refactoring function with different methods - javascript
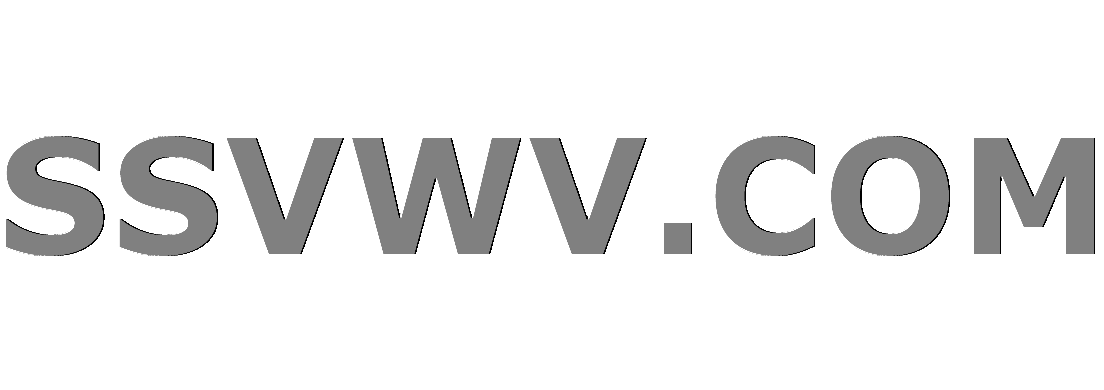
Multi tool use
This function current takes in an array, and outputs it a character with its count if the element's length is greater than one
Is there a way I can do this same thing, perhaps with a different javascript array method without having to use a new array or a result variable?
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
let newArray = ;
let result = arr.forEach(e => e.length > 1 ? newArray.push(`${e[0]}${e.length}`) : newArray.push(e))
return newArray.join("");
}
console.log(compressCharacters(a));
javascript
add a comment |
This function current takes in an array, and outputs it a character with its count if the element's length is greater than one
Is there a way I can do this same thing, perhaps with a different javascript array method without having to use a new array or a result variable?
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
let newArray = ;
let result = arr.forEach(e => e.length > 1 ? newArray.push(`${e[0]}${e.length}`) : newArray.push(e))
return newArray.join("");
}
console.log(compressCharacters(a));
javascript
add a comment |
This function current takes in an array, and outputs it a character with its count if the element's length is greater than one
Is there a way I can do this same thing, perhaps with a different javascript array method without having to use a new array or a result variable?
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
let newArray = ;
let result = arr.forEach(e => e.length > 1 ? newArray.push(`${e[0]}${e.length}`) : newArray.push(e))
return newArray.join("");
}
console.log(compressCharacters(a));
javascript
This function current takes in an array, and outputs it a character with its count if the element's length is greater than one
Is there a way I can do this same thing, perhaps with a different javascript array method without having to use a new array or a result variable?
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
let newArray = ;
let result = arr.forEach(e => e.length > 1 ? newArray.push(`${e[0]}${e.length}`) : newArray.push(e))
return newArray.join("");
}
console.log(compressCharacters(a));
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
let newArray = ;
let result = arr.forEach(e => e.length > 1 ? newArray.push(`${e[0]}${e.length}`) : newArray.push(e))
return newArray.join("");
}
console.log(compressCharacters(a));
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
let newArray = ;
let result = arr.forEach(e => e.length > 1 ? newArray.push(`${e[0]}${e.length}`) : newArray.push(e))
return newArray.join("");
}
console.log(compressCharacters(a));
javascript
javascript
edited Nov 13 '18 at 1:16


Ele
22.8k42044
22.8k42044
asked Nov 13 '18 at 1:11
totalnoobtotalnoob
3261631
3261631
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
You can just use map()
instead of forEach()
and return the values you want. There's no need for the extra array. It's often the case than forEach + push()
== map()
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
return arr.map(e => e.length > 1
? `${e[0]}${e.length}`
: e)
.join("");
}
console.log(compressCharacters(a));
add a comment |
You can construct and immediately return a map
ped array, that's joined by the empty string:
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => (
arr.map(str => str[0] + (str.length > 1 ? str.length : ''))
.join('')
)
console.log(compressCharacters(a));
When you're trying to construct a new array by transforming all elements from some other array, .map
is the appropriate method to use. forEach
should be reserved for generic iteration with side effects, when you aren't trying to construct a new object. (when you are trying to construct a new object, but it's not an array, or the new array isn't one-to-one with the old array, usually you can use .reduce
)
add a comment |
This is an alternative using the function reduce
to build the desired output.
