Does the main method get an initialized array or are the strings in the command line enter directly in to the...
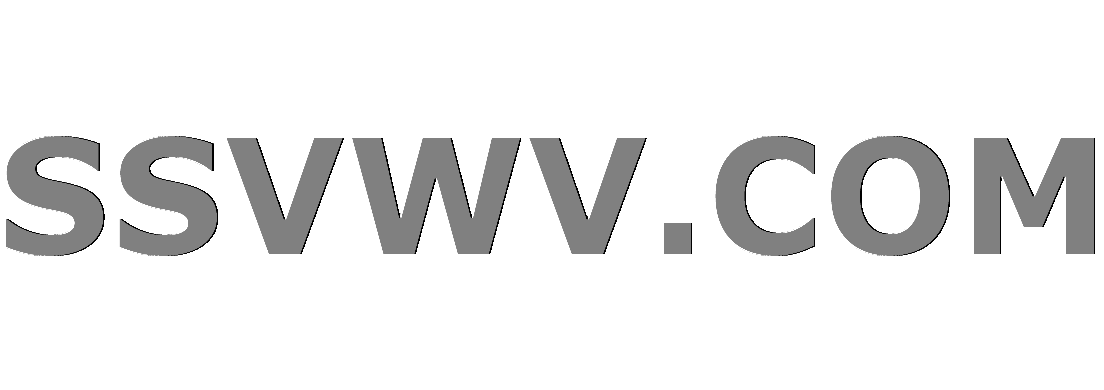
Multi tool use
up vote
-2
down vote
favorite
For example, if I want to print an array length, I can not do that:
public class Test{
public static void main(String args){
System.out.println(worngParam({"first", "second", "ect"}));
}
public static int worngParam(String strings){
return strings.length;
}
}
This is an error!
The first two lines in main must be
String strings = {"first", "second", "ect"};
System.out.println(worngParam(strings));
But even so I can do that:
System.out.println(args.length);//If of course args is not empty
My question is how does the parameter get into the main method?
Although any method can accept a constant variabls such as 3, "word", 'a'
. But she can not get an initialization of an array like this {1,8}
or {"word2", "word3"}
java arrays parameters command-line-arguments main
add a comment |
up vote
-2
down vote
favorite
For example, if I want to print an array length, I can not do that:
public class Test{
public static void main(String args){
System.out.println(worngParam({"first", "second", "ect"}));
}
public static int worngParam(String strings){
return strings.length;
}
}
This is an error!
The first two lines in main must be
String strings = {"first", "second", "ect"};
System.out.println(worngParam(strings));
But even so I can do that:
System.out.println(args.length);//If of course args is not empty
My question is how does the parameter get into the main method?
Although any method can accept a constant variabls such as 3, "word", 'a'
. But she can not get an initialization of an array like this {1,8}
or {"word2", "word3"}
java arrays parameters command-line-arguments main
1
You have to passnew String {"first", "second", "ect"}
instead of{"first", "second", "ect"}
when calling a method.
– GriffeyDog
Nov 7 at 21:53
1
{ "first", "second" }
is called an array initializer, and is shorthand fornew String { "first", "second" }
. The thing is, omitting thenew String
part is only allowed at the time of declaration of the array.
– MC Emperor
Nov 7 at 21:58
1
Theargs
string array is constructed by the JVM and passed to yourmain(String)
method upon start.
– MC Emperor
Nov 7 at 22:03
add a comment |
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
For example, if I want to print an array length, I can not do that:
public class Test{
public static void main(String args){
System.out.println(worngParam({"first", "second", "ect"}));
}
public static int worngParam(String strings){
return strings.length;
}
}
This is an error!
The first two lines in main must be
String strings = {"first", "second", "ect"};
System.out.println(worngParam(strings));
But even so I can do that:
System.out.println(args.length);//If of course args is not empty
My question is how does the parameter get into the main method?
Although any method can accept a constant variabls such as 3, "word", 'a'
. But she can not get an initialization of an array like this {1,8}
or {"word2", "word3"}
java arrays parameters command-line-arguments main
For example, if I want to print an array length, I can not do that:
public class Test{
public static void main(String args){
System.out.println(worngParam({"first", "second", "ect"}));
}
public static int worngParam(String strings){
return strings.length;
}
}
This is an error!
