Do I need different objects of PreparedStatement for each different SQL Query?
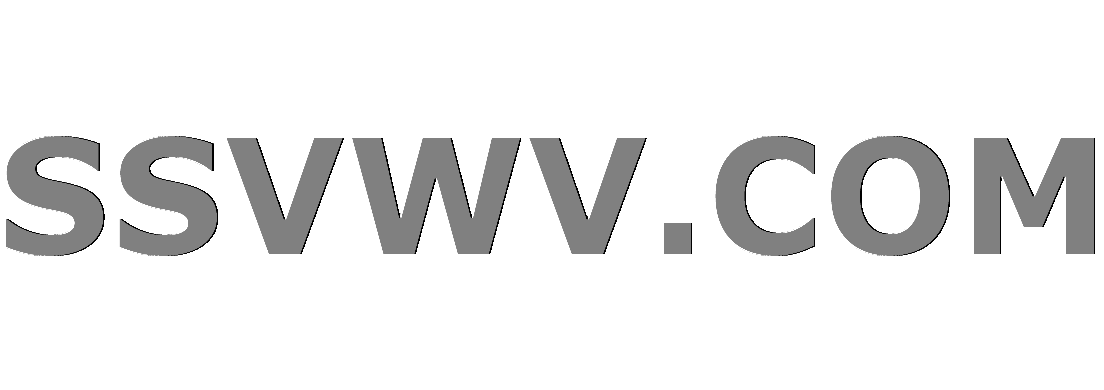
Multi tool use
I am working on a java application and using Derby Database.
I have one object of PreparedStatement named pstmt.
1) Can I use same object (here pstmt) for every SELECT Statements like these and others Statements (Would it be an efficient way) ?
For Ex:
pstmt = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 WHERE .....");
or I have to create different objects per different SQL Statement like below..
pstmt1 = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
pstmt2 = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
pstmt3 = conn.prepareStatement("SELECT * FROM TABLE1 WHERE .....");
2) And what about other Statements like CREATE, INSERT, UPDATE etc. Can I use a common object for each type of operation (like one object for every SELECT Statements, one for every INSERT, one for UPDATE Statements and so on..)
I know the advantages of it but I am confused with efficient usage of it.
java jdbc prepared-statement
add a comment |
I am working on a java application and using Derby Database.
I have one object of PreparedStatement named pstmt.
1) Can I use same object (here pstmt) for every SELECT Statements like these and others Statements (Would it be an efficient way) ?
For Ex:
pstmt = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 WHERE .....");
or I have to create different objects per different SQL Statement like below..
pstmt1 = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
pstmt2 = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
pstmt3 = conn.prepareStatement("SELECT * FROM TABLE1 WHERE .....");
2) And what about other Statements like CREATE, INSERT, UPDATE etc. Can I use a common object for each type of operation (like one object for every SELECT Statements, one for every INSERT, one for UPDATE Statements and so on..)
I know the advantages of it but I am confused with efficient usage of it.
java jdbc prepared-statement
add a comment |
I am working on a java application and using Derby Database.
I have one object of PreparedStatement named pstmt.
1) Can I use same object (here pstmt) for every SELECT Statements like these and others Statements (Would it be an efficient way) ?
For Ex:
pstmt = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 WHERE .....");
or I have to create different objects per different SQL Statement like below..
pstmt1 = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
pstmt2 = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
pstmt3 = conn.prepareStatement("SELECT * FROM TABLE1 WHERE .....");
2) And what about other Statements like CREATE, INSERT, UPDATE etc. Can I use a common object for each type of operation (like one object for every SELECT Statements, one for every INSERT, one for UPDATE Statements and so on..)
I know the advantages of it but I am confused with efficient usage of it.
java jdbc prepared-statement
I am working on a java application and using Derby Database.
I have one object of PreparedStatement named pstmt.
1) Can I use same object (here pstmt) for every SELECT Statements like these and others Statements (Would it be an efficient way) ?
