Importing data using SQLBulkCopy
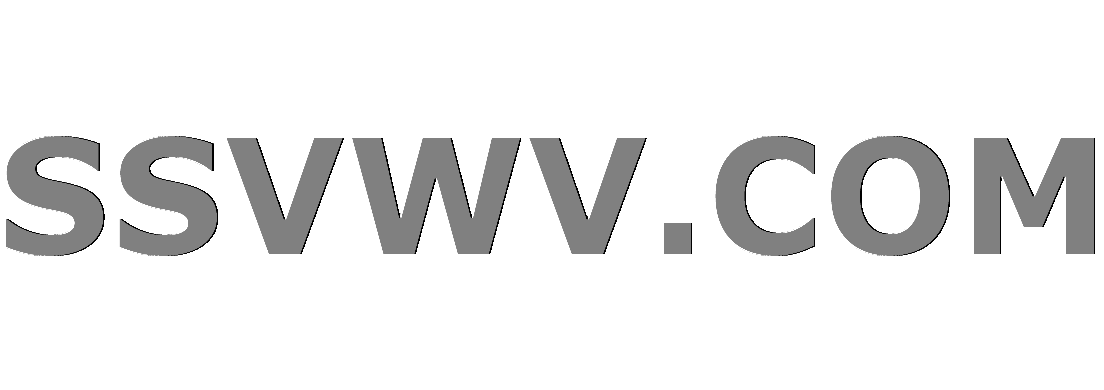
Multi tool use
We have a legacy application that is dumping large volumes of data to tab delimited files. Each file contains a single record type and all fields are fixed length.
These files can readily be imported into corresponding tables in our SQL server database using the BCP utility from the command line.
We have a VB.Net program written in VS 2003 that imports these files using the SQLDMO.BulkCopy routine.
We are updating the system to use VS 2010 with SQL Server 2008 and according to the Microsoft documentation SQLDMO is no longer available.
I have searched on the internet and have rewritten the import routine to import the tab delimited files into a DataTable using the Microsoft.Jet.OLEDB.4.0 provider. The SqlClient.BulkCopy object is then used to import this DataTable. This issue I am having is that fields in the tab delimited file that are set to spaces are being treating as NULLs when imported into the DataTable. When the DataTable is processed by the SqlClient.BulkCopy the copy fails because the null values are rejected by the SQL table fields that are defined as NOT NULL.
Code being tested is shown below
Private Sub Button1_Click(sender As System.Object, e As System.EventArgs) Handles Button1.Click
Dim data As DataTable = RetrieveSourceData()
CopyData(data)
End Sub
Private Function RetrieveSourceData() As DataTable
Dim connstring As String = "Provider=Microsoft.Jet.OLEDB.4.0; Data Source=C:temp;Extended Properties='text;HDR=No;FMT=TabDelimited'"
Dim sourcedata As New DataTable
Using conn As New OleDb.OleDbConnection(connstring)
conn.Open()
Dim command As New OleDb.OleDbCommand("Select * from X1.CSV", conn)
Dim adapter As New OleDb.OleDbDataAdapter(command)
adapter.Fill(sourcedata)
conn.Close()
End Using
Return sourcedata
End Function
Private Sub CopyData(SourceData As DataTable)
Dim dbConnString As String = "Data Source=(local);Initial Catalog=XtractDB;User ID=xxxx;Password=yyyy;"
Using bcp As New SqlClient.SqlBulkCopy(dbConnString)
bcp.DestinationTableName = "X1"
bcp.BatchSize = 1000
bcp.WriteToServer(SourceData)
End Using
End Sub
In order for the input file to be recognised as TabDelimited I have had to create a schema.ini file in the same directory as the input file. The contents are shown below
[X1.CSV]
Format=TabDelimited
Is there any way I can force the fields with spaces not to be treated as NULL when creating the DataTable?
Is this the best approach for processing the Bulk Copy via a VB.Net program?
TIA,
Andy
Save to: Default
Switch color theme
Select message background color...
Select message area width...
Adjust message text font size...
Disable auto links
Enable acronyms
Disable message header
Enable auto quote
Update the title of this thread...
SQL Bulk Copy Thread #1544244 Message #1544244
vb.net sqlbulkcopy
add a comment |
We have a legacy application that is dumping large volumes of data to tab delimited files. Each file contains a single record type and all fields are fixed length.
These files can readily be imported into corresponding tables in our SQL server database using the BCP utility from the command line.
We have a VB.Net program written in VS 2003 that imports these files using the SQLDMO.BulkCopy routine.
