How to open whole html page as modal?
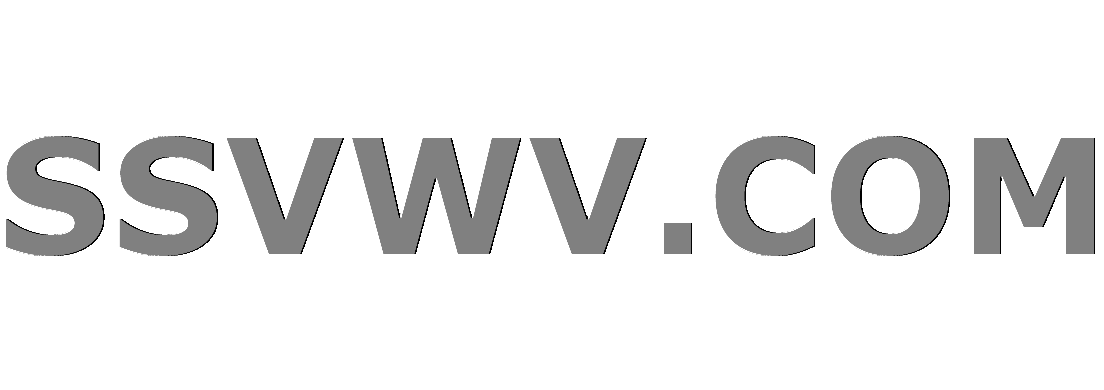
Multi tool use
up vote
0
down vote
favorite
I'm very new to angularjs and currently studying mean stack. I'm using this code to open an html page then getting the id of clicked user.
<a href="/edit/{{ person._id }}">
<button type="button" ng-show="management.editAccess" class="btn btn-primary">Edit</button>
</a>
But I want to open my html as a modal, I tried this method here http://plnkr.co/edit/Y7jDj2hORWmJ95c96qoG?p=preview but it doesn't show the html, Please help me on how to open my whole html as modal.
angularjs mean-stack
add a comment |
up vote
0
down vote
favorite
I'm very new to angularjs and currently studying mean stack. I'm using this code to open an html page then getting the id of clicked user.
<a href="/edit/{{ person._id }}">
<button type="button" ng-show="management.editAccess" class="btn btn-primary">Edit</button>
</a>
But I want to open my html as a modal, I tried this method here http://plnkr.co/edit/Y7jDj2hORWmJ95c96qoG?p=preview but it doesn't show the html, Please help me on how to open my whole html as modal.
angularjs mean-stack
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm very new to angularjs and currently studying mean stack. I'm using this code to open an html page then getting the id of clicked user.
<a href="/edit/{{ person._id }}">
<button type="button" ng-show="management.editAccess" class="btn btn-primary">Edit</button>
</a>
But I want to open my html as a modal, I tried this method here http://plnkr.co/edit/Y7jDj2hORWmJ95c96qoG?p=preview but it doesn't show the html, Please help me on how to open my whole html as modal.
angularjs mean-stack
I'm very new to angularjs and currently studying mean stack. I'm using this code to open an html page then getting the id of clicked user.
<a href="/edit/{{ person._id }}">
<button type="button" ng-show="management.editAccess" class="btn btn-primary">Edit</button>
</a>
But I want to open my html as a modal, I tried this method here http://plnkr.co/edit/Y7jDj2hORWmJ95c96qoG?p=preview but it doesn't show the html, Please help me on how to open my whole html as modal.
angularjs mean-stack
angularjs mean-stack
asked Nov 11 at 11:15


In Utero
12110
12110
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
You need to $http.get
the template in html and then using $sce
render it.
