WKWebView on Mac - clear sensitive JavaScript calls from memory
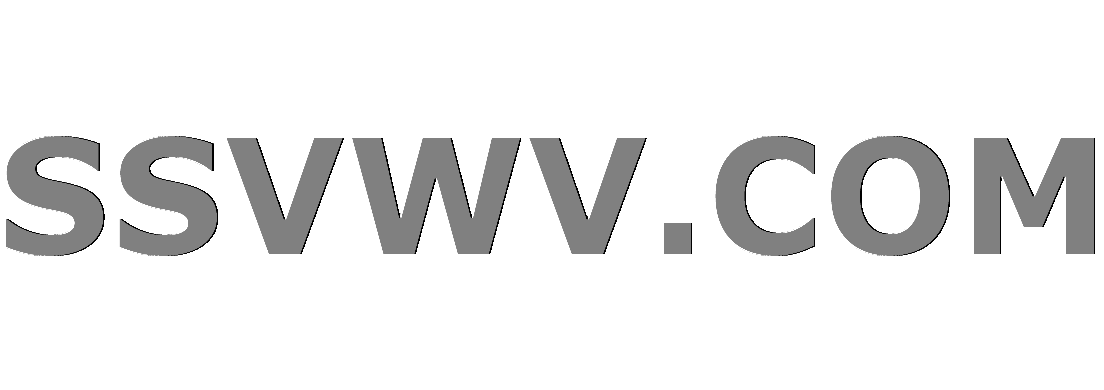
Multi tool use
I'm working on a Mac app written in Swift which loads a website in a WKWebView
using the following code:
let webConfiguration = WKWebViewConfiguration()
webConfiguration.websiteDataStore = WKWebsiteDataStore.nonPersistent() //set it to "private browsing"
self.webview = WKWebView(frame: .zero, configuration: webConfiguration)
self.webview?.uiDelegate = self
self.webview?.navigationDelegate = self
self.view.addSubview(self.webview!)
let myURL = URL(string: "http://127.0.0.1:8000/")
let mRequest = URLRequest(url: myURL!, cachePolicy: NSURLRequest.CachePolicy.reloadIgnoringLocalAndRemoteCacheData)
self.webview?.load(mRequest)
The native code then calls a javascript function on the site using:
self.webview?.evaluateJavaScript("randomjsfunction('" + secret + "')", completionHandler: nil)
The Javascript function exists, and runs correctly (it just removes an element from the page, nothing else).
Afterwards the WKWebView is removed together with the parent NSViewController
using:
let myURL = URL(string: "about:blank")
let mRequest = URLRequest(url: myURL!)
self.webview?.load(mRequest)
self.webview?.invalidateRestorableState()
self.webview?.stopLoading()
self.webview?.removeFromSuperview()
self.webview?.uiDelegate = nil
self.webview?.navigationDelegate = nil
self.webview = nil
self.removeFromParent()
self.dismiss(self.parent)
After a few minutes I create a memory dump of the process, and the Javascipt function call together with the passed parameters are still present in memory:
I would need to ensure that the parameters of the Javascript call are cleared from memory once it's no longer used. However not even destroying the entire webview seems to free up that memory.
I'v tried to add the JS call into a userscript using the addUserScript()
function and then calling that function with evaluateJavaScript()
, but even the addUserScript()
calls are presented in memory.
Based on WebPage RunJavaScriptInMainFrame
presented in the dump I found the underlying function in WebKit's code. But I don't know how to progress on that end.
javascript swift memory-management wkwebview
add a comment |
I'm working on a Mac app written in Swift which loads a website in a WKWebView
using the following code:
let webConfiguration = WKWebViewConfiguration()
webConfiguration.websiteDataStore = WKWebsiteDataStore.nonPersistent() //set it to "private browsing"
self.webview = WKWebView(frame: .zero, configuration: webConfiguration)
self.webview?.uiDelegate = self
self.webview?.navigationDelegate = self
self.view.addSubview(self.webview!)
let myURL = URL(string: "http://127.0.0.1:8000/")
let mRequest = URLRequest(url: myURL!, cachePolicy: NSURLRequest.CachePolicy.reloadIgnoringLocalAndRemoteCacheData)
self.webview?.load(mRequest)
The native code then calls a javascript function on the site using:
self.webview?.evaluateJavaScript("randomjsfunction('" + secret + "')", completionHandler: nil)
The Javascript function exists, and runs correctly (it just removes an element from the page, nothing else).
Afterwards the WKWebView is removed together with the parent NSViewController
using:
let myURL = URL(string: "about:blank")
let mRequest = URLRequest(url: myURL!)
self.webview?.load(mRequest)
self.webview?.invalidateRestorableState()
self.webview?.stopLoading()
self.webview?.removeFromSuperview()
self.webview?.uiDelegate = nil
self.webview?.navigationDelegate = nil
self.webview = nil
self.removeFromParent()
self.dismiss(self.parent)
After a few minutes I create a memory dump of the process, and the Javascipt function call together with the passed parameters are still present in memory:
I would need to ensure that the parameters of the Javascript call are cleared from memory once it's no longer used. However not even destroying the entire webview seems to free up that memory.
