reading structured binary data in python3.6 using struct
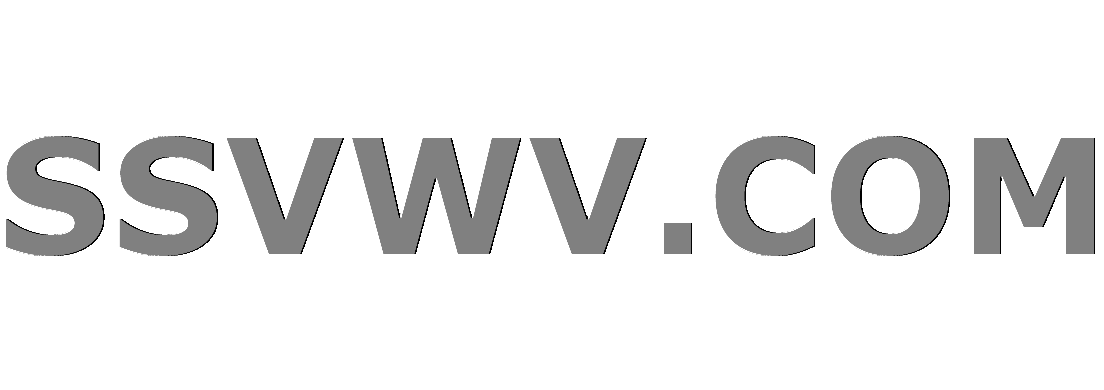
Multi tool use
up vote
0
down vote
favorite
I really tried to look for this doubt in many ways but maybe since I never worked with binary files before I don't have any idea what the keywords to search similar things to help me out. That's why I am asking here.
So, I have a file:
path = 'myPath/file.pd0'
in_file = open(path, "rb")
read_file = in_file.read()
type(read_file)
when I try to check what is inside read_file I get:
b'x7fx7fxccx05x00x0f$x00`x00xa2x00$x02xe6x02xa8x03xd0x032x04dx04x96x04xa6x04xe0x04'
The type of read_file is bytes. When I try to use struct since it is the function people suggest I get the following error:
import struct
struct.unpack('hhl', read_file[0:30])
error: unpack requires a buffer of 16 bytes
No matter what fmt I get unpack requires a buffer of n bytes.
The file structure that I am trying to read is defined as follow:
HEADER
(6 BYTES + [2 x No. OF DATA TYPES])
FIXED LEADER DATA
(60 BYTES)
VARIABLE LEADER DATA
(66 BYTES)
CORRELATION MAGNITUDE
(2 BYTES + 4 BYTES PER DEPTH CELL)
Any idea how I could start reading these bytes using struct or something similar in python?
Thank you
python struct binary ascii python-3.6
add a comment |
up vote
0
down vote
favorite
I really tried to look for this doubt in many ways but maybe since I never worked with binary files before I don't have any idea what the keywords to search similar things to help me out. That's why I am asking here.
So, I have a file:
path = 'myPath/file.pd0'
in_file = open(path, "rb")
read_file = in_file.read()
type(read_file)
when I try to check what is inside read_file I get:
b'x7fx7fxccx05x00x0f$x00`x00xa2x00$x02xe6x02xa8x03xd0x032x04dx04x96x04xa6x04xe0x04'
The type of read_file is bytes. When I try to use struct since it is the function people suggest I get the following error:
import struct
struct.unpack('hhl', read_file[0:30])
error: unpack requires a buffer of 16 bytes
No matter what fmt I get unpack requires a buffer of n bytes.
The file structure that I am trying to read is defined as follow:
HEADER
(6 BYTES + [2 x No. OF DATA TYPES])
FIXED LEADER DATA
(60 BYTES)
VARIABLE LEADER DATA
(66 BYTES)
CORRELATION MAGNITUDE
(2 BYTES + 4 BYTES PER DEPTH CELL)
Any idea how I could start reading these bytes using struct or something similar in python?
Thank you
python struct binary ascii python-3.6
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I really tried to look for this doubt in many ways but maybe since I never worked with binary files before I don't have any idea what the keywords to search similar things to help me out. That's why I am asking here.
So, I have a file:
path = 'myPath/file.pd0'
in_file = open(path, "rb")
read_file = in_file.read()
type(read_file)
when I try to check what is inside read_file I get:
b'x7fx7fxccx05x00x0f$x00`x00xa2x00$x02xe6x02xa8x03xd0x032x04dx04x96x04xa6x04xe0x04'
The type of read_file is bytes. When I try to use struct since it is the function people suggest I get the following error:
import struct
struct.unpack('hhl', read_file[0:30])
error: unpack requires a buffer of 16 bytes
No matter what fmt I get unpack requires a buffer of n bytes.
