Android create file causing java.io.IOException
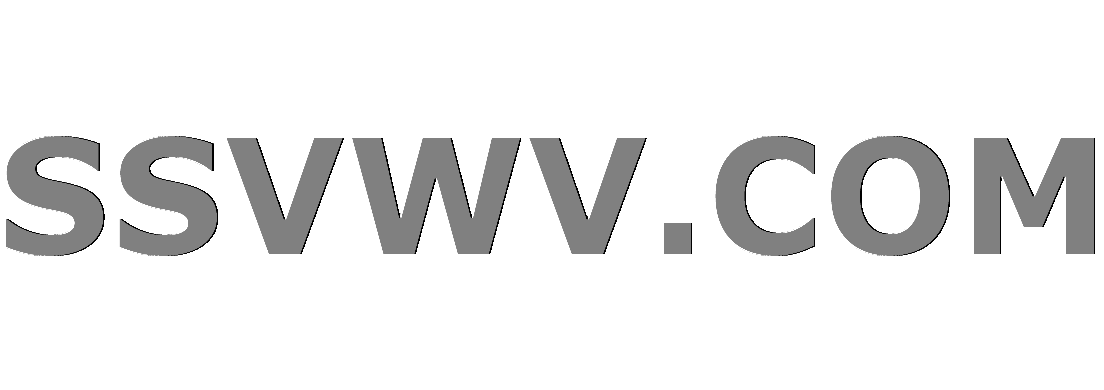
Multi tool use
up vote
0
down vote
favorite
I’m trying to implement a way to create pdf files in my app. Currently I’m using PDF Writer APW for that. I followed the instruction from jguerinet and pasted all necessary files to the project (as new package). But when I run the code, I always get the below exception. I also tried to use File downloads = Environment.getExternalStorageDirectory();
instead of File downloads = Environment.getDataDirectory();
wihtout any sucess. Can someone please tell me, what I’m doing wrong?
2018-11-10 12:52:38.843 31238-31238/com.abc.systeminfo I/PDF: Writing file to /data/PDFWriterAPW.pdf
2018-11-10 12:52:38.845 31238-31238/com.abc.systeminfo W/PDF IOException: java.io.IOException: Permission denied
at java.io.UnixFileSystem.createFileExclusively0(Native Method)
at java.io.UnixFileSystem.createFileExclusively(UnixFileSystem.java:281)
at java.io.File.createNewFile(File.java:1000)
at com.abc.systeminfo.CreateFile.outputToFile(CreateFile.java:72)
at com.abc.systeminfo.CreateFile.<init>(CreateFile.java:28)
at com.abc.systeminfo.ActivityStart$1.onClick(ActivityStart.java:54)
at android.view.View.performClick(View.java:6256)
at android.view.View$PerformClick.run(View.java:24701)
at android.os.Handler.handleCallback(Handler.java:789)
at android.os.Handler.dispatchMessage(Handler.java:98)
at android.os.Looper.loop(Looper.java:164)
at android.app.ActivityThread.main(ActivityThread.java:6541)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:240)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:767)
CreateFile.java
package com.abc.systeminfo;
import android.os.Environment;
import android.util.Log;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import crl.android.pdfwriter.PDFWriter;
import crl.android.pdfwriter.StandardFonts;
import crl.android.pdfwriter.Transformation;
public class CreateFile {
PDFWriter mPDFWriter;
String pdfcontent;
String encoding = "ISO-8859-1";
String fileName = "PDFWriterAPW.pdf";
public CreateFile(String pdfcontent) {
mPDFWriter = new PDFWriter();
this.pdfcontent = pdfcontent; // will be used later instead of String s
String s = generateHelloWorldPDF();
outputToFile(fileName, s, encoding);
}
public String generateHelloWorldPDF() {
mPDFWriter.setFont(StandardFonts.SUBTYPE, StandardFonts.TIMES_ROMAN);
mPDFWriter.addRawContent("1 0 0 rgn");
