NameError occurring after calling nested function
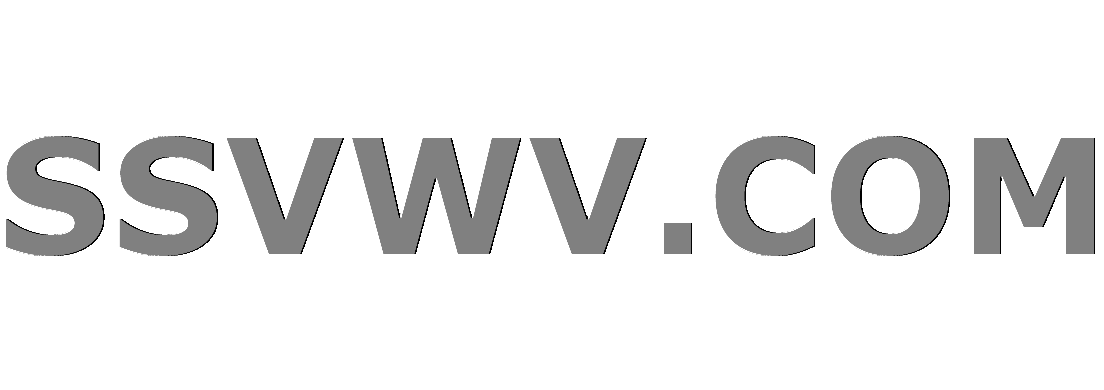
Multi tool use
up vote
1
down vote
favorite
So I split a .txt file into a list of lists (shown below). However, when I try to run print(splitKeyword(keywords[1][0]))
to try and print the first element of the second list/element within keywordList, I get the error: NameError: name 'keywordList' is not defined
. How can I fix this?
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
splitKeyword(textFileVar)
print(keywordList[1][0])
results = functionOne("text1.txt")
print(results)
This is the text1.txt/textFile/textFileVar contents
hello,world
123,456
This is what keywordList looks like when printed:
[[hello, world], [123, 456]]
python list function file
add a comment |
up vote
1
down vote
favorite
So I split a .txt file into a list of lists (shown below). However, when I try to run print(splitKeyword(keywords[1][0]))
to try and print the first element of the second list/element within keywordList, I get the error: NameError: name 'keywordList' is not defined
. How can I fix this?
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
splitKeyword(textFileVar)
print(keywordList[1][0])
results = functionOne("text1.txt")
print(results)
This is the text1.txt/textFile/textFileVar contents
hello,world
123,456
This is what keywordList looks like when printed:
[[hello, world], [123, 456]]
python list function file
1
you are returning from the function but not catching. so try to keywordList = splitKeyword(textFileVar) as keywordList is local to that function.
– Jay Parikh
Nov 11 at 5:41
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
So I split a .txt file into a list of lists (shown below). However, when I try to run print(splitKeyword(keywords[1][0]))
to try and print the first element of the second list/element within keywordList, I get the error: NameError: name 'keywordList' is not defined
. How can I fix this?
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
splitKeyword(textFileVar)
print(keywordList[1][0])
results = functionOne("text1.txt")
print(results)
This is the text1.txt/textFile/textFileVar contents
hello,world
123,456
This is what keywordList looks like when printed:
[[hello, world], [123, 456]]
python list function file
So I split a .txt file into a list of lists (shown below). However, when I try to run print(splitKeyword(keywords[1][0]))
to try and print the first element of the second list/element within keywordList, I get the error: NameError: name 'keywordList' is not defined
. How can I fix this?
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
splitKeyword(textFileVar)
print(keywordList[1][0])
results = functionOne("text1.txt")
print(results)
This is the text1.txt/textFile/textFileVar contents
hello,world
123,456
This is what keywordList looks like when printed:
[[hello, world], [123, 456]]
python list function file
python list function file
asked Nov 11 at 5:38
CosmicCat
505
505
1
you are returning from the function but not catching. so try to keywordList = splitKeyword(textFileVar) as keywordList is local to that function.
– Jay Parikh
Nov 11 at 5:41
add a comment |
1
you are returning from the function but not catching. so try to keywordList = splitKeyword(textFileVar) as keywordList is local to that function.
– Jay Parikh
Nov 11 at 5:41
1
1
you are returning from the function but not catching. so try to keywordList = splitKeyword(textFileVar) as keywordList is local to that function.
– Jay Parikh
Nov 11 at 5:41
you are returning from the function but not catching. so try to keywordList = splitKeyword(textFileVar) as keywordList is local to that function.
– Jay Parikh
Nov 11 at 5:41
add a comment |
3 Answers
3
active
oldest
votes
up vote
1
down vote
accepted
Try this:
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
output = splitKeyword(textFileVar)
print(output[1][0])
return output
results = functionOne("text1.txt")
print(results)
look at return keywordList
in splitKeyword
function. it returns the value(keywordList
). but in other scopes you can not access that variable, so you need to store that in something.
is the 'return output' needed? when I remove it, I get the console output I'm looking for but the word 'None' appears right under it
– CosmicCat
Nov 11 at 6:23
I think it is because of another line of your code. but remove it and check if you want.