const a = ['aaa', 'z', 'eeeee'],
result = a.reduce((a, [m], i, arr) => {
let {length} = arr[i];
return a + m + (length <= 1 ? "" : length);
}, "");
console.log(result);
...............
– totalnoob
Nov 13 '18 at 4:38
can you explain how this works? I've never used an array in the reduce method before or deconstructed the length of an array like that before
– totalnoob
Nov 13 '18 at 4:42
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272339%2frefactoring-function-with-different-methods-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can just use map()
instead of forEach()
and return the values you want. There's no need for the extra array. It's often the case than forEach + push()
== map()
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
return arr.map(e => e.length > 1
? `${e[0]}${e.length}`
: e)
.join("");
}
console.log(compressCharacters(a));
add a comment |
You can just use map()
instead of forEach()
and return the values you want. There's no need for the extra array. It's often the case than forEach + push()
== map()
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
return arr.map(e => e.length > 1
? `${e[0]}${e.length}`
: e)
.join("");
}
console.log(compressCharacters(a));
add a comment |
You can just use map()
instead of forEach()
and return the values you want. There's no need for the extra array. It's often the case than forEach + push()
== map()
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
return arr.map(e => e.length > 1
? `${e[0]}${e.length}`
: e)
.join("");
}
console.log(compressCharacters(a));
You can just use map()
instead of forEach()
and return the values you want. There's no need for the extra array. It's often the case than forEach + push()
== map()
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
return arr.map(e => e.length > 1
? `${e[0]}${e.length}`
: e)
.join("");
}
console.log(compressCharacters(a));
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
return arr.map(e => e.length > 1
? `${e[0]}${e.length}`
: e)
.join("");
}
console.log(compressCharacters(a));
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => {
return arr.map(e => e.length > 1
? `${e[0]}${e.length}`
: e)
.join("");
}
console.log(compressCharacters(a));
answered Nov 13 '18 at 1:17


Mark MeyerMark Meyer
38.1k33159
38.1k33159
add a comment |
add a comment |
You can construct and immediately return a map
ped array, that's joined by the empty string:
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => (
arr.map(str => str[0] + (str.length > 1 ? str.length : ''))
.join('')
)
console.log(compressCharacters(a));
When you're trying to construct a new array by transforming all elements from some other array, .map
is the appropriate method to use. forEach
should be reserved for generic iteration with side effects, when you aren't trying to construct a new object. (when you are trying to construct a new object, but it's not an array, or the new array isn't one-to-one with the old array, usually you can use .reduce
)
add a comment |
You can construct and immediately return a map
ped array, that's joined by the empty string:
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => (
arr.map(str => str[0] + (str.length > 1 ? str.length : ''))
.join('')
)
console.log(compressCharacters(a));
When you're trying to construct a new array by transforming all elements from some other array, .map
is the appropriate method to use. forEach
should be reserved for generic iteration with side effects, when you aren't trying to construct a new object. (when you are trying to construct a new object, but it's not an array, or the new array isn't one-to-one with the old array, usually you can use .reduce
)
add a comment |
You can construct and immediately return a map
ped array, that's joined by the empty string:
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => (
arr.map(str => str[0] + (str.length > 1 ? str.length : ''))
.join('')
)
console.log(compressCharacters(a));
When you're trying to construct a new array by transforming all elements from some other array, .map
is the appropriate method to use. forEach
should be reserved for generic iteration with side effects, when you aren't trying to construct a new object. (when you are trying to construct a new object, but it's not an array, or the new array isn't one-to-one with the old array, usually you can use .reduce
)
You can construct and immediately return a map
ped array, that's joined by the empty string:
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => (
arr.map(str => str[0] + (str.length > 1 ? str.length : ''))
.join('')
)
console.log(compressCharacters(a));
When you're trying to construct a new array by transforming all elements from some other array, .map
is the appropriate method to use. forEach
should be reserved for generic iteration with side effects, when you aren't trying to construct a new object. (when you are trying to construct a new object, but it's not an array, or the new array isn't one-to-one with the old array, usually you can use .reduce
)
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => (
arr.map(str => str[0] + (str.length > 1 ? str.length : ''))
.join('')
)
console.log(compressCharacters(a));
const a = ['aaa', 'z', 'eeeee'];
const compressCharacters = arr => (
arr.map(str => str[0] + (str.length > 1 ? str.length : ''))
.join('')
)
console.log(compressCharacters(a));
answered Nov 13 '18 at 1:18
CertainPerformanceCertainPerformance
81.3k143865
81.3k143865
add a comment |
add a comment |
This is an alternative using the function reduce
to build the desired output.