For Ex:
pstmt = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 WHERE .....");
or I have to create different objects per different SQL Statement like below..
pstmt1 = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
pstmt2 = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
pstmt3 = conn.prepareStatement("SELECT * FROM TABLE1 WHERE .....");
2) And what about other Statements like CREATE, INSERT, UPDATE etc. Can I use a common object for each type of operation (like one object for every SELECT Statements, one for every INSERT, one for UPDATE Statements and so on..)
I know the advantages of it but I am confused with efficient usage of it.
java jdbc prepared-statement
java jdbc prepared-statement
edited Nov 13 '18 at 5:11
Mureinik
181k22132200
181k22132200
asked May 21 '15 at 14:56
Eagle_EyeEagle_Eye
5511618
5511618
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
When you call conn.prepareStatement
you are creating a new object (assuming the Connection
object isn't some sort of caching factory pattern, which is probably a safe assumption to make). But from your question you aren't asking about using the same object, but reusing the same variable, pstmt
. There's nothing wrong with doing that, just make sure to close()
the previous one, otherwise you'd be leaking cursors:
pstmt = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
// Missing pstmt.close();
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
The second assingment loses the reference to the previous pstmt
, without close()
being called on it. Hopefully, it will be closed when the garbage collector picks it up (depending on the database-specific implementation of the JDBC driver). But even if it does, you're leaving a dangling cursor open until the garbage collector finally gets round to it.
What is caching factory pattern and leaking cursors ? Can you attach any link to refer these ?
– Eagle_Eye
May 22 '15 at 7:17
A leaking cursor is any statement you didn'tclose()
. Wrt caching - aConnection
object can, in theory, return a previously usedPreparedStatement
object if it already prepared the same string (i.e., a cache), although I admit I've yet to encounter a JDBC implementation that did so.
– Mureinik
May 22 '15 at 9:20
add a comment |
It is perfectly fine to reuse same PreparedStatement
. I would rather say that it is more efficient way than creating multiple PreparedStatement
objects. For further details, you can go through this link http://www.jooq.org/doc/3.2/manual/sql-execution/reusing-statements/#N129B1
About the second doubt, instead of using different PrepareStatement
objects for CREATE
, INSERT
and UPDATE
, (if the use doesn't overlap) you can even reuse single PreparedStatement
object for all the statements.
"if the use doesn't overlap" - What does this means ?
– Eagle_Eye
May 22 '15 at 7:10
So I can work with a single object for any type of statements ?
– Eagle_Eye
May 22 '15 at 7:16
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f30377394%2fdo-i-need-different-objects-of-preparedstatement-for-each-different-sql-query%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
When you call conn.prepareStatement
you are creating a new object (assuming the Connection
object isn't some sort of caching factory pattern, which is probably a safe assumption to make). But from your question you aren't asking about using the same object, but reusing the same variable, pstmt
. There's nothing wrong with doing that, just make sure to close()
the previous one, otherwise you'd be leaking cursors:
pstmt = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
// Missing pstmt.close();
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
The second assingment loses the reference to the previous pstmt
, without close()
being called on it. Hopefully, it will be closed when the garbage collector picks it up (depending on the database-specific implementation of the JDBC driver). But even if it does, you're leaving a dangling cursor open until the garbage collector finally gets round to it.
What is caching factory pattern and leaking cursors ? Can you attach any link to refer these ?
– Eagle_Eye
May 22 '15 at 7:17
A leaking cursor is any statement you didn'tclose()
. Wrt caching - aConnection
object can, in theory, return a previously usedPreparedStatement
object if it already prepared the same string (i.e., a cache), although I admit I've yet to encounter a JDBC implementation that did so.
