Serializing multiple documents into one file using YamlDotNet
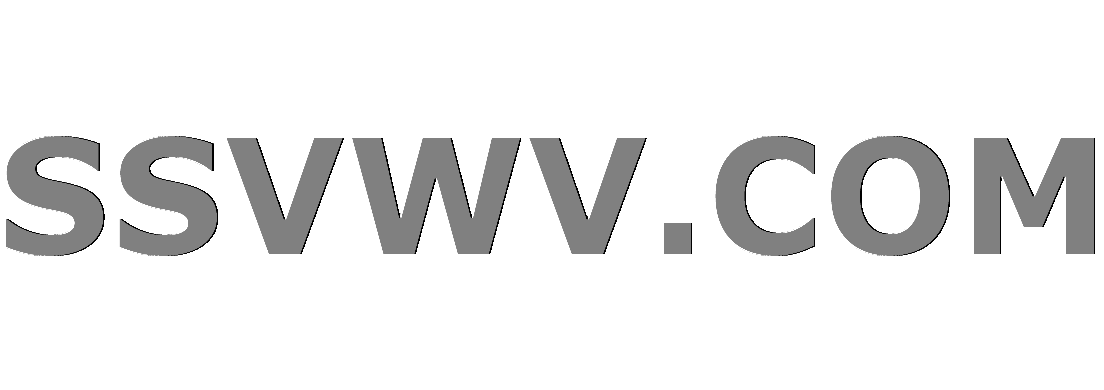
Multi tool use
I have an instrument that has multiple accessories that can be attached and removed. Each accessory has settings specific to it, and some of those settings will change when the accessory is recalibrated.
I want to store the setting data for the accessories as documents in a single YAML file using YamlDotNet. The settings file will be updated whenever an accessory is recalibrated. I've found plenty of posts on deserializing multiple documents in a single file, but nothing on serializing multiple documents INTO a single file (I'm new to YamlDotNet. Can you tell?). Specifically, how do you get the document separator, ---, and the end document indicator, ..., into the file?
For the sake of illustration, here's some example code. First, a class to hold accessory settings:
public class Accessory
{
public string AccessoryName { get; set; }
public float CalibrationConstant1 { get; set; }
public float CalibrationConstant2 { get; set; }
public DateTime CalibrationDate { get; set; }
}
and a list to hold a number of accessories (could be anything from one to ten of them):
public class AccessoryList
{
public List<Accessory> accessory;
public AccessoryList()
{
accessory = new List<Accessory>();
}
}
My attempt at serializing the settings is:
ISerializer serializer = new SerializerBuilder().EmitDefaults().Build();
string file = Application.StartupPath + @"Accessories.yml";
using (StreamWriter writer = File.CreateText(file))
foreach (Accessory accessory in AccessoryList.accessory)
{
serializer.Serialize(writer, accessory);
}
So, let's say we have two accessories. The resulting .yml file would look like this:
AccessoryName: Gargleblaster Pan-Galactic
CalibrationConstant1: 3.1415927
CalibrationConstant2: 2.718
CalibrationDate: 2018-11-13T12:33:55.9900338-05:00
AccessoryName: Phaser Set To Stun
CalibrationConstant1: 1.234321
CalibrationConstant2: 1.618
CalibrationDate: 2018-11-10T01:23:37.1453278-05:00
As expected, no sign of --- or ..., and when I deserialize the file I get only the second accessory's data, strongly indicating that I need to get those sequences in there. I tried writing strings in quotes, but what appeared in the file was, drum roll please, strings in quotes.
Any help would be greatly appreciated! Sometimes the most obvious things are the hardest for a newbie to see.
serialization yamldotnet
add a comment |
I have an instrument that has multiple accessories that can be attached and removed. Each accessory has settings specific to it, and some of those settings will change when the accessory is recalibrated.
I want to store the setting data for the accessories as documents in a single YAML file using YamlDotNet. The settings file will be updated whenever an accessory is recalibrated. I've found plenty of posts on deserializing multiple documents in a single file, but nothing on serializing multiple documents INTO a single file (I'm new to YamlDotNet. Can you tell?). Specifically, how do you get the document separator, ---, and the end document indicator, ..., into the file?
For the sake of illustration, here's some example code. First, a class to hold accessory settings:
public class Accessory
{
public string AccessoryName { get; set; }
public float CalibrationConstant1 { get; set; }
public float CalibrationConstant2 { get; set; }
public DateTime CalibrationDate { get; set; }
}
and a list to hold a number of accessories (could be anything from one to ten of them):
public class AccessoryList
{
public List<Accessory> accessory;
public AccessoryList()
{
accessory = new List<Accessory>();
}
}
My attempt at serializing the settings is:
ISerializer serializer = new SerializerBuilder().EmitDefaults().Build();
string file = Application.StartupPath + @"Accessories.yml";
using (StreamWriter writer = File.CreateText(file))
foreach (Accessory accessory in AccessoryList.accessory)
{
serializer.Serialize(writer, accessory);
}
So, let's say we have two accessories. The resulting .yml file would look like this:
AccessoryName: Gargleblaster Pan-Galactic
CalibrationConstant1: 3.1415927
CalibrationConstant2: 2.718
CalibrationDate: 2018-11-13T12:33:55.9900338-05:00
AccessoryName: Phaser Set To Stun
CalibrationConstant1: 1.234321
CalibrationConstant2: 1.618
CalibrationDate: 2018-11-10T01:23:37.1453278-05:00
As expected, no sign of --- or ..., and when I deserialize the file I get only the second accessory's data, strongly indicating that I need to get those sequences in there. I tried writing strings in quotes, but what appeared in the file was, drum roll please, strings in quotes.
Any help would be greatly appreciated! Sometimes the most obvious things are the hardest for a newbie to see.
serialization yamldotnet
add a comment |
I have an instrument that has multiple accessories that can be attached and removed. Each accessory has settings specific to it, and some of those settings will change when the accessory is recalibrated.
I want to store the setting data for the accessories as documents in a single YAML file using YamlDotNet. The settings file will be updated whenever an accessory is recalibrated. I've found plenty of posts on deserializing multiple documents in a single file, but nothing on serializing multiple documents INTO a single file (I'm new to YamlDotNet. Can you tell?). Specifically, how do you get the document separator, ---, and the end document indicator, ..., into the file?
For the sake of illustration, here's some example code. First, a class to hold accessory settings:
public class Accessory
{
public string AccessoryName { get; set; }
public float CalibrationConstant1 { get; set; }
public float CalibrationConstant2 { get; set; }
public DateTime CalibrationDate { get; set; }
}
and a list to hold a number of accessories (could be anything from one to ten of them):
public class AccessoryList
{
public List<Accessory> accessory;
public AccessoryList()
{
accessory = new List<Accessory>();
}
}
My attempt at serializing the settings is:
ISerializer serializer = new SerializerBuilder().EmitDefaults().Build();
string file = Application.StartupPath + @"Accessories.yml";
using (StreamWriter writer = File.CreateText(file))
foreach (Accessory accessory in AccessoryList.accessory)
{
serializer.Serialize(writer, accessory);
}
So, let's say we have two accessories. The resulting .yml file would look like this:
AccessoryName: Gargleblaster Pan-Galactic
CalibrationConstant1: 3.1415927
CalibrationConstant2: 2.718
CalibrationDate: 2018-11-13T12:33:55.9900338-05:00
AccessoryName: Phaser Set To Stun
CalibrationConstant1: 1.234321
CalibrationConstant2: 1.618
CalibrationDate: 2018-11-10T01:23:37.1453278-05:00
As expected, no sign of --- or ..., and when I deserialize the file I get only the second accessory's data, strongly indicating that I need to get those sequences in there. I tried writing strings in quotes, but what appeared in the file was, drum roll please, strings in quotes.
Any help would be greatly appreciated! Sometimes the most obvious things are the hardest for a newbie to see.
serialization yamldotnet
I have an instrument that has multiple accessories that can be attached and removed. Each accessory has settings specific to it, and some of those settings will change when the accessory is recalibrated.
I want to store the setting data for the accessories as documents in a single YAML file using YamlDotNet. The settings file will be updated whenever an accessory is recalibrated. I've found plenty of posts on deserializing multiple documents in a single file, but nothing on serializing multiple documents INTO a single file (I'm new to YamlDotNet. Can you tell?). Specifically, how do you get the document separator, ---, and the end document indicator, ..., into the file?
For the sake of illustration, here's some example code. First, a class to hold accessory settings:
public class Accessory
{
public string AccessoryName { get; set; }
public float CalibrationConstant1 { get; set; }
public float CalibrationConstant2 { get; set; }
public DateTime CalibrationDate { get; set; }
}
and a list to hold a number of accessories (could be anything from one to ten of them):
public class AccessoryList
{
public List<Accessory> accessory;
public AccessoryList()
{
accessory = new List<Accessory>();
}
}
My attempt at serializing the settings is:
ISerializer serializer = new SerializerBuilder().EmitDefaults().Build();
string file = Application.StartupPath + @"Accessories.yml";
using (StreamWriter writer = File.CreateText(file))
foreach (Accessory accessory in AccessoryList.accessory)
{
serializer.Serialize(writer, accessory);
}
So, let's say we have two accessories. The resulting .yml file would look like this:
AccessoryName: Gargleblaster Pan-Galactic
CalibrationConstant1: 3.1415927
CalibrationConstant2: 2.718
CalibrationDate: 2018-11-13T12:33:55.9900338-05:00
AccessoryName: Phaser Set To Stun
CalibrationConstant1: 1.234321
CalibrationConstant2: 1.618
CalibrationDate: 2018-11-10T01:23:37.1453278-05:00
As expected, no sign of --- or ..., and when I deserialize the file I get only the second accessory's data, strongly indicating that I need to get those sequences in there. I tried writing strings in quotes, but what appeared in the file was, drum roll please, strings in quotes.
Any help would be greatly appreciated! Sometimes the most obvious things are the hardest for a newbie to see.
serialization yamldotnet
serialization yamldotnet
asked Nov 13 '18 at 18:49
Dave RDave R
214
214
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53287680%2fserializing-multiple-documents-into-one-file-using-yamldotnet%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53287680%2fserializing-multiple-documents-into-one-file-using-yamldotnet%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
n6k774gu ux RW