I'v tried to add the JS call into a userscript using the addUserScript()
function and then calling that function with evaluateJavaScript()
, but even the addUserScript()
calls are presented in memory.
Based on WebPage RunJavaScriptInMainFrame
presented in the dump I found the underlying function in WebKit's code. But I don't know how to progress on that end.
javascript swift memory-management wkwebview
add a comment |
I'm working on a Mac app written in Swift which loads a website in a WKWebView
using the following code:
let webConfiguration = WKWebViewConfiguration()
webConfiguration.websiteDataStore = WKWebsiteDataStore.nonPersistent() //set it to "private browsing"
self.webview = WKWebView(frame: .zero, configuration: webConfiguration)
self.webview?.uiDelegate = self
self.webview?.navigationDelegate = self
self.view.addSubview(self.webview!)
let myURL = URL(string: "http://127.0.0.1:8000/")
let mRequest = URLRequest(url: myURL!, cachePolicy: NSURLRequest.CachePolicy.reloadIgnoringLocalAndRemoteCacheData)
self.webview?.load(mRequest)
The native code then calls a javascript function on the site using:
self.webview?.evaluateJavaScript("randomjsfunction('" + secret + "')", completionHandler: nil)
The Javascript function exists, and runs correctly (it just removes an element from the page, nothing else).
Afterwards the WKWebView is removed together with the parent NSViewController
using:
let myURL = URL(string: "about:blank")
let mRequest = URLRequest(url: myURL!)
self.webview?.load(mRequest)
self.webview?.invalidateRestorableState()
self.webview?.stopLoading()
self.webview?.removeFromSuperview()
self.webview?.uiDelegate = nil
self.webview?.navigationDelegate = nil
self.webview = nil
self.removeFromParent()
self.dismiss(self.parent)
After a few minutes I create a memory dump of the process, and the Javascipt function call together with the passed parameters are still present in memory:
I would need to ensure that the parameters of the Javascript call are cleared from memory once it's no longer used. However not even destroying the entire webview seems to free up that memory.
I'v tried to add the JS call into a userscript using the addUserScript()
function and then calling that function with evaluateJavaScript()
, but even the addUserScript()
calls are presented in memory.
Based on WebPage RunJavaScriptInMainFrame
presented in the dump I found the underlying function in WebKit's code. But I don't know how to progress on that end.
javascript swift memory-management wkwebview
I'm working on a Mac app written in Swift which loads a website in a WKWebView
using the following code:
let webConfiguration = WKWebViewConfiguration()
webConfiguration.websiteDataStore = WKWebsiteDataStore.nonPersistent() //set it to "private browsing"
self.webview = WKWebView(frame: .zero, configuration: webConfiguration)
self.webview?.uiDelegate = self
self.webview?.navigationDelegate = self
self.view.addSubview(self.webview!)
let myURL = URL(string: "http://127.0.0.1:8000/")
let mRequest = URLRequest(url: myURL!, cachePolicy: NSURLRequest.CachePolicy.reloadIgnoringLocalAndRemoteCacheData)
self.webview?.load(mRequest)
The native code then calls a javascript function on the site using:
self.webview?.evaluateJavaScript("randomjsfunction('" + secret + "')", completionHandler: nil)
The Javascript function exists, and runs correctly (it just removes an element from the page, nothing else).
Afterwards the WKWebView is removed together with the parent NSViewController
using:
let myURL = URL(string: "about:blank")
let mRequest = URLRequest(url: myURL!)
self.webview?.load(mRequest)
self.webview?.invalidateRestorableState()
self.webview?.stopLoading()
self.webview?.removeFromSuperview()
self.webview?.uiDelegate = nil
self.webview?.navigationDelegate = nil
self.webview = nil
self.removeFromParent()
self.dismiss(self.parent)
After a few minutes I create a memory dump of the process, and the Javascipt function call together with the passed parameters are still present in memory:
I would need to ensure that the parameters of the Javascript call are cleared from memory once it's no longer used. However not even destroying the entire webview seems to free up that memory.
I'v tried to add the JS call into a userscript using the addUserScript()
function and then calling that function with evaluateJavaScript()
, but even the addUserScript()
calls are presented in memory.
Based on WebPage RunJavaScriptInMainFrame
presented in the dump I found the underlying function in WebKit's code. But I don't know how to progress on that end.
javascript swift memory-management wkwebview
javascript swift memory-management wkwebview
asked Nov 13 '18 at 13:53
Torin42Torin42
215
215
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282570%2fwkwebview-on-mac-clear-sensitive-javascript-calls-from-memory%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282570%2fwkwebview-on-mac-clear-sensitive-javascript-calls-from-memory%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ssxIc DibJ2 2v8hKypjwllZm0EMQ0EMPosuG