– Mureinik
May 22 '15 at 9:20
add a comment |
When you call conn.prepareStatement
you are creating a new object (assuming the Connection
object isn't some sort of caching factory pattern, which is probably a safe assumption to make). But from your question you aren't asking about using the same object, but reusing the same variable, pstmt
. There's nothing wrong with doing that, just make sure to close()
the previous one, otherwise you'd be leaking cursors:
pstmt = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
// Missing pstmt.close();
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
The second assingment loses the reference to the previous pstmt
, without close()
being called on it. Hopefully, it will be closed when the garbage collector picks it up (depending on the database-specific implementation of the JDBC driver). But even if it does, you're leaving a dangling cursor open until the garbage collector finally gets round to it.
What is caching factory pattern and leaking cursors ? Can you attach any link to refer these ?
– Eagle_Eye
May 22 '15 at 7:17
A leaking cursor is any statement you didn'tclose()
. Wrt caching - aConnection
object can, in theory, return a previously usedPreparedStatement
object if it already prepared the same string (i.e., a cache), although I admit I've yet to encounter a JDBC implementation that did so.
– Mureinik
May 22 '15 at 9:20
add a comment |
When you call conn.prepareStatement
you are creating a new object (assuming the Connection
object isn't some sort of caching factory pattern, which is probably a safe assumption to make). But from your question you aren't asking about using the same object, but reusing the same variable, pstmt
. There's nothing wrong with doing that, just make sure to close()
the previous one, otherwise you'd be leaking cursors:
pstmt = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
// Missing pstmt.close();
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
The second assingment loses the reference to the previous pstmt
, without close()
being called on it. Hopefully, it will be closed when the garbage collector picks it up (depending on the database-specific implementation of the JDBC driver). But even if it does, you're leaving a dangling cursor open until the garbage collector finally gets round to it.
When you call conn.prepareStatement
you are creating a new object (assuming the Connection
object isn't some sort of caching factory pattern, which is probably a safe assumption to make). But from your question you aren't asking about using the same object, but reusing the same variable, pstmt
. There's nothing wrong with doing that, just make sure to close()
the previous one, otherwise you'd be leaking cursors:
pstmt = conn.prepareStatement("SELECT NAME FROM TABLE1 ORDER BY NAME");
// Missing pstmt.close();
pstmt = conn.prepareStatement("SELECT * FROM TABLE1 ORDER BY NAME");
The second assingment loses the reference to the previous pstmt
, without close()
being called on it. Hopefully, it will be closed when the garbage collector picks it up (depending on the database-specific implementation of the JDBC driver). But even if it does, you're leaving a dangling cursor open until the garbage collector finally gets round to it.
answered May 21 '15 at 15:03
MureinikMureinik
181k22132200
181k22132200
What is caching factory pattern and leaking cursors ? Can you attach any link to refer these ?
– Eagle_Eye
May 22 '15 at 7:17
A leaking cursor is any statement you didn'tclose()
. Wrt caching - aConnection
object can, in theory, return a previously usedPreparedStatement
object if it already prepared the same string (i.e., a cache), although I admit I've yet to encounter a JDBC implementation that did so.
– Mureinik
May 22 '15 at 9:20
add a comment |
What is caching factory pattern and leaking cursors ? Can you attach any link to refer these ?
– Eagle_Eye
May 22 '15 at 7:17
A leaking cursor is any statement you didn'tclose()
. Wrt caching - aConnection
object can, in theory, return a previously usedPreparedStatement
object if it already prepared the same string (i.e., a cache), although I admit I've yet to encounter a JDBC implementation that did so.
– Mureinik
May 22 '15 at 9:20
What is caching factory pattern and leaking cursors ? Can you attach any link to refer these ?
– Eagle_Eye
May 22 '15 at 7:17
What is caching factory pattern and leaking cursors ? Can you attach any link to refer these ?