We are updating the system to use VS 2010 with SQL Server 2008 and according to the Microsoft documentation SQLDMO is no longer available.
I have searched on the internet and have rewritten the import routine to import the tab delimited files into a DataTable using the Microsoft.Jet.OLEDB.4.0 provider. The SqlClient.BulkCopy object is then used to import this DataTable. This issue I am having is that fields in the tab delimited file that are set to spaces are being treating as NULLs when imported into the DataTable. When the DataTable is processed by the SqlClient.BulkCopy the copy fails because the null values are rejected by the SQL table fields that are defined as NOT NULL.
Code being tested is shown below
Private Sub Button1_Click(sender As System.Object, e As System.EventArgs) Handles Button1.Click
Dim data As DataTable = RetrieveSourceData()
CopyData(data)
End Sub
Private Function RetrieveSourceData() As DataTable
Dim connstring As String = "Provider=Microsoft.Jet.OLEDB.4.0; Data Source=C:temp;Extended Properties='text;HDR=No;FMT=TabDelimited'"
Dim sourcedata As New DataTable
Using conn As New OleDb.OleDbConnection(connstring)
conn.Open()
Dim command As New OleDb.OleDbCommand("Select * from X1.CSV", conn)
Dim adapter As New OleDb.OleDbDataAdapter(command)
adapter.Fill(sourcedata)
conn.Close()
End Using
Return sourcedata
End Function
Private Sub CopyData(SourceData As DataTable)
Dim dbConnString As String = "Data Source=(local);Initial Catalog=XtractDB;User ID=xxxx;Password=yyyy;"
Using bcp As New SqlClient.SqlBulkCopy(dbConnString)
bcp.DestinationTableName = "X1"
bcp.BatchSize = 1000
bcp.WriteToServer(SourceData)
End Using
End Sub
In order for the input file to be recognised as TabDelimited I have had to create a schema.ini file in the same directory as the input file. The contents are shown below
[X1.CSV]
Format=TabDelimited
Is there any way I can force the fields with spaces not to be treated as NULL when creating the DataTable?
Is this the best approach for processing the Bulk Copy via a VB.Net program?
TIA,
Andy
Save to: Default
Switch color theme
Select message background color...
Select message area width...
Adjust message text font size...
Disable auto links
Enable acronyms
Disable message header
Enable auto quote
Update the title of this thread...
SQL Bulk Copy Thread #1544244 Message #1544244
vb.net sqlbulkcopy
I have managed to get this working as intended by loading the DataTable in RetrieveSourceData using a stream reader rather than by using OleDb.
– Andy
May 25 '12 at 7:51
add a comment |
We have a legacy application that is dumping large volumes of data to tab delimited files. Each file contains a single record type and all fields are fixed length.
These files can readily be imported into corresponding tables in our SQL server database using the BCP utility from the command line.
We have a VB.Net program written in VS 2003 that imports these files using the SQLDMO.BulkCopy routine.
We are updating the system to use VS 2010 with SQL Server 2008 and according to the Microsoft documentation SQLDMO is no longer available.
I have searched on the internet and have rewritten the import routine to import the tab delimited files into a DataTable using the Microsoft.Jet.OLEDB.4.0 provider. The SqlClient.BulkCopy object is then used to import this DataTable. This issue I am having is that fields in the tab delimited file that are set to spaces are being treating as NULLs when imported into the DataTable. When the DataTable is processed by the SqlClient.BulkCopy the copy fails because the null values are rejected by the SQL table fields that are defined as NOT NULL.
Code being tested is shown below
Private Sub Button1_Click(sender As System.Object, e As System.EventArgs) Handles Button1.Click
Dim data As DataTable = RetrieveSourceData()
CopyData(data)
End Sub
Private Function RetrieveSourceData() As DataTable
Dim connstring As String = "Provider=Microsoft.Jet.OLEDB.4.0; Data Source=C:temp;Extended Properties='text;HDR=No;FMT=TabDelimited'"
Dim sourcedata As New DataTable
Using conn As New OleDb.OleDbConnection(connstring)
conn.Open()
Dim command As New OleDb.OleDbCommand("Select * from X1.CSV", conn)
Dim adapter As New OleDb.OleDbDataAdapter(command)
adapter.Fill(sourcedata)
conn.Close()
End Using
Return sourcedata
End Function
Private Sub CopyData(SourceData As DataTable)
Dim dbConnString As String = "Data Source=(local);Initial Catalog=XtractDB;User ID=xxxx;Password=yyyy;"
Using bcp As New SqlClient.SqlBulkCopy(dbConnString)
bcp.DestinationTableName = "X1"
bcp.BatchSize = 1000
bcp.WriteToServer(SourceData)
End Using
End Sub
In order for the input file to be recognised as TabDelimited I have had to create a schema.ini file in the same directory as the input file. The contents are shown below
[X1.CSV]
Format=TabDelimited
Is there any way I can force the fields with spaces not to be treated as NULL when creating the DataTable?
