How to use Redux with React
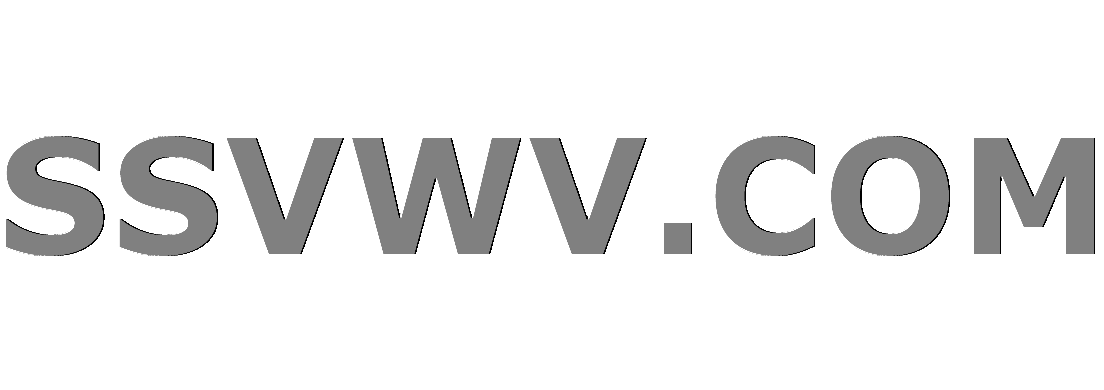
Multi tool use
What I Just want to fetch data from api and show it at frontend. I am using Redux to call the api using it's ACTIONS and REDUCERS. In Reducers i take the intialstate as empty array.When API is successfully called, I am updating store state.Below is the practical which can help to understand concept easily.
store.js
import { createStore } from 'redux';
import reducer from './reducers/reducer';
let store = createStore(reducer)
export default store
actions.js
import {
FETCH_IMAGES_SUCCESS
} from './actionTypes'
export function fetchImages() {
return dispatch => {
return fetch("https://api.com/data")
.then(res => res.json())
.then(json => {
dispatch(fetchImagesSuccess(json.posts));
return json.posts;
})
};
}
export const fetchImagesSuccess = images => ({
type: FETCH_IMAGES_SUCCESS,
payload: { images }
});
reducer.js
import {
FETCH_IMAGES_SUCCESS
} from '../actions/actionTypes'
const initialState = {
images:
}
const reducer = (state = initialState, action) => {
switch (action.type) {
case FETCH_IMAGES_SUCCESS:
return {...state,images:action.payload.images}
default:
return state
}
}
export default reducer;
Now, Please tell me what should i need to do to call that Redux action and
get Data from the API.I am using React to display data.
Thanks.
reactjs redux
add a comment |
What I Just want to fetch data from api and show it at frontend. I am using Redux to call the api using it's ACTIONS and REDUCERS. In Reducers i take the intialstate as empty array.When API is successfully called, I am updating store state.Below is the practical which can help to understand concept easily.
store.js
import { createStore } from 'redux';
import reducer from './reducers/reducer';
let store = createStore(reducer)
export default store
actions.js
import {
FETCH_IMAGES_SUCCESS
} from './actionTypes'
export function fetchImages() {
return dispatch => {
return fetch("https://api.com/data")
.then(res => res.json())
.then(json => {
dispatch(fetchImagesSuccess(json.posts));
return json.posts;
})
};
}
export const fetchImagesSuccess = images => ({
type: FETCH_IMAGES_SUCCESS,
payload: { images }
});
reducer.js
import {
FETCH_IMAGES_SUCCESS
} from '../actions/actionTypes'
const initialState = {
images:
}
const reducer = (state = initialState, action) => {
switch (action.type) {
case FETCH_IMAGES_SUCCESS:
return {...state,images:action.payload.images}
default:
return state
}
}
export default reducer;
Now, Please tell me what should i need to do to call that Redux action and
get Data from the API.I am using React to display data.
Thanks.
reactjs redux
3
redux.js.org/basics/usagewithreact
– AndyJ
Nov 13 '18 at 16:01
I don't want the documentation link. Can you explain me in shorten?
