy = 'http://' + ConfigHandler.getConfig().iosPrefetchProxyIp +
':' + ConfigHandler.getConfig().iosPrefetchProxyPort
options['proxy'] = proxy
}
this.httpClient = request.get(options, (err, response, body) => {
let result = {}
if (!err) {
this.response = response
this.bodyLen = body.length
logger.debug('%s Response info, %s', this.tag, this.resourceInfo())
result = {
statuscode: response.statusCode,
headers: response.headers,
body: body
}
} else {
logger.error('%s, fetchUrl() failed! cause=%s, url=%s', JSON.stringify(this), err.message,
this.url)
}
if (this.httpClient) {
logger.debug('%s, fetchUrl() ending connection!', JSON.stringify(this.httpClient))
this.httpClient.abort()
}
if (response && response.socket) {
response.socket.destroy()
}
callBack(result, err)
})
}
cancelFetch () {
if (this.httpClient) {
logger.debug('%s, Cancelling fetch for url=%s', this.tag, this.url)
this.httpClient.abort()
this.httpClient = null
this.url = null
}
this.response.socket.removeAllListeners()
}
}
close () {
if (this.response && this.response.socket) {
this.response.socket.destroy()
//logger.debug('%s, close(), after socket.destroy(), statusTCP=%s', JSON.stringify(this.response), this.response.socket)
}
if (this.httpClient) {
logger.debug('%s, Closing HTTP connection for url=%s', this.tag, this.url)
this.httpClient.abort()
this.httpClient = null
this.url = null
this.response = null
}
}
javascript node.js sockets tcplistener
add a comment |
I have a program that uses 'request' module to fetch html.
Is there a way to manually close socket connection after receiving a response other than abort as well as remove all listeners from that particular request instance.
The main problem is that, as the program continues making requests, the number of file descriptors and listeners, keeps growing, regardless of using abort() after the fetch is done.
Finally, the program ends after it reaches a maxListeners size, which is already set for 20000.
const request=require('request')
fetchUrl (params, tag, callBack) {
this.url = params.url
this.tag = tag
let headers = { 'User-Agent': params.useragent }
let options = { followRedirects: false, keepAlive: false, url: params.url, headers: headers, gzip: true, encoding: null }
let proxy = 'None'
let userAgent = params.useragent
if (parseInt(ConfigHandler.getConfig().spfUseProxy) === 1) {
if (parseInt(ConfigHandler.getConfig().spfUseHermesUserAgent) === 1) {
userAgent = params.useragent + ' Hughes-PFB/' +
params.agentid + '/' + params.tracetags
headers['User-Agent'] = userAgent
headers['Connection'] = 'Close'
}
proxy = 'http://' + ConfigHandler.getConfig().iosPrefetchProxyIp +
':' + ConfigHandler.getConfig().iosPrefetchProxyPort
options['proxy'] = proxy
}
this.httpClient = request.get(options, (err, response, body) => {
let result = {}
if (!err) {
this.response = response
this.bodyLen = body.length
logger.debug('%s Response info, %s', this.tag, this.resourceInfo())
result = {
statuscode: response.statusCode,
headers: response.headers,
body: body
}
} else {
logger.error('%s, fetchUrl() failed! cause=%s, url=%s', JSON.stringify(this), err.message,
this.url)
}
if (this.httpClient) {
logger.debug('%s, fetchUrl() ending connection!', JSON.stringify(this.httpClient))
this.httpClient.abort()
}
if (response && response.socket) {
response.socket.destroy()
}
callBack(result, err)
})
}
cancelFetch () {
if (this.httpClient) {
logger.debug('%s, Cancelling fetch for url=%s', this.tag, this.url)
this.httpClient.abort()
this.httpClient = null
this.url = null
}
this.response.socket.removeAllListeners()
}
}
close () {
if (this.response && this.response.socket) {
this.response.socket.destroy()
//logger.debug('%s, close(), after socket.destroy(), statusTCP=%s', JSON.stringify(this.response), this.response.socket)
}
if (this.httpClient) {
logger.debug('%s, Closing HTTP connection for url=%s', this.tag, this.url)
this.httpClient.abort()
this.httpClient = null
this.url = null
this.response = null
}
}
javascript node.js sockets tcplistener
share you code to better understand the problem
– front_end_dev
Nov 12 '18 at 15:09
I just added it, sorry.