<button data-toggle="modal" data-target="#theModal" ng-click="get()">get</button>
<div id="theModal" class="modal fade text-center">
<div class="modal-dialog">
<div class="modal-content">
<div ng-bind-html="trustedHtml"></div>
</div>
</div>
</div>
JS:
$scope.get = function() {
$http({
method: 'GET',
url: 'Lab6.html',
})
.then(function successCallback(data){
$scope.data = data.data;
console.log($scope.data);
$scope.trustedHtml = $sce.trustAsHtml($scope.data);
},
function errorCallback(response){
console.log(response);
console.log('error');
});
}
Here is working plunker: http://plnkr.co/edit/SdCAep1dDH8UcpprwDH6?p=preview
Also I would argue that you should probably be using Angular UI Bootstrap to create your modal dialogs http://angular-ui.github.io/bootstrap/
Here is a cut down version of how to open a modal using Angular UI Bootrstrap:
$scope.open = function (vehicle) {
var modalInstance = $modal.open({
templateUrl: 'myModalContent.html',
resolve: {
items: function () {
return $scope.items;
}
}
});
};
it's working for my pages with MainCtrl but my edit.html has editCtrl, how should I resolve this
– In Utero
Nov 11 at 12:33
@InUtero Use ui.bootstrap (in which You'll be able to pass $scope to other controller) - read documentation about ui.bootstrap.modal,
– BartoszTermena
Nov 11 at 12:45
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
You need to $http.get
the template in html and then using $sce
render it.
<button data-toggle="modal" data-target="#theModal" ng-click="get()">get</button>
<div id="theModal" class="modal fade text-center">
<div class="modal-dialog">
<div class="modal-content">
<div ng-bind-html="trustedHtml"></div>
</div>
</div>
</div>
JS:
$scope.get = function() {
$http({
method: 'GET',
url: 'Lab6.html',
})
.then(function successCallback(data){
$scope.data = data.data;
console.log($scope.data);
$scope.trustedHtml = $sce.trustAsHtml($scope.data);
},
function errorCallback(response){
console.log(response);
console.log('error');
});
}
Here is working plunker: http://plnkr.co/edit/SdCAep1dDH8UcpprwDH6?p=preview
Also I would argue that you should probably be using Angular UI Bootstrap to create your modal dialogs http://angular-ui.github.io/bootstrap/
Here is a cut down version of how to open a modal using Angular UI Bootrstrap:
$scope.open = function (vehicle) {
var modalInstance = $modal.open({
templateUrl: 'myModalContent.html',
resolve: {
items: function () {
return $scope.items;
}
}
});
};
it's working for my pages with MainCtrl but my edit.html has editCtrl, how should I resolve this
– In Utero
Nov 11 at 12:33
@InUtero Use ui.bootstrap (in which You'll be able to pass $scope to other controller) - read documentation about ui.bootstrap.modal,
– BartoszTermena
Nov 11 at 12:45
add a comment |
up vote
1
down vote
You need to $http.get
the template in html and then using $sce
render it.
<button data-toggle="modal" data-target="#theModal" ng-click="get()">get</button>
<div id="theModal" class="modal fade text-center">
<div class="modal-dialog">
<div class="modal-content">
<div ng-bind-html="trustedHtml"></div>
</div>
</div>
</div>
JS:
$scope.get = function() {
$http({
method: 'GET',
url: 'Lab6.html',
})
.then(function successCallback(data){
$scope.data = data.data;
console.log($scope.data);
$scope.trustedHtml = $sce.trustAsHtml($scope.data);
},
function errorCallback(response){
console.log(response);
console.log('error');
});
}
Here is working plunker: http://plnkr.co/edit/SdCAep1dDH8UcpprwDH6?p=preview
Also I would argue that you should probably be using Angular UI Bootstrap to create your modal dialogs http://angular-ui.github.io/bootstrap/
Here is a cut down version of how to open a modal using Angular UI Bootrstrap:
$scope.open = function (vehicle) {
var modalInstance = $modal.open({
templateUrl: 'myModalContent.html',
resolve: {
items: function () {
return $scope.items;
}
}
});
};
it's working for my pages with MainCtrl but my edit.html has editCtrl, how should I resolve this
– In Utero
Nov 11 at 12:33
@InUtero Use ui.bootstrap (in which You'll be able to pass $scope to other controller) - read documentation about ui.bootstrap.modal,
– BartoszTermena
Nov 11 at 12:45
add a comment |
up vote
1
down vote
up vote
1
down vote
You need to $http.get
the template in html and then using $sce
render it.
