D3 slider with ordinal values gets stuck on tick 8
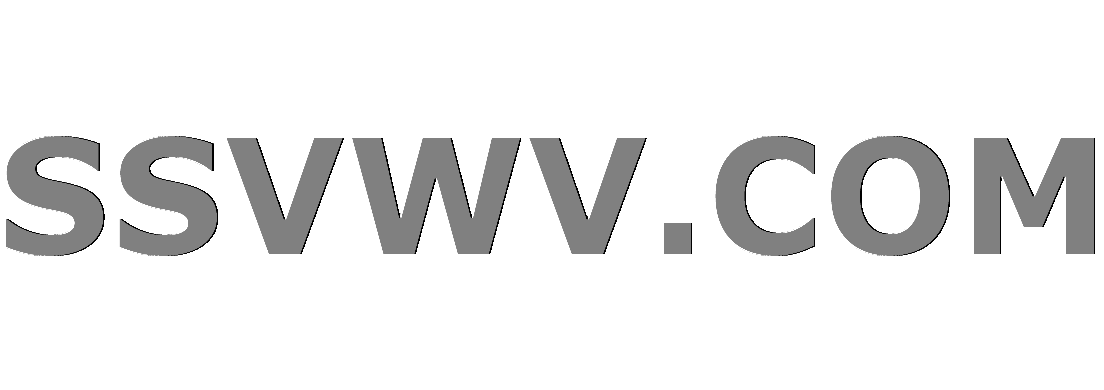
Multi tool use
I am trying to implement a D3 slider with ordinal values for each tick.
I have used the following slider implementation:
https://github.com/MasterMaps/d3-slider
https://codepen.io/DougManuel/full/avRyMg
Here is my .js (myslider.js) code with the slider implementation:
var data2 = ['18:00','18:15','18:30','18:45','19:00','19:15','19:30','19:45','20:00','20:15','20:30','20:45','21:00','21:15','21:30','21:45','22:00','22:15','22:30','22:45'];
var w = window.innerWidth || document.body.clientWidth,
h = 700;
drawSlider();
function drawSlider() {
svg = d3.select("body").append("svg:svg")
.attr("width", w)
.attr("height", h);
svg.append('text')
.attr('class','timeband')
.attr("x", w/2)
.attr("y", h/2)
.attr('text-anchor','middle')
.attr('font-family','sans-serif')
.attr('font-weight','bold')
.attr('font-size','50px')
.attr('fill','black')
.text(data2[0]);
d3.select('#slider2').call(
d3.slider()
.scale(d3.scale.ordinal().domain(data2).rangePoints([0, 1],0.5))
.axis(d3.svg.axis())
.snap(true)
.value(data2[0])
.on("slideend", function(evt, value) {
svg.selectAll("text.timeband")
.text(value)
})
);
}
Here is my html code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
</head>
<body>
<div id="slider2" style="margin-bottom:30px;"></div>
<script src="d3/d3.js"></script> <!--D3 Version 3-->
<script type="text/javascript" src="d3/slider.js"></script>
<!--<script type="text/javascript" src="https://github.com/MasterMaps/d3-slider/blob/master/d3.slider.js"></script>-->
<link rel="stylesheet" href="css/d3.slider.css" />
<!--<link rel="stylesheet" href="https://github.com/MasterMaps/d3-slider/blob/master/d3.slider.css"/>-->
<script type="text/javascript" src="js/myslider.js"></script>
</body>
My slider does not move beyond tick no 8 with the value '19:45'. I think it is dependent on the configuration of the rangePoints function of the scale. I have tried multiple combinations but was not able to figure out the optimal setting.
javascript d3.js slider
add a comment |
I am trying to implement a D3 slider with ordinal values for each tick.