– Igor Kamalov
Nov 12 '18 at 15:11
which module you are using forrequest
– front_end_dev
Nov 12 '18 at 15:21
Im using 'request.js'
– Igor Kamalov
Nov 12 '18 at 16:42
add a comment |
I have a program that uses 'request' module to fetch html.
Is there a way to manually close socket connection after receiving a response other than abort as well as remove all listeners from that particular request instance.
The main problem is that, as the program continues making requests, the number of file descriptors and listeners, keeps growing, regardless of using abort() after the fetch is done.
Finally, the program ends after it reaches a maxListeners size, which is already set for 20000.
const request=require('request')
fetchUrl (params, tag, callBack) {
this.url = params.url
this.tag = tag
let headers = { 'User-Agent': params.useragent }
let options = { followRedirects: false, keepAlive: false, url: params.url, headers: headers, gzip: true, encoding: null }
let proxy = 'None'
let userAgent = params.useragent
if (parseInt(ConfigHandler.getConfig().spfUseProxy) === 1) {
if (parseInt(ConfigHandler.getConfig().spfUseHermesUserAgent) === 1) {
userAgent = params.useragent + ' Hughes-PFB/' +
params.agentid + '/' + params.tracetags
headers['User-Agent'] = userAgent
headers['Connection'] = 'Close'
}
proxy = 'http://' + ConfigHandler.getConfig().iosPrefetchProxyIp +
':' + ConfigHandler.getConfig().iosPrefetchProxyPort
options['proxy'] = proxy
}
this.httpClient = request.get(options, (err, response, body) => {
let result = {}
if (!err) {
this.response = response
this.bodyLen = body.length
logger.debug('%s Response info, %s', this.tag, this.resourceInfo())
result = {
statuscode: response.statusCode,
headers: response.headers,
body: body
}
} else {
logger.error('%s, fetchUrl() failed! cause=%s, url=%s', JSON.stringify(this), err.message,
this.url)
}
if (this.httpClient) {
logger.debug('%s, fetchUrl() ending connection!', JSON.stringify(this.httpClient))
this.httpClient.abort()
}
if (response && response.socket) {
response.socket.destroy()
}
callBack(result, err)
})
}
cancelFetch () {
if (this.httpClient) {
logger.debug('%s, Cancelling fetch for url=%s', this.tag, this.url)
this.httpClient.abort()
this.httpClient = null
this.url = null
}
this.response.socket.removeAllListeners()
}
}
close () {
if (this.response && this.response.socket) {
this.response.socket.destroy()
//logger.debug('%s, close(), after socket.destroy(), statusTCP=%s', JSON.stringify(this.response), this.response.socket)
}
if (this.httpClient) {
logger.debug('%s, Closing HTTP connection for url=%s', this.tag, this.url)
this.httpClient.abort()
this.httpClient = null
this.url = null
this.response = null
}
}
javascript node.js sockets tcplistener
I have a program that uses 'request' module to fetch html.
Is there a way to manually close socket connection after receiving a response other than abort as well as remove all listeners from that particular request instance.
The main problem is that, as the program continues making requests, the number of file descriptors and listeners, keeps growing, regardless of using abort() after the fetch is done.
Finally, the program ends after it reaches a maxListeners size, which is already set for 20000.