<button data-toggle="modal" data-target="#theModal" ng-click="get()">get</button>
<div id="theModal" class="modal fade text-center">
<div class="modal-dialog">
<div class="modal-content">
<div ng-bind-html="trustedHtml"></div>
</div>
</div>
</div>
JS:
$scope.get = function() {
$http({
method: 'GET',
url: 'Lab6.html',
})
.then(function successCallback(data){
$scope.data = data.data;
console.log($scope.data);
$scope.trustedHtml = $sce.trustAsHtml($scope.data);
},
function errorCallback(response){
console.log(response);
console.log('error');
});
}
Here is working plunker: http://plnkr.co/edit/SdCAep1dDH8UcpprwDH6?p=preview
Also I would argue that you should probably be using Angular UI Bootstrap to create your modal dialogs http://angular-ui.github.io/bootstrap/
Here is a cut down version of how to open a modal using Angular UI Bootrstrap:
$scope.open = function (vehicle) {
var modalInstance = $modal.open({
templateUrl: 'myModalContent.html',
resolve: {
items: function () {
return $scope.items;
}
}
});
};
You need to $http.get
the template in html and then using $sce
render it.
<button data-toggle="modal" data-target="#theModal" ng-click="get()">get</button>
<div id="theModal" class="modal fade text-center">
<div class="modal-dialog">
<div class="modal-content">
<div ng-bind-html="trustedHtml"></div>
</div>
</div>
</div>
JS:
$scope.get = function() {
$http({
method: 'GET',
url: 'Lab6.html',
})
.then(function successCallback(data){
$scope.data = data.data;
console.log($scope.data);
$scope.trustedHtml = $sce.trustAsHtml($scope.data);
},
function errorCallback(response){
console.log(response);
console.log('error');
});
}
Here is working plunker: http://plnkr.co/edit/SdCAep1dDH8UcpprwDH6?p=preview
Also I would argue that you should probably be using Angular UI Bootstrap to create your modal dialogs http://angular-ui.github.io/bootstrap/
Here is a cut down version of how to open a modal using Angular UI Bootrstrap:
$scope.open = function (vehicle) {
var modalInstance = $modal.open({
templateUrl: 'myModalContent.html',
resolve: {
items: function () {
return $scope.items;
}
}
});
};
answered Nov 11 at 12:05
BartoszTermena
37816
37816
it's working for my pages with MainCtrl but my edit.html has editCtrl, how should I resolve this
– In Utero
Nov 11 at 12:33
@InUtero Use ui.bootstrap (in which You'll be able to pass $scope to other controller) - read documentation about ui.bootstrap.modal,
– BartoszTermena
Nov 11 at 12:45
add a comment |
it's working for my pages with MainCtrl but my edit.html has editCtrl, how should I resolve this
– In Utero
Nov 11 at 12:33
@InUtero Use ui.bootstrap (in which You'll be able to pass $scope to other controller) - read documentation about ui.bootstrap.modal,
– BartoszTermena
Nov 11 at 12:45
it's working for my pages with MainCtrl but my edit.html has editCtrl, how should I resolve this
– In Utero
Nov 11 at 12:33
it's working for my pages with MainCtrl but my edit.html has editCtrl, how should I resolve this
– In Utero
Nov 11 at 12:33
@InUtero Use ui.bootstrap (in which You'll be able to pass $scope to other controller) - read documentation about ui.bootstrap.modal,
– BartoszTermena
Nov 11 at 12:45
@InUtero Use ui.bootstrap (in which You'll be able to pass $scope to other controller) - read documentation about ui.bootstrap.modal,
– BartoszTermena
Nov 11 at 12:45
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53248160%2fhow-to-open-whole-html-page-as-modal%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
jKpbfTOCtsfvLcIl6YqPcTxeAJo6Ag9QJhqgvzp0NtximY8VlmJ7aJSmm,Cs3AhbQb5Paq9