mPDFWriter.addTextAsHex(70, 50, 12, "68656c6c6f20776f726c6420286173206865782921");
mPDFWriter.setFont(StandardFonts.SUBTYPE, StandardFonts.COURIER, StandardFonts.WIN_ANSI_ENCODING);
mPDFWriter.addRawContent("0 0 0 rgn");
mPDFWriter.addText(30, 90, 10, "� CRL", Transformation.DEGREES_270_ROTATION);
mPDFWriter.newPage();
mPDFWriter.addRawContent(" 0 dn");
mPDFWriter.addRawContent("1 wn");
mPDFWriter.addRawContent("0 0 1 RGn");
mPDFWriter.addRawContent("0 1 0 rgn");
mPDFWriter.addRectangle(40, 50, 280, 50);
mPDFWriter.addText(85, 75, 18, "Code Research Laboratories");
mPDFWriter.newPage();
mPDFWriter.setFont(StandardFonts.SUBTYPE, StandardFonts.COURIER_BOLD);
mPDFWriter.addText(150, 150, 14, "http://coderesearchlabs.com");
mPDFWriter.addLine(150, 140, 270, 140);
int pageCount = mPDFWriter.getPageCount();
for (int i = 0; i < pageCount; i++) {
mPDFWriter.setCurrentPage(i);
mPDFWriter.addText(10, 10, 8, Integer.toString(i + 1) + " / " + Integer.toString(pageCount));
}
String s = mPDFWriter.asString();
return s;
}
private void outputToFile(String fileName, String pdfContent, String encoding) {
File downloads = Environment.getDataDirectory();
//File downloads = Environment.getExternalStorageDirectory();
if (!downloads.exists() && !downloads.mkdirs())
throw new RuntimeException("Could not create download folder");
File newFile = new File(downloads, fileName);
Log.i("PDF", "Writing file to " + newFile);
try {
newFile.createNewFile();
try {
FileOutputStream pdfFile = new FileOutputStream(newFile);
pdfFile.write(pdfContent.getBytes(encoding));
pdfFile.close();
} catch (FileNotFoundException e) {
Log.w("PDF FileNotFound ", e);
}
} catch (IOException e) {
Log.w("PDF IOException", e);
}
}
}
AndroidManifest
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.abc.systeminfo">
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".ActivityStart">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>

New contributor
Edward84 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
I’m trying to implement a way to create pdf files in my app. Currently I’m using PDF Writer APW for that. I followed the instruction from jguerinet and pasted all necessary files to the project (as new package). But when I run the code, I always get the below exception. I also tried to use File downloads = Environment.getExternalStorageDirectory();
instead of File downloads = Environment.getDataDirectory();
wihtout any sucess. Can someone please tell me, what I’m doing wrong?
