Increment element at index
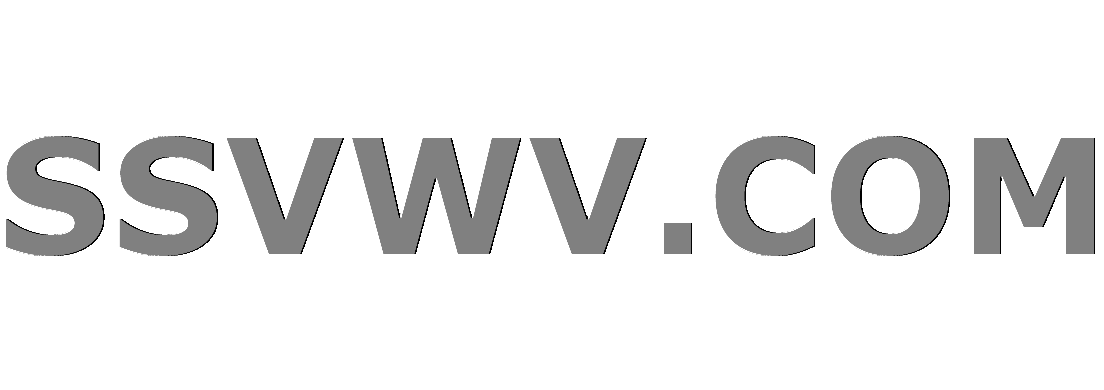
Multi tool use
up vote
3
down vote
favorite
I am working on the guide.elm-lang website on the Random example.
I am trying to add a feature that shows you the total number of times you threw the dice, and some stats on how many times you got each face.
To do this I've changed the model to look like this:
type alias Model =
{
die_face : Int,
total_throws : Int,
stats: Array.Array Int
}
and this is what I do to update the model:
{ model |
die_face = face,
total_throws = model.total_throws + 1,
stats = Array.set face ((Array.get face model.stats) + 1) model.stats
}
this throws an error that tells me:
This
get
call produces:
#Maybe# Int
But (+) only works with #Int# and #Float# values.
Which refers to Array.get
not returning a Int
but a Maybe
and therefore I can't add it to the number 1.
I have tried using lists to achieve the same purpose but since they are not indexed I am not sure what to increment when I map over it. I am thinking of using records to do this and figure out a way to map the record keys to the face
Int.
In general my question is. What is a good method to increment an element at index [x] of an Array in elm ? Or if I am just thinking about this wrong, what would be the elm way ?
arrays elm maybe
add a comment |
up vote
3
down vote
favorite
I am working on the guide.elm-lang website on the Random example.
I am trying to add a feature that shows you the total number of times you threw the dice, and some stats on how many times you got each face.
To do this I've changed the model to look like this:
type alias Model =
{
die_face : Int,
total_throws : Int,
stats: Array.Array Int
}
and this is what I do to update the model:
{ model |
die_face = face,
total_throws = model.total_throws + 1,
stats = Array.set face ((Array.get face model.stats) + 1) model.stats
}
this throws an error that tells me:
This
get
call produces:
#Maybe# Int
But (+) only works with #Int# and #Float# values.
Which refers to Array.get
not returning a Int
but a Maybe
and therefore I can't add it to the number 1.
I have tried using lists to achieve the same purpose but since they are not indexed I am not sure what to increment when I map over it. I am thinking of using records to do this and figure out a way to map the record keys to the face
Int.
In general my question is. What is a good method to increment an element at index [x] of an Array in elm ? Or if I am just thinking about this wrong, what would be the elm way ?
arrays elm maybe
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
I am working on the guide.elm-lang website on the Random example.
I am trying to add a feature that shows you the total number of times you threw the dice, and some stats on how many times you got each face.
To do this I've changed the model to look like this:
type alias Model =
{
die_face : Int,
total_throws : Int,
stats: Array.Array Int
}
and this is what I do to update the model:
{ model |
die_face = face,
total_throws = model.total_throws + 1,
stats = Array.set face ((Array.get face model.stats) + 1) model.stats
}
this throws an error that tells me:
This
get
call produces:
#Maybe# Int
But (+) only works with #Int# and #Float# values.
Which refers to Array.get
not returning a Int
but a Maybe
and therefore I can't add it to the number 1.
I have tried using lists to achieve the same purpose but since they are not indexed I am not sure what to increment when I map over it. I am thinking of using records to do this and figure out a way to map the record keys to the face
Int.