Is this the best approach for processing the Bulk Copy via a VB.Net program?
TIA,
Andy
Save to: Default
Switch color theme
Select message background color...
Select message area width...
Adjust message text font size...
Disable auto links
Enable acronyms
Disable message header
Enable auto quote
Update the title of this thread...
SQL Bulk Copy Thread #1544244 Message #1544244
vb.net sqlbulkcopy
We have a legacy application that is dumping large volumes of data to tab delimited files. Each file contains a single record type and all fields are fixed length.
These files can readily be imported into corresponding tables in our SQL server database using the BCP utility from the command line.
We have a VB.Net program written in VS 2003 that imports these files using the SQLDMO.BulkCopy routine.
We are updating the system to use VS 2010 with SQL Server 2008 and according to the Microsoft documentation SQLDMO is no longer available.
I have searched on the internet and have rewritten the import routine to import the tab delimited files into a DataTable using the Microsoft.Jet.OLEDB.4.0 provider. The SqlClient.BulkCopy object is then used to import this DataTable. This issue I am having is that fields in the tab delimited file that are set to spaces are being treating as NULLs when imported into the DataTable. When the DataTable is processed by the SqlClient.BulkCopy the copy fails because the null values are rejected by the SQL table fields that are defined as NOT NULL.
Code being tested is shown below
Private Sub Button1_Click(sender As System.Object, e As System.EventArgs) Handles Button1.Click
Dim data As DataTable = RetrieveSourceData()
CopyData(data)
End Sub
Private Function RetrieveSourceData() As DataTable
Dim connstring As String = "Provider=Microsoft.Jet.OLEDB.4.0; Data Source=C:temp;Extended Properties='text;HDR=No;FMT=TabDelimited'"
Dim sourcedata As New DataTable
Using conn As New OleDb.OleDbConnection(connstring)
conn.Open()
Dim command As New OleDb.OleDbCommand("Select * from X1.CSV", conn)
Dim adapter As New OleDb.OleDbDataAdapter(command)
adapter.Fill(sourcedata)
conn.Close()
End Using
Return sourcedata
End Function
Private Sub CopyData(SourceData As DataTable)
Dim dbConnString As String = "Data Source=(local);Initial Catalog=XtractDB;User ID=xxxx;Password=yyyy;"
Using bcp As New SqlClient.SqlBulkCopy(dbConnString)
bcp.DestinationTableName = "X1"
bcp.BatchSize = 1000
bcp.WriteToServer(SourceData)
End Using
End Sub
In order for the input file to be recognised as TabDelimited I have had to create a schema.ini file in the same directory as the input file. The contents are shown below
[X1.CSV]
Format=TabDelimited
Is there any way I can force the fields with spaces not to be treated as NULL when creating the DataTable?
Is this the best approach for processing the Bulk Copy via a VB.Net program?
TIA,
Andy
Save to: Default
Switch color theme
Select message background color...
Select message area width...
Adjust message text font size...
Disable auto links
Enable acronyms
Disable message header
Enable auto quote
Update the title of this thread...
SQL Bulk Copy Thread #1544244 Message #1544244
vb.net sqlbulkcopy
vb.net sqlbulkcopy
asked May 25 '12 at 7:12
AndyAndy
612
612
I have managed to get this working as intended by loading the DataTable in RetrieveSourceData using a stream reader rather than by using OleDb.
– Andy
May 25 '12 at 7:51
add a comment |
I have managed to get this working as intended by loading the DataTable in RetrieveSourceData using a stream reader rather than by using OleDb.
– Andy
May 25 '12 at 7:51
I have managed to get this working as intended by loading the DataTable in RetrieveSourceData using a stream reader rather than by using OleDb.
– Andy
May 25 '12 at 7:51
I have managed to get this working as intended by loading the DataTable in RetrieveSourceData using a stream reader rather than by using OleDb.
– Andy
May 25 '12 at 7:51
add a comment |
1 Answer
1
active
oldest
votes
SqlDMO is deprecated in Sql server 2012 but it's available till Sqlserver 2008 R2 version.