2018-11-10 12:52:38.843 31238-31238/com.abc.systeminfo I/PDF: Writing file to /data/PDFWriterAPW.pdf
2018-11-10 12:52:38.845 31238-31238/com.abc.systeminfo W/PDF IOException: java.io.IOException: Permission denied
at java.io.UnixFileSystem.createFileExclusively0(Native Method)
at java.io.UnixFileSystem.createFileExclusively(UnixFileSystem.java:281)
at java.io.File.createNewFile(File.java:1000)
at com.abc.systeminfo.CreateFile.outputToFile(CreateFile.java:72)
at com.abc.systeminfo.CreateFile.<init>(CreateFile.java:28)
at com.abc.systeminfo.ActivityStart$1.onClick(ActivityStart.java:54)
at android.view.View.performClick(View.java:6256)
at android.view.View$PerformClick.run(View.java:24701)
at android.os.Handler.handleCallback(Handler.java:789)
at android.os.Handler.dispatchMessage(Handler.java:98)
at android.os.Looper.loop(Looper.java:164)
at android.app.ActivityThread.main(ActivityThread.java:6541)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:240)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:767)
CreateFile.java
package com.abc.systeminfo;
import android.os.Environment;
import android.util.Log;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import crl.android.pdfwriter.PDFWriter;
import crl.android.pdfwriter.StandardFonts;
import crl.android.pdfwriter.Transformation;
public class CreateFile {
PDFWriter mPDFWriter;
String pdfcontent;
String encoding = "ISO-8859-1";
String fileName = "PDFWriterAPW.pdf";
public CreateFile(String pdfcontent) {
mPDFWriter = new PDFWriter();
this.pdfcontent = pdfcontent; // will be used later instead of String s
String s = generateHelloWorldPDF();
outputToFile(fileName, s, encoding);
}
public String generateHelloWorldPDF() {
mPDFWriter.setFont(StandardFonts.SUBTYPE, StandardFonts.TIMES_ROMAN);
mPDFWriter.addRawContent("1 0 0 rgn");
mPDFWriter.addTextAsHex(70, 50, 12, "68656c6c6f20776f726c6420286173206865782921");
mPDFWriter.setFont(StandardFonts.SUBTYPE, StandardFonts.COURIER, StandardFonts.WIN_ANSI_ENCODING);
mPDFWriter.addRawContent("0 0 0 rgn");
mPDFWriter.addText(30, 90, 10, "� CRL", Transformation.DEGREES_270_ROTATION);
mPDFWriter.newPage();
mPDFWriter.addRawContent(" 0 dn");
mPDFWriter.addRawContent("1 wn");
mPDFWriter.addRawContent("0 0 1 RGn");
mPDFWriter.addRawContent("0 1 0 rgn");
mPDFWriter.addRectangle(40, 50, 280, 50);
mPDFWriter.addText(85, 75, 18, "Code Research Laboratories");
mPDFWriter.newPage();
mPDFWriter.setFont(StandardFonts.SUBTYPE, StandardFonts.COURIER_BOLD);
mPDFWriter.addText(150, 150, 14, "http://coderesearchlabs.com");
mPDFWriter.addLine(150, 140, 270, 140);
int pageCount = mPDFWriter.getPageCount();
for (int i = 0; i < pageCount; i++) {
mPDFWriter.setCurrentPage(i);
mPDFWriter.addText(10, 10, 8, Integer.toString(i + 1) + " / " + Integer.toString(pageCount));
}
String s = mPDFWriter.asString();
return s;
}
private void outputToFile(String fileName, String pdfContent, String encoding) {
File downloads = Environment.getDataDirectory();
//File downloads = Environment.getExternalStorageDirectory();
if (!downloads.exists() && !downloads.mkdirs())
throw new RuntimeException("Could not create download folder");
File newFile = new File(downloads, fileName);
Log.i("PDF", "Writing file to " + newFile);
try {
newFile.createNewFile();
try {
FileOutputStream pdfFile = new FileOutputStream(newFile);
pdfFile.write(pdfContent.getBytes(encoding));
pdfFile.close();
} catch (FileNotFoundException e) {
Log.w("PDF FileNotFound ", e);
}
} catch (IOException e) {
Log.w("PDF IOException", e);
}
}
}
AndroidManifest
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.abc.systeminfo">
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".ActivityStart">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>

New contributor
Edward84 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I’m trying to implement a way to create pdf files in my app. Currently I’m using PDF Writer APW for that. I followed the instruction from jguerinet and pasted all necessary files to the project (as new package). But when I run the code, I always get the below exception. I also tried to use File downloads = Environment.getExternalStorageDirectory();
instead of File downloads = Environment.getDataDirectory();
wihtout any sucess. Can someone please tell me, what I’m doing wrong?