– mehrdad-pedramfar
Nov 11 at 6:30
when I keep return output, I don't get none, but when I remove it, i get none, will this cause any issues?
– CosmicCat
Nov 11 at 6:37
that is because ofresults = functionOne("text1.txt")
. return output will return the value soresults
won't be empty(None), if you remove it, your function does not have any outputs so results would be None.
– mehrdad-pedramfar
Nov 11 at 6:44
add a comment |
up vote
0
down vote
Your keywordList
is local to the function splitKeyword()
, not to the function functionOne()
. That's why you're getting a NameError.
Is there a workaround?
– CosmicCat
Nov 11 at 5:49
@CosmicCat Well your function splitKeyword() returns something, so you might as well use this return right? Take what it returns in a variable and then use this variable to print it.
– Arjofocolovi
Nov 11 at 5:51
add a comment |
up vote
0
down vote
keywordlist is a local variable to the function splitKeyword which return it so you can directly use this function and reduce the code.
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
print(splitKeyword(textFileVar))
results = functionOne("text1.txt")
print(results)
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Try this:
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
output = splitKeyword(textFileVar)
print(output[1][0])
return output
results = functionOne("text1.txt")
print(results)
look at return keywordList
in splitKeyword
function. it returns the value(keywordList
). but in other scopes you can not access that variable, so you need to store that in something.
is the 'return output' needed? when I remove it, I get the console output I'm looking for but the word 'None' appears right under it
– CosmicCat
Nov 11 at 6:23
I think it is because of another line of your code. but remove it and check if you want.
– mehrdad-pedramfar
Nov 11 at 6:30
when I keep return output, I don't get none, but when I remove it, i get none, will this cause any issues?
– CosmicCat
Nov 11 at 6:37
that is because ofresults = functionOne("text1.txt")
. return output will return the value soresults
won't be empty(None), if you remove it, your function does not have any outputs so results would be None.
– mehrdad-pedramfar
Nov 11 at 6:44
add a comment |
up vote
1
down vote
accepted
Try this:
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
output = splitKeyword(textFileVar)
print(output[1][0])
return output
results = functionOne("text1.txt")
print(results)
look at return keywordList
in splitKeyword
function. it returns the value(keywordList
). but in other scopes you can not access that variable, so you need to store that in something.
is the 'return output' needed? when I remove it, I get the console output I'm looking for but the word 'None' appears right under it
– CosmicCat
Nov 11 at 6:23
I think it is because of another line of your code. but remove it and check if you want.
– mehrdad-pedramfar
Nov 11 at 6:30
when I keep return output, I don't get none, but when I remove it, i get none, will this cause any issues?
– CosmicCat
Nov 11 at 6:37
that is because ofresults = functionOne("text1.txt")
. return output will return the value soresults
won't be empty(None), if you remove it, your function does not have any outputs so results would be None.
– mehrdad-pedramfar
Nov 11 at 6:44
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Try this:
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
output = splitKeyword(textFileVar)
print(output[1][0])
return output
results = functionOne("text1.txt")
print(results)
look at return keywordList
in splitKeyword
function. it returns the value(keywordList
). but in other scopes you can not access that variable, so you need to store that in something.
Try this:
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
output = splitKeyword(textFileVar)
print(output[1][0])
return output
results = functionOne("text1.txt")
print(results)
look at return keywordList
in splitKeyword
function. it returns the value(keywordList
). but in other scopes you can not access that variable, so you need to store that in something.
answered Nov 11 at 6:13
mehrdad-pedramfar
3,83211233
3,83211233
is the 'return output' needed? when I remove it, I get the console output I'm looking for but the word 'None' appears right under it
– CosmicCat
Nov 11 at 6:23
I think it is because of another line of your code. but remove it and check if you want.
– mehrdad-pedramfar
Nov 11 at 6:30
when I keep return output, I don't get none, but when I remove it, i get none, will this cause any issues?
– CosmicCat
Nov 11 at 6:37
that is because ofresults = functionOne("text1.txt")
. return output will return the value soresults
won't be empty(None), if you remove it, your function does not have any outputs so results would be None.
– mehrdad-pedramfar
Nov 11 at 6:44
add a comment |
is the 'return output' needed? when I remove it, I get the console output I'm looking for but the word 'None' appears right under it
– CosmicCat
Nov 11 at 6:23
I think it is because of another line of your code. but remove it and check if you want.
– mehrdad-pedramfar
Nov 11 at 6:30
when I keep return output, I don't get none, but when I remove it, i get none, will this cause any issues?
– CosmicCat
Nov 11 at 6:37
that is because ofresults = functionOne("text1.txt")
. return output will return the value soresults
won't be empty(None), if you remove it, your function does not have any outputs so results would be None.