const request=require('request')
fetchUrl (params, tag, callBack) {
this.url = params.url
this.tag = tag
let headers = { 'User-Agent': params.useragent }
let options = { followRedirects: false, keepAlive: false, url: params.url, headers: headers, gzip: true, encoding: null }
let proxy = 'None'
let userAgent = params.useragent
if (parseInt(ConfigHandler.getConfig().spfUseProxy) === 1) {
if (parseInt(ConfigHandler.getConfig().spfUseHermesUserAgent) === 1) {
userAgent = params.useragent + ' Hughes-PFB/' +
params.agentid + '/' + params.tracetags
headers['User-Agent'] = userAgent
headers['Connection'] = 'Close'
}
proxy = 'http://' + ConfigHandler.getConfig().iosPrefetchProxyIp +
':' + ConfigHandler.getConfig().iosPrefetchProxyPort
options['proxy'] = proxy
}
this.httpClient = request.get(options, (err, response, body) => {
let result = {}
if (!err) {
this.response = response
this.bodyLen = body.length
logger.debug('%s Response info, %s', this.tag, this.resourceInfo())
result = {
statuscode: response.statusCode,
headers: response.headers,
body: body
}
} else {
logger.error('%s, fetchUrl() failed! cause=%s, url=%s', JSON.stringify(this), err.message,
this.url)
}
if (this.httpClient) {
logger.debug('%s, fetchUrl() ending connection!', JSON.stringify(this.httpClient))
this.httpClient.abort()
}
if (response && response.socket) {
response.socket.destroy()
}
callBack(result, err)
})
}
cancelFetch () {
if (this.httpClient) {
logger.debug('%s, Cancelling fetch for url=%s', this.tag, this.url)
this.httpClient.abort()
this.httpClient = null
this.url = null
}
this.response.socket.removeAllListeners()
}
}
close () {
if (this.response && this.response.socket) {
this.response.socket.destroy()
//logger.debug('%s, close(), after socket.destroy(), statusTCP=%s', JSON.stringify(this.response), this.response.socket)
}
if (this.httpClient) {
logger.debug('%s, Closing HTTP connection for url=%s', this.tag, this.url)
this.httpClient.abort()
this.httpClient = null
this.url = null
this.response = null
}
}
const request=require('request')
fetchUrl (params, tag, callBack) {
this.url = params.url
this.tag = tag
let headers = { 'User-Agent': params.useragent }
let options = { followRedirects: false, keepAlive: false, url: params.url, headers: headers, gzip: true, encoding: null }
let proxy = 'None'
let userAgent = params.useragent
if (parseInt(ConfigHandler.getConfig().spfUseProxy) === 1) {
if (parseInt(ConfigHandler.getConfig().spfUseHermesUserAgent) === 1) {
userAgent = params.useragent + ' Hughes-PFB/' +
params.agentid + '/' + params.tracetags
headers['User-Agent'] = userAgent
headers['Connection'] = 'Close'
}
proxy = 'http://' + ConfigHandler.getConfig().iosPrefetchProxyIp +
':' + ConfigHandler.getConfig().iosPrefetchProxyPort
options['proxy'] = proxy
}
this.httpClient = request.get(options, (err, response, body) => {
let result = {}
if (!err) {
this.response = response
this.bodyLen = body.length
logger.debug('%s Response info, %s', this.tag, this.resourceInfo())
result = {
statuscode: response.statusCode,
headers: response.headers,
body: body
}
} else {
logger.error('%s, fetchUrl() failed! cause=%s, url=%s', JSON.stringify(this), err.message,
this.url)
}
if (this.httpClient) {
logger.debug('%s, fetchUrl() ending connection!', JSON.stringify(this.httpClient))
this.httpClient.abort()
}
if (response && response.socket) {
response.socket.destroy()
}
callBack(result, err)
})
}
cancelFetch () {
if (this.httpClient) {
logger.debug('%s, Cancelling fetch for url=%s', this.tag, this.url)
this.httpClient.abort()
this.httpClient = null
this.url = null
}
this.response.socket.removeAllListeners()
}
}
close () {
if (this.response && this.response.socket) {
this.response.socket.destroy()
//logger.debug('%s, close(), after socket.destroy(), statusTCP=%s', JSON.stringify(this.response), this.response.socket)
}
if (this.httpClient) {
logger.debug('%s, Closing HTTP connection for url=%s', this.tag, this.url)