2018-11-10 12:52:38.843 31238-31238/com.abc.systeminfo I/PDF: Writing file to /data/PDFWriterAPW.pdf
2018-11-10 12:52:38.845 31238-31238/com.abc.systeminfo W/PDF IOException: java.io.IOException: Permission denied
at java.io.UnixFileSystem.createFileExclusively0(Native Method)
at java.io.UnixFileSystem.createFileExclusively(UnixFileSystem.java:281)
at java.io.File.createNewFile(File.java:1000)
at com.abc.systeminfo.CreateFile.outputToFile(CreateFile.java:72)
at com.abc.systeminfo.CreateFile.<init>(CreateFile.java:28)
at com.abc.systeminfo.ActivityStart$1.onClick(ActivityStart.java:54)
at android.view.View.performClick(View.java:6256)
at android.view.View$PerformClick.run(View.java:24701)
at android.os.Handler.handleCallback(Handler.java:789)
at android.os.Handler.dispatchMessage(Handler.java:98)
at android.os.Looper.loop(Looper.java:164)
at android.app.ActivityThread.main(ActivityThread.java:6541)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:240)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:767)
CreateFile.java
package com.abc.systeminfo;
import android.os.Environment;
import android.util.Log;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import crl.android.pdfwriter.PDFWriter;
import crl.android.pdfwriter.StandardFonts;
import crl.android.pdfwriter.Transformation;
public class CreateFile {
PDFWriter mPDFWriter;
String pdfcontent;
String encoding = "ISO-8859-1";
String fileName = "PDFWriterAPW.pdf";
public CreateFile(String pdfcontent) {
mPDFWriter = new PDFWriter();
this.pdfcontent = pdfcontent; // will be used later instead of String s
String s = generateHelloWorldPDF();
outputToFile(fileName, s, encoding);
}
public String generateHelloWorldPDF() {
mPDFWriter.setFont(StandardFonts.SUBTYPE, StandardFonts.TIMES_ROMAN);
mPDFWriter.addRawContent("1 0 0 rgn");
mPDFWriter.addTextAsHex(70, 50, 12, "68656c6c6f20776f726c6420286173206865782921");
mPDFWriter.setFont(StandardFonts.SUBTYPE, StandardFonts.COURIER, StandardFonts.WIN_ANSI_ENCODING);
mPDFWriter.addRawContent("0 0 0 rgn");
mPDFWriter.addText(30, 90, 10, "� CRL", Transformation.DEGREES_270_ROTATION);
mPDFWriter.newPage();
mPDFWriter.addRawContent(" 0 dn");
mPDFWriter.addRawContent("1 wn");
mPDFWriter.addRawContent("0 0 1 RGn");
mPDFWriter.addRawContent("0 1 0 rgn");
mPDFWriter.addRectangle(40, 50, 280, 50);
mPDFWriter.addText(85, 75, 18, "Code Research Laboratories");
mPDFWriter.newPage();
mPDFWriter.setFont(StandardFonts.SUBTYPE, StandardFonts.COURIER_BOLD);
mPDFWriter.addText(150, 150, 14, "http://coderesearchlabs.com");
mPDFWriter.addLine(150, 140, 270, 140);
int pageCount = mPDFWriter.getPageCount();
for (int i = 0; i < pageCount; i++) {
mPDFWriter.setCurrentPage(i);
mPDFWriter.addText(10, 10, 8, Integer.toString(i + 1) + " / " + Integer.toString(pageCount));
}
String s = mPDFWriter.asString();
return s;
}
private void outputToFile(String fileName, String pdfContent, String encoding) {
File downloads = Environment.getDataDirectory();
//File downloads = Environment.getExternalStorageDirectory();
if (!downloads.exists() && !downloads.mkdirs())
throw new RuntimeException("Could not create download folder");
File newFile = new File(downloads, fileName);
Log.i("PDF", "Writing file to " + newFile);
try {
newFile.createNewFile();
try {
FileOutputStream pdfFile = new FileOutputStream(newFile);
pdfFile.write(pdfContent.getBytes(encoding));
pdfFile.close();
} catch (FileNotFoundException e) {
Log.w("PDF FileNotFound ", e);
}
} catch (IOException e) {
Log.w("PDF IOException", e);
}
}
}
AndroidManifest
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.abc.systeminfo">
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".ActivityStart">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>

New contributor
Edward84 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I’m trying to implement a way to create pdf files in my app. Currently I’m using PDF Writer APW for that. I followed the instruction from jguerinet and pasted all necessary files to the project (as new package). But when I run the code, I always get the below exception. I also tried to use File downloads = Environment.getExternalStorageDirectory();
instead of File downloads = Environment.getDataDirectory();
wihtout any sucess. Can someone please tell me, what I’m doing wrong?