const a = ['aaa', 'z', 'eeeee'],
result = a.reduce((a, [m], i, arr) => {
let {length} = arr[i];
return a + m + (length <= 1 ? "" : length);
}, "");
console.log(result);
...............
– totalnoob
Nov 13 '18 at 4:38
can you explain how this works? I've never used an array in the reduce method before or deconstructed the length of an array like that before
– totalnoob
Nov 13 '18 at 4:42
add a comment |
This is an alternative using the function reduce
to build the desired output.
const a = ['aaa', 'z', 'eeeee'],
result = a.reduce((a, [m], i, arr) => {
let {length} = arr[i];
return a + m + (length <= 1 ? "" : length);
}, "");
console.log(result);
...............
– totalnoob
Nov 13 '18 at 4:38
can you explain how this works? I've never used an array in the reduce method before or deconstructed the length of an array like that before
– totalnoob
Nov 13 '18 at 4:42
add a comment |
This is an alternative using the function reduce
to build the desired output.
const a = ['aaa', 'z', 'eeeee'],
result = a.reduce((a, [m], i, arr) => {
let {length} = arr[i];
return a + m + (length <= 1 ? "" : length);
}, "");
console.log(result);
This is an alternative using the function reduce
to build the desired output.
const a = ['aaa', 'z', 'eeeee'],
result = a.reduce((a, [m], i, arr) => {
let {length} = arr[i];
return a + m + (length <= 1 ? "" : length);
}, "");
console.log(result);
const a = ['aaa', 'z', 'eeeee'],
result = a.reduce((a, [m], i, arr) => {
let {length} = arr[i];
return a + m + (length <= 1 ? "" : length);
}, "");
console.log(result);
const a = ['aaa', 'z', 'eeeee'],
result = a.reduce((a, [m], i, arr) => {
let {length} = arr[i];
return a + m + (length <= 1 ? "" : length);
}, "");
console.log(result);
edited Nov 13 '18 at 2:13
answered Nov 13 '18 at 1:29


EleEle
22.8k42044
22.8k42044
...............
– totalnoob
Nov 13 '18 at 4:38
can you explain how this works? I've never used an array in the reduce method before or deconstructed the length of an array like that before
– totalnoob
Nov 13 '18 at 4:42
add a comment |
...............
– totalnoob
Nov 13 '18 at 4:38
can you explain how this works? I've never used an array in the reduce method before or deconstructed the length of an array like that before
– totalnoob
Nov 13 '18 at 4:42
...............
– totalnoob
Nov 13 '18 at 4:38
...............
– totalnoob
Nov 13 '18 at 4:38
can you explain how this works? I've never used an array in the reduce method before or deconstructed the length of an array like that before
– totalnoob
Nov 13 '18 at 4:42
can you explain how this works? I've never used an array in the reduce method before or deconstructed the length of an array like that before
– totalnoob
Nov 13 '18 at 4:42
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272339%2frefactoring-function-with-different-methods-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
h rkrev,Oy,5RtKGa,LCMCBV,5s0hpl90ccV8s4zDEWqA