– user10298495
Nov 13 '18 at 16:03
@Rajat That is just being lazy
– Boy With Silver Wings
Nov 13 '18 at 16:11
1
@Rajat Just sit down and read about Redux and react-redux (connector between react and redux) first. Docs are actually meant for that. SO is meant for situations when you get stuck programmatically doing something beyond docs maybe
– kushalvm
Nov 13 '18 at 16:22
add a comment |
What I Just want to fetch data from api and show it at frontend. I am using Redux to call the api using it's ACTIONS and REDUCERS. In Reducers i take the intialstate as empty array.When API is successfully called, I am updating store state.Below is the practical which can help to understand concept easily.
store.js
import { createStore } from 'redux';
import reducer from './reducers/reducer';
let store = createStore(reducer)
export default store
actions.js
import {
FETCH_IMAGES_SUCCESS
} from './actionTypes'
export function fetchImages() {
return dispatch => {
return fetch("https://api.com/data")
.then(res => res.json())
.then(json => {
dispatch(fetchImagesSuccess(json.posts));
return json.posts;
})
};
}
export const fetchImagesSuccess = images => ({
type: FETCH_IMAGES_SUCCESS,
payload: { images }
});
reducer.js
import {
FETCH_IMAGES_SUCCESS
} from '../actions/actionTypes'
const initialState = {
images:
}
const reducer = (state = initialState, action) => {
switch (action.type) {
case FETCH_IMAGES_SUCCESS:
return {...state,images:action.payload.images}
default:
return state
}
}
export default reducer;
Now, Please tell me what should i need to do to call that Redux action and
get Data from the API.I am using React to display data.
Thanks.
reactjs redux
What I Just want to fetch data from api and show it at frontend. I am using Redux to call the api using it's ACTIONS and REDUCERS. In Reducers i take the intialstate as empty array.When API is successfully called, I am updating store state.Below is the practical which can help to understand concept easily.
store.js
import { createStore } from 'redux';
import reducer from './reducers/reducer';
let store = createStore(reducer)
export default store
actions.js
import {
FETCH_IMAGES_SUCCESS
} from './actionTypes'
export function fetchImages() {
return dispatch => {
return fetch("https://api.com/data")
.then(res => res.json())
.then(json => {
dispatch(fetchImagesSuccess(json.posts));
return json.posts;
})
};
}
export const fetchImagesSuccess = images => ({
type: FETCH_IMAGES_SUCCESS,
payload: { images }
});
reducer.js
import {
FETCH_IMAGES_SUCCESS
} from '../actions/actionTypes'
const initialState = {
images:
}
const reducer = (state = initialState, action) => {
switch (action.type) {
case FETCH_IMAGES_SUCCESS:
return {...state,images:action.payload.images}
default:
return state
}
}
export default reducer;
Now, Please tell me what should i need to do to call that Redux action and
get Data from the API.I am using React to display data.
Thanks.
reactjs redux
reactjs redux
edited Nov 13 '18 at 15:56
asked Nov 13 '18 at 15:50
user10298495
3
redux.js.org/basics/usagewithreact
– AndyJ
Nov 13 '18 at 16:01
I don't want the documentation link. Can you explain me in shorten?
– user10298495
Nov 13 '18 at 16:03
@Rajat That is just being lazy
– Boy With Silver Wings
Nov 13 '18 at 16:11
1
@Rajat Just sit down and read about Redux and react-redux (connector between react and redux) first. Docs are actually meant for that. SO is meant for situations when you get stuck programmatically doing something beyond docs maybe
– kushalvm
Nov 13 '18 at 16:22
add a comment |
3
redux.js.org/basics/usagewithreact
– AndyJ
Nov 13 '18 at 16:01
I don't want the documentation link. Can you explain me in shorten?
– user10298495
Nov 13 '18 at 16:03
@Rajat That is just being lazy
– Boy With Silver Wings
Nov 13 '18 at 16:11
1
@Rajat Just sit down and read about Redux and react-redux (connector between react and redux) first. Docs are actually meant for that. SO is meant for situations when you get stuck programmatically doing something beyond docs maybe
– kushalvm
Nov 13 '18 at 16:22
3
3
redux.js.org/basics/usagewithreact
– AndyJ
Nov 13 '18 at 16:01
redux.js.org/basics/usagewithreact
– AndyJ
Nov 13 '18 at 16:01
I don't want the documentation link. Can you explain me in shorten?