2018-11-10 12:52:38.843 31238-31238/com.abc.systeminfo I/PDF: Writing file to /data/PDFWriterAPW.pdf
2018-11-10 12:52:38.845 31238-31238/com.abc.systeminfo W/PDF IOException: java.io.IOException: Permission denied
at java.io.UnixFileSystem.createFileExclusively0(Native Method)
at java.io.UnixFileSystem.createFileExclusively(UnixFileSystem.java:281)
at java.io.File.createNewFile(File.java:1000)
at com.abc.systeminfo.CreateFile.outputToFile(CreateFile.java:72)
at com.abc.systeminfo.CreateFile.<init>(CreateFile.java:28)
at com.abc.systeminfo.ActivityStart$1.onClick(ActivityStart.java:54)
at android.view.View.performClick(View.java:6256)
at android.view.View$PerformClick.run(View.java:24701)
at android.os.Handler.handleCallback(Handler.java:789)
at android.os.Handler.dispatchMessage(Handler.java:98)
at android.os.Looper.loop(Looper.java:164)
at android.app.ActivityThread.main(ActivityThread.java:6541)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:240)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:767)
CreateFile.java
package com.abc.systeminfo;
import android.os.Environment;
import android.util.Log;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import crl.android.pdfwriter.PDFWriter;
import crl.android.pdfwriter.StandardFonts;
import crl.android.pdfwriter.Transformation;
public class CreateFile {
PDFWriter mPDFWriter;
String pdfcontent;
String encoding = "ISO-8859-1";
String fileName = "PDFWriterAPW.pdf";
public CreateFile(String pdfcontent) {
mPDFWriter = new PDFWriter();
this.pdfcontent = pdfcontent; // will be used later instead of String s
String s = generateHelloWorldPDF();
outputToFile(fileName, s, encoding);
}
public String generateHelloWorldPDF() {
mPDFWriter.setFont(StandardFonts.SUBTYPE, StandardFonts.TIMES_ROMAN);
mPDFWriter.addRawContent("1 0 0 rgn");
mPDFWriter.addTextAsHex(70, 50, 12, "68656c6c6f20776f726c6420286173206865782921");
mPDFWriter.setFont(StandardFonts.SUBTYPE, StandardFonts.COURIER, StandardFonts.WIN_ANSI_ENCODING);
mPDFWriter.addRawContent("0 0 0 rgn");
mPDFWriter.addText(30, 90, 10, "� CRL", Transformation.DEGREES_270_ROTATION);
mPDFWriter.newPage();
mPDFWriter.addRawContent(" 0 dn");
mPDFWriter.addRawContent("1 wn");
mPDFWriter.addRawContent("0 0 1 RGn");
mPDFWriter.addRawContent("0 1 0 rgn");
mPDFWriter.addRectangle(40, 50, 280, 50);
mPDFWriter.addText(85, 75, 18, "Code Research Laboratories");
mPDFWriter.newPage();
mPDFWriter.setFont(StandardFonts.SUBTYPE, StandardFonts.COURIER_BOLD);
mPDFWriter.addText(150, 150, 14, "http://coderesearchlabs.com");
mPDFWriter.addLine(150, 140, 270, 140);
int pageCount = mPDFWriter.getPageCount();
for (int i = 0; i < pageCount; i++) {
mPDFWriter.setCurrentPage(i);
mPDFWriter.addText(10, 10, 8, Integer.toString(i + 1) + " / " + Integer.toString(pageCount));
}
String s = mPDFWriter.asString();
return s;
}
private void outputToFile(String fileName, String pdfContent, String encoding) {
File downloads = Environment.getDataDirectory();
//File downloads = Environment.getExternalStorageDirectory();
if (!downloads.exists() && !downloads.mkdirs())
throw new RuntimeException("Could not create download folder");
File newFile = new File(downloads, fileName);
Log.i("PDF", "Writing file to " + newFile);
try {
newFile.createNewFile();
try {
FileOutputStream pdfFile = new FileOutputStream(newFile);
pdfFile.write(pdfContent.getBytes(encoding));
pdfFile.close();
} catch (FileNotFoundException e) {
Log.w("PDF FileNotFound ", e);
}
} catch (IOException e) {