– Eagle_Eye
May 22 '15 at 7:17
A leaking cursor is any statement you didn't
close()
. Wrt caching - a Connection
object can, in theory, return a previously used PreparedStatement
object if it already prepared the same string (i.e., a cache), although I admit I've yet to encounter a JDBC implementation that did so.– Mureinik
May 22 '15 at 9:20
A leaking cursor is any statement you didn't
close()
. Wrt caching - a Connection
object can, in theory, return a previously used PreparedStatement
object if it already prepared the same string (i.e., a cache), although I admit I've yet to encounter a JDBC implementation that did so.– Mureinik
May 22 '15 at 9:20
add a comment |
It is perfectly fine to reuse same PreparedStatement
. I would rather say that it is more efficient way than creating multiple PreparedStatement
objects. For further details, you can go through this link http://www.jooq.org/doc/3.2/manual/sql-execution/reusing-statements/#N129B1
About the second doubt, instead of using different PrepareStatement
objects for CREATE
, INSERT
and UPDATE
, (if the use doesn't overlap) you can even reuse single PreparedStatement
object for all the statements.
"if the use doesn't overlap" - What does this means ?
– Eagle_Eye
May 22 '15 at 7:10
So I can work with a single object for any type of statements ?
– Eagle_Eye
May 22 '15 at 7:16
add a comment |
It is perfectly fine to reuse same PreparedStatement
. I would rather say that it is more efficient way than creating multiple PreparedStatement
objects. For further details, you can go through this link http://www.jooq.org/doc/3.2/manual/sql-execution/reusing-statements/#N129B1
About the second doubt, instead of using different PrepareStatement
objects for CREATE
, INSERT
and UPDATE
, (if the use doesn't overlap) you can even reuse single PreparedStatement
object for all the statements.
"if the use doesn't overlap" - What does this means ?
– Eagle_Eye
May 22 '15 at 7:10
So I can work with a single object for any type of statements ?
– Eagle_Eye
May 22 '15 at 7:16
add a comment |
It is perfectly fine to reuse same PreparedStatement
. I would rather say that it is more efficient way than creating multiple PreparedStatement
objects. For further details, you can go through this link http://www.jooq.org/doc/3.2/manual/sql-execution/reusing-statements/#N129B1
About the second doubt, instead of using different PrepareStatement
objects for CREATE
, INSERT
and UPDATE
, (if the use doesn't overlap) you can even reuse single PreparedStatement
object for all the statements.
It is perfectly fine to reuse same PreparedStatement
. I would rather say that it is more efficient way than creating multiple PreparedStatement
objects. For further details, you can go through this link http://www.jooq.org/doc/3.2/manual/sql-execution/reusing-statements/#N129B1
About the second doubt, instead of using different PrepareStatement
objects for CREATE
, INSERT
and UPDATE
, (if the use doesn't overlap) you can even reuse single PreparedStatement
object for all the statements.
edited May 21 '15 at 15:08
answered May 21 '15 at 15:01
santosh-patilsantosh-patil
1,14111225
1,14111225
"if the use doesn't overlap" - What does this means ?
– Eagle_Eye
May 22 '15 at 7:10
So I can work with a single object for any type of statements ?
– Eagle_Eye
May 22 '15 at 7:16
add a comment |
"if the use doesn't overlap" - What does this means ?
– Eagle_Eye
May 22 '15 at 7:10
So I can work with a single object for any type of statements ?
– Eagle_Eye
May 22 '15 at 7:16
"if the use doesn't overlap" - What does this means ?
– Eagle_Eye
May 22 '15 at 7:10
"if the use doesn't overlap" - What does this means ?
– Eagle_Eye
May 22 '15 at 7:10
So I can work with a single object for any type of statements ?
– Eagle_Eye
May 22 '15 at 7:16
So I can work with a single object for any type of statements ?
– Eagle_Eye
May 22 '15 at 7:16
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f30377394%2fdo-i-need-different-objects-of-preparedstatement-for-each-different-sql-query%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vfQ24KjMa7wfCivqZBDNUB JLcXPhX,nBkRiBOmQQNhyx0 T rMk4toD,wsRr 9D OBVGqX7BiR2QUZc57VfkMkmap