– mehrdad-pedramfar
Nov 11 at 6:44
is the 'return output' needed? when I remove it, I get the console output I'm looking for but the word 'None' appears right under it
– CosmicCat
Nov 11 at 6:23
is the 'return output' needed? when I remove it, I get the console output I'm looking for but the word 'None' appears right under it
– CosmicCat
Nov 11 at 6:23
I think it is because of another line of your code. but remove it and check if you want.
– mehrdad-pedramfar
Nov 11 at 6:30
I think it is because of another line of your code. but remove it and check if you want.
– mehrdad-pedramfar
Nov 11 at 6:30
when I keep return output, I don't get none, but when I remove it, i get none, will this cause any issues?
– CosmicCat
Nov 11 at 6:37
when I keep return output, I don't get none, but when I remove it, i get none, will this cause any issues?
– CosmicCat
Nov 11 at 6:37
that is because of
results = functionOne("text1.txt")
. return output will return the value so results
won't be empty(None), if you remove it, your function does not have any outputs so results would be None.– mehrdad-pedramfar
Nov 11 at 6:44
that is because of
results = functionOne("text1.txt")
. return output will return the value so results
won't be empty(None), if you remove it, your function does not have any outputs so results would be None.– mehrdad-pedramfar
Nov 11 at 6:44
add a comment |
up vote
0
down vote
Your keywordList
is local to the function splitKeyword()
, not to the function functionOne()
. That's why you're getting a NameError.
Is there a workaround?
– CosmicCat
Nov 11 at 5:49
@CosmicCat Well your function splitKeyword() returns something, so you might as well use this return right? Take what it returns in a variable and then use this variable to print it.
– Arjofocolovi
Nov 11 at 5:51
add a comment |
up vote
0
down vote
Your keywordList
is local to the function splitKeyword()
, not to the function functionOne()
. That's why you're getting a NameError.
Is there a workaround?
– CosmicCat
Nov 11 at 5:49
@CosmicCat Well your function splitKeyword() returns something, so you might as well use this return right? Take what it returns in a variable and then use this variable to print it.
– Arjofocolovi
Nov 11 at 5:51
add a comment |
up vote
0
down vote
up vote
0
down vote
Your keywordList
is local to the function splitKeyword()
, not to the function functionOne()
. That's why you're getting a NameError.
Your keywordList
is local to the function splitKeyword()
, not to the function functionOne()
. That's why you're getting a NameError.
answered Nov 11 at 5:45
Arjofocolovi
82
82
Is there a workaround?
– CosmicCat
Nov 11 at 5:49
@CosmicCat Well your function splitKeyword() returns something, so you might as well use this return right? Take what it returns in a variable and then use this variable to print it.
– Arjofocolovi
Nov 11 at 5:51
add a comment |
Is there a workaround?
– CosmicCat
Nov 11 at 5:49
@CosmicCat Well your function splitKeyword() returns something, so you might as well use this return right? Take what it returns in a variable and then use this variable to print it.
– Arjofocolovi
Nov 11 at 5:51
Is there a workaround?
– CosmicCat
Nov 11 at 5:49
Is there a workaround?
– CosmicCat
Nov 11 at 5:49
@CosmicCat Well your function splitKeyword() returns something, so you might as well use this return right? Take what it returns in a variable and then use this variable to print it.
– Arjofocolovi
Nov 11 at 5:51
@CosmicCat Well your function splitKeyword() returns something, so you might as well use this return right? Take what it returns in a variable and then use this variable to print it.
– Arjofocolovi
Nov 11 at 5:51
add a comment |
up vote
0
down vote
keywordlist is a local variable to the function splitKeyword which return it so you can directly use this function and reduce the code.
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
print(splitKeyword(textFileVar))
results = functionOne("text1.txt")
print(results)
add a comment |
up vote
0
down vote
keywordlist is a local variable to the function splitKeyword which return it so you can directly use this function and reduce the code.
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
print(splitKeyword(textFileVar))
results = functionOne("text1.txt")
print(results)
add a comment |
up vote
0
down vote
up vote
0
down vote
keywordlist is a local variable to the function splitKeyword which return it so you can directly use this function and reduce the code.
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
print(splitKeyword(textFileVar))
results = functionOne("text1.txt")
print(results)
keywordlist is a local variable to the function splitKeyword which return it so you can directly use this function and reduce the code.
def functionOne(textFile):
textFileVar = open(textFile, 'r')
def splitKeyword(argument):
keywordList =
for line in argument:
keywordList.append(line.strip().split(','))
return keywordList
print(splitKeyword(textFileVar))
results = functionOne("text1.txt")
print(results)
answered Nov 11 at 5:52
Moussa
788
788
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53246143%2fnameerror-occurring-after-calling-nested-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hNUX63eb
1
you are returning from the function but not catching. so try to keywordList = splitKeyword(textFileVar) as keywordList is local to that function.
– Jay Parikh
Nov 11 at 5:41