Log.w("PDF IOException", e);
}
}
}
AndroidManifest
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.abc.systeminfo">
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".ActivityStart">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>


New contributor
Edward84 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Edward84 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Edward84 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Nov 10 at 12:05
Edward84
61
61
New contributor
Edward84 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Edward84 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Edward84 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
You need to request permission in runtime before doing that operation like below (this is needed for devices which use lollipop and above android os)
if (ContextCompat.checkSelfPermission(thisActivity,Manifest.permission.WRITE_EXTERNAL_STORAGE)!= PackageManager.PERMISSION_GRANTED) {
// Permission is not granted
if (ActivityCompat.shouldShowRequestPermissionRationale(thisActivity,
Manifest.permission.WRITE_EXTERNAL_STORAGE)) {
// Show an explanation to the user *asynchronously* -- don't block
// this thread waiting for the user's response! After the user
// sees the explanation, try again to request the permission.
} else {
// No explanation needed; request the permission
ActivityCompat.requestPermissions(thisActivity,
new String{Manifest.permission.WRITE_EXTERNAL_STORAGE},
MY_PERMISSIONS_WRITE_EXTERNAL_STORAGE);
}
}
That worked for writing on external storage. Thank you a lot for that. But why can't I write a file to internal storage usingFile downloads = Environment.getDataDirectory();
?
– Edward84
Nov 10 at 14:24
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You need to request permission in runtime before doing that operation like below (this is needed for devices which use lollipop and above android os)
if (ContextCompat.checkSelfPermission(thisActivity,Manifest.permission.WRITE_EXTERNAL_STORAGE)!= PackageManager.PERMISSION_GRANTED) {
// Permission is not granted
if (ActivityCompat.shouldShowRequestPermissionRationale(thisActivity,
Manifest.permission.WRITE_EXTERNAL_STORAGE)) {
// Show an explanation to the user *asynchronously* -- don't block
// this thread waiting for the user's response! After the user
// sees the explanation, try again to request the permission.
} else {
// No explanation needed; request the permission
ActivityCompat.requestPermissions(thisActivity,
new String{Manifest.permission.WRITE_EXTERNAL_STORAGE},
MY_PERMISSIONS_WRITE_EXTERNAL_STORAGE);
}
}
That worked for writing on external storage. Thank you a lot for that. But why can't I write a file to internal storage usingFile downloads = Environment.getDataDirectory();
?
– Edward84
Nov 10 at 14:24
add a comment |
up vote
0
down vote
You need to request permission in runtime before doing that operation like below (this is needed for devices which use lollipop and above android os)
if (ContextCompat.checkSelfPermission(thisActivity,Manifest.permission.WRITE_EXTERNAL_STORAGE)!= PackageManager.PERMISSION_GRANTED) {
// Permission is not granted
if (ActivityCompat.shouldShowRequestPermissionRationale(thisActivity,
Manifest.permission.WRITE_EXTERNAL_STORAGE)) {
// Show an explanation to the user *asynchronously* -- don't block
// this thread waiting for the user's response! After the user
// sees the explanation, try again to request the permission.