I have used the following slider implementation:
https://github.com/MasterMaps/d3-slider
https://codepen.io/DougManuel/full/avRyMg
Here is my .js (myslider.js) code with the slider implementation:
var data2 = ['18:00','18:15','18:30','18:45','19:00','19:15','19:30','19:45','20:00','20:15','20:30','20:45','21:00','21:15','21:30','21:45','22:00','22:15','22:30','22:45'];
var w = window.innerWidth || document.body.clientWidth,
h = 700;
drawSlider();
function drawSlider() {
svg = d3.select("body").append("svg:svg")
.attr("width", w)
.attr("height", h);
svg.append('text')
.attr('class','timeband')
.attr("x", w/2)
.attr("y", h/2)
.attr('text-anchor','middle')
.attr('font-family','sans-serif')
.attr('font-weight','bold')
.attr('font-size','50px')
.attr('fill','black')
.text(data2[0]);
d3.select('#slider2').call(
d3.slider()
.scale(d3.scale.ordinal().domain(data2).rangePoints([0, 1],0.5))
.axis(d3.svg.axis())
.snap(true)
.value(data2[0])
.on("slideend", function(evt, value) {
svg.selectAll("text.timeband")
.text(value)
})
);
}
Here is my html code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
</head>
<body>
<div id="slider2" style="margin-bottom:30px;"></div>
<script src="d3/d3.js"></script> <!--D3 Version 3-->
<script type="text/javascript" src="d3/slider.js"></script>
<!--<script type="text/javascript" src="https://github.com/MasterMaps/d3-slider/blob/master/d3.slider.js"></script>-->
<link rel="stylesheet" href="css/d3.slider.css" />
<!--<link rel="stylesheet" href="https://github.com/MasterMaps/d3-slider/blob/master/d3.slider.css"/>-->
<script type="text/javascript" src="js/myslider.js"></script>
</body>
My slider does not move beyond tick no 8 with the value '19:45'. I think it is dependent on the configuration of the rangePoints function of the scale. I have tried multiple combinations but was not able to figure out the optimal setting.
javascript d3.js slider
add a comment |
I am trying to implement a D3 slider with ordinal values for each tick.
I have used the following slider implementation:
https://github.com/MasterMaps/d3-slider
https://codepen.io/DougManuel/full/avRyMg
Here is my .js (myslider.js) code with the slider implementation:
var data2 = ['18:00','18:15','18:30','18:45','19:00','19:15','19:30','19:45','20:00','20:15','20:30','20:45','21:00','21:15','21:30','21:45','22:00','22:15','22:30','22:45'];
var w = window.innerWidth || document.body.clientWidth,
h = 700;
drawSlider();
function drawSlider() {
svg = d3.select("body").append("svg:svg")
.attr("width", w)
.attr("height", h);
svg.append('text')
.attr('class','timeband')
.attr("x", w/2)
.attr("y", h/2)
.attr('text-anchor','middle')
.attr('font-family','sans-serif')
.attr('font-weight','bold')
.attr('font-size','50px')
.attr('fill','black')
.text(data2[0]);
d3.select('#slider2').call(
d3.slider()
.scale(d3.scale.ordinal().domain(data2).rangePoints([0, 1],0.5))
.axis(d3.svg.axis())
.snap(true)
.value(data2[0])
.on("slideend", function(evt, value) {
svg.selectAll("text.timeband")
.text(value)
})
);
}
Here is my html code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
</head>
<body>
<div id="slider2" style="margin-bottom:30px;"></div>
<script src="d3/d3.js"></script> <!--D3 Version 3-->
<script type="text/javascript" src="d3/slider.js"></script>
<!--<script type="text/javascript" src="https://github.com/MasterMaps/d3-slider/blob/master/d3.slider.js"></script>-->
<link rel="stylesheet" href="css/d3.slider.css" />
<!--<link rel="stylesheet" href="https://github.com/MasterMaps/d3-slider/blob/master/d3.slider.css"/>-->
<script type="text/javascript" src="js/myslider.js"></script>
</body>
My slider does not move beyond tick no 8 with the value '19:45'. I think it is dependent on the configuration of the rangePoints function of the scale. I have tried multiple combinations but was not able to figure out the optimal setting.
javascript d3.js slider
I am trying to implement a D3 slider with ordinal values for each tick.
I have used the following slider implementation:
https://github.com/MasterMaps/d3-slider
https://codepen.io/DougManuel/full/avRyMg
Here is my .js (myslider.js) code with the slider implementation:
var data2 = ['18:00','18:15','18:30','18:45','19:00','19:15','19:30','19:45','20:00','20:15','20:30','20:45','21:00','21:15','21:30','21:45','22:00','22:15','22:30','22:45'];
var w = window.innerWidth || document.body.clientWidth,
h = 700;
drawSlider();
function drawSlider() {
svg = d3.select("body").append("svg:svg")
.attr("width", w)
.attr("height", h);
svg.append('text')
.attr('class','timeband')
.attr("x", w/2)
.attr("y", h/2)
.attr('text-anchor','middle')
.attr('font-family','sans-serif')
.attr('font-weight','bold')
.attr('font-size','50px')
.attr('fill','black')
.text(data2[0]);
d3.select('#slider2').call(
d3.slider()
.scale(d3.scale.ordinal().domain(data2).rangePoints([0, 1],0.5))
.axis(d3.svg.axis())
.snap(true)
.value(data2[0])
.on("slideend", function(evt, value) {
svg.selectAll("text.timeband")
.text(value)
})
);
}
Here is my html code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
</head>
<body>
<div id="slider2" style="margin-bottom:30px;"></div>
<script src="d3/d3.js"></script> <!--D3 Version 3-->
<script type="text/javascript" src="d3/slider.js"></script>
<!--<script type="text/javascript" src="https://github.com/MasterMaps/d3-slider/blob/master/d3.slider.js"></script>-->
<link rel="stylesheet" href="css/d3.slider.css" />
<!--<link rel="stylesheet" href="https://github.com/MasterMaps/d3-slider/blob/master/d3.slider.css"/>-->
<script type="text/javascript" src="js/myslider.js"></script>
</body>
My slider does not move beyond tick no 8 with the value '19:45'. I think it is dependent on the configuration of the rangePoints function of the scale. I have tried multiple combinations but was not able to figure out the optimal setting.
