widget_tweaks render_field filtering the return of a dropbox
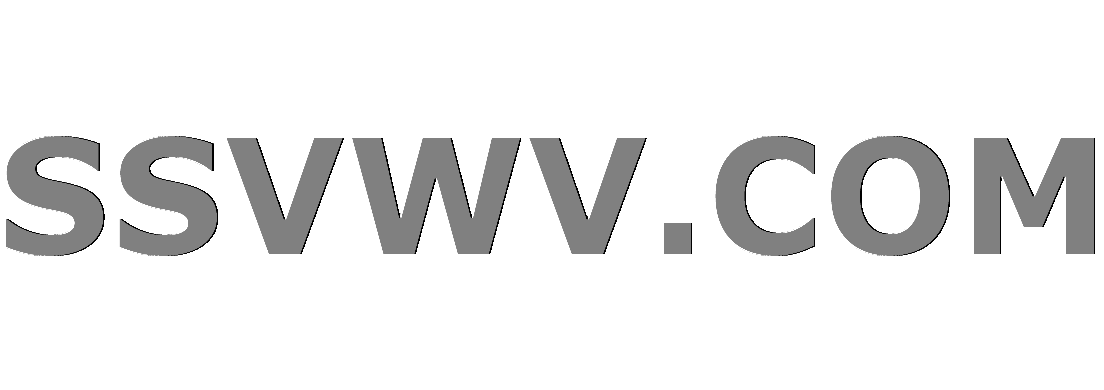
Multi tool use
I am using this template
{% extends "website/_layouts/base.html" %}
{% load widget_tweaks %}
{% block title %}Matricula de Membros{% endblock %}
{% block conteudo %}
<div class="container mt-5">
<div class="row">
<div class="col-lg-12 col-md-12 col-sm-12 col-xs-12">
<div class="card">
<div class="card-body">
<h5 class="card-title">Matrícula de Membros</h5>
<p class="card-text">
Complete o formulário abaixo para matricular
um <code>Membro</code> em um evento.
</p>
<form method="post">
<!-- Não se esqueça dessa tag -->
{% csrf_token %}
<!-- Estudante -->
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text">Estudante</span>
</div>
{% render_field form.estudante class+="form-control" hidden=true %}
<span class="input-group-text">{{ estudante.nome }}</span>
</div>
<hr>
<!-- Curso -->
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text">Evento</span>
</div>
{% render_field form.cursoPeriodo class+="form-control" %}
</div>
<hr>
<div class="text-right">
<a href="{% url 'website:lista_estudantes' %}" class="btn btn-outline-primary">Voltar</a>
<button class="btn btn-primary">Enviar</button>
</div>
</form>
</div>
</div>
</div>
</div>
{% endblock %}
with this view:
# MATRICULA DE MEMBROS
# ----------------------------------------------
class MatriculaCreateView(CreateView):
template_name = "website/matricula.html"
model = CursoPeriodoEstudante
form_class = MatriculaMembroForm
success_url = reverse_lazy("website:lista_estudantes")
def get_initial(self):
return {'estudante': Estudante.objetos.get(id=self.kwargs['pk'])}
def get_context_data(self, **kwargs):
context = super(MatriculaCreateView, self).get_context_data(**kwargs)
context['estudante'] = Estudante.objetos.get(id=self.kwargs['pk'])
context['pk'] = self.kwargs['pk']
return context
and this form:
class MatriculaMembroForm(forms.ModelForm):
class Meta:
# Modelo base
model = CursoPeriodoEstudante
# Campos que estarão no form
fields = [
'estudante',
'cursoPeriodo'
]
And this model:
class CursoPeriodoEstudante(models.Model):
aprovacao = models.BooleanField(
null=False,
blank=False,
default=False
)
presencas = models.BooleanField(
null=False,
blank=False,
default=False
)
financeiro = models.BooleanField(
null=False,
blank=False,
default=False
)
provas = models.BooleanField(
null=False,
blank=False,
default=False
)
trabalhos = models.BooleanField(
null=False,
blank=False,
default=False
)
pagamento = models.IntegerField(
null=False,
blank=False,
default=0
)
cursoPeriodo = models.ForeignKey(CursoPeriodo, on_delete=models.CASCADE)
estudante = models.ForeignKey(Estudante, on_delete=models.CASCADE)
objetos = models.Manager()
To show this page:enter image description here.
As you can see, the field "modelo" comes with all the lines that I wrote in the table. But I only want to show the fields with "matriculasAbertas"=1, as you can see in my table: enter image description here
What can I do to filter it?
django-widget-tweaks
add a comment |
I am using this template
{% extends "website/_layouts/base.html" %}
{% load widget_tweaks %}
{% block title %}Matricula de Membros{% endblock %}
{% block conteudo %}
<div class="container mt-5">
<div class="row">
<div class="col-lg-12 col-md-12 col-sm-12 col-xs-12">
<div class="card">
<div class="card-body">
<h5 class="card-title">Matrícula de Membros</h5>
<p class="card-text">
Complete o formulário abaixo para matricular
um <code>Membro</code> em um evento.