The file structure that I am trying to read is defined as follow:
HEADER
(6 BYTES + [2 x No. OF DATA TYPES])
FIXED LEADER DATA
(60 BYTES)
VARIABLE LEADER DATA
(66 BYTES)
CORRELATION MAGNITUDE
(2 BYTES + 4 BYTES PER DEPTH CELL)
Any idea how I could start reading these bytes using struct or something similar in python?
Thank you
python struct binary ascii python-3.6
I really tried to look for this doubt in many ways but maybe since I never worked with binary files before I don't have any idea what the keywords to search similar things to help me out. That's why I am asking here.
So, I have a file:
path = 'myPath/file.pd0'
in_file = open(path, "rb")
read_file = in_file.read()
type(read_file)
when I try to check what is inside read_file I get:
b'x7fx7fxccx05x00x0f$x00`x00xa2x00$x02xe6x02xa8x03xd0x032x04dx04x96x04xa6x04xe0x04'
The type of read_file is bytes. When I try to use struct since it is the function people suggest I get the following error:
import struct
struct.unpack('hhl', read_file[0:30])
error: unpack requires a buffer of 16 bytes
No matter what fmt I get unpack requires a buffer of n bytes.
The file structure that I am trying to read is defined as follow:
HEADER
(6 BYTES + [2 x No. OF DATA TYPES])
FIXED LEADER DATA
(60 BYTES)
VARIABLE LEADER DATA
(66 BYTES)
CORRELATION MAGNITUDE
(2 BYTES + 4 BYTES PER DEPTH CELL)
Any idea how I could start reading these bytes using struct or something similar in python?
Thank you
python struct binary ascii python-3.6
python struct binary ascii python-3.6
asked Nov 10 at 19:13
moehbon
267
267
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
unpack()
expects bytes which are the exact length of the format described by its first argument.
The format string 'hhl'
describes data of 16 bytes (on your machine - see below), so you must pass a byte string of 16.
If you want to parse only part of the bytes, you can do this:
fmt = 'hhl'
size = struct.calcsize(fmt)
struct.unpack(fmt, data[:size])
Additionally, your format string doesn't have a byte order, size and alignment specifier. It is assumed to be "native" by default. This means your code is system-dependent, which is probably not what you want for parsing a file format.
You might need different alignments for different parts of the file.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
unpack()
expects bytes which are the exact length of the format described by its first argument.
The format string 'hhl'
describes data of 16 bytes (on your machine - see below), so you must pass a byte string of 16.
If you want to parse only part of the bytes, you can do this:
fmt = 'hhl'
size = struct.calcsize(fmt)
struct.unpack(fmt, data[:size])
Additionally, your format string doesn't have a byte order, size and alignment specifier. It is assumed to be "native" by default. This means your code is system-dependent, which is probably not what you want for parsing a file format.
You might need different alignments for different parts of the file.
add a comment |
up vote
0
down vote
unpack()
expects bytes which are the exact length of the format described by its first argument.
The format string 'hhl'
describes data of 16 bytes (on your machine - see below), so you must pass a byte string of 16.
If you want to parse only part of the bytes, you can do this:
fmt = 'hhl'
size = struct.calcsize(fmt)
struct.unpack(fmt, data[:size])
Additionally, your format string doesn't have a byte order, size and alignment specifier. It is assumed to be "native" by default. This means your code is system-dependent, which is probably not what you want for parsing a file format.
You might need different alignments for different parts of the file.
add a comment |
up vote
0
down vote
up vote
0
down vote
unpack()
expects bytes which are the exact length of the format described by its first argument.
The format string 'hhl'
describes data of 16 bytes (on your machine - see below), so you must pass a byte string of 16.
If you want to parse only part of the bytes, you can do this:
fmt = 'hhl'
size = struct.calcsize(fmt)
struct.unpack(fmt, data[:size])
Additionally, your format string doesn't have a byte order, size and alignment specifier. It is assumed to be "native" by default. This means your code is system-dependent, which is probably not what you want for parsing a file format.
You might need different alignments for different parts of the file.
unpack()
expects bytes which are the exact length of the format described by its first argument.
The format string 'hhl'
describes data of 16 bytes (on your machine - see below), so you must pass a byte string of 16.
If you want to parse only part of the bytes, you can do this:
fmt = 'hhl'
size = struct.calcsize(fmt)
struct.unpack(fmt, data[:size])
Additionally, your format string doesn't have a byte order, size and alignment specifier. It is assumed to be "native" by default. This means your code is system-dependent, which is probably not what you want for parsing a file format.
You might need different alignments for different parts of the file.
edited Nov 10 at 19:42
answered Nov 10 at 19:34
roeen30
37619
37619
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53242511%2freading-structured-binary-data-in-python3-6-using-struct%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UCvage u 7gz QS ETubCrqB,v7CtZEmWmsFlm,HV8,i