SqlBulkCopy is available in all .net frameworks till 4.5 except 1.1.
Refer this link for verification
You can use BULK INSERT (command line utility) to insert multiple records at once.
BULK
INSERT Tablename
FROM 'c:csvtest.txt'
WITH
(
FIELDTERMINATOR = ','
,ROWTERMINATOR = 'n'
--,FIRSTROW = 2
--,MAXERRORS = 0
)
GO
Thanks Romil - want to use vb.net routine as part of a larger process that provides logging and scheduling services. Also want to avoid SqlDmo if will be deprecated.
– Andy
May 25 '12 at 7:53
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f10750043%2fimporting-data-using-sqlbulkcopy%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
SqlDMO is deprecated in Sql server 2012 but it's available till Sqlserver 2008 R2 version.
SqlBulkCopy is available in all .net frameworks till 4.5 except 1.1.
Refer this link for verification
You can use BULK INSERT (command line utility) to insert multiple records at once.
BULK
INSERT Tablename
FROM 'c:csvtest.txt'
WITH
(
FIELDTERMINATOR = ','
,ROWTERMINATOR = 'n'
--,FIRSTROW = 2
--,MAXERRORS = 0
)
GO
Thanks Romil - want to use vb.net routine as part of a larger process that provides logging and scheduling services. Also want to avoid SqlDmo if will be deprecated.
– Andy
May 25 '12 at 7:53
add a comment |
SqlDMO is deprecated in Sql server 2012 but it's available till Sqlserver 2008 R2 version.
SqlBulkCopy is available in all .net frameworks till 4.5 except 1.1.
Refer this link for verification
You can use BULK INSERT (command line utility) to insert multiple records at once.
BULK
INSERT Tablename
FROM 'c:csvtest.txt'
WITH
(
FIELDTERMINATOR = ','
,ROWTERMINATOR = 'n'
--,FIRSTROW = 2
--,MAXERRORS = 0
)
GO
Thanks Romil - want to use vb.net routine as part of a larger process that provides logging and scheduling services. Also want to avoid SqlDmo if will be deprecated.
– Andy
May 25 '12 at 7:53
add a comment |
SqlDMO is deprecated in Sql server 2012 but it's available till Sqlserver 2008 R2 version.
SqlBulkCopy is available in all .net frameworks till 4.5 except 1.1.
Refer this link for verification
You can use BULK INSERT (command line utility) to insert multiple records at once.
BULK
INSERT Tablename
FROM 'c:csvtest.txt'
WITH
(
FIELDTERMINATOR = ','
,ROWTERMINATOR = 'n'
--,FIRSTROW = 2
--,MAXERRORS = 0
)
GO
SqlDMO is deprecated in Sql server 2012 but it's available till Sqlserver 2008 R2 version.
SqlBulkCopy is available in all .net frameworks till 4.5 except 1.1.
Refer this link for verification
You can use BULK INSERT (command line utility) to insert multiple records at once.
BULK
INSERT Tablename
FROM 'c:csvtest.txt'
WITH
(
FIELDTERMINATOR = ','
,ROWTERMINATOR = 'n'
--,FIRSTROW = 2
--,MAXERRORS = 0
)
GO
edited May 25 '12 at 7:26
answered May 25 '12 at 7:19
Romil Kumar JainRomil Kumar Jain
16k73977
16k73977
Thanks Romil - want to use vb.net routine as part of a larger process that provides logging and scheduling services. Also want to avoid SqlDmo if will be deprecated.
– Andy
May 25 '12 at 7:53
add a comment |
Thanks Romil - want to use vb.net routine as part of a larger process that provides logging and scheduling services. Also want to avoid SqlDmo if will be deprecated.
– Andy
May 25 '12 at 7:53
Thanks Romil - want to use vb.net routine as part of a larger process that provides logging and scheduling services. Also want to avoid SqlDmo if will be deprecated.
– Andy
May 25 '12 at 7:53
Thanks Romil - want to use vb.net routine as part of a larger process that provides logging and scheduling services. Also want to avoid SqlDmo if will be deprecated.
– Andy
May 25 '12 at 7:53
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f10750043%2fimporting-data-using-sqlbulkcopy%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zlegv BNh7fsZVC5qAUVWRiRivI1BBK2y,Z1 if
I have managed to get this working as intended by loading the DataTable in RetrieveSourceData using a stream reader rather than by using OleDb.
– Andy
May 25 '12 at 7:51