this.httpClient.abort()
this.httpClient = null
this.url = null
this.response = null
}
}
const request=require('request')
fetchUrl (params, tag, callBack) {
this.url = params.url
this.tag = tag
let headers = { 'User-Agent': params.useragent }
let options = { followRedirects: false, keepAlive: false, url: params.url, headers: headers, gzip: true, encoding: null }
let proxy = 'None'
let userAgent = params.useragent
if (parseInt(ConfigHandler.getConfig().spfUseProxy) === 1) {
if (parseInt(ConfigHandler.getConfig().spfUseHermesUserAgent) === 1) {
userAgent = params.useragent + ' Hughes-PFB/' +
params.agentid + '/' + params.tracetags
headers['User-Agent'] = userAgent
headers['Connection'] = 'Close'
}
proxy = 'http://' + ConfigHandler.getConfig().iosPrefetchProxyIp +
':' + ConfigHandler.getConfig().iosPrefetchProxyPort
options['proxy'] = proxy
}
this.httpClient = request.get(options, (err, response, body) => {
let result = {}
if (!err) {
this.response = response
this.bodyLen = body.length
logger.debug('%s Response info, %s', this.tag, this.resourceInfo())
result = {
statuscode: response.statusCode,
headers: response.headers,
body: body
}
} else {
logger.error('%s, fetchUrl() failed! cause=%s, url=%s', JSON.stringify(this), err.message,
this.url)
}
if (this.httpClient) {
logger.debug('%s, fetchUrl() ending connection!', JSON.stringify(this.httpClient))
this.httpClient.abort()
}
if (response && response.socket) {
response.socket.destroy()
}
callBack(result, err)
})
}
cancelFetch () {
if (this.httpClient) {
logger.debug('%s, Cancelling fetch for url=%s', this.tag, this.url)
this.httpClient.abort()
this.httpClient = null
this.url = null
}
this.response.socket.removeAllListeners()
}
}
close () {
if (this.response && this.response.socket) {
this.response.socket.destroy()
//logger.debug('%s, close(), after socket.destroy(), statusTCP=%s', JSON.stringify(this.response), this.response.socket)
}
if (this.httpClient) {
logger.debug('%s, Closing HTTP connection for url=%s', this.tag, this.url)
this.httpClient.abort()
this.httpClient = null
this.url = null
this.response = null
}
}
javascript node.js sockets tcplistener
javascript node.js sockets tcplistener
edited Nov 12 '18 at 16:42
Igor Kamalov
asked Nov 12 '18 at 15:02
Igor KamalovIgor Kamalov
428
428
share you code to better understand the problem
– front_end_dev
Nov 12 '18 at 15:09
I just added it, sorry.
– Igor Kamalov
Nov 12 '18 at 15:11
which module you are using forrequest
– front_end_dev
Nov 12 '18 at 15:21
Im using 'request.js'
– Igor Kamalov
Nov 12 '18 at 16:42
add a comment |
share you code to better understand the problem
– front_end_dev
Nov 12 '18 at 15:09
I just added it, sorry.
– Igor Kamalov
Nov 12 '18 at 15:11
which module you are using forrequest
– front_end_dev
Nov 12 '18 at 15:21
Im using 'request.js'
– Igor Kamalov
Nov 12 '18 at 16:42
share you code to better understand the problem
– front_end_dev
Nov 12 '18 at 15:09
share you code to better understand the problem
– front_end_dev
Nov 12 '18 at 15:09
I just added it, sorry.
– Igor Kamalov
Nov 12 '18 at 15:11
I just added it, sorry.
– Igor Kamalov
Nov 12 '18 at 15:11
which module you are using for
request
– front_end_dev
Nov 12 '18 at 15:21
which module you are using for
request
– front_end_dev
Nov 12 '18 at 15:21
Im using 'request.js'
– Igor Kamalov
Nov 12 '18 at 16:42
Im using 'request.js'
– Igor Kamalov
Nov 12 '18 at 16:42
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53264876%2fclose-socket-connection-in-request-js%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53264876%2fclose-socket-connection-in-request-js%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5ir7,Cbl6nNuJ
share you code to better understand the problem
– front_end_dev
Nov 12 '18 at 15:09
I just added it, sorry.
– Igor Kamalov
Nov 12 '18 at 15:11
which module you are using for
request
– front_end_dev
Nov 12 '18 at 15:21
Im using 'request.js'
– Igor Kamalov
Nov 12 '18 at 16:42