</p>
<form method="post">
<!-- Não se esqueça dessa tag -->
{% csrf_token %}
<!-- Estudante -->
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text">Estudante</span>
</div>
{% render_field form.estudante class+="form-control" hidden=true %}
<span class="input-group-text">{{ estudante.nome }}</span>
</div>
<hr>
<!-- Curso -->
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text">Evento</span>
</div>
{% render_field form.cursoPeriodo class+="form-control" %}
</div>
<hr>
<div class="text-right">
<a href="{% url 'website:lista_estudantes' %}" class="btn btn-outline-primary">Voltar</a>
<button class="btn btn-primary">Enviar</button>
</div>
</form>
</div>
</div>
</div>
</div>
{% endblock %}
with this view:
# MATRICULA DE MEMBROS
# ----------------------------------------------
class MatriculaCreateView(CreateView):
template_name = "website/matricula.html"
model = CursoPeriodoEstudante
form_class = MatriculaMembroForm
success_url = reverse_lazy("website:lista_estudantes")
def get_initial(self):
return {'estudante': Estudante.objetos.get(id=self.kwargs['pk'])}
def get_context_data(self, **kwargs):
context = super(MatriculaCreateView, self).get_context_data(**kwargs)
context['estudante'] = Estudante.objetos.get(id=self.kwargs['pk'])
context['pk'] = self.kwargs['pk']
return context
and this form:
class MatriculaMembroForm(forms.ModelForm):
class Meta:
# Modelo base
model = CursoPeriodoEstudante
# Campos que estarão no form
fields = [
'estudante',
'cursoPeriodo'
]
And this model:
class CursoPeriodoEstudante(models.Model):
aprovacao = models.BooleanField(
null=False,
blank=False,
default=False
)
presencas = models.BooleanField(
null=False,
blank=False,
default=False
)
financeiro = models.BooleanField(
null=False,
blank=False,
default=False
)
provas = models.BooleanField(
null=False,
blank=False,
default=False
)
trabalhos = models.BooleanField(
null=False,
blank=False,
default=False
)
pagamento = models.IntegerField(
null=False,
blank=False,
default=0
)
cursoPeriodo = models.ForeignKey(CursoPeriodo, on_delete=models.CASCADE)
estudante = models.ForeignKey(Estudante, on_delete=models.CASCADE)
objetos = models.Manager()
To show this page:enter image description here.
As you can see, the field "modelo" comes with all the lines that I wrote in the table. But I only want to show the fields with "matriculasAbertas"=1, as you can see in my table: enter image description here
What can I do to filter it?
django-widget-tweaks
add a comment |
I am using this template
{% extends "website/_layouts/base.html" %}
{% load widget_tweaks %}
{% block title %}Matricula de Membros{% endblock %}
{% block conteudo %}
<div class="container mt-5">
<div class="row">
<div class="col-lg-12 col-md-12 col-sm-12 col-xs-12">
<div class="card">
<div class="card-body">
<h5 class="card-title">Matrícula de Membros</h5>
<p class="card-text">
Complete o formulário abaixo para matricular
um <code>Membro</code> em um evento.
</p>
<form method="post">
<!-- Não se esqueça dessa tag -->
{% csrf_token %}
<!-- Estudante -->
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text">Estudante</span>
</div>
{% render_field form.estudante class+="form-control" hidden=true %}
<span class="input-group-text">{{ estudante.nome }}</span>
</div>
<hr>
<!-- Curso -->
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text">Evento</span>
</div>
{% render_field form.cursoPeriodo class+="form-control" %}
</div>
<hr>
<div class="text-right">
<a href="{% url 'website:lista_estudantes' %}" class="btn btn-outline-primary">Voltar</a>
<button class="btn btn-primary">Enviar</button>
</div>
</form>
</div>
</div>
</div>
</div>
{% endblock %}
with this view:
# MATRICULA DE MEMBROS
# ----------------------------------------------
class MatriculaCreateView(CreateView):
template_name = "website/matricula.html"
model = CursoPeriodoEstudante
form_class = MatriculaMembroForm
success_url = reverse_lazy("website:lista_estudantes")
def get_initial(self):
return {'estudante': Estudante.objetos.get(id=self.kwargs['pk'])}
def get_context_data(self, **kwargs):
context = super(MatriculaCreateView, self).get_context_data(**kwargs)
context['estudante'] = Estudante.objetos.get(id=self.kwargs['pk'])
context['pk'] = self.kwargs['pk']
return context
and this form:
class MatriculaMembroForm(forms.ModelForm):
class Meta:
# Modelo base
model = CursoPeriodoEstudante
# Campos que estarão no form
fields = [
'estudante',
'cursoPeriodo'
]
And this model:
class CursoPeriodoEstudante(models.Model):
aprovacao = models.BooleanField(
null=False,
blank=False,
default=False
)
presencas = models.BooleanField(
null=False,
blank=False,
default=False
)
financeiro = models.BooleanField(
null=False,
blank=False,
default=False
)
provas = models.BooleanField(
null=False,
blank=False,
default=False
)
trabalhos = models.BooleanField(
null=False,
blank=False,
default=False
)
pagamento = models.IntegerField(
null=False,
blank=False,
default=0
)
cursoPeriodo = models.ForeignKey(CursoPeriodo, on_delete=models.CASCADE)
estudante = models.ForeignKey(Estudante, on_delete=models.CASCADE)
objetos = models.Manager()
To show this page:enter image description here.