– user10298495
Nov 13 '18 at 16:03
I don't want the documentation link. Can you explain me in shorten?
– user10298495
Nov 13 '18 at 16:03
@Rajat That is just being lazy
– Boy With Silver Wings
Nov 13 '18 at 16:11
@Rajat That is just being lazy
– Boy With Silver Wings
Nov 13 '18 at 16:11
1
1
@Rajat Just sit down and read about Redux and react-redux (connector between react and redux) first. Docs are actually meant for that. SO is meant for situations when you get stuck programmatically doing something beyond docs maybe
– kushalvm
Nov 13 '18 at 16:22
@Rajat Just sit down and read about Redux and react-redux (connector between react and redux) first. Docs are actually meant for that. SO is meant for situations when you get stuck programmatically doing something beyond docs maybe
– kushalvm
Nov 13 '18 at 16:22
add a comment |
4 Answers
4
active
oldest
votes
In React redux usage page you can use functions like mapStateToProps and connect to do that
add a comment |
You need a middleware like Redux-Saga or Redux-Thunk to talk with the actions and the global store maintained using Redux.
You may follow this Tutorial: https://redux.js.org/basics/exampletodolist
If you are going with Redux-Thunk, you need to modify your store assign like this:
const store = createStore(rootReducer, applyMiddleware(thunk));
Now, have a container to all the Parent component you have.
import { connect } from 'react-redux';
import App from '../components/App';
export function mapStateToProps(appState) {
return {
/* this is where you get your store data through the reducer returned
state */
};
}
export function mapDispatchToProps(dispatch) {
return {
// make all your action dispatches here
// for ex: getData(payload) => dispatch({type: GETDATA, payload: payload})
};
}
export default connect(mapStateToProps, mapDispatchToProps)(App);
i am using redux-thunk. Please explain me How can i call a Redux action from my React frontend and then got the returned state?
– user10298495
Nov 13 '18 at 16:08
I am seeing "<Provider store={store}>" in the todo example. what is Provider and do i need to use it in react?
– user10298495
Nov 13 '18 at 16:14
@Rajat Provider infuses the store to your component at root level. This is Composition. I think you need to go through react documents properly. it is well explained there
– Ashish Kirodian
Nov 13 '18 at 16:17
Can you please edit your above code, where you used the redux thunk? As you didn't import it and tell how to use it with store. Thanks.
– user10298495
Nov 13 '18 at 16:30
@Rajat check the first line of code in my answer. middleware is assigned when you create store. which is done at index.js
– Ashish Kirodian
Nov 13 '18 at 16:32
|
show 6 more comments
As Mustafa said you need to use mapStateToProps
. Let me explain myself.
What you just done is just the configuration for the main store (there's only one in redux). Now you need to use it in your components, but how ? When you create a Component the content of the store
will be passed as props
with the help of Containers
.
Containers are the way to link your store with your react component.
Said that, you need to install redux
and react-redux
. In your code above you have successfully configured the store with the reducers with redux
library. Now you need react-redux
to create the Container (which wraps your react component).
Here is an example of how to put this all together:
https://codepen.io/anon/pen/RqKyQZ?editors=1010
Thanks for your answer. But can you please tell me how can i?
– user10298495
Nov 13 '18 at 16:17
can you please share any refrence with me. But please don't share docs link as they are lengthy to read.
– user10298495
Nov 13 '18 at 16:19
i will provide an example, give me a minute.
– giwiro
Nov 13 '18 at 16:19
sure, please do.