The first two lines in main must be
String strings = {"first", "second", "ect"};
System.out.println(worngParam(strings));
But even so I can do that:
System.out.println(args.length);//If of course args is not empty
My question is how does the parameter get into the main method?
Although any method can accept a constant variabls such as 3, "word", 'a'
. But she can not get an initialization of an array like this {1,8}
or {"word2", "word3"}
java arrays parameters command-line-arguments main
java arrays parameters command-line-arguments main
edited Nov 7 at 21:43
asked Nov 7 at 21:27
ploni almoni
32
32
1
You have to passnew String {"first", "second", "ect"}
instead of{"first", "second", "ect"}
when calling a method.
– GriffeyDog
Nov 7 at 21:53
1
{ "first", "second" }
is called an array initializer, and is shorthand fornew String { "first", "second" }
. The thing is, omitting thenew String
part is only allowed at the time of declaration of the array.
– MC Emperor
Nov 7 at 21:58
1
Theargs
string array is constructed by the JVM and passed to yourmain(String)
method upon start.
– MC Emperor
Nov 7 at 22:03
add a comment |
1
You have to passnew String {"first", "second", "ect"}
instead of{"first", "second", "ect"}
when calling a method.
– GriffeyDog
Nov 7 at 21:53
1
{ "first", "second" }
is called an array initializer, and is shorthand fornew String { "first", "second" }
. The thing is, omitting thenew String
part is only allowed at the time of declaration of the array.
– MC Emperor
Nov 7 at 21:58
1
Theargs
string array is constructed by the JVM and passed to yourmain(String)
method upon start.
– MC Emperor
Nov 7 at 22:03
1
1
You have to pass
new String {"first", "second", "ect"}
instead of {"first", "second", "ect"}
when calling a method.– GriffeyDog
Nov 7 at 21:53
You have to pass
new String {"first", "second", "ect"}
instead of {"first", "second", "ect"}
when calling a method.– GriffeyDog
Nov 7 at 21:53
1
1
{ "first", "second" }
is called an array initializer, and is shorthand for new String { "first", "second" }
. The thing is, omitting the new String
part is only allowed at the time of declaration of the array.– MC Emperor
Nov 7 at 21:58
{ "first", "second" }
is called an array initializer, and is shorthand for new String { "first", "second" }
. The thing is, omitting the new String
part is only allowed at the time of declaration of the array.– MC Emperor
Nov 7 at 21:58
1
1
The
args
string array is constructed by the JVM and passed to your main(String)
method upon start.– MC Emperor
Nov 7 at 22:03
The
args
string array is constructed by the JVM and passed to your main(String)
method upon start.– MC Emperor
Nov 7 at 22:03
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
Supposed you run your java class file like this
javac Test.java
java -cp . Test firstParam secondParam thirdParam
Then in your main method args will have a value like
args = new String{"firstParam", "secondParam", "thirdParam"};
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Supposed you run your java class file like this
javac Test.java
java -cp . Test firstParam secondParam thirdParam
Then in your main method args will have a value like
args = new String{"firstParam", "secondParam", "thirdParam"};
add a comment |
up vote
0
down vote
accepted
Supposed you run your java class file like this
javac Test.java
java -cp . Test firstParam secondParam thirdParam
Then in your main method args will have a value like
args = new String{"firstParam", "secondParam", "thirdParam"};
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Supposed you run your java class file like this
javac Test.java
java -cp . Test firstParam secondParam thirdParam
Then in your main method args will have a value like
args = new String{"firstParam", "secondParam", "thirdParam"};
Supposed you run your java class file like this
javac Test.java
java -cp . Test firstParam secondParam thirdParam
Then in your main method args will have a value like
args = new String{"firstParam", "secondParam", "thirdParam"};
edited Nov 10 at 19:12
answered Nov 7 at 23:35
Donat
3566
3566
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53198087%2fdoes-the-main-method-get-an-initialized-array-or-are-the-strings-in-the-command%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zzD1dK
1
You have to pass
new String {"first", "second", "ect"}
instead of{"first", "second", "ect"}
when calling a method.– GriffeyDog
Nov 7 at 21:53
1
{ "first", "second" }
is called an array initializer, and is shorthand fornew String { "first", "second" }
. The thing is, omitting thenew String
part is only allowed at the time of declaration of the array.– MC Emperor
Nov 7 at 21:58
1
The
args
string array is constructed by the JVM and passed to yourmain(String)
method upon start.– MC Emperor
Nov 7 at 22:03