As you can see, the field "modelo" comes with all the lines that I wrote in the table. But I only want to show the fields with "matriculasAbertas"=1, as you can see in my table: enter image description here
What can I do to filter it?
django-widget-tweaks
I am using this template
{% extends "website/_layouts/base.html" %}
{% load widget_tweaks %}
{% block title %}Matricula de Membros{% endblock %}
{% block conteudo %}
<div class="container mt-5">
<div class="row">
<div class="col-lg-12 col-md-12 col-sm-12 col-xs-12">
<div class="card">
<div class="card-body">
<h5 class="card-title">Matrícula de Membros</h5>
<p class="card-text">
Complete o formulário abaixo para matricular
um <code>Membro</code> em um evento.
</p>
<form method="post">
<!-- Não se esqueça dessa tag -->
{% csrf_token %}
<!-- Estudante -->
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text">Estudante</span>
</div>
{% render_field form.estudante class+="form-control" hidden=true %}
<span class="input-group-text">{{ estudante.nome }}</span>
</div>
<hr>
<!-- Curso -->
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text">Evento</span>
</div>
{% render_field form.cursoPeriodo class+="form-control" %}
</div>
<hr>
<div class="text-right">
<a href="{% url 'website:lista_estudantes' %}" class="btn btn-outline-primary">Voltar</a>
<button class="btn btn-primary">Enviar</button>
</div>
</form>
</div>
</div>
</div>
</div>
{% endblock %}
with this view:
# MATRICULA DE MEMBROS
# ----------------------------------------------
class MatriculaCreateView(CreateView):
template_name = "website/matricula.html"
model = CursoPeriodoEstudante
form_class = MatriculaMembroForm
success_url = reverse_lazy("website:lista_estudantes")
def get_initial(self):
return {'estudante': Estudante.objetos.get(id=self.kwargs['pk'])}
def get_context_data(self, **kwargs):
context = super(MatriculaCreateView, self).get_context_data(**kwargs)
context['estudante'] = Estudante.objetos.get(id=self.kwargs['pk'])
context['pk'] = self.kwargs['pk']
return context
and this form:
class MatriculaMembroForm(forms.ModelForm):
class Meta:
# Modelo base
model = CursoPeriodoEstudante
# Campos que estarão no form
fields = [
'estudante',
'cursoPeriodo'
]
And this model:
class CursoPeriodoEstudante(models.Model):
aprovacao = models.BooleanField(
null=False,
blank=False,
default=False
)
presencas = models.BooleanField(
null=False,
blank=False,
default=False
)
financeiro = models.BooleanField(
null=False,
blank=False,
default=False
)
provas = models.BooleanField(
null=False,
blank=False,
default=False
)
trabalhos = models.BooleanField(
null=False,
blank=False,
default=False
)
pagamento = models.IntegerField(
null=False,
blank=False,
default=0
)
cursoPeriodo = models.ForeignKey(CursoPeriodo, on_delete=models.CASCADE)
estudante = models.ForeignKey(Estudante, on_delete=models.CASCADE)
objetos = models.Manager()
To show this page:enter image description here.
As you can see, the field "modelo" comes with all the lines that I wrote in the table. But I only want to show the fields with "matriculasAbertas"=1, as you can see in my table: enter image description here
What can I do to filter it?
django-widget-tweaks
django-widget-tweaks
asked Nov 12 '18 at 23:06
Alex SteeveAlex Steeve
34
34
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53271395%2fwidget-tweaks-render-field-filtering-the-return-of-a-dropbox%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53271395%2fwidget-tweaks-render-field-filtering-the-return-of-a-dropbox%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
obfUK5mzTxCzm,1wDVbYJ9UGJDoWg,2,HsXdtf,5pIQcNhB6dyFCstI,RgUUgK Zr6dxzTdjwgGw6RwsrzCQ V39T5YYV,eikb4FWKwdU