In general my question is. What is a good method to increment an element at index [x] of an Array in elm ? Or if I am just thinking about this wrong, what would be the elm way ?
arrays elm maybe
I am working on the guide.elm-lang website on the Random example.
I am trying to add a feature that shows you the total number of times you threw the dice, and some stats on how many times you got each face.
To do this I've changed the model to look like this:
type alias Model =
{
die_face : Int,
total_throws : Int,
stats: Array.Array Int
}
and this is what I do to update the model:
{ model |
die_face = face,
total_throws = model.total_throws + 1,
stats = Array.set face ((Array.get face model.stats) + 1) model.stats
}
this throws an error that tells me:
This
get
call produces:
#Maybe# Int
But (+) only works with #Int# and #Float# values.
Which refers to Array.get
not returning a Int
but a Maybe
and therefore I can't add it to the number 1.
I have tried using lists to achieve the same purpose but since they are not indexed I am not sure what to increment when I map over it. I am thinking of using records to do this and figure out a way to map the record keys to the face
Int.
In general my question is. What is a good method to increment an element at index [x] of an Array in elm ? Or if I am just thinking about this wrong, what would be the elm way ?
arrays elm maybe
arrays elm maybe
edited Nov 10 at 22:03
glennsl
8,853102545
8,853102545
asked Nov 10 at 21:51


Nicola Pedretti
1,81911522
1,81911522
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
5
down vote
accepted
Array.get
returns a Maybe
because it has to account for the case where the index is outside the array. The simplest way of getting around that is using Maybe.withDefault
with a reasonable default value:
Array.set face (((Array.get face model.stats) |> Maybe.withDefault 0) + 1) model.stats
It might be a good idea to write a helper function for this though, to clean up the code a bit. Something like this:
incrementAt : Int -> Array Int -> Array Int
incrementAt index array =
case Array.get index array of
Just value ->
Array.set index (value + 1) array
Nothing ->
array
You can also use Array.Extra.update
if you don't mind the extra dependency.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
5
down vote
accepted
Array.get
returns a Maybe
because it has to account for the case where the index is outside the array. The simplest way of getting around that is using Maybe.withDefault
with a reasonable default value:
Array.set face (((Array.get face model.stats) |> Maybe.withDefault 0) + 1) model.stats
It might be a good idea to write a helper function for this though, to clean up the code a bit. Something like this:
incrementAt : Int -> Array Int -> Array Int
incrementAt index array =
case Array.get index array of
Just value ->
Array.set index (value + 1) array
Nothing ->
array
You can also use Array.Extra.update
if you don't mind the extra dependency.
add a comment |
up vote
5
down vote
accepted
Array.get
returns a Maybe
because it has to account for the case where the index is outside the array. The simplest way of getting around that is using Maybe.withDefault
with a reasonable default value:
Array.set face (((Array.get face model.stats) |> Maybe.withDefault 0) + 1) model.stats
It might be a good idea to write a helper function for this though, to clean up the code a bit. Something like this:
incrementAt : Int -> Array Int -> Array Int
incrementAt index array =
case Array.get index array of
Just value ->
Array.set index (value + 1) array
Nothing ->
array
You can also use Array.Extra.update
if you don't mind the extra dependency.
add a comment |
up vote
5
down vote
accepted
up vote
5
down vote
accepted
Array.get
returns a Maybe
because it has to account for the case where the index is outside the array. The simplest way of getting around that is using Maybe.withDefault
with a reasonable default value:
Array.set face (((Array.get face model.stats) |> Maybe.withDefault 0) + 1) model.stats
It might be a good idea to write a helper function for this though, to clean up the code a bit. Something like this:
incrementAt : Int -> Array Int -> Array Int
incrementAt index array =
case Array.get index array of
Just value ->
Array.set index (value + 1) array
Nothing ->
array
You can also use Array.Extra.update
if you don't mind the extra dependency.
Array.get
returns a Maybe
because it has to account for the case where the index is outside the array. The simplest way of getting around that is using Maybe.withDefault
with a reasonable default value:
Array.set face (((Array.get face model.stats) |> Maybe.withDefault 0) + 1) model.stats
It might be a good idea to write a helper function for this though, to clean up the code a bit. Something like this:
incrementAt : Int -> Array Int -> Array Int
incrementAt index array =
case Array.get index array of
Just value ->
Array.set index (value + 1) array
Nothing ->
array
You can also use Array.Extra.update
if you don't mind the extra dependency.
answered Nov 10 at 22:01
glennsl
8,853102545
8,853102545
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243760%2fincrement-element-at-index%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
x5frSFLWXKt2o3afvAdJ0P3p