javascript d3.js slider
javascript d3.js slider
asked Nov 12 '18 at 11:04


edwig noronhaedwig noronha
85
85
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
There is a bug in d3-slider if the stops can be indexed (strings) (line [281]).
if ( value[ 0 ] >= value[ 1 ] ) return;
This line is there to prevent that range sliders value[0]
is larger then value[1]
. For non-range sliders with string ticks this depends on what string is the current value.
If the string is 20:00
the condition is true and so no animation/positioning of the slider.
Change the line to
if (toType(value) == "array" && value.length == 2 && value[ 0 ] >= value[ 1 ] ) return;
to make the test only valid for range sliders.
this works perfectly. Thank you.
– edwig noronha
Nov 12 '18 at 17:24
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53260825%2fd3-slider-with-ordinal-values-gets-stuck-on-tick-8%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is a bug in d3-slider if the stops can be indexed (strings) (line [281]).
if ( value[ 0 ] >= value[ 1 ] ) return;
This line is there to prevent that range sliders value[0]
is larger then value[1]
. For non-range sliders with string ticks this depends on what string is the current value.
If the string is 20:00
the condition is true and so no animation/positioning of the slider.
Change the line to
if (toType(value) == "array" && value.length == 2 && value[ 0 ] >= value[ 1 ] ) return;
to make the test only valid for range sliders.
this works perfectly. Thank you.
– edwig noronha
Nov 12 '18 at 17:24
add a comment |
There is a bug in d3-slider if the stops can be indexed (strings) (line [281]).
if ( value[ 0 ] >= value[ 1 ] ) return;
This line is there to prevent that range sliders value[0]
is larger then value[1]
. For non-range sliders with string ticks this depends on what string is the current value.
If the string is 20:00
the condition is true and so no animation/positioning of the slider.
Change the line to
if (toType(value) == "array" && value.length == 2 && value[ 0 ] >= value[ 1 ] ) return;
to make the test only valid for range sliders.
this works perfectly. Thank you.
– edwig noronha
Nov 12 '18 at 17:24
add a comment |
There is a bug in d3-slider if the stops can be indexed (strings) (line [281]).
if ( value[ 0 ] >= value[ 1 ] ) return;
This line is there to prevent that range sliders value[0]
is larger then value[1]
. For non-range sliders with string ticks this depends on what string is the current value.
If the string is 20:00
the condition is true and so no animation/positioning of the slider.
Change the line to
if (toType(value) == "array" && value.length == 2 && value[ 0 ] >= value[ 1 ] ) return;
to make the test only valid for range sliders.
There is a bug in d3-slider if the stops can be indexed (strings) (line [281]).
if ( value[ 0 ] >= value[ 1 ] ) return;
This line is there to prevent that range sliders value[0]
is larger then value[1]
. For non-range sliders with string ticks this depends on what string is the current value.
If the string is 20:00
the condition is true and so no animation/positioning of the slider.
Change the line to
if (toType(value) == "array" && value.length == 2 && value[ 0 ] >= value[ 1 ] ) return;
to make the test only valid for range sliders.
answered Nov 12 '18 at 13:38
rioV8rioV8
4,1802311
4,1802311
this works perfectly. Thank you.
– edwig noronha
Nov 12 '18 at 17:24
add a comment |
this works perfectly. Thank you.
– edwig noronha
Nov 12 '18 at 17:24
this works perfectly. Thank you.
– edwig noronha
Nov 12 '18 at 17:24
this works perfectly. Thank you.
– edwig noronha
Nov 12 '18 at 17:24
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53260825%2fd3-slider-with-ordinal-values-gets-stuck-on-tick-8%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
n,oqxf2 0V1