} else {
// No explanation needed; request the permission
ActivityCompat.requestPermissions(thisActivity,
new String{Manifest.permission.WRITE_EXTERNAL_STORAGE},
MY_PERMISSIONS_WRITE_EXTERNAL_STORAGE);
}
}
That worked for writing on external storage. Thank you a lot for that. But why can't I write a file to internal storage usingFile downloads = Environment.getDataDirectory();
?
– Edward84
Nov 10 at 14:24
add a comment |
up vote
0
down vote
up vote
0
down vote
You need to request permission in runtime before doing that operation like below (this is needed for devices which use lollipop and above android os)
if (ContextCompat.checkSelfPermission(thisActivity,Manifest.permission.WRITE_EXTERNAL_STORAGE)!= PackageManager.PERMISSION_GRANTED) {
// Permission is not granted
if (ActivityCompat.shouldShowRequestPermissionRationale(thisActivity,
Manifest.permission.WRITE_EXTERNAL_STORAGE)) {
// Show an explanation to the user *asynchronously* -- don't block
// this thread waiting for the user's response! After the user
// sees the explanation, try again to request the permission.
} else {
// No explanation needed; request the permission
ActivityCompat.requestPermissions(thisActivity,
new String{Manifest.permission.WRITE_EXTERNAL_STORAGE},
MY_PERMISSIONS_WRITE_EXTERNAL_STORAGE);
}
}
You need to request permission in runtime before doing that operation like below (this is needed for devices which use lollipop and above android os)
if (ContextCompat.checkSelfPermission(thisActivity,Manifest.permission.WRITE_EXTERNAL_STORAGE)!= PackageManager.PERMISSION_GRANTED) {
// Permission is not granted
if (ActivityCompat.shouldShowRequestPermissionRationale(thisActivity,
Manifest.permission.WRITE_EXTERNAL_STORAGE)) {
// Show an explanation to the user *asynchronously* -- don't block
// this thread waiting for the user's response! After the user
// sees the explanation, try again to request the permission.
} else {
// No explanation needed; request the permission
ActivityCompat.requestPermissions(thisActivity,
new String{Manifest.permission.WRITE_EXTERNAL_STORAGE},
MY_PERMISSIONS_WRITE_EXTERNAL_STORAGE);
}
}
answered Nov 10 at 12:22


Lucefer
8001510
8001510
That worked for writing on external storage. Thank you a lot for that. But why can't I write a file to internal storage usingFile downloads = Environment.getDataDirectory();
?
– Edward84
Nov 10 at 14:24
add a comment |
That worked for writing on external storage. Thank you a lot for that. But why can't I write a file to internal storage usingFile downloads = Environment.getDataDirectory();
?
– Edward84
Nov 10 at 14:24
That worked for writing on external storage. Thank you a lot for that. But why can't I write a file to internal storage using
File downloads = Environment.getDataDirectory();
?– Edward84
Nov 10 at 14:24
That worked for writing on external storage. Thank you a lot for that. But why can't I write a file to internal storage using
File downloads = Environment.getDataDirectory();
?– Edward84
Nov 10 at 14:24
add a comment |
Edward84 is a new contributor. Be nice, and check out our Code of Conduct.
Edward84 is a new contributor. Be nice, and check out our Code of Conduct.
Edward84 is a new contributor. Be nice, and check out our Code of Conduct.
Edward84 is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53238761%2fandroid-create-file-causing-java-io-ioexception%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
nk7C7Isb siXp1GXraZ5HyHQo1jNC8qKoMf27XQX WjgzH6WXpR,8gQ s3 J mw8lsivvUVSBqsSHB5m kS SjFI9,htzIqUFXPh3Zi