– user10298495
Nov 13 '18 at 16:20
done: codepen.io/anon/pen/RqKyQZ?editors=1010 If you found it useful like it ^^
– giwiro
Nov 13 '18 at 16:38
|
show 6 more comments
You need to use mapStateToProps similar to the code below. Let say your reducer is called test and it is part of a state.
const mapStateToProps = (state, props) =>
({
router: props.router,
test: state.test
});
Then test
will be used as a property in a React class. Obviously you need to include respective imports for React.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53284689%2fhow-to-use-redux-with-react%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
In React redux usage page you can use functions like mapStateToProps and connect to do that
add a comment |
In React redux usage page you can use functions like mapStateToProps and connect to do that
add a comment |
In React redux usage page you can use functions like mapStateToProps and connect to do that
In React redux usage page you can use functions like mapStateToProps and connect to do that
answered Nov 13 '18 at 16:09


Mustafa MohamedMustafa Mohamed
311
311
add a comment |
add a comment |
You need a middleware like Redux-Saga or Redux-Thunk to talk with the actions and the global store maintained using Redux.
You may follow this Tutorial: https://redux.js.org/basics/exampletodolist
If you are going with Redux-Thunk, you need to modify your store assign like this:
const store = createStore(rootReducer, applyMiddleware(thunk));
Now, have a container to all the Parent component you have.
import { connect } from 'react-redux';
import App from '../components/App';
export function mapStateToProps(appState) {
return {
/* this is where you get your store data through the reducer returned
state */
};
}
export function mapDispatchToProps(dispatch) {
return {
// make all your action dispatches here
// for ex: getData(payload) => dispatch({type: GETDATA, payload: payload})
};
}
export default connect(mapStateToProps, mapDispatchToProps)(App);
i am using redux-thunk. Please explain me How can i call a Redux action from my React frontend and then got the returned state?
– user10298495
Nov 13 '18 at 16:08
I am seeing "<Provider store={store}>" in the todo example. what is Provider and do i need to use it in react?
– user10298495
Nov 13 '18 at 16:14
@Rajat Provider infuses the store to your component at root level. This is Composition. I think you need to go through react documents properly. it is well explained there
– Ashish Kirodian
Nov 13 '18 at 16:17
Can you please edit your above code, where you used the redux thunk? As you didn't import it and tell how to use it with store. Thanks.
– user10298495
Nov 13 '18 at 16:30
@Rajat check the first line of code in my answer. middleware is assigned when you create store. which is done at index.js
– Ashish Kirodian
Nov 13 '18 at 16:32
|
show 6 more comments
You need a middleware like Redux-Saga or Redux-Thunk to talk with the actions and the global store maintained using Redux.
You may follow this Tutorial: https://redux.js.org/basics/exampletodolist
If you are going with Redux-Thunk, you need to modify your store assign like this:
const store = createStore(rootReducer, applyMiddleware(thunk));
Now, have a container to all the Parent component you have.
import { connect } from 'react-redux';
import App from '../components/App';
export function mapStateToProps(appState) {
return {
/* this is where you get your store data through the reducer returned
state */
};
}
export function mapDispatchToProps(dispatch) {
return {
// make all your action dispatches here
// for ex: getData(payload) => dispatch({type: GETDATA, payload: payload})
};
}
export default connect(mapStateToProps, mapDispatchToProps)(App);
i am using redux-thunk. Please explain me How can i call a Redux action from my React frontend and then got the returned state?
– user10298495
Nov 13 '18 at 16:08
I am seeing "<Provider store={store}>" in the todo example. what is Provider and do i need to use it in react?
– user10298495
Nov 13 '18 at 16:14
@Rajat Provider infuses the store to your component at root level. This is Composition. I think you need to go through react documents properly. it is well explained there
– Ashish Kirodian
Nov 13 '18 at 16:17
Can you please edit your above code, where you used the redux thunk? As you didn't import it and tell how to use it with store. Thanks.
– user10298495
Nov 13 '18 at 16:30
@Rajat check the first line of code in my answer. middleware is assigned when you create store. which is done at index.js
– Ashish Kirodian
Nov 13 '18 at 16:32
|
show 6 more comments
You need a middleware like Redux-Saga or Redux-Thunk to talk with the actions and the global store maintained using Redux.
You may follow this Tutorial: https://redux.js.org/basics/exampletodolist
If you are going with Redux-Thunk, you need to modify your store assign like this:
const store = createStore(rootReducer, applyMiddleware(thunk));
Now, have a container to all the Parent component you have.
import { connect } from 'react-redux';
import App from '../components/App';
export function mapStateToProps(appState) {
return {
/* this is where you get your store data through the reducer returned
state */
};
}
export function mapDispatchToProps(dispatch) {
return {
// make all your action dispatches here
// for ex: getData(payload) => dispatch({type: GETDATA, payload: payload})
};
}
export default connect(mapStateToProps, mapDispatchToProps)(App);
You need a middleware like Redux-Saga or Redux-Thunk to talk with the actions and the global store maintained using Redux.
You may follow this Tutorial: https://redux.js.org/basics/exampletodolist
If you are going with Redux-Thunk, you need to modify your store assign like this:
const store = createStore(rootReducer, applyMiddleware(thunk));
Now, have a container to all the Parent component you have.
import { connect } from 'react-redux';
import App from '../components/App';
export function mapStateToProps(appState) {
return {
/* this is where you get your store data through the reducer returned
state */
};
}
export function mapDispatchToProps(dispatch) {
return {
// make all your action dispatches here
// for ex: getData(payload) => dispatch({type: GETDATA, payload: payload})
};
}
export default connect(mapStateToProps, mapDispatchToProps)(App);
edited Nov 13 '18 at 16:15
answered Nov 13 '18 at 16:05


Ashish KirodianAshish Kirodian
865
865
i am using redux-thunk. Please explain me How can i call a Redux action from my React frontend and then got the returned state?
– user10298495
Nov 13 '18 at 16:08
I am seeing "<Provider store={store}>" in the todo example. what is Provider and do i need to use it in react?
– user10298495
Nov 13 '18 at 16:14
@Rajat Provider infuses the store to your component at root level. This is Composition. I think you need to go through react documents properly. it is well explained there
– Ashish Kirodian
Nov 13 '18 at 16:17
Can you please edit your above code, where you used the redux thunk? As you didn't import it and tell how to use it with store. Thanks.
– user10298495
Nov 13 '18 at 16:30
@Rajat check the first line of code in my answer. middleware is assigned when you create store. which is done at index.js
– Ashish Kirodian
Nov 13 '18 at 16:32
|
show 6 more comments
i am using redux-thunk. Please explain me How can i call a Redux action from my React frontend and then got the returned state?
– user10298495
Nov 13 '18 at 16:08
I am seeing "<Provider store={store}>" in the todo example. what is Provider and do i need to use it in react?
– user10298495
Nov 13 '18 at 16:14
@Rajat Provider infuses the store to your component at root level. This is Composition. I think you need to go through react documents properly. it is well explained there
– Ashish Kirodian
Nov 13 '18 at 16:17
Can you please edit your above code, where you used the redux thunk? As you didn't import it and tell how to use it with store. Thanks.
– user10298495
Nov 13 '18 at 16:30
@Rajat check the first line of code in my answer. middleware is assigned when you create store. which is done at index.js
– Ashish Kirodian
Nov 13 '18 at 16:32
i am using redux-thunk. Please explain me How can i call a Redux action from my React frontend and then got the returned state?
– user10298495
Nov 13 '18 at 16:08
i am using redux-thunk. Please explain me How can i call a Redux action from my React frontend and then got the returned state?
– user10298495
Nov 13 '18 at 16:08
I am seeing "<Provider store={store}>" in the todo example. what is Provider and do i need to use it in react?
– user10298495
Nov 13 '18 at 16:14
I am seeing "<Provider store={store}>" in the todo example. what is Provider and do i need to use it in react?
– user10298495
Nov 13 '18 at 16:14
@Rajat Provider infuses the store to your component at root level. This is Composition. I think you need to go through react documents properly. it is well explained there
– Ashish Kirodian
Nov 13 '18 at 16:17
@Rajat Provider infuses the store to your component at root level. This is Composition. I think you need to go through react documents properly. it is well explained there
– Ashish Kirodian
Nov 13 '18 at 16:17
Can you please edit your above code, where you used the redux thunk? As you didn't import it and tell how to use it with store. Thanks.
– user10298495
Nov 13 '18 at 16:30
Can you please edit your above code, where you used the redux thunk? As you didn't import it and tell how to use it with store. Thanks.
– user10298495
Nov 13 '18 at 16:30
@Rajat check the first line of code in my answer. middleware is assigned when you create store. which is done at index.js
– Ashish Kirodian
Nov 13 '18 at 16:32
@Rajat check the first line of code in my answer. middleware is assigned when you create store. which is done at index.js
– Ashish Kirodian
Nov 13 '18 at 16:32
|
show 6 more comments
As Mustafa said you need to use mapStateToProps
. Let me explain myself.
What you just done is just the configuration for the main store (there's only one in redux). Now you need to use it in your components, but how ? When you create a Component the content of the store
will be passed as props
with the help of Containers
.
Containers are the way to link your store with your react component.
Said that, you need to install redux
and react-redux
. In your code above you have successfully configured the store with the reducers with redux
library. Now you need react-redux
to create the Container (which wraps your react component).
Here is an example of how to put this all together:
https://codepen.io/anon/pen/RqKyQZ?editors=1010
Thanks for your answer. But can you please tell me how can i?
– user10298495
Nov 13 '18 at 16:17
can you please share any refrence with me. But please don't share docs link as they are lengthy to read.
– user10298495
Nov 13 '18 at 16:19
i will provide an example, give me a minute.
– giwiro
Nov 13 '18 at 16:19
sure, please do.
– user10298495
Nov 13 '18 at 16:20
done: codepen.io/anon/pen/RqKyQZ?editors=1010 If you found it useful like it ^^
– giwiro
Nov 13 '18 at 16:38
|
show 6 more comments
As Mustafa said you need to use mapStateToProps
. Let me explain myself.
What you just done is just the configuration for the main store (there's only one in redux). Now you need to use it in your components, but how ? When you create a Component the content of the store
will be passed as props
with the help of Containers
.
Containers are the way to link your store with your react component.
Said that, you need to install redux
and react-redux
. In your code above you have successfully configured the store with the reducers with redux
library. Now you need react-redux
to create the Container (which wraps your react component).
Here is an example of how to put this all together:
https://codepen.io/anon/pen/RqKyQZ?editors=1010
Thanks for your answer. But can you please tell me how can i?
– user10298495
Nov 13 '18 at 16:17
can you please share any refrence with me. But please don't share docs link as they are lengthy to read.
– user10298495
Nov 13 '18 at 16:19
i will provide an example, give me a minute.
– giwiro
Nov 13 '18 at 16:19
sure, please do.
– user10298495
Nov 13 '18 at 16:20
done: codepen.io/anon/pen/RqKyQZ?editors=1010 If you found it useful like it ^^
– giwiro
Nov 13 '18 at 16:38
|
show 6 more comments
As Mustafa said you need to use mapStateToProps
. Let me explain myself.
What you just done is just the configuration for the main store (there's only one in redux). Now you need to use it in your components, but how ? When you create a Component the content of the store
will be passed as props
with the help of Containers
.
Containers are the way to link your store with your react component.
Said that, you need to install redux
and react-redux
. In your code above you have successfully configured the store with the reducers with redux
library. Now you need react-redux
to create the Container (which wraps your react component).
Here is an example of how to put this all together:
https://codepen.io/anon/pen/RqKyQZ?editors=1010
As Mustafa said you need to use mapStateToProps
. Let me explain myself.
What you just done is just the configuration for the main store (there's only one in redux). Now you need to use it in your components, but how ? When you create a Component the content of the store
will be passed as props
with the help of Containers
.
Containers are the way to link your store with your react component.
Said that, you need to install redux
and react-redux
. In your code above you have successfully configured the store with the reducers with redux
library. Now you need react-redux
to create the Container (which wraps your react component).
Here is an example of how to put this all together:
https://codepen.io/anon/pen/RqKyQZ?editors=1010
edited Nov 13 '18 at 16:38
answered Nov 13 '18 at 16:15
giwirogiwiro
13616
13616
Thanks for your answer. But can you please tell me how can i?
– user10298495
Nov 13 '18 at 16:17
can you please share any refrence with me. But please don't share docs link as they are lengthy to read.
– user10298495
Nov 13 '18 at 16:19
i will provide an example, give me a minute.
– giwiro
Nov 13 '18 at 16:19
sure, please do.
– user10298495
Nov 13 '18 at 16:20
done: codepen.io/anon/pen/RqKyQZ?editors=1010 If you found it useful like it ^^
– giwiro
Nov 13 '18 at 16:38
|
show 6 more comments
Thanks for your answer. But can you please tell me how can i?
– user10298495
Nov 13 '18 at 16:17
can you please share any refrence with me. But please don't share docs link as they are lengthy to read.
– user10298495
Nov 13 '18 at 16:19
i will provide an example, give me a minute.
– giwiro
Nov 13 '18 at 16:19
sure, please do.
– user10298495
Nov 13 '18 at 16:20
done: codepen.io/anon/pen/RqKyQZ?editors=1010 If you found it useful like it ^^
– giwiro
Nov 13 '18 at 16:38
Thanks for your answer. But can you please tell me how can i?
– user10298495
Nov 13 '18 at 16:17
Thanks for your answer. But can you please tell me how can i?
– user10298495
Nov 13 '18 at 16:17
can you please share any refrence with me. But please don't share docs link as they are lengthy to read.
– user10298495
Nov 13 '18 at 16:19
can you please share any refrence with me. But please don't share docs link as they are lengthy to read.
– user10298495
Nov 13 '18 at 16:19
i will provide an example, give me a minute.
– giwiro
Nov 13 '18 at 16:19
i will provide an example, give me a minute.
– giwiro
Nov 13 '18 at 16:19
sure, please do.
– user10298495
Nov 13 '18 at 16:20
sure, please do.
– user10298495
Nov 13 '18 at 16:20
done: codepen.io/anon/pen/RqKyQZ?editors=1010 If you found it useful like it ^^
– giwiro
Nov 13 '18 at 16:38
done: codepen.io/anon/pen/RqKyQZ?editors=1010 If you found it useful like it ^^
– giwiro
Nov 13 '18 at 16:38
|
show 6 more comments
You need to use mapStateToProps similar to the code below. Let say your reducer is called test and it is part of a state.
const mapStateToProps = (state, props) =>
({
router: props.router,
test: state.test
});
Then test
will be used as a property in a React class. Obviously you need to include respective imports for React.
add a comment |
You need to use mapStateToProps similar to the code below. Let say your reducer is called test and it is part of a state.
const mapStateToProps = (state, props) =>
({
router: props.router,
test: state.test
});
Then test
will be used as a property in a React class. Obviously you need to include respective imports for React.
add a comment |
You need to use mapStateToProps similar to the code below. Let say your reducer is called test and it is part of a state.
const mapStateToProps = (state, props) =>
({
router: props.router,
test: state.test
});
Then test
will be used as a property in a React class. Obviously you need to include respective imports for React.
You need to use mapStateToProps similar to the code below. Let say your reducer is called test and it is part of a state.
const mapStateToProps = (state, props) =>
({
router: props.router,
test: state.test
});
Then test
will be used as a property in a React class. Obviously you need to include respective imports for React.
answered Nov 13 '18 at 17:07
Kanan FarzaliKanan Farzali
322320
322320
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53284689%2fhow-to-use-redux-with-react%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cQuhoK1bf5BikApJu4994N4Lo,rBIrf9mFLqT eYtuBJi Wma4cayfUWO,BOyxC,ccRrX,Klo8DpntOMy5e uuZWIQ
3
redux.js.org/basics/usagewithreact
– AndyJ
Nov 13 '18 at 16:01
I don't want the documentation link. Can you explain me in shorten?
– user10298495
Nov 13 '18 at 16:03
@Rajat That is just being lazy
– Boy With Silver Wings
Nov 13 '18 at 16:11
1
@Rajat Just sit down and read about Redux and react-redux (connector between react and redux) first. Docs are actually meant for that. SO is meant for situations when you get stuck programmatically doing something beyond docs maybe
– kushalvm
